Learn How to Easily Handle Forms with JavaScript
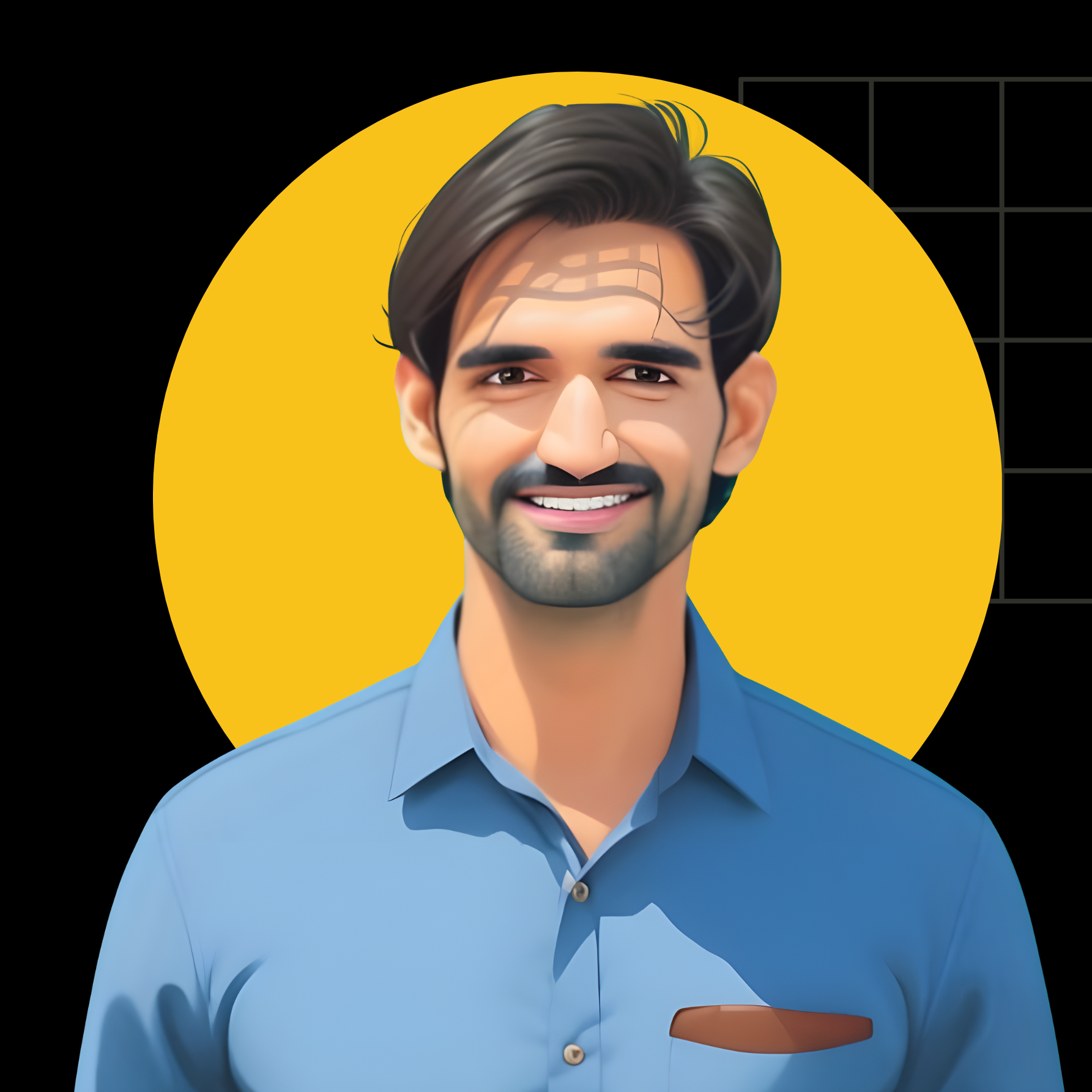
Table of contents
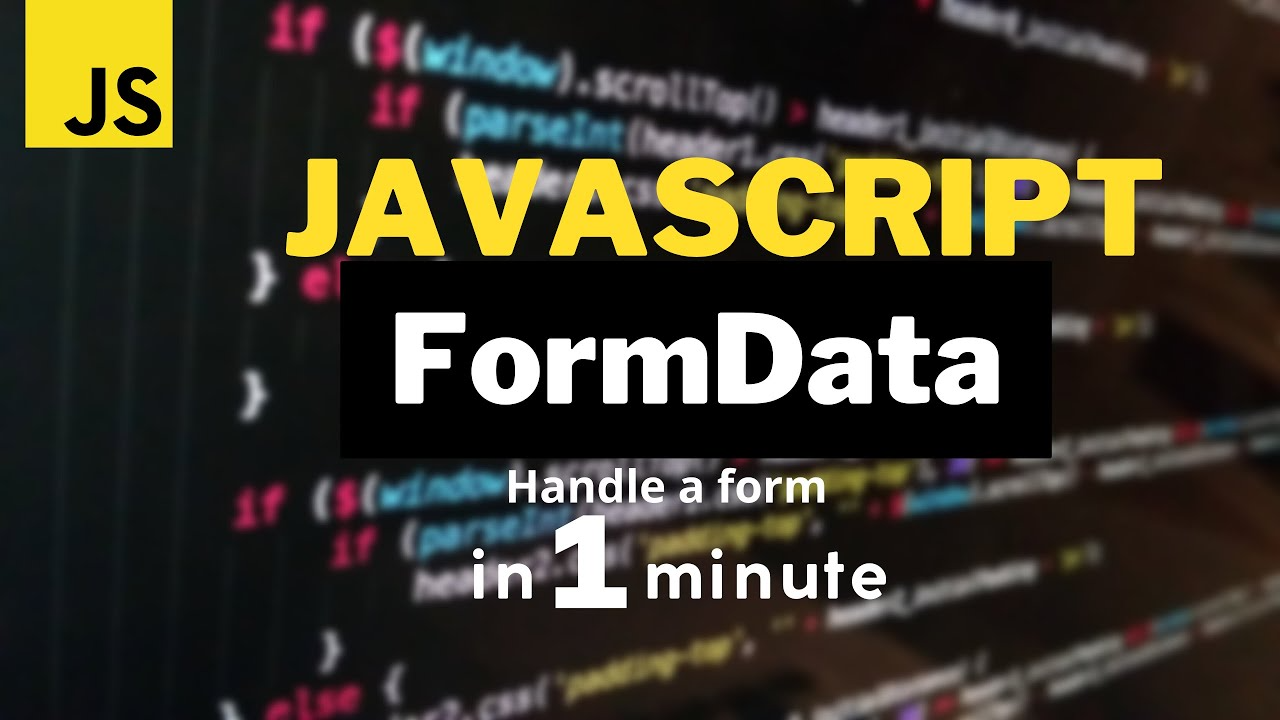
In modern web development, handling user input effectively is crucial for creating dynamic and interactive forms. One common challenge developers face is managing form data when multiple checkboxes are involved. In this article, we'll walk you through a practical example of how to capture and display all selected checkbox values using JavaScript, ensuring a smooth user experience and accurate data collection.
Understanding the Problem
When users interact with forms that include checkboxes, it's essential to capture all their selections. A typical issue arises when multiple checkboxes share the same name
attribute; by default, browsers only retain the last checked value. To address this, we need a solution that ensures all selected values are recorded and processed correctly.
Solution Overview
We will use a combination of HTML and JavaScript to create a form and handle its submission. Our goal is to gather all selected checkbox values and include them in our data object. Here’s a step-by-step guide:
Create the HTML Form
First, we set up a simple HTML form with various input types, including text fields, radio buttons, and checkboxes.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Form Handling Example</title> </head> <body> <form onsubmit="handleSubmit(event)"> <input type="text" placeholder="name" name="name" /> <input type="text" placeholder="email" name="email" /> <input type="text" placeholder="age" name="age" /> <!-- radio buttons --> <input type="radio" name="gender" value="male" /> Male <input type="radio" name="gender" value="female" /> Female <br /> <!-- checkboxes --> <input type="checkbox" name="interests" value="sports" /> Sports <input type="checkbox" name="interests" value="music" /> Music <input type="checkbox" name="interests" value="reading" /> Reading <br /> <button type="submit">Submit</button> </form> </body> </html>
JavaScript for Handling Form Submission
Next, we need JavaScript to handle form submission, process the form data, and ensure that all selected checkbox values are included.
<script> function handleSubmit(event) { event.preventDefault(); // Prevent the default form submission let form = event.target; let formData = new FormData(form); // Create an object from FormData entries let formObj = Object.fromEntries(formData.entries()); // Process checkboxes to ensure all values are included let interests = formData.getAll("interests"); if (interests.length > 0) { formObj["interests"] = interests; } console.log(formObj); alert(JSON.stringify(formObj)); // Convert the object to a JSON string } </script>
Key Points
FormData API: The
FormData
API allows us to easily retrieve form data, including handling multiple values for checkboxes.Handling Checkbox Values: By using
formData.getAll("interests")
, we can capture all selected checkbox values and store them in an array.Converting to Object: We convert the
FormData
entries to a plain object and then manually add the array of interests, ensuring our form data is comprehensive.
Conclusion
By following this guide, you'll be equipped to handle similar form data challenges in your projects, creating more robust and user-friendly web applications.
Subscribe to my newsletter
Read articles from Zeeshan Mukhtar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
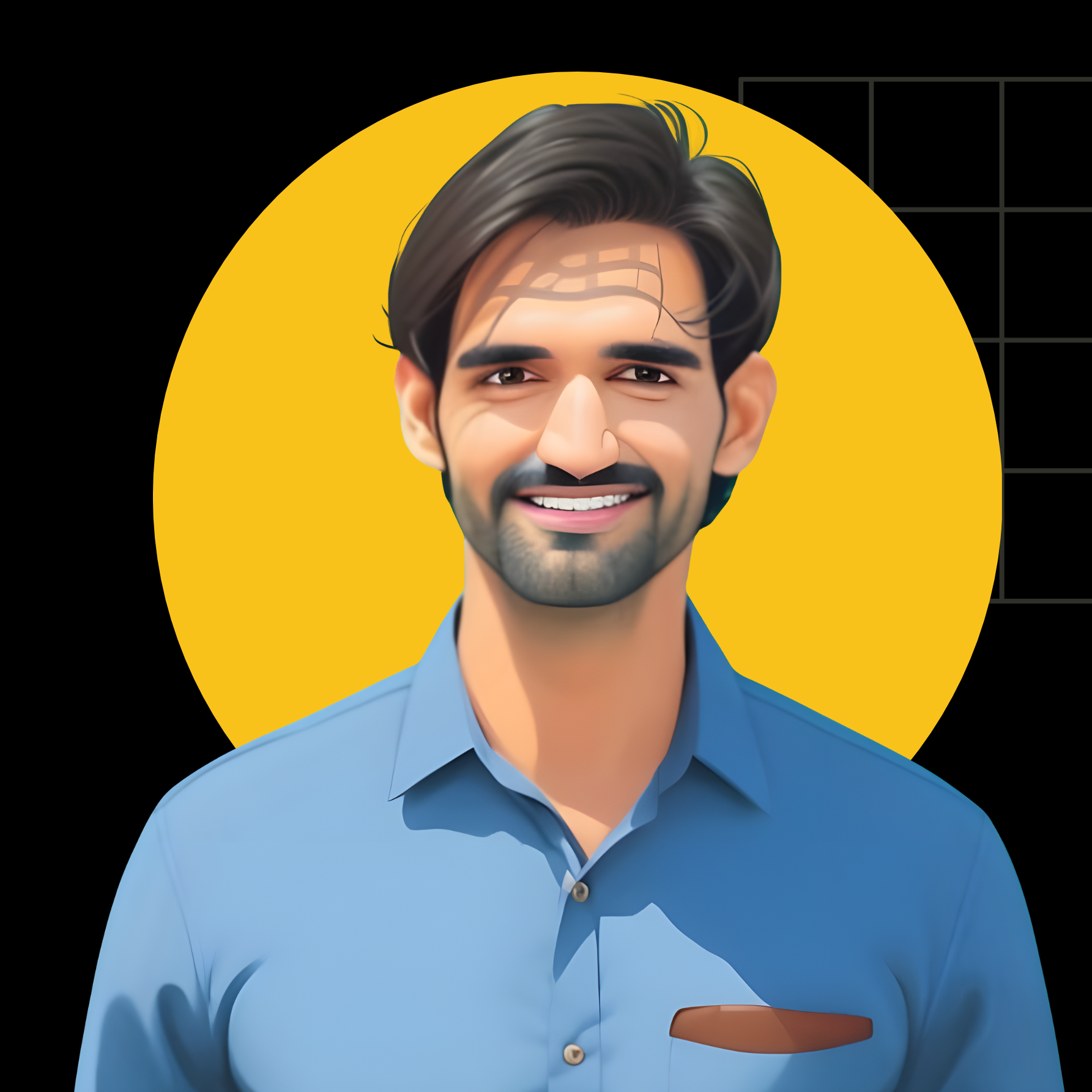
Zeeshan Mukhtar
Zeeshan Mukhtar
Tech enthusiast and community builder, sharing knowledge through articles. Let's learn, grow, and support each other. 🌟 #CodingForAll 🤝