Web Components: The Secret Weapon You're Not Using (Yet)
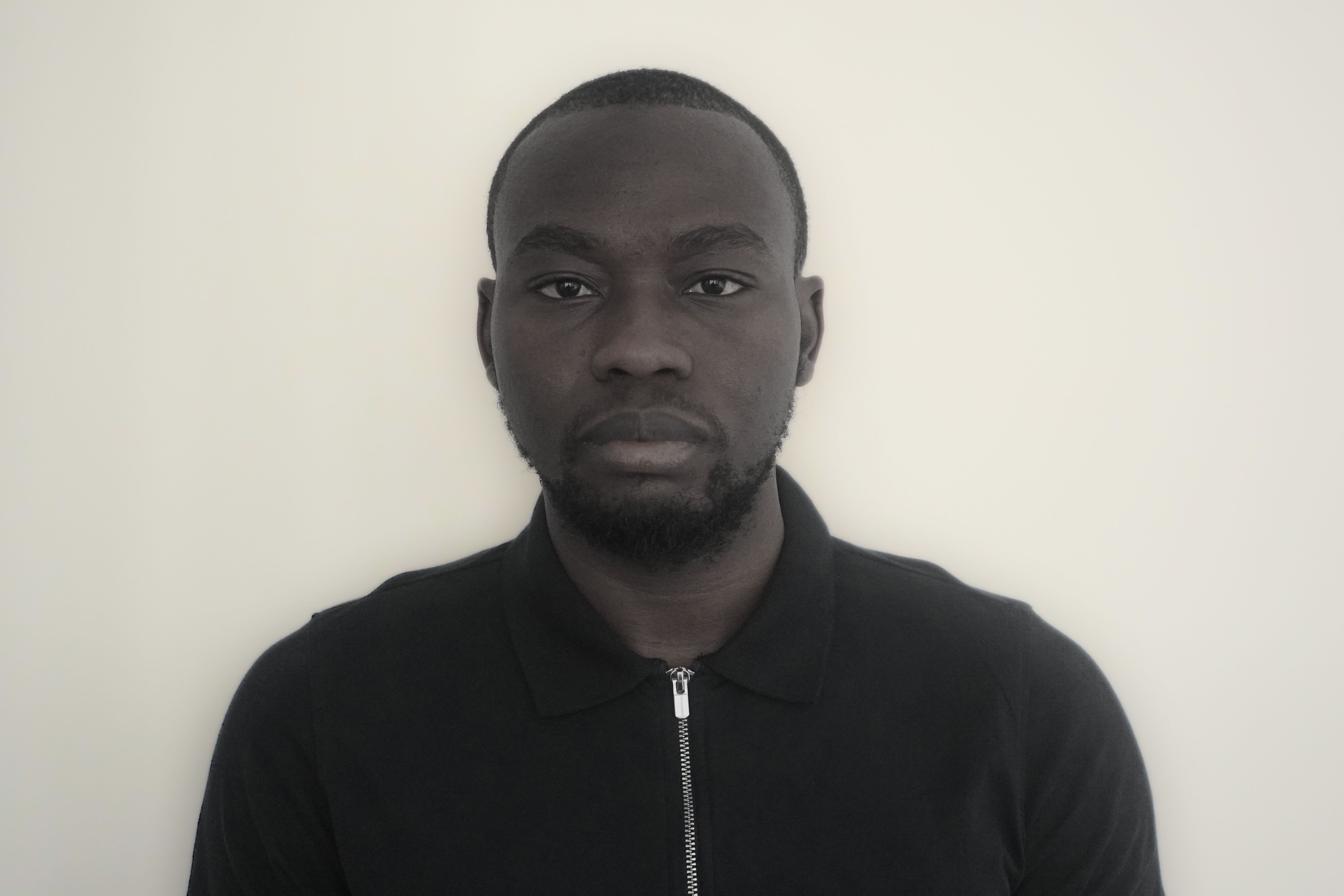
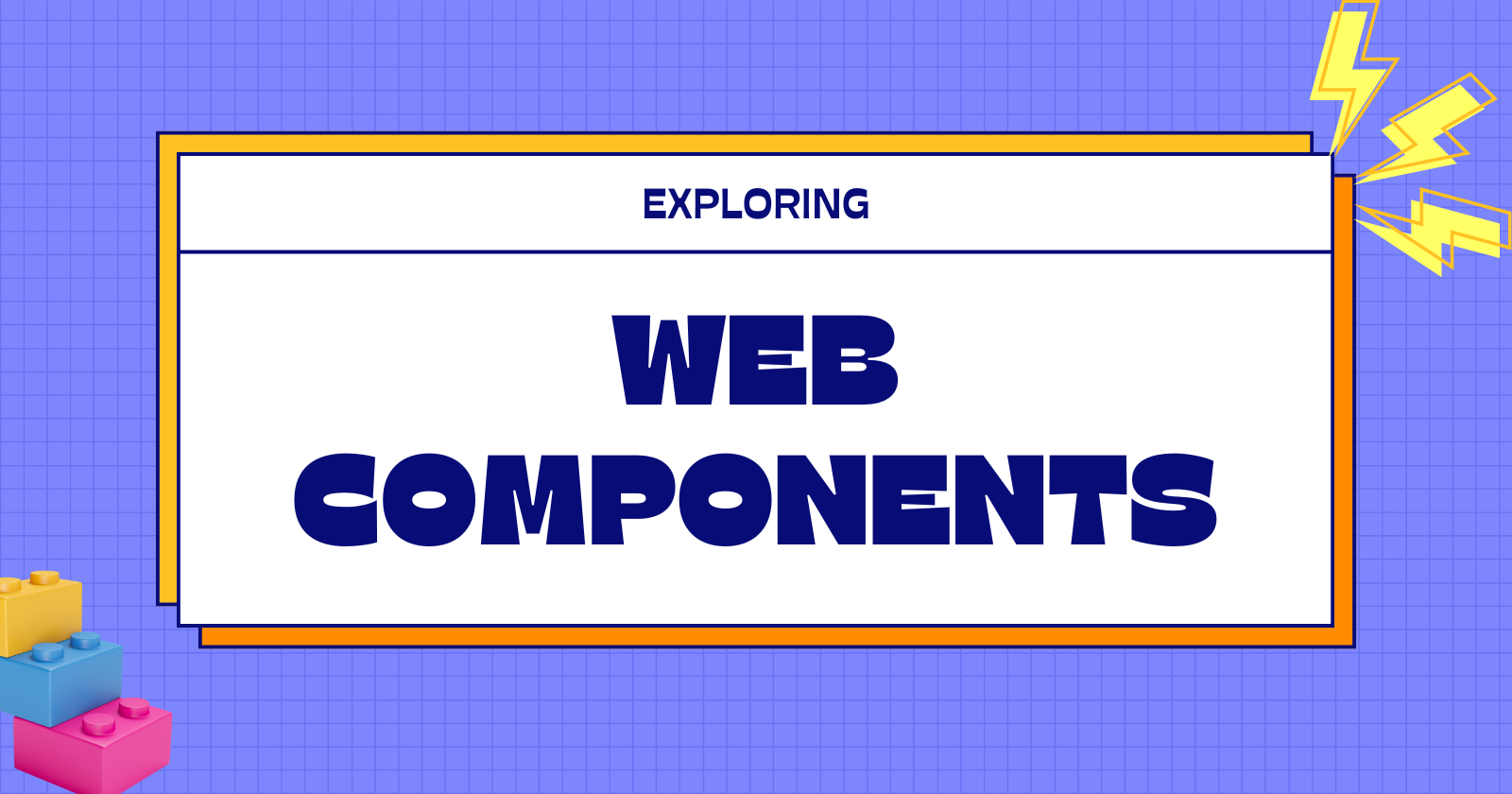
Hey there, fellow code wrangler ๐๐ฝ. Remember the last time you had to rebuild that same dropdown menu for the umpteenth time? Or when your carefully crafted CSS got steamrolled by some rogue styles from who-knows-where? If you're nodding along, it's time we had a talk about web components.
What's the Deal with Web Components? ๐ค
Web components are like that one friend who's got their life together. They're self-contained, know how to play nice with others, and don't leave a mess for you to clean up. In dev speak, they're custom HTML elements that encapsulate their own HTML, CSS, and JavaScript.
But why should you care? Well, imagine never having to worry about your styles leaking or your JavaScript conflicting with other parts of your app. Sounds too good to be true, right? Stick with me, and I'll show you it's not.
The Building Blocks Web components are built on three key technologies:
Web components are built on three key technologies:
Custom Elements
Shadow DOM
HTML Templates
Let's break these down with some code that'll make you go, "Huh, that's pretty neat."
Custom Elements: Your HTML, Your Rules
Ever wanted to create your own HTML tag? You can with custom elements. Check this out:
class CoolButton extends HTMLElement {
connectedCallback() {
this.innerHTML = `<button>I'm cooler than the other buttons</button>`;
}
}
customElements.define('cool-button', CoolButton);
Now you can use <cool-button></cool-button>
in your HTML. Just like that, you've extended HTML. Feel the power!
Shadow DOM: Where CSS Goes to Behave
Shadow DOM is like a bouncer but for your component's styles. It keeps them in and everyone else's out. Here's how it works:
class StyledComponent extends HTMLElement {
constructor() {
super();
this.attachShadow({mode: 'open'});
this.shadowRoot.innerHTML = `
<style>
p { color: red; font-weight: bold; }
</style>
<p>
I'm red and bold, and I won't let any other styles
tell me otherwise!
</p>
`;
}
}
customElements.define('styled-component', StyledComponent);
Try overriding that red text from your main CSS. Go on, I'll wait. (Spoiler: you can't.)
HTML Templates: Copy-Paste on Steroids
Templates let you define a chunk of HTML that you can use over and over. It's like copy-paste, but way cooler:
<template id="my-template">
<h2>Welcome to my world</h2>
<p>Where the code is clean and the components are reusable</p>
</template>
<script>
class TemplateComponent extends HTMLElement {
constructor() {
super();
const template = document.getElementById('my-template');
const content = template.content.cloneNode(true);
this.attachShadow({mode: 'open'}).appendChild(content);
}
}
customElements.define('template-component', TemplateComponent);
</script>
A Todo List That Doesn't Suck
Let's create something useful - a todo list that won't make you pull your hair out:
class SuperTodoList extends HTMLElement {
constructor() {
super();
this.attachShadow({mode: 'open'});
this.todos = JSON.parse(localStorage.getItem('todos')) || [];
this.render();
}
render() {
this.shadowRoot.innerHTML = `
<style>
:host { display: block; font-family: sans-serif; max-width: 300px;
margin: 0 auto; }
input, button { font-size: 1rem; padding: 5px; margin: 5px 0; }
ul { padding-left: 20px; }
li { margin: 10px 0; }
.done { text-decoration: line-through; color: #888; }
</style>
<h2>Get Stuff Done</h2>
<input type="text" id="new-todo" placeholder="What needs doing?">
<button id="add-todo">Add</button>
<ul id="todo-list"></ul>
`;
this.updateTodoList();
this.shadowRoot.getElementById('add-todo').addEventListener('click',
() => this.addTodo());
}
addTodo() {
const input = this.shadowRoot.getElementById('new-todo');
if (input.value.trim()) {
this.todos.push({text: input.value.trim(), done: false});
input.value = '';
this.updateTodoList();
}
}
updateTodoList() {
const list = this.shadowRoot.getElementById('todo-list');
list.innerHTML = this.todos.map((todo, index) => `
<li class="${todo.done ? 'done' : ''}">
<input type="checkbox" ${todo.done ? 'checked' : ''}
data-index="${index}">
${todo.text}
</li>
`).join('');
list.addEventListener('change', (e) => {
if (e.target.type === 'checkbox') {
this.toggleTodo(parseInt(e.target.dataset.index));
}
});
localStorage.setItem('todos', JSON.stringify(this.todos));
}
toggleTodo(index) {
this.todos[index].done = !this.todos[index].done;
this.updateTodoList();
}
}
customElements.define('super-todo-list', SuperTodoList);
Now you've got a todo list that remembers your items, lets you check them off, and doesn't care what CSS framework you're using. Drop this bad boy anywhere in your HTML:
<super-todo-list></super-todo-list>
Why You'll Love Web Components
Reusability: Build it once, use it everywhere. Your future self will thank you.
Encapsulation: Your component's internals are nobody else's business.
Framework Agnostic: React, Vue, Angular? Web components don't care. They play nice with everyone.
Future-Proof: They're based on web standards. They're not going anywhere.
The Road Ahead
Sure, web components aren't all sunshine and rainbows. You might hit some bumps:
Browser Support: Most modern browsers are on board, but for those IE stragglers, you might need polyfills.
Learning Curve: It's a new way of thinking, but hey, remember when you first learned flexbox?
State Management: For complex apps, you might want to bring in some help (Redux, I'm looking at you).
But don't let that stop you. Web components aren't just a passing trend - they're a fundamental part of modern web development. If you haven't jumped on board yet, now's the perfect time to start your journey.
Your Next Move
Web components aren't just changing the game - they've become an integral part of it. While they've been around for years, many developers are still discovering new ways to leverage their power. They're not a silver bullet, but when used effectively, they can significantly improve code organization, reusability, and maintainability.
So, what are you waiting for? Dive in. Experiment. Break things (in a development environment, of course). Who knows? The next big innovation in web components might come from you.
Remember, every expert was once a beginner. Your web component journey starts now.
Thanks for diving into web components with me!
Have you experimented with web components? What challenges did you face? Any cool tricks you've discovered? Drop your experiences, questions, or even your "Hello, World!" in the comments below.
And hey, if you found this helpful, why not subscribe for more dev insights? I'll be exploring more web technologies, sharing tips, and maybe even featuring some of your comments in future posts.
Until next time, keep coding and stay curious! ๐
Don't forget to share this article if you enjoyed it - spreading knowledge helps us all level up together.
See you in the next one!
Subscribe to my newsletter
Read articles from Ameen Alade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
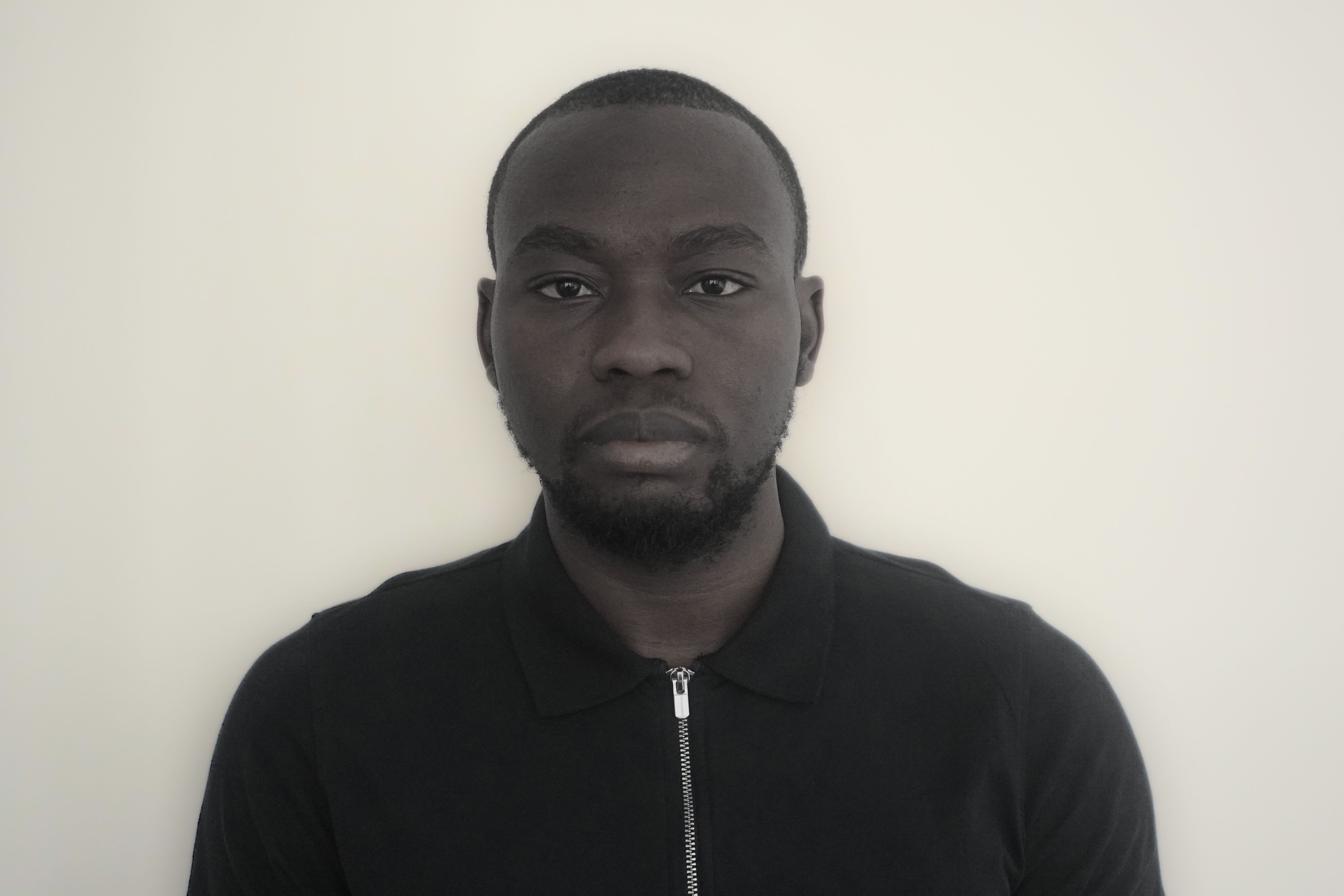
Ameen Alade
Ameen Alade
Hi, Iโm Ameen Alade.๐๐ฝ I'm a UX focused Frontend Developer with an eye for designing and creating unique digital experiences.