When to Use JPA Specification Over Native Query or JPQL in Java Spring Boot
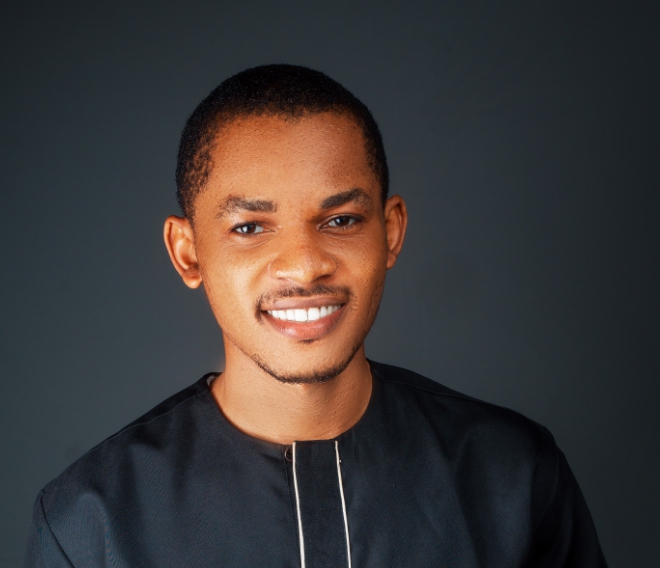
In Spring Boot applications, accessing and querying data are essential tasks that one must carry out at some point. For different scenarios, developers frequently select between JPQL (Java Persistence Query Language), Native Query, and JPA Specification. This article examines the advantages of the JPA specification and situations in which it could be preferable to JPQL or Native Query.
Understanding Your Options
Native Query refers to using repository techniques to write raw SQL directly. gives complete control over SQL execution, but it is database-specific and prone to errors.
JPQL functions similarly to SQL, but with entity objects instead of database tables. It offers an object-oriented method for data querying; nevertheless, for large queries, it may become less understandable and complex.
JPA Specification: A component of the JPA criterion API that enables programmatic creation of type-safe, dynamic queries. provides a scalable, adaptable method for creating queries.
Advantages of JPA Specification
Type Safety and Refactoring Support
JPA Specification offers type-safe queries, catching errors at compile time instead of runtime and reducing bugs related to typos or incorrect field names.
public class CustomerSpecification { public static Specification<Customer> hasName(String name) { return (root, query, criteriaBuilder) -> criteriaBuilder.equal(root.get("name"), name); } }
Dynamic Query Building
It excels in building complex, dynamic queries programmatically. This is particularly useful for scenarios with optional parameters and complex search functionalities.
public static Specification<Customer> getCustomerSpecs(String name, Integer age) { return (root, query, criteriaBuilder) -> { List<Predicate> predicates = new ArrayList<>(); if (name != null) { predicates.add(criteriaBuilder.equal(root.get("name"), name)); } if (age != null) { predicates.add(criteriaBuilder.equal(root.get("age"), age)); } return criteriaBuilder.and(predicates.toArray(new Predicate[0])); }; }
Separation of Concerns
JPA Specification encourages a clear separation of concerns. Specifications can be reused across different services and repositories, promoting code reuse and cleaner architecture.
@Service public class CustomerService { @Autowired private CustomerRepository customerRepository; public List<Customer> findCustomers(String name, Integer age) { Specification<Customer> specs = CustomerSpecification.getCustomerSpecs(name, age); return customerRepository.findAll(specs); } }
Enhanced Readability and Maintainability
Specification queries are often more readable and maintainable than complex JPQL or Native Queries. The programmatic approach breaks down query construction into smaller, manageable pieces.
@Repository public interface CustomerRepository extends JpaSpecificationExecutor<Customer>, JpaRepository<Customer, Long> { }
Database Independence
JPA Specification abstracts the underlying database implementation, making code more portable and database-agnostic. This contrasts with Native Queries, which are tightly coupled to specific SQL dialects.
Limitations of JPA Specification
Performance: Native Queries can be optimized for specific databases, potentially offering better performance for complex, performance-critical queries.
Complex SQL: Native queries might be necessary for highly complex SQL queries leveraging specific database features.
Learning Curve: JPA Specification has a steeper learning curve and can be more verbose than JPQL or Native Queries for simple queries.
When to Use Native Query or JPQL
Performance-Critical Queries: Use native queries when performance is critical, and you need to leverage specific database optimizations.
Simple and Readable Queries: For straightforward queries, JPQL can be more concise and readable than the verbosity of the JPA Specification.
Database-Specific Features: When using database-specific features or functions not supported by JPA.
Comparing Approaches
Native Query
@Query(value = "SELECT * FROM customers WHERE name = ?1 AND age = ?2", nativeQuery = true)
List<Customer> findByNameAndAge(String name, Integer age);
JPQL
@Query("SELECT c FROM Customer c WHERE c.name = :name AND c.age = :age")
List<Customer> findByNameAndAge(@Param("name") String name, @Param("age") Integer age);
JPA Specification
public class CustomerSpecification {
public static Specification<Customer> hasNameAndAge(String name, Integer age) {
return (root, query, criteriaBuilder) -> {
List<Predicate> predicates = new ArrayList<>();
if (name != null) {
predicates.add(criteriaBuilder.equal(root.get("name"), name));
}
if (age != null) {
predicates.add(criteriaBuilder.equal(root.get("age"), age));
}
return criteriaBuilder.and(predicates.toArray(new Predicate[0]));
};
}
}
public interface CustomerRepository extends JpaSpecificationExecutor<Customer>, JpaRepository<Customer, Long> {
}
List<Customer> customers = customerRepository.findAll(CustomerSpecification.hasNameAndAge("John", 30));
Conclusion
JPA specification is frequently a superior option for intricate, dynamic queries because of its type, safety, flexibility, and maintainability. Native queries, however, might be better suited for performance-critical applications or for utilizing sophisticated, database-specific SQL functionality. JPQL offers medium ground by using an object-oriented querying methodology.
The decision between JPA Specification, Native Query, and JPQL should ultimately be made in light of your project's particular requirements, considering query complexity, performance requirements, and maintainability. By making educated judgments and being aware of the advantages and disadvantages of each technique, you may create reliable and effective Spring Boot apps.
Subscribe to my newsletter
Read articles from Chinemeze ThankGod directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
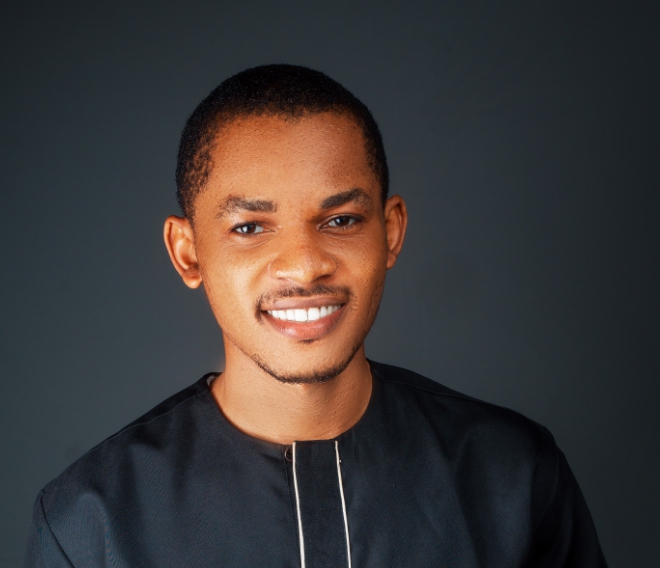
Chinemeze ThankGod
Chinemeze ThankGod
Software Engineer | DevOps Engineer | Problem Solver | Mentor | Systems Designer | Tech Enthusiast With 5 years of experience in software development, I specialize in creating robust Java applications and solving complex problems. I have a passion for teaching and mentoring, having guided many aspiring tech professionals on their journey. In addition to coding, I enjoy writing technical articles, indulging in music, and tackling challenging math problems. Always eager to learn and share knowledge, I thrive on innovation and creativity in the tech world.