Chapter 7: Styling
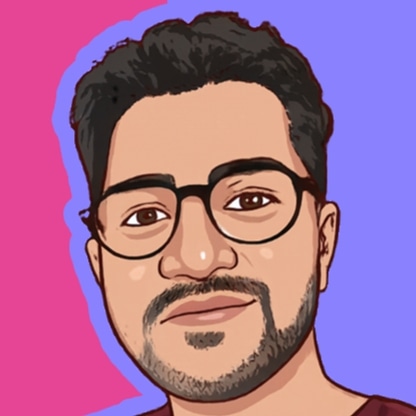
Welcome back to our React Native series. By now, you’ve learned the basics of creating a React Native app, handling state, props, navigation, and even fetching data from APIs. Now, it's time to make our apps look great! In this chapter, we’ll dive into styling in React Native, making your app visually appealing and user-friendly.
7.1 Why Styling Matters
Styling is crucial for user experience. A well-styled app not only looks good but also feels intuitive and easy to use. In React Native, styling is done using JavaScript, which makes it similar to how we style in web development but with some differences.
7.2 Basics of Styling
React Native uses a subset of CSS for styling, but it leverages JavaScript objects instead of traditional CSS syntax. Let’s start with the basics.
Create a new file called App.js
and add the following code:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.header}>Welcome to React Native Styling</Text>
<Text style={styles.paragraph}>
Styling in React Native is simple and powerful. Let's make your app look amazing!
</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#f5f5f5',
padding: 20,
},
header: {
fontSize: 24,
fontWeight: 'bold',
marginBottom: 20,
color: '#333',
},
paragraph: {
fontSize: 16,
color: '#666',
textAlign: 'center',
},
});
export default App;
Understanding the Code
StyleSheet: React Native provides the
StyleSheet
API to create styles. It’s a way to define a set of styles that can be reused across your components.Styles as Objects: Styles are defined as JavaScript objects. Each property in the object corresponds to a style property.
flex: Flexbox is used for layout in React Native. The
flex
property defines how a component should expand to fill its container.justifyContent & alignItems: These properties control the alignment of children along the main axis and the cross axis, respectively.
7.3 Adding More Styles
Let’s add some more styles to make our app look even better. We’ll add a button with some custom styles.
import React from 'react';
import { View, Text, StyleSheet, TouchableOpacity } from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.header}>Welcome to React Native Styling</Text>
<Text style={styles.paragraph}>
Styling in React Native is simple and powerful. Let's make your app look amazing!
</Text>
<TouchableOpacity style={styles.button}>
<Text style={styles.buttonText}>Press Me</Text>
</TouchableOpacity>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#f5f5f5',
padding: 20,
},
header: {
fontSize: 24,
fontWeight: 'bold',
marginBottom: 20,
color: '#333',
},
paragraph: {
fontSize: 16,
color: '#666',
textAlign: 'center',
marginBottom: 20,
},
button: {
backgroundColor: '#007BFF',
paddingVertical: 10,
paddingHorizontal: 20,
borderRadius: 5,
},
buttonText: {
color: '#fff',
fontSize: 16,
fontWeight: 'bold',
},
});
export default App;
Understanding the New Styles
TouchableOpacity: This component makes any view touchable. It’s a great way to create buttons.
button: We styled the button with
backgroundColor
,paddingVertical
,paddingHorizontal
, andborderRadius
.buttonText: This style is applied to the text inside the button, making it white and bold.
7.4 Responsive Design
Responsive design is crucial for mobile apps since they run on various devices with different screen sizes. React Native’s Flexbox layout makes it easy to create responsive designs.
Here’s a quick example of a responsive layout:
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
padding: 20,
},
box: {
width: '80%',
height: 100,
backgroundColor: '#4CAF50',
marginBottom: 20,
justifyContent: 'center',
alignItems: 'center',
},
boxText: {
color: '#fff',
fontSize: 18,
},
});
In the above example, the box
component will take 80% of the width of its parent container, making it responsive to different screen sizes.
Styling in React Native is both powerful and flexible, allowing you to create beautiful, responsive designs with ease. We’ve covered the basics of using StyleSheet
, Flexbox, and creating reusable styles. Experiment with different styles and layouts to see what works best for your app.
In the next chapter, we’ll dive into React Navigation, helping you create seamless and intuitive navigation experiences in your app.
Happy coding!
Subscribe to my newsletter
Read articles from Vashishth Gajjar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
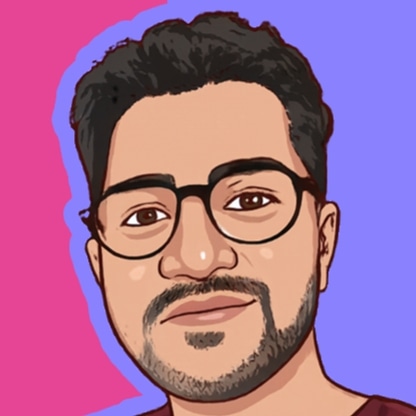
Vashishth Gajjar
Vashishth Gajjar
Empowering Tech Enthusiasts with Content & Code 🚀 CS Grad at UTA | Full Stack Developer 💻 | Problem Solver 🧠