Best practices for efficient string handling in Java

Table of contents
- Introduction
- Importance of string handling in Java
- 1.Using StringBuilder for Concatenation
- 2.Prefer String.format for Readable Formatting
- 3.Leverage String.split for Splitting Strings
- 4.Utilize String.join for Joining Strings
- 5.Implement String.intern for Memory Efficiency
- 6.Apply String.replace for Substring Replacement
- 7.Use String.substring for Extracting Substrings
- 8.Be Mindful of String.equals vs ==
- 9.Trim Unnecessary Whitespaces with String.trim
- 10.Avoid Using new String()
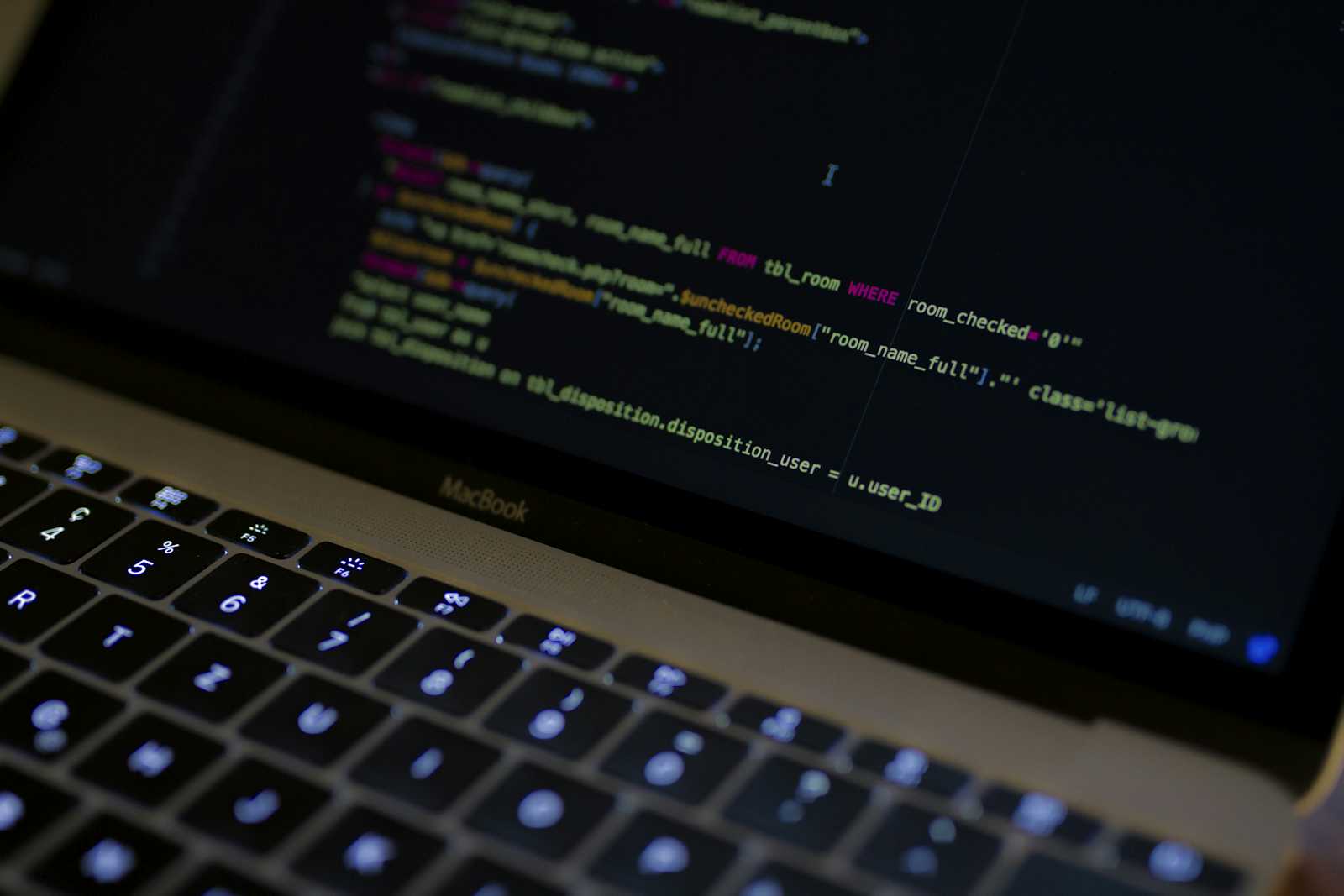
Introduction
Efficient string handling is vital in java, as string are fundamental to many applications. Understanding Java's immutable strings, and the proper use of StringBuilder and StringBuffer can significantly enhance performance. This article provides best practice for optimizing string operations in Java.
Importance of string handling in Java
String handling is crucial in Java due to the extensive use of strings in applications. Efficient string operations boots performance, especially when processing large text volumes or frequent manipulations. Proper string handling improves memory management and execution speed, leading to scalable applications.
Adhering to best practices ensures maintainable and robust code, essential for high-quality software development. Understanding these concepts is vital for any Java developer.
1.Using StringBuilder for Concatenation
StringBuilder is mutable and optimized for concatenation operations, making it much faster than using String with the + operator for multiple concatenations.
Example :
StringBuilder sb = new StringBuilder();
sb.append("Hello");
sb.append(" ");
sb.append("World");
String result = sb.toString();
2.Prefer String.format for Readable Formatting
Prefer String.format for Readable Formatting String.format is a powerful tool for creating formatted strings with placeholders, improving readability and maintainability.
Example :
String name = "John";
int age = 30;
String formattedString = String.format("Name: %s, Age: %d", name, age);
3.Leverage String.split for Splitting Strings
Use String.split to divide a string into an array based on a specified delimiter, simplifying the process of breaking down strings into manageable parts.
Example :
String sentence = "Java is fun";
String[] words = sentence.split(" ");
4.Utilize String.join for Joining Strings
String.join combines multiple strings into a single string with a specified delimiter, making code cleaner and more efficient.
Example :
String[] words = {"Java", "is", "fun"};
String joinedString = String.join(" ", words);
5.Implement String.intern for Memory Efficiency
String.intern stores only one instance of each string in the string pool, reducing memory usage by avoiding duplicates.
Example :
String str1 = new String("Hello").intern();
String str2 = "Hello";
boolean areSame = (str1 == str2); // true
6.Apply String.replace for Substring Replacement
String.replace replaces occurrences of a substring with another substring, streamlining text modification tasks.
Example :
String original = "Hello, World!";
String modified = original.replace("World", "Java");
7.Use String.substring for Extracting Substrings
String.substring extracts a part of the string based on start and end indices, useful for extracting specific segments of a string.
Example :
String text = "Hello, World!";
String sub = text.substring(7, 12); // "World"
8.Be Mindful of String.equals vs ==
Use String.equals for content comparison rather than ==, which checks for reference equality.
Example :
String str1 = new String("Hello");
String str2 = "Hello";
boolean areEqual = str1.equals(str2); // true
9.Trim Unnecessary Whitespaces with String.trim
String.trim removes leading and trailing whitespaces, ensuring cleaner data handling.
Example :
String withSpaces = " Hello, World! ";
String trimmed = withSpaces.trim(); // "Hello, World!"
10.Avoid Using new String()
Avoid creating strings with new String() as it creates unnecessary string objects, instead use string literals. Avoid Using new String()
Example :
String str = "Hello"; // Preferred over new String("Hello");
By following these best practices, Java developers can handle strings more efficiently, leading to improved performance and cleaner, more maintainable code.
Subscribe to my newsletter
Read articles from Pradip Vala directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
