Leveraging Anti-Joins in SQL for Advanced Database Engineering: Techniques and Best Practices
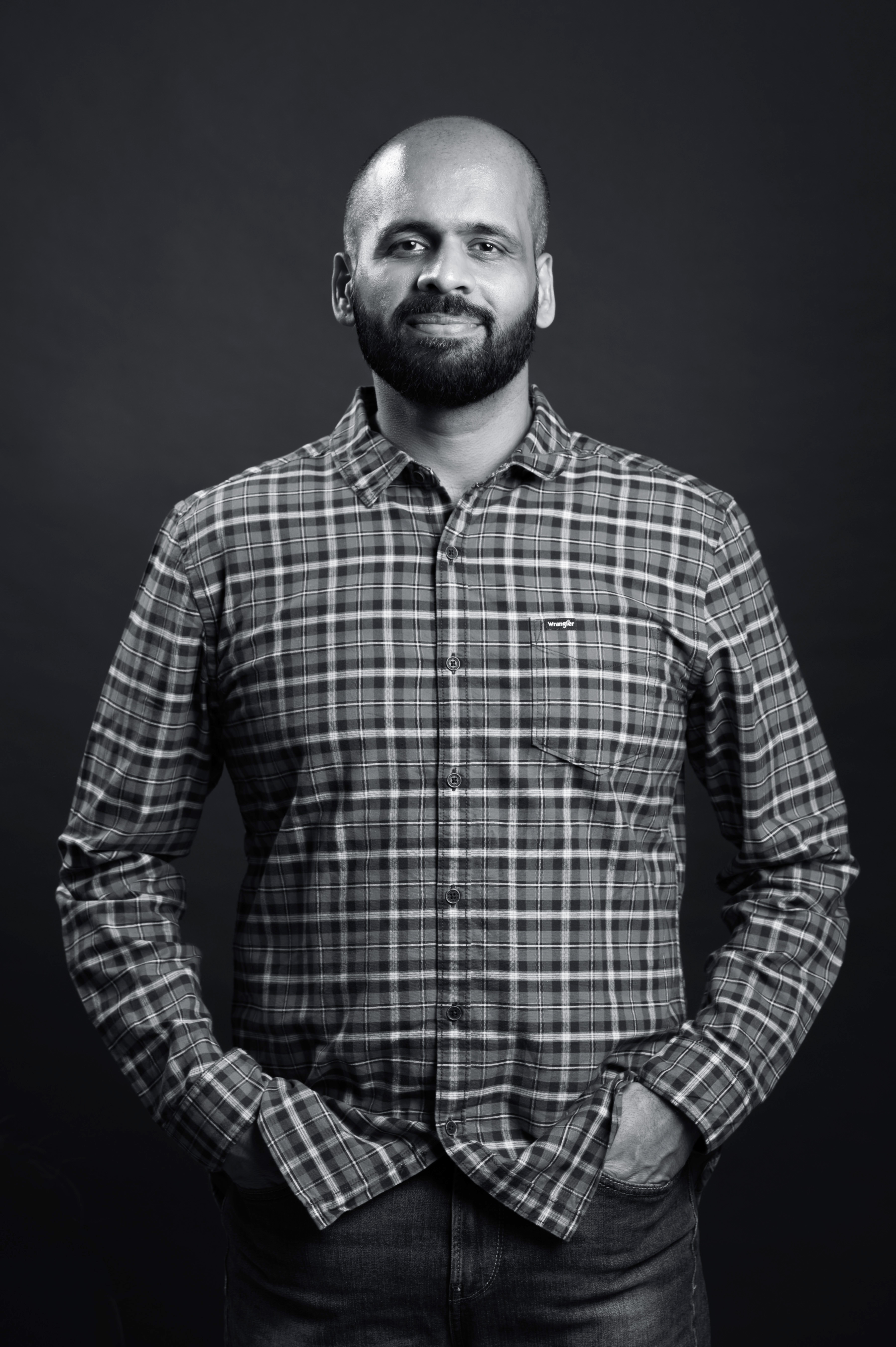
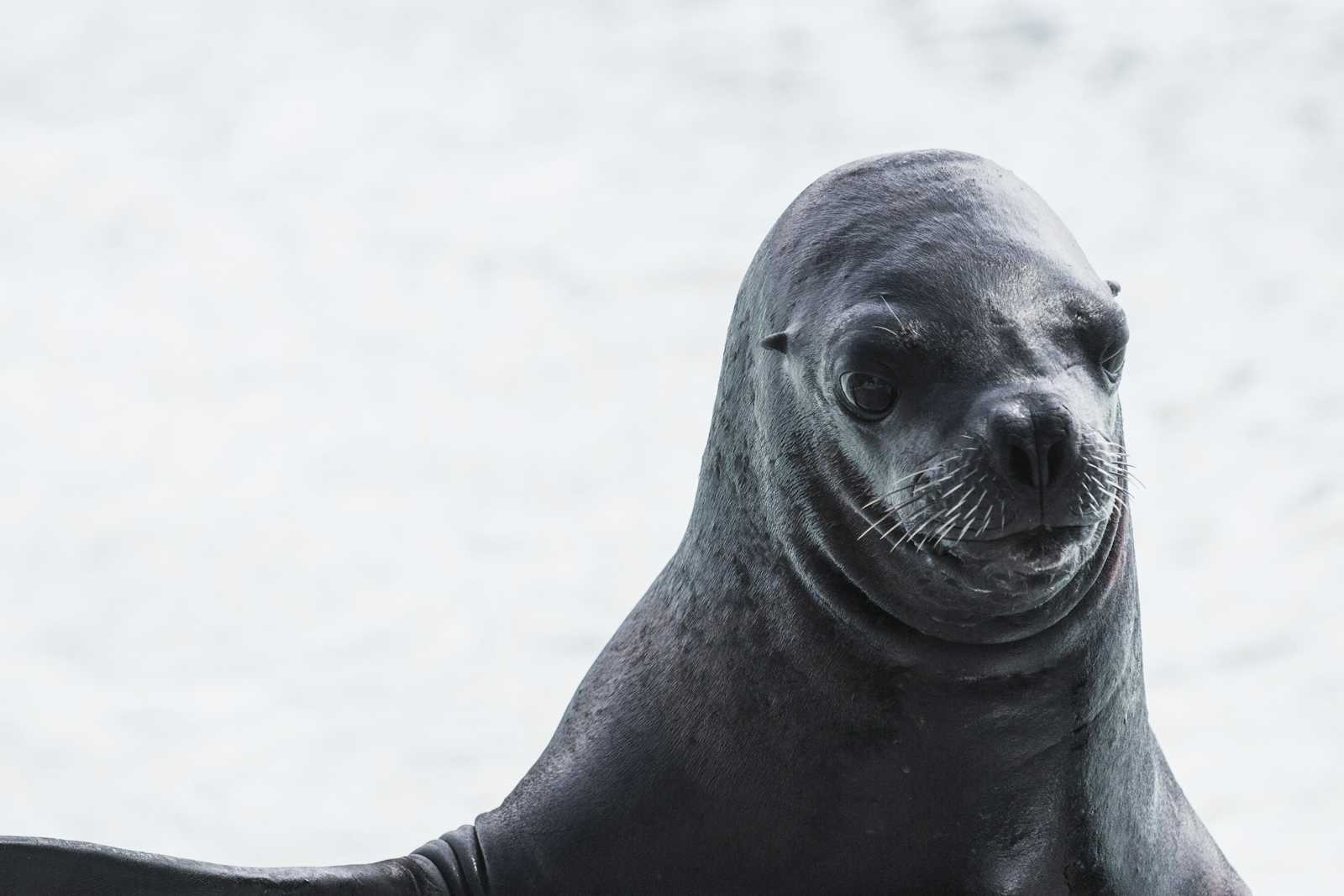
Optimizing Natural Joins in MariaDB Using the Sort-Merge Algorithm
The Sort-Merge join algorithm is a powerful technique for optimizing join operations, especially when dealing with large datasets. This algorithm works by first sorting the input tables on the join keys and then merging the sorted tables. While MariaDB typically uses hash joins or nested loop joins for joining tables, understanding the principles of Sort-Merge joins can help you optimize performance, particularly for natural joins, by mimicking the benefits through index optimization and query structuring.
How the Sort-Merge Join Algorithm Works
Sorting Phase:
- Both input tables are sorted based on the join keys.
Merging Phase:
- The sorted tables are scanned in a synchronized manner, and matching rows are merged based on the join condition.
Benefits of Sort-Merge Joins
Efficient for Large Datasets: Especially beneficial for joining large tables where both tables can be sorted and merged efficiently.
Suitable for Range Queries: Performs well when dealing with range-based joins.
Reduced Random I/O: Sorting helps in accessing rows sequentially, reducing random I/O operations.
Implementing Sort-Merge Join Optimization in MariaDB
While MariaDB does not natively implement the Sort-Merge join algorithm, you can achieve similar performance benefits by leveraging indexing, proper query structuring, and optimizer hints.
1. Indexing
Ensure that the columns used in the join condition are indexed. Indexes facilitate efficient sorting and merging operations.
CREATE INDEX idx_table1_join_key ON table1(join_key);
CREATE INDEX idx_table2_join_key ON table2(join_key);
2. Using ORDER BY and FORCE INDEX
You can encourage the optimizer to use indexes and sort the tables by the join key before performing the join.
SELECT *
FROM table1 FORCE INDEX (idx_table1_join_key)
JOIN table2 FORCE INDEX (idx_table2_join_key) ON table1.join_key = table2.join_key
ORDER BY table1.join_key, table2.join_key;
3. Query Optimization with EXPLAIN
Use the EXPLAIN
command to analyze the query execution plan and ensure that the optimizer is using the indexes and sorting efficiently.
EXPLAIN
SELECT *
FROM table1
JOIN table2 ON table1.join_key = table2.join_key
ORDER BY table1.join_key, table2.join_key;
4. Optimizer Hints
Use optimizer hints to influence the query execution plan. For example, you can use STRAIGHT_JOIN
to force the join order.
SELECT STRAIGHT_JOIN *
FROM table1
JOIN table2 ON table1.join_key = table2.join_key
ORDER BY table1.join_key, table2.join_key;
5. Temporary Tables
For very large datasets, consider using temporary tables to pre-sort the data.
CREATE TEMPORARY TABLE temp_table1 AS
SELECT * FROM table1 ORDER BY join_key;
CREATE TEMPORARY TABLE temp_table2 AS
SELECT * FROM table2 ORDER BY join_key;
SELECT *
FROM temp_table1
JOIN temp_table2 ON temp_table1.join_key = temp_table2.join_key;
Example: Optimizing a Natural Join
Assume we have two tables, employees
and departments
, and we want to perform a natural join on the department_id
:
Schema Definition
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
name VARCHAR(100),
department_id INT,
INDEX(department_id)
);
CREATE TABLE departments (
department_id INT PRIMARY KEY,
department_name VARCHAR(100),
INDEX(department_id)
);
Optimized Natural Join Query
EXPLAIN
SELECT *
FROM employees
JOIN departments ON employees.department_id = departments.department_id
ORDER BY employees.department_id, departments.department_id;
Conclusion
While MariaDB does not natively support Sort-Merge joins, you can achieve similar performance benefits by leveraging indexing, query structuring, and optimizer hints. By ensuring that join keys are indexed, using ORDER BY
clauses, analyzing execution plans with EXPLAIN
, and employing temporary tables when necessary, you can optimize natural joins to perform efficiently on large datasets. These techniques help in reducing random I/O operations and ensuring that join operations are executed in a streamlined and performant manner.
Subscribe to my newsletter
Read articles from Shiv Iyer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
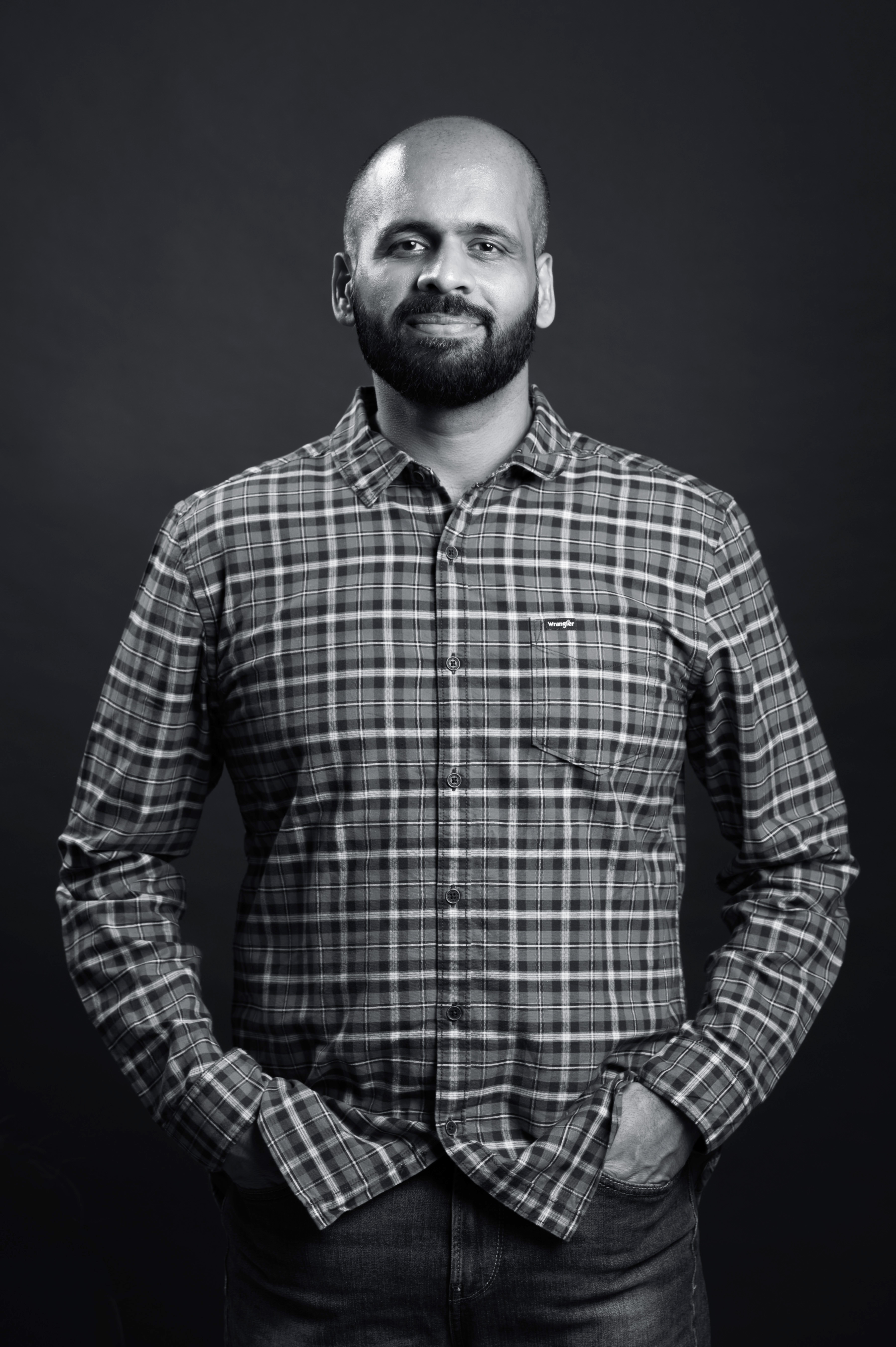
Shiv Iyer
Shiv Iyer
Over two decades of experience as a Database Architect and Database Engineer with core expertize in Database Systems Architecture/Internals, Performance Engineering, Scalability, Distributed Database Systems, SQL Tuning, Index Optimization, Cloud Database Infrastructure Optimization, Disk I/O Optimization, Data Migration and Database Security. I am the founder CEO of MinervaDB Inc. and ChistaDATA Inc.