Chapter 3: State and Props
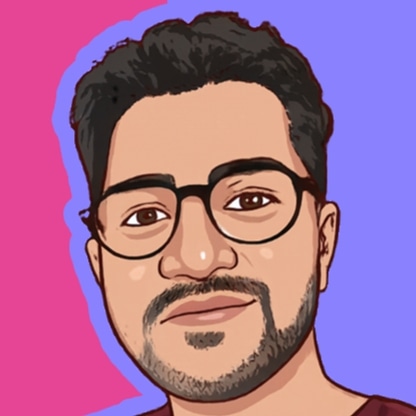
Welcome back to our React Native journey. In the last chapter, we explored components and JSX, the building blocks of any React Native app. Today, we're going to dive into state and props, two essential concepts that make our components dynamic and interactive.
3.1 What Are State and Props?
Before we jump into code, let’s understand what state and props are:
State: Think of state as the heart of your component. It’s an object that holds data that may change over time. State is managed within the component and can be updated using the
setState
function.Props: Short for properties, props are used to pass data from one component to another. Props are read-only and cannot be modified by the component that receives them.
3.2 Using State in Functional Components
React introduced hooks in version 16.8, allowing functional components to use state. The useState
hook is the most commonly used hook for managing state.
Here’s an example:
import React, { useState } from 'react';
import { View, Text, Button, StyleSheet } from 'react-native';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<View style={styles.container}>
<Text style={styles.countText}>Count: {count}</Text>
<Button title="Increase" onPress={() => setCount(count + 1)} />
</View>
);
};
const styles = StyleSheet.create({
container: {
padding: 20,
alignItems: 'center',
},
countText: {
fontSize: 20,
marginBottom: 10,
},
});
export default Counter;
In this example, Counter
is a functional component with state. The useState
hook initializes the count
state variable to 0 and provides a function setCount
to update it.
3.3 Using Props to Pass Data
Props allow you to pass data from a parent component to a child component. Let’s modify our previous example to use props.
import React from 'react';
import { View, Text, Button, StyleSheet } from 'react-native';
const Counter = ({ initialCount }) => {
const [count, setCount] = useState(initialCount);
return (
<View style={styles.container}>
<Text style={styles.countText}>Count: {count}</Text>
<Button title="Increase" onPress={() => setCount(count + 1)} />
</View>
);
};
const App = () => {
return (
<View style={styles.appContainer}>
<Counter initialCount={5} />
</View>
);
};
const styles = StyleSheet.create({
container: {
padding: 20,
alignItems: 'center',
},
countText: {
fontSize: 20,
marginBottom: 10,
},
appContainer: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
export default App;
In this updated example, Counter
receives an initialCount
prop from the App
component. The initialCount
prop sets the initial value of the count
state.
3.4 Combining State and Props
You can combine state and props to create more dynamic and interactive components. Let’s create a simple shopping cart example to illustrate this.
import React, { useState } from 'react';
import { View, Text, Button, StyleSheet } from 'react-native';
const Product = ({ name, price, onAddToCart }) => {
return (
<View style={styles.productContainer}>
<Text style={styles.productText}>{name} - ${price}</Text>
<Button title="Add to Cart" onPress={onAddToCart} />
</View>
);
};
const ShoppingCart = () => {
const [cart, setCart] = useState([]);
const handleAddToCart = (product) => {
setCart([...cart, product]);
};
return (
<View style={styles.cartContainer}>
<Product name="Apple" price={1} onAddToCart={() => handleAddToCart('Apple')} />
<Product name="Banana" price={0.5} onAddToCart={() => handleAddToCart('Banana')} />
<Text style={styles.cartText}>Cart: {cart.join(', ')}</Text>
</View>
);
};
const styles = StyleSheet.create({
productContainer: {
marginBottom: 10,
},
productText: {
fontSize: 18,
},
cartContainer: {
padding: 20,
alignItems: 'center',
},
cartText: {
fontSize: 20,
marginTop: 20,
},
});
export default ShoppingCart;
In this example:
Product
is a component that receivesname
,price
, andonAddToCart
props.ShoppingCart
manages the cart state and updates it when a product is added.
Understanding state and props is crucial for building interactive React Native apps. State allows your components to manage their data, while props let you pass data and event handlers between components. This combination is powerful and enables you to create dynamic, responsive user interfaces.
In the next chapter, we’ll delve into handling events and user interactions in React Native.
Keep coding!
Subscribe to my newsletter
Read articles from Vashishth Gajjar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
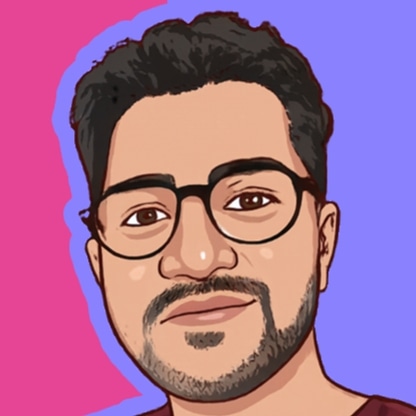
Vashishth Gajjar
Vashishth Gajjar
Empowering Tech Enthusiasts with Content & Code 🚀 CS Grad at UTA | Full Stack Developer 💻 | Problem Solver 🧠