JavaScript Prototypes: A Beginner's Guide (Lesson 2)
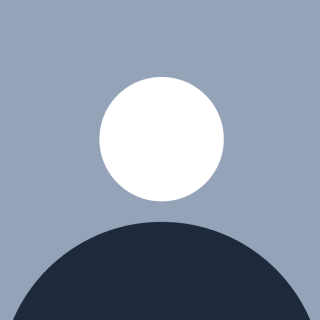
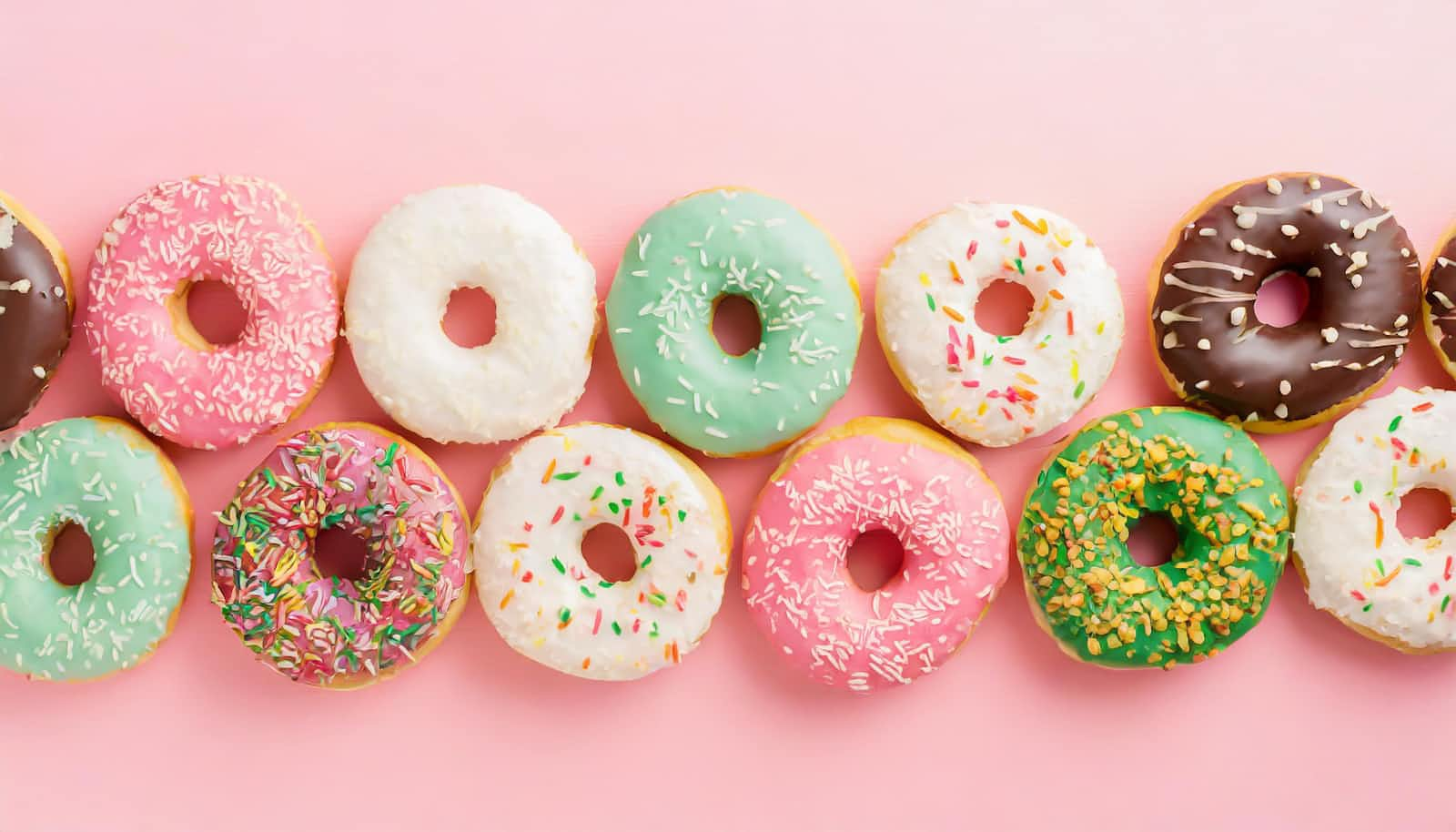
Back in Action with JS Prototypes
It's been a while since my last blog post but glad to be back in action to show you all how to create prototypes :)
Since this blog mentions a cafe in its name, it's only natural for us to create a prototype for a dessert.
- Define the Constructor Function
We start by defining a Dessert class using the function keyword. This constructor function sets the properties of the dessert:
function Dessert(name, sugar, rating) {
this.name = name;
this.sugar = sugar;
this.rating = rating;
}
- Create a Prototype Object
Next, we create a dessertPrototype object. This prototype object contains methods that we want to share among all dessert instances. For example, we define a method to print a statement based on the dessert's rating:
const dessertPrototype = {
giveRating: function() {
if (this.rating > 6) {
console.log(`${this.name} is amazing`);
} else {
console.log(`${this.name} is ok`); }
}
};
- Create a New Dessert Object
To create a dessert object that uses the dessertPrototype, we link it to the prototype and then add the necessary properties. Here's how to do it:
newDessert = Object.create(dessertPrototype);
newDessert.name = "Cake";
newDessert.sugar = 30;
newDessert.rating = 5
The above code is basically similar to the E6 syntax of const newDessert = new Dessert("Cake", 30, 5);
Lastly, we call the giveRating method on the newDessert object
newDessert.giveRating()
The method works! Since
dessertPrototype
is the prototype ofnewDessert
, we are able to call thegiveRating
method on the newDessert object.
We just created our first prototype and was able to link to newDessert. Congrats!. Now give yourself the actual reward of a dessert.
Subscribe to my newsletter
Read articles from Coding Cafe directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by