Implementing Versioning and Documentation for ASP.NET Core Web APIs
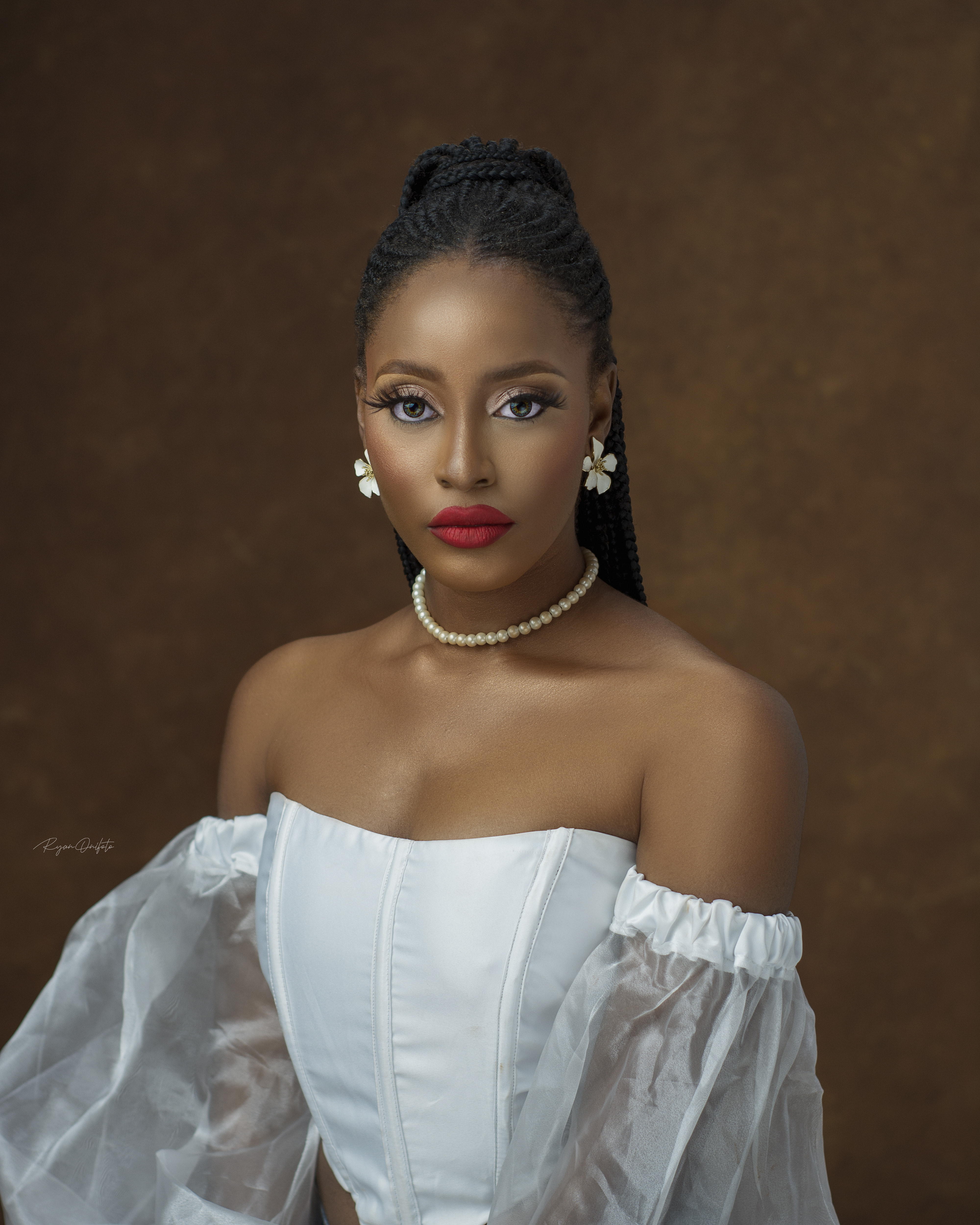
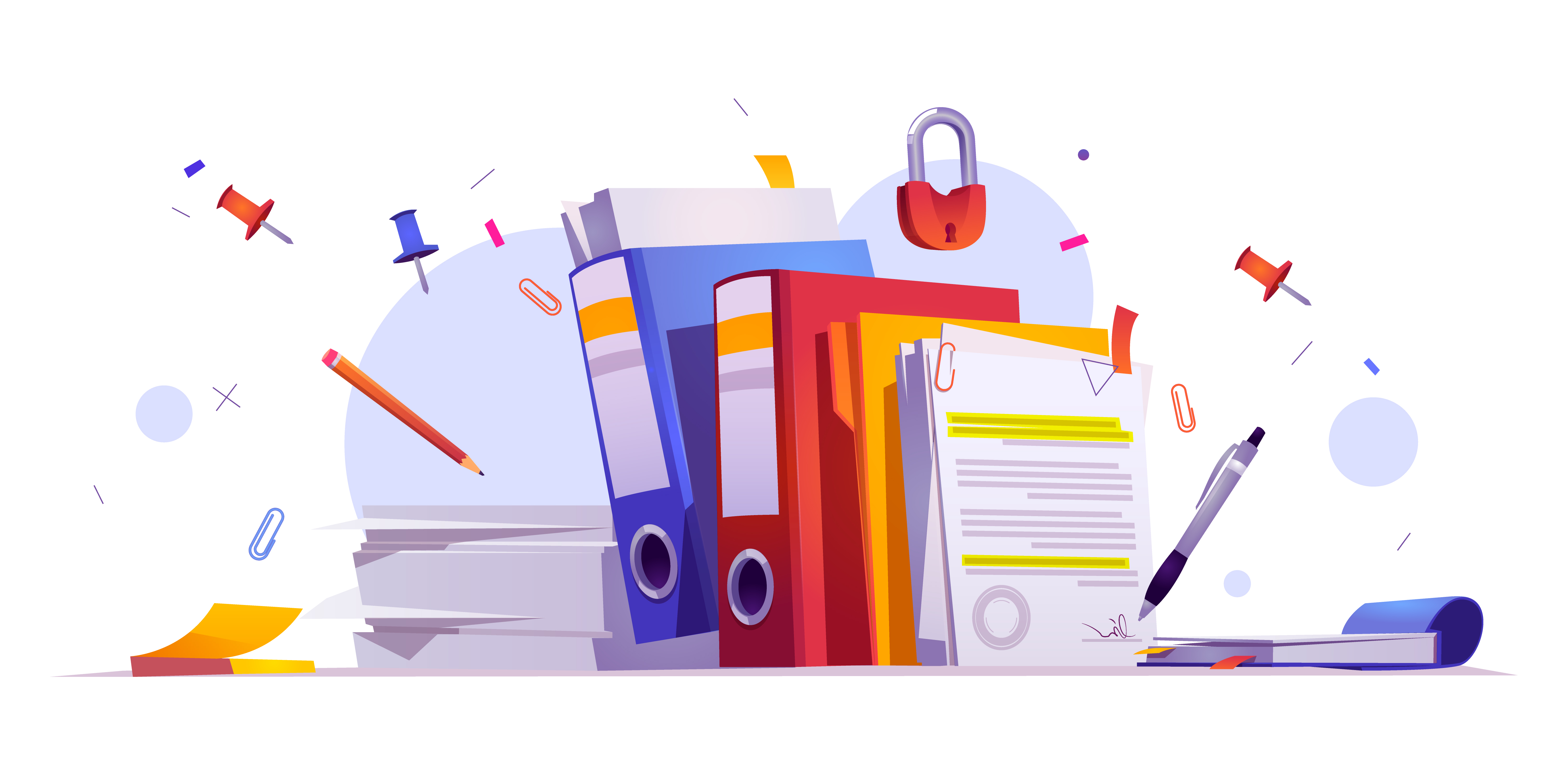
Welcome to the 10th installment of the Mastering C# series! Whoop Whoop! I am incredibly proud of all the technical guides and tutorials we've covered so far.
Today’s article is a beginner’s guide to versioning and documentingASP.NET.Core APIs. As a documentation engineer, I find this topic particularly important. Stay tuned, as this guide promises to be packed with valuable tips and tricks!
Pre-requisites
To fully benefit from this article, readers should have the following prerequisites:
Basic Knowledge ofASP.NETCore
Understanding of Web API Fundamentals
Basic concepts of RESTful APIs
Knowledge of HTTP methods (GET, POST, PUT, DELETE)
Familiarity with JSON data format
C# Programming Skills
Basic to intermediate knowledge of C# programming
Experience with object-oriented programming concepts
Development Environment Setup
Visual Studio or Visual Studio Code installed
.NET SDK installed (latest version recommended)
Familiarity with Dependency Injection
- Basic understanding of dependency injection and its usage in ASP.NET Core
Experience with NuGet Package Manager
- Ability to install and manage NuGet packages in a .NET project
Basic Understanding of Version Control Systems
- Familiarity with Git for source control management (optional but recommended)
Table of Contents
Introduction to API Versioning and Documentation
Versioning Strategies for Evolving APIs Without Breaking Changes
Implementing Versioning in ASP.NET Core
Documenting Web APIs Using Swagger/OpenAPI Specifications
Generating Interactive API Documentation with Swagger UI
Advanced Documentation Features
Testing and Maintaining API Documentation
Best Practices and Tips
Conclusion
Introduction to API Versioning and Documentation
Importance of API Versioning and Documentation
When you build and improve web APIs, it is essential to manage changes without breaking existing functionality for users. This is where API versioning comes in. It allows you to update and improve your API while keeping old versions available for users who need them. Good documentation is equally important because it helps developers understand how to use your API, making their work easier and more efficient.
Overview of ASP.NET Core Web APIs
ASP.NET Core is a powerful framework for building web applications, including APIs. With ASP.NET Core, you can create APIs that are fast, secure, and scalable. These APIs can handle requests from various clients, like web browsers, mobile apps, and other services, making it an excellent choice for modern web development.
Versioning Strategies for Evolving APIs Without Breaking Changes
When you update your API, you want to ensure that existing users aren't affected. Here are some common strategies to version your API:
URI Versioning
What it is: Adding the version number directly into the URL.
Example:
https://api.example.com/v1/products
Benefits: Easy to understand and implement.
Query String Versioning
What it is: Adding the version number as a query parameter in the URL.
Benefits: Keeps the URL structure clean and simple.
Header Versioning
What it is: Sending the version number in the HTTP headers of the request.
Example:
GET
https://api.example.com/products
withHeader: api-version: 1
Benefits: Keeps the URL clean and allows for more flexible versioning.
Media Type Versioning
What it is: Using the
Accept
header to specify the version.Example:
Accept: application/vnd.example.v1+json
Benefits: Allows for fine-grained control over different versions and formats.
Best Practices for API Versioning
Be Consistent:
Choose one versioning strategy and stick with it across your entire API.Document Clearly:
Make sure your documentation explains how to use the versioning system.Communicate Changes:
Inform users well in advance before deprecating older versions.Keep It Simple:
Don’t overcomplicate your versioning strategy; it should be easy for users to understand and use.
Implementing Versioning in ASP.NET Core
Versioning is essential for evolving your API without breaking existing clients. Here's how to set it up in a simple way:
Setting up Versioning in ASP.NET Core
Create a NewASP.NETCore Web API Project
Open Visual Studio or Visual Studio Code.
Create a new ASP.NET Core Web API project.
Install the Versioning Package
Open the NuGet Package Manager.
Search for and install
Microsoft.AspNetCore.Mvc.Versioning
.
Using Microsoft.AspNetCore.Mvc.Versioning
Configure Versioning in Startup.cs
Open
Startup.cs
.Add the versioning service in the
ConfigureServices
method:
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
// Add API versioning
services.AddApiVersioning(options =>
{
options.AssumeDefaultVersionWhenUnspecified = true;
options.DefaultApiVersion = new ApiVersion(1, 0);
options.ReportApiVersions = true;
});
}
Version Your Controllers
Open or create a controller (e.g.,
WeatherForecastController
).Add versioning attributes:
[ApiController]
[Route("api/v{version:apiVersion}/[controller]")]
[ApiVersion("1.0")]
public class WeatherForecastController : ControllerBase
{
[HttpGet]
public IActionResult GetV1()
{
return Ok("Weather forecast v1.0");
}
}
[ApiController]
[Route("api/v{version:apiVersion}/[controller]")]
[ApiVersion("2.0")]
public class WeatherForecastV2Controller : ControllerBase
{
[HttpGet]
public IActionResult GetV2()
{
return Ok("Weather forecast v2.0");
}
}
Examples and Code Walkthrough
Define the Default API Version
- In the
AddApiVersioning
configuration, you set the default version to 1.0. This means if no version is specified, version 1.0 will be used.
- In the
Accessing Versioned Endpoints
To access the versioned endpoints, use the following URLs:
Version 1.0:
https://localhost:5001/api/v1/WeatherForecast
Version 2.0:
https://localhost:5001/api/v2/WeatherForecast
Testing the Versioning
Run your application.
Use a browser or tool like Postman to test the endpoints.
You should see different responses based on the version specified in the URL.
Documenting Web APIs Using Swagger/OpenAPI Specifications
Introduction to Swagger and OpenAPI
Swagger and OpenAPI are tools that help you document your APIs. They make it easy to understand what your API does and how to use it. Swagger provides a user-friendly interface to interact with your API, while OpenAPI is a standard format for describing APIs.
Setting up Swashbuckle in ASP.NET Core
Swashbuckle is a library that integrates Swagger with ASP.NET Core. Here's how to set it up:
Install the Swashbuckle Package:
Open your project in Visual Studio.
Install the Swashbuckle.AspNetCore package using NuGet Package Manager.
dotnet add package Swashbuckle.AspNetCore
Configure Swashbuckle:
- Open
Startup.cs
and add Swashbuckle in theConfigureServices
method.
- Open
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddSwaggerGen();
}
- Add the Swagger middleware in the
Configure
method.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseSwagger();
app.UseSwaggerUI(c =>
{
c.SwaggerEndpoint("/swagger/v1/swagger.json", "My API V1");
c.RoutePrefix = string.Empty;
});
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
Run Your Project:
- Start your project and navigate to
http://localhost:<port>/swagger
. You should see the Swagger UI with your API documentation.
- Start your project and navigate to
Annotating API Methods for Documentation
To make your API documentation more informative, you can add annotations to your API methods. These annotations describe what each method does, its parameters, and responses.
Add Annotations to Your Controller Methods:
using Microsoft.AspNetCore.Mvc; using System.Collections.Generic; using Swashbuckle.AspNetCore.Annotations; [ApiController] [Route("api/[controller]")] public class WeatherForecastController : ControllerBase { [HttpGet] [SwaggerOperation(Summary = "Gets all weather forecasts", Description = "Returns a list of weather forecasts for the next 5 days.")] [SwaggerResponse(200, "Success", typeof(IEnumerable<WeatherForecast>))] public IEnumerable<WeatherForecast> Get() { // Your code here } }
Build and Run:
- Rebuild your project and check the Swagger UI to see the updated documentation with the annotations.
Customizing Swagger Metadata
You can customize the metadata that Swagger displays, such as the API title, description, and version.
Update the Swagger Configuration:
- In the
ConfigureServices
method inStartup.cs
, update theAddSwaggerGen
method to include custom metadata.
- In the
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo
{
Title = "My API",
Version = "v1",
Description = "An example API for demonstrating Swagger",
Contact = new OpenApiContact
{
Name = "Your Name",
Email = "your-email@example.com"
}
});
});
Rebuild and Run:
- After making these changes, rebuild and run your project again. The Swagger UI should now display the custom metadata you provided.
Generating Interactive API Documentation with Swagger UI
Swagger UI is a powerful tool that allows you to visualize and interact with your API. Let's break down how to use it.
1. Integrating Swagger UI with ASP.NET Core
First, we'll add Swagger UI to your ASP.NET Core project. Follow these steps:
Install the Swashbuckle.AspNetCore package:
Open your project in Visual Studio or Visual Studio Code.
In the terminal or Package Manager Console, run:
dotnet add package Swashbuckle.AspNetCore
Configure Swagger in your
Startup.cs
file:Open
Startup.cs
and add the following lines to theConfigureServices
method:public void ConfigureServices(IServiceCollection services) { services.AddControllers(); services.AddSwaggerGen(); }
Enable Swagger in the HTTP request pipeline:
In the
Configure
method ofStartup.cs
, add:public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); app.UseSwagger(); app.UseSwaggerUI(c => c.SwaggerEndpoint("/swagger/v1/swagger.json", "My API V1")); } app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); }
Run your application:
Start your ASP.NET Core project and navigate to
http://localhost:5000/swagger
in your browser.You should see the Swagger UI with your API documentation.
2. Customizing Swagger UI Appearance and Behavior
You can customize the look and feel of Swagger UI to match your needs. Here’s how:
Change the Swagger UI settings:
In the
Configure
method where you set upSwaggerUI
, you can customize its settings:app.UseSwaggerUI(c => { c.SwaggerEndpoint("/swagger/v1/swagger.json", "My API V1"); c.RoutePrefix = string.Empty; // Set Swagger UI at the app's root c.DocumentTitle = "My API Documentation"; // Set the page title c.InjectStylesheet("/swagger-ui/custom.css"); // Add custom CSS });
Add a custom stylesheet:
Create a custom CSS file in your project (e.g.,
wwwroot/swagger-ui/custom.css
) and add your styles:.swagger-ui .topbar { background-color: #4CAF50; /* Change the top bar color */ }
3. Using Swagger UI to Test API Endpoints
Swagger UI makes it easy to test your API endpoints directly from the browser.
Explore the API documentation:
Open Swagger UI (
http://localhost:5000/swagger
).You will see a list of all your API endpoints.
Test an endpoint:
Click on any endpoint to expand it.
You will see details about the endpoint, including parameters and responses.
Click the "Try it out" button.
Fill in any required parameters and click "Execute".
You will see the response from the API directly in the browser.
Advanced Documentation Features
In this section, we'll cover some advanced features to make your API documentation even more useful. These features include adding authentication information, documenting API versioning, and generating client SDKs from OpenAPI specifications.
Adding Authentication Information
When your API requires authentication, it's important to include this information in your documentation. This helps users understand how to securely access your API.
Add Security Definitions: Define the type of authentication your API uses (e.g., Bearer Token, API Key).
Annotate Endpoints: Specify which endpoints require authentication and how to provide the necessary credentials.
Example in Swagger:
services.AddSwaggerGen(c =>
{
c.AddSecurityDefinition("Bearer", new OpenApiSecurityScheme
{
In = ParameterLocation.Header,
Description = "Please enter token",
Name = "Authorization",
Type = SecuritySchemeType.ApiKey,
BearerFormat = "JWT",
Scheme = "Bearer"
});
c.AddSecurityRequirement(new OpenApiSecurityRequirement
{
{
new OpenApiSecurityScheme
{
Reference = new OpenApiReference
{
Type = ReferenceType.SecurityScheme,
Id = "Bearer"
}
},
new string[] {}
}
});
});
Documenting API Versioning in Swagger
If your API supports multiple versions, it's helpful to document each version clearly. This helps users know which version of the API to use and what features are available in each version.
Group Endpoints by Version: Organize your endpoints by version in the Swagger UI.
Annotate Controllers: Use attributes to specify the version for each controller.
Example in Swagger:
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo { Title = "API V1", Version = "v1" });
c.SwaggerDoc("v2", new OpenApiInfo { Title = "API V2", Version = "v2" });
});
Generating Client SDKs from OpenAPI Specifications
You can generate client SDKs from your OpenAPI specification to make it easier for developers to interact with your API in various programming languages.
Generate SDKs: Use tools like Swagger Codegen or OpenAPI Generator to create client libraries.
Provide SDKs: Make the generated SDKs available to your users, along with usage instructions.
Example:
Generate a client SDK using OpenAPI Generator:
openapi-generator-cli generate -i swagger.json -g csharp -o ./generated-client
Share the SDK with your users and provide instructions on how to integrate it into their projects.
By adding these advanced documentation features, you make your API more accessible and easier to use for developers, enhancing the overall user experience.
Testing and Maintaining API Documentation
Creating great API documentation is just the beginning. It is equally important to keep it accurate and up-to-date as your API evolves. Here’s how you can ensure your documentation remains reliable:
Keeping Documentation Up-to-Date with API Changes
Whenever you update your API, make sure to update the documentation too. This can be as simple as adding new endpoints, updating existing ones, or noting any deprecated features. Keeping a habit of documenting changes as you go can save you a lot of trouble later.
Automated Testing of API Documentation
Automated testing helps ensure that your API and its documentation are in sync. Use tools that automatically generate tests based on your documentation. This way, if an API endpoint is updated or changed, your tests will fail, indicating that your documentation needs an update.
Tools for Continuous Integration and Documentation Deployment
Continuous Integration (CI) tools can help automate the process of testing and deploying your documentation. Whenever you push code changes to your repository, CI tools can run tests to check if the documentation is still accurate and then automatically deploy the latest version.
Popular Tools for API Documentation Testing and CI:
Swagger Validator:
Validates your OpenAPI/Swagger specifications to ensure they are correct.Postman:
A powerful tool for testing your API endpoints and ensuring they match the documentation.GitHub Actions:
Automate your CI/CD workflows, including testing and deploying documentation.Travis CI / Jenkins:
Other CI tools that can be set up to automate testing and deployment processes.
Best Practices and Tips
Ensuring Consistency in Documentation
Use Standard Formats:
Stick to a consistent format for your API documentation. Tools like Swagger/OpenAPI can help maintain this.Regular Updates:
Whenever you change your API, update the documentation immediately to keep it accurate.Clear Descriptions:
Write clear and concise descriptions for each endpoint, parameter, and response to make it easy to understand.
Handling Deprecated Endpoints
Mark Deprecated Endpoints:
Clearly mark any endpoints that are deprecated in your documentation. Use annotations or notes to indicate this.Provide Alternatives:
Suggest newer or preferred endpoints that users should use instead.Set a Timeline:
Inform users when the deprecated endpoints will be removed so they have time to update their applications.
Examples from Real-World Applications
Show Real Examples:
Include examples from actual applications to demonstrate how to use the API. This helps users see how it works in practice.Sample Code:
Provide sample code snippets in various programming languages to show how to make requests to your API.Use Cases:
Describe common use cases and scenarios where your API can be used, making it easier for users to understand its benefits and applications.
Conclusion
Congratulations on reaching the end of our beginner's guide to versioning and documenting ASP.NET Core APIs! Let's quickly recap the key points we covered:
Versioning Strategies:
We learned about different ways to version your APIs, ensuring you can make changes without breaking existing clients.Swagger and OpenAPI:
We explored how to use these tools to create clear and interactive documentation for your APIs.Practical Implementation:
We walked through setting up versioning and documentation in an ASP.NET Core project, step-by-step.
Remember, versioning and documenting your APIs not only helps you manage changes but also makes it easier for others to understand and use your APIs effectively.
Additional Resources
To continue learning and improving your skills, here are some great resources:
ASP.NETCore Documentation:
Microsoft's officialASP.NETCore documentation is a comprehensive resource for all things ASP.NET Core.Swagger/OpenAPI Documentation:
The Swagger and OpenAPI official documentation provide in-depth guides and examples.Online Tutorials:
Websites like Pluralsight, Udemy, and Codecademy offer courses on ASP.NET Core and API development.Community Forums:
Join communities like Stack Overflow and the ASP.NETCore GitHub repository to ask questions and share knowledge.
I hope you found this guide helpful and learned something new. Stay tuned for the next article in the "Mastering C#" series: Optimizing Performance in ASP.NET Core Web APIs: Caching, Compression, and Response Minification
Happy coding!
Subscribe to my newsletter
Read articles from Opaluwa Emidowo-ojo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
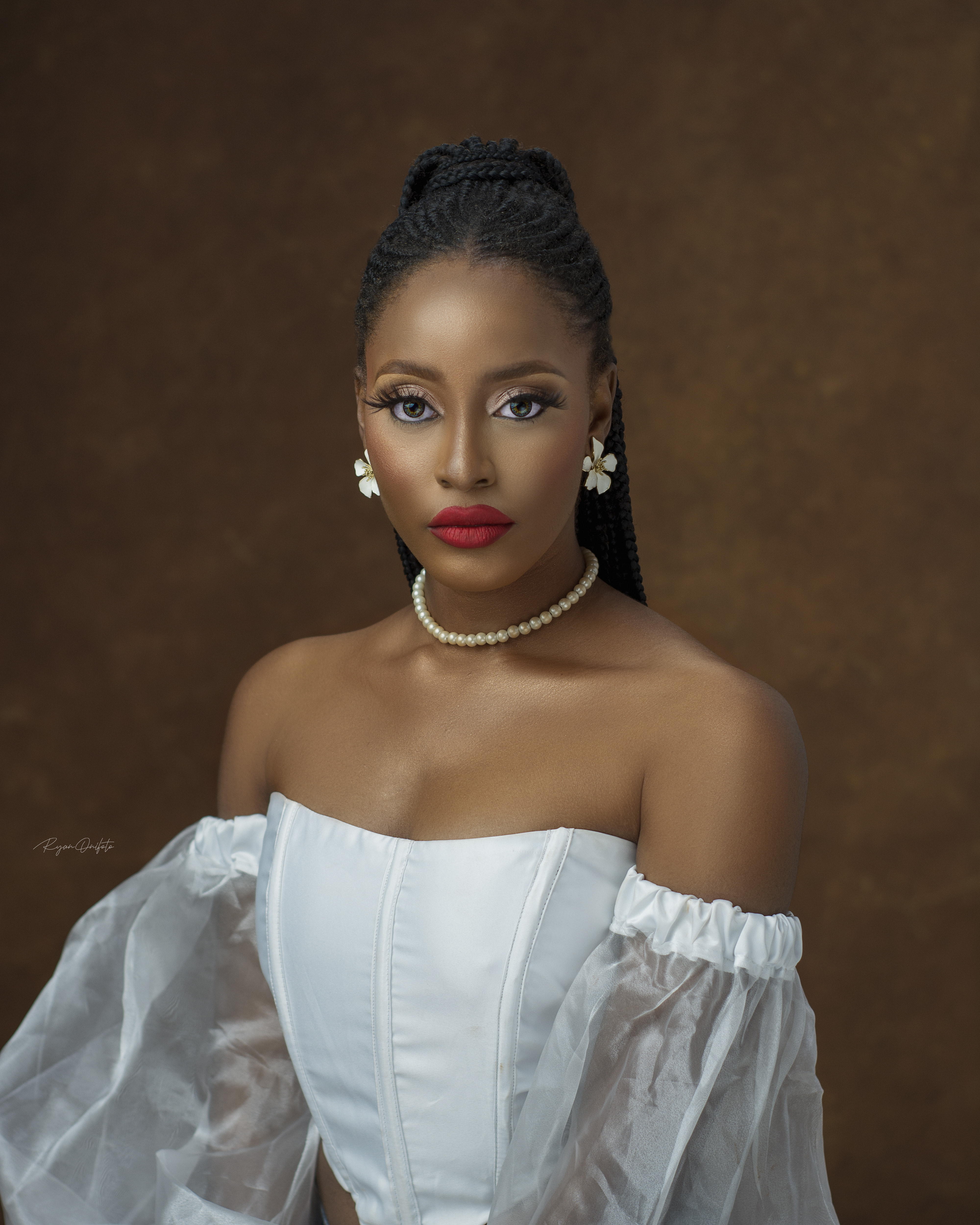