Understanding Singleton Design Pattern in C#
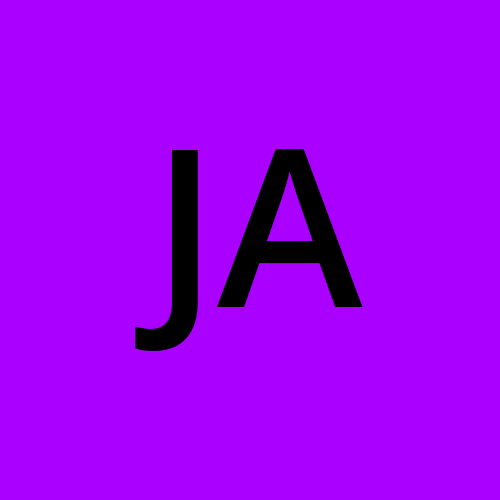
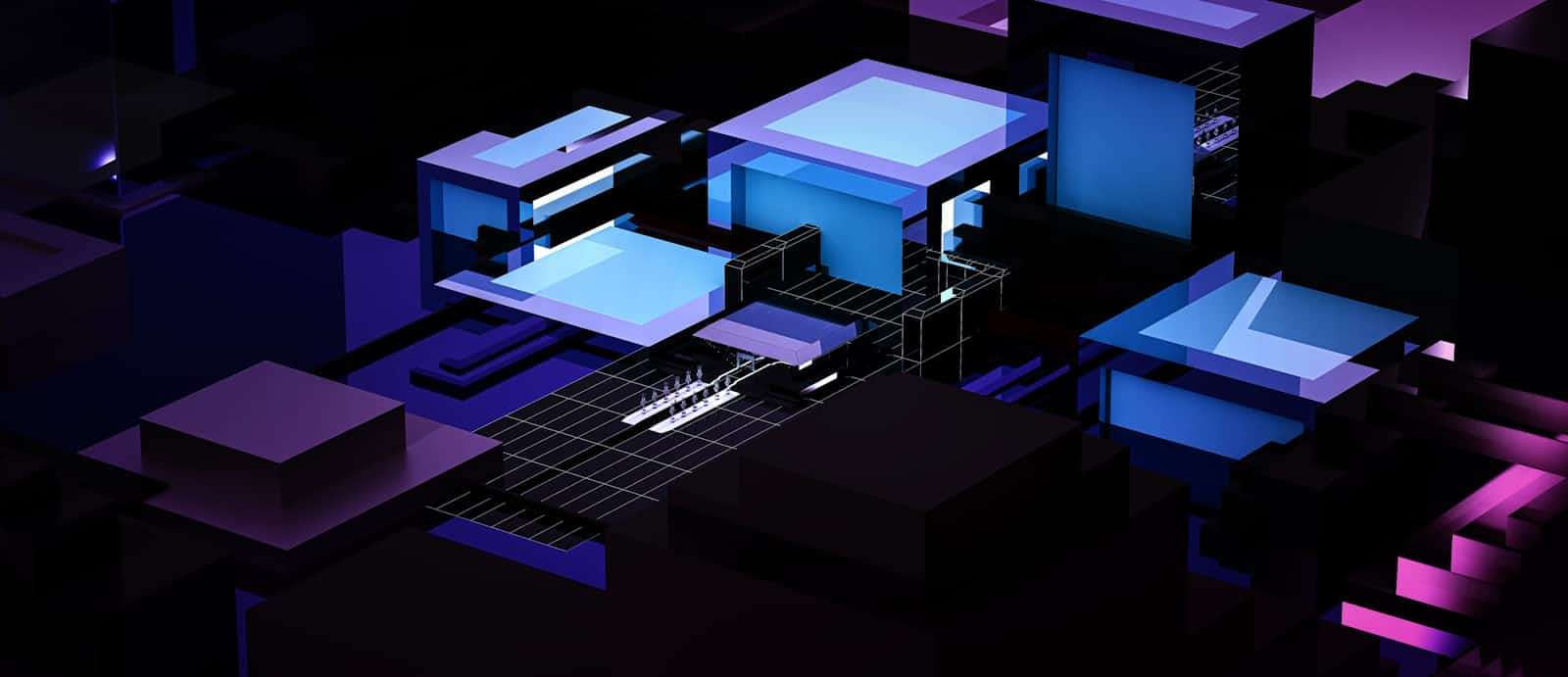
The Singleton design pattern is one of the most widely used patterns in software development. It ensures that a class has only one instance and provides a global point of access to that instance. This pattern is particularly useful in scenarios where a single instance of a class is required to coordinate actions across the system. In this blog post, we will explore the Singleton design pattern in C#, understand its benefits, and see how it can be implemented.
What is the Singleton Design Pattern?
The Singleton design pattern restricts the instantiation of a class to one "single" instance. This is useful when exactly one object is needed to coordinate actions across the system. The pattern involves a single class which is responsible to create an object while ensuring that only one object gets created. This class provides a way to access its only object which can be accessed directly without the need to instantiate the object of the class.
When to Use Singleton Pattern
The Singleton pattern is useful in various scenarios, such as:
Configuration Settings: When application configuration settings need to be accessed in multiple places throughout the application.
Logging: Ensuring that all classes log to the same file or logging mechanism.
Caching: Managing a global cache of objects.
Thread Pooling: Coordinating threads in a multithreaded application.
Implementing Singleton in C
There are different ways to implement the Singleton pattern in C#. Let’s explore the most common implementations.
Basic Singleton Implementation
The simplest form of Singleton in C# is implemented using a static instance and a private constructor.
public class Singleton
{
private static Singleton instance = null;
// Private constructor ensures that object is not instantiated from outside the class.
private Singleton() { }
public static Singleton Instance
{
get
{
if (instance == null)
{
instance = new Singleton();
}
return instance;
}
}
}
Thread-Safe Singleton
In a multithreaded environment, the basic implementation can cause issues if multiple threads try to create an instance simultaneously. To make it thread-safe, we can use a lock object.
public class Singleton
{
private static Singleton instance = null;
private static readonly object padlock = new object();
private Singleton() { }
public static Singleton Instance
{
get
{
lock (padlock)
{
if (instance == null)
{
instance = new Singleton();
}
return instance;
}
}
}
}
Lazy Initialization Singleton
C# provides a Lazy<T>
type that ensures that the instance is created only when it is accessed for the first time. This approach is thread-safe and ensures lazy initialization.
public class Singleton
{
private static readonly Lazy<Singleton> lazyInstance = new Lazy<Singleton>(() => new Singleton());
private Singleton() { }
public static Singleton Instance
{
get
{
return lazyInstance.Value;
}
}
}
Advantages of Singleton Pattern
Controlled Access: Ensures that there is controlled access to the sole instance.
Reduced Namespace Pollution: Since only one instance exists, there’s less clutter in the namespace.
Reduced Memory Footprint: Only one instance is created, reducing the memory footprint.
Global Point of Access: Provides a global point of access to the instance.
Disadvantages of Singleton Pattern
Global State: Singleton pattern can introduce a global state into an application, which can make it harder to debug and test.
Concurrency Issues: Special care must be taken in multithreaded environments to avoid issues related to concurrent access.
Hidden Dependencies: Can hide dependencies, making the code less clear and harder to understand.
Conclusion
The Singleton design pattern is a powerful and useful pattern when used appropriately. It ensures that a class has only one instance and provides a global point of access to that instance. In C#, there are various ways to implement a Singleton, from a basic implementation to more complex thread-safe versions. While the Singleton pattern offers many benefits, it also comes with certain drawbacks that developers should be aware of. By understanding these nuances, you can effectively leverage the Singleton pattern in your C# applications to maintain clean, efficient, and maintainable code.
Happy coding!
Subscribe to my newsletter
Read articles from Joshua Akosa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
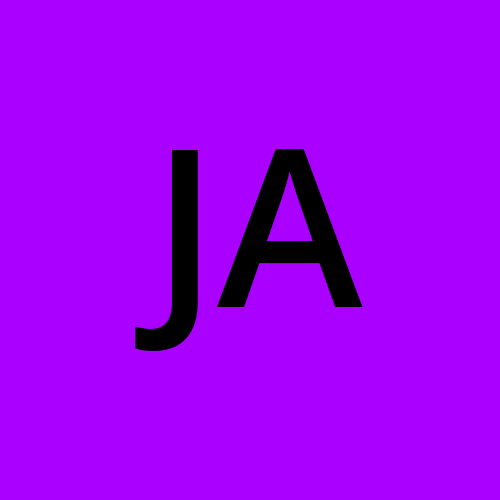