Understanding Stack Data Structures
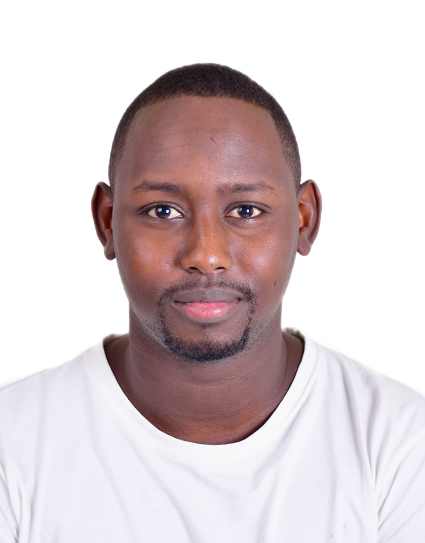
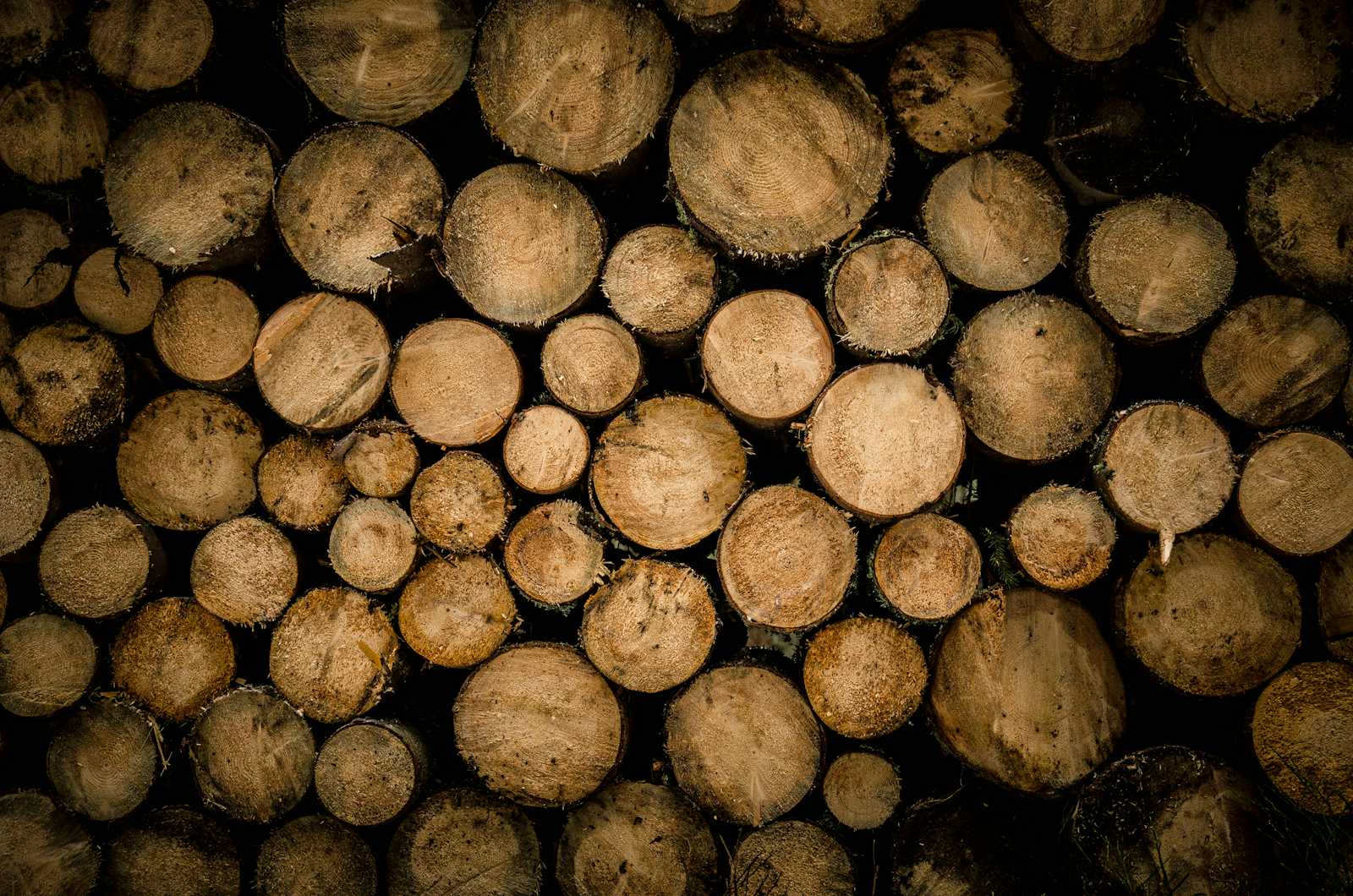
Introduction
Stacks are one of the most fundamental data structures in computer science, offering a simple yet powerful way to manage data. Imagine trying to keep track of tasks you've completed throughout the day; a stack would be like a pile of sticky notes where you add new tasks on top and remove them from the top. In this article, you'll learn about the concept of stacks, their implementation, and practical applications. By the end, you'll understand how to use stacks effectively in your own projects.
What Is a Stack?
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. This means that the last element added to the stack is the first one to be removed. Stacks are analogous to a stack of plates; you can only take the top plate off, and you can only add a new plate on top.
Why Should You Use a Stack?
Stacks are useful in various scenarios where data needs to be temporarily stored and then accessed in reverse order. They are commonly used in:
Undo functionality in text editors
Expression evaluation (e.g., parsing mathematical expressions)
Function call management in programming languages
Stacks are easy to implement and provide efficient O(1) time complexity for both push and pop operations, making them ideal for these tasks.
Implementing a Stack
Below is a basic implementation of a stack using JavaScript. This implementation uses a singly linked list to manage the elements.
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class Stack {
constructor() {
this.top = null;
this.bottom = null;
this.length = 0;
}
peek() {
return this.top;
}
push(value) {
const newNode = new Node(value);
if (this.length === 0) {
this.top = newNode;
this.bottom = newNode;
} else {
const temp = this.top;
this.top = newNode;
this.top.next = temp;
}
this.length++;
return this;
}
pop() {
if (this.length === 0) {
return null;
} else {
this.top = this.top.next;
this.length--;
return this;
}
}
}
const myStack = new Stack();
myStack.push(1);
myStack.push(2);
myStack.push(3);
console.log(myStack);
myStack.pop();
console.log(myStack);
Code Breakdown
Node Class: Represents each element in the stack, containing a value and a pointer to the next node.
Stack Class: Manages the stack operations including:
push(value)
: Adds a new element to the top of the stack.pop()
: Removes the top element from the stack.peek()
: Returns the top element without removing it.
Push Operation
The push
method adds a new value to the top of the stack. If the stack is empty, the new node becomes both the top and bottom. Otherwise, it becomes the new top, and its next
pointer points to the previous top node.
Pop Operation
The pop
method removes the top element from the stack. It decreases the stack's length and updates the top pointer to the next node.
Conclusion
Stacks are a versatile and efficient data structure that play a crucial role in various computing applications. From managing function calls to enabling undo functionality, they offer a simple yet powerful way to handle data. By understanding and implementing stacks, you can solve a wide range of problems more efficiently.
Whether you're building a new feature or optimising an existing system, mastering stacks will give you a valuable tool in your programming toolkit. So, go ahead and experiment with the provided code—there's no better way to learn than by doing!
Subscribe to my newsletter
Read articles from Muhire Josué directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
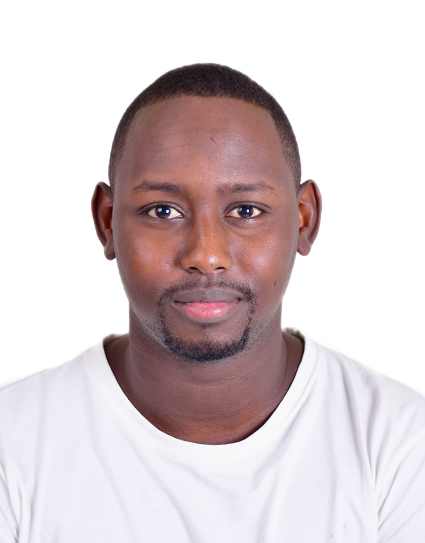
Muhire Josué
Muhire Josué
I am a backend developer, interested in writing about backend engineering, DevOps and tooling.