🧑💻 A Beginner’s Guide to Writing Smart Contracts with Solidity 🚀

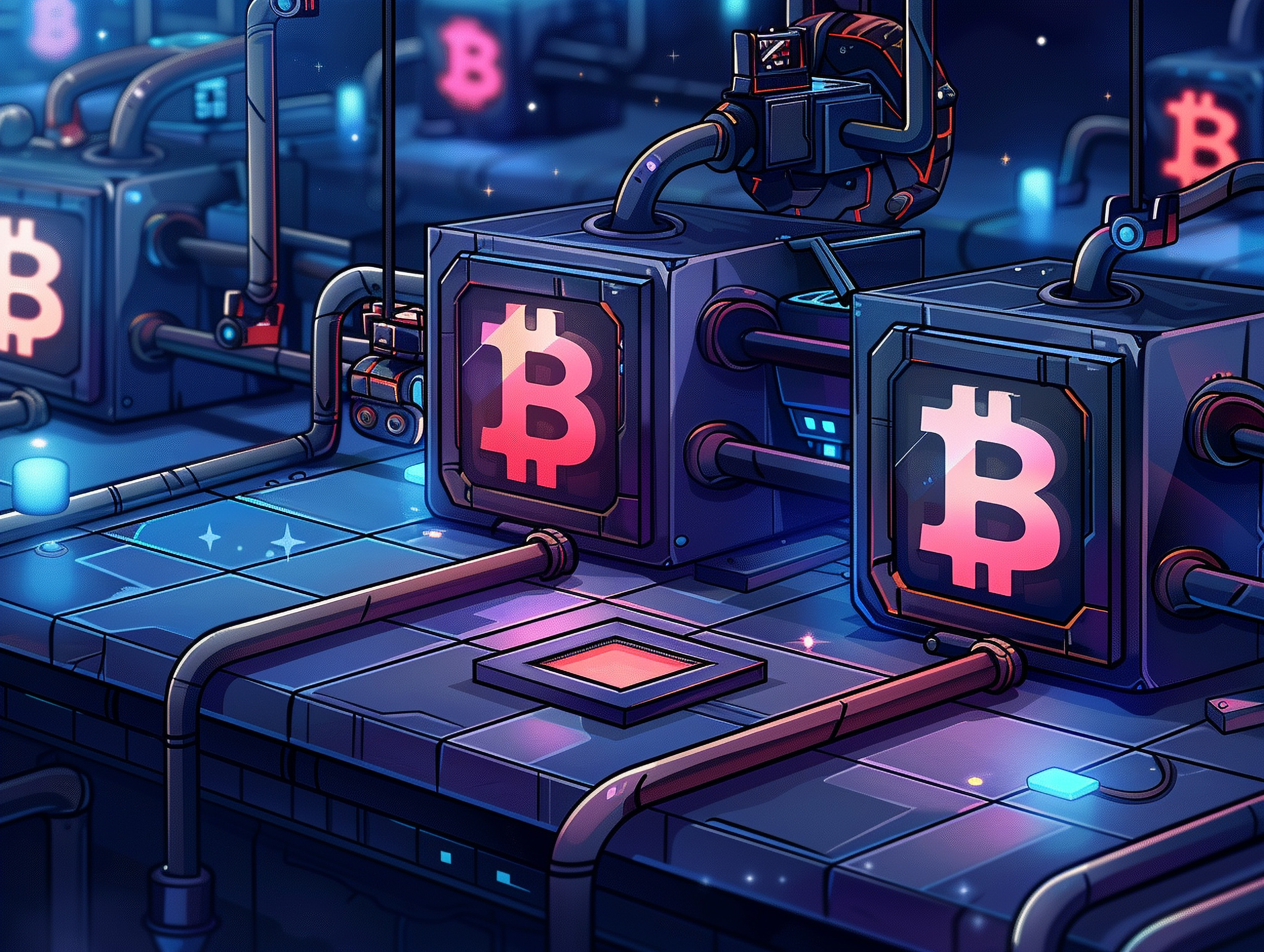
🛠️ Setting Up Your Development Environment
We will use Remix IDE, an online integrated development environment, to write, deploy, and interact with smart contracts. No installation required! Just visit Remix IDE in your browser.
✍️ Writing Your First Smart Contract
Let’s create a simple smart contract that stores and retrieves a value.
1. Basic Smart Contract Example
Here’s a basic Solidity smart contract:
solidityCopy code// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 public storedData;
// Function to set the value of storedData
function set(uint256 x) public {
storedData = x;
}
// Function to get the value of storedData
function get() public view returns (uint256) {
return storedData;
}
}
Explanation:
pragma solidity ^0.8.0;
: Specifies the Solidity compiler version.contract SimpleStorage
: Defines a new contract named SimpleStorage.uint256 public storedData;
: A state variable storedData accessible publicly.function set(uint256 x) public
: Allows setting the value of storedData.function get() public view returns (uint256)
: Retrieves the value of storedData.
🚀 Deploying Your Smart Contract Using Remix
Open Remix IDE: Go to Remix.
Create a New File: Click on the file explorer icon on the left panel, then click on the "New File" button. Name your file
SimpleStorage.sol
and paste the smart contract code into the editor.Compile the Contract: Go to the "Solidity Compiler" tab on the left panel. Make sure the compiler version matches the version specified in your contract (
0.8.0
in this case). Click the "Compile SimpleStorage.sol" button.Deploy the Contract:
Switch to the "Deploy & Run Transactions" tab.
Choose the "JavaScript VM" environment for testing.
Click the "Deploy" button next to your contract.
Interact with Your Contract:
After deployment, your contract will appear under "Deployed Contracts" in the "Deploy & Run Transactions" tab.
Expand your contract to see the available functions.
Use the
set
function to set a value.Use the
get
function to retrieve the stored value.
🔄 Interacting with Your Contract Using Remix
Once deployed, you can interact with your smart contract directly from the Remix interface:
Set Value:
In the "Deployed Contracts" section, find the
set
function.Enter a value in the input box and click the "transact" button.
Get Value:
Find the
get
function in the same section.Click the "call" button to retrieve the stored value. The result will be displayed directly in the Remix interface.
🛠️ Common Mistakes and Tips
🔧 Ensure Proper Compiler Version: Always specify the correct compiler version with
pragma
.💸 Handle Gas Costs: Be aware of the gas costs associated with deploying and interacting with contracts.
🔍 Test Thoroughly: Use Remix’s JavaScript VM to test your contracts before deploying to the Ethereum mainnet.
🎓 Conclusion
Congratulations! 🎉 You've just written, deployed, and interacted with your first smart contract using Solidity and Remix. As you continue exploring, you’ll uncover more advanced features and best practices for building decentralized applications. Keep experimenting and learning, and happy coding!
📚 Further Reading and Resources
Solidity Documentation: Official Solidity documentation.
CryptoZombies: Fun, interactive tutorial for learning Solidity.
OpenZeppelin Contracts: Library of secure smart contracts.
Feel free to ask questions or share your thoughts in the comments below. Happy coding! 🚀
Subscribe to my newsletter
Read articles from Parth Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Parth Chauhan
Parth Chauhan
I'm Parth Chauhan, a versatile web developer with expertise in both frontend and backend technologies. My passion lies in crafting clean, minimalistic designs and implementing effective branding strategies to create engaging and user-centric experiences. I am proficient in Java, Python, C, C++, SQL, MongoDB, computer networks, OS, NoSQL, and DSA. I thrive on bringing ideas to life by building solutions from scratch and am always excited to explore and master new technologies. My approach is centered around coding with precision and creativity, ensuring each project is both functional and aesthetically pleasing. Currently, I'm open to freelance opportunities and eager to collaborate on new ventures. Let’s connect to discuss how we can work together to enhance your project and achieve outstanding results.