Manipulating Bits

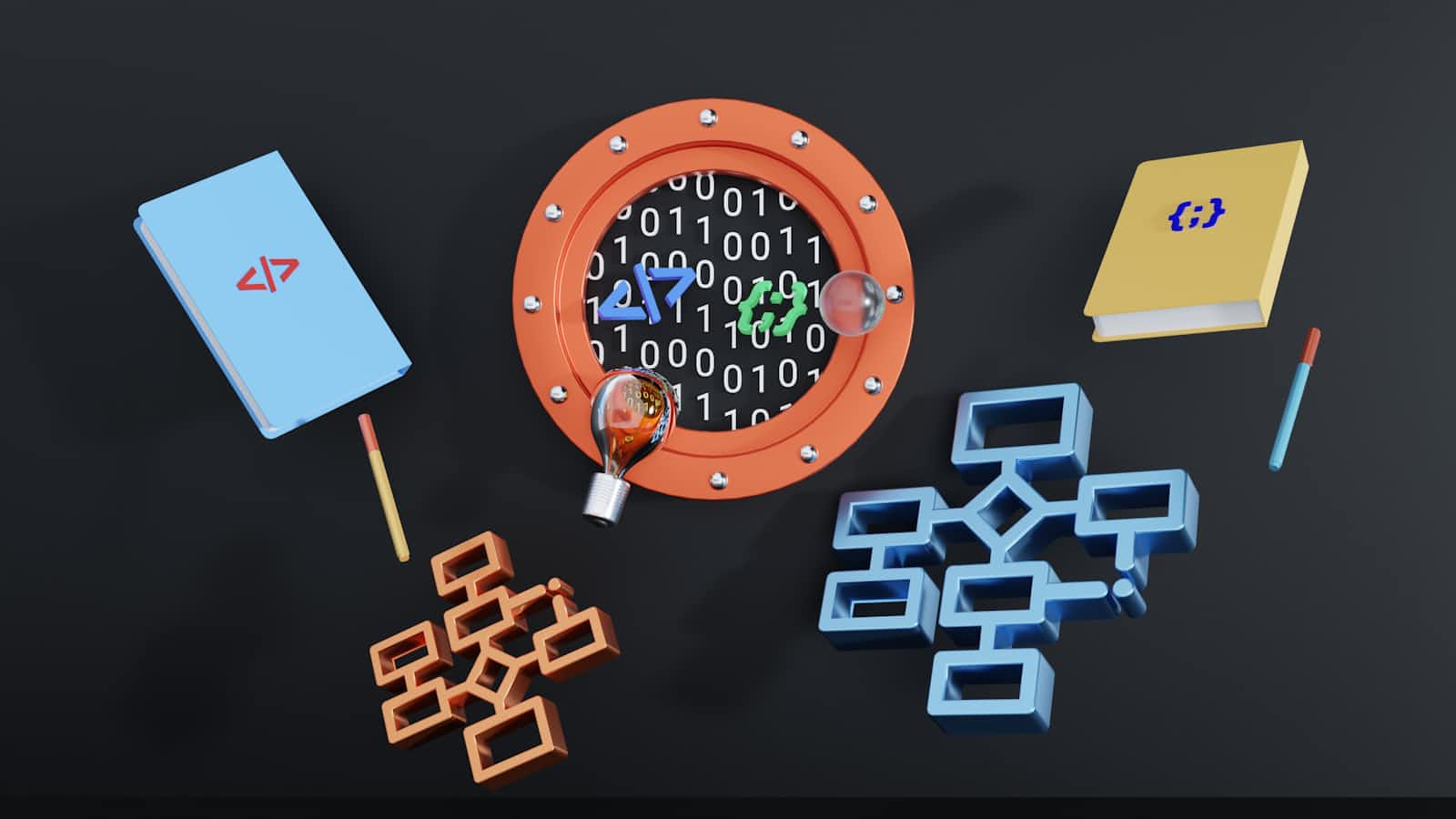
Find the Bit at ith Position
To find the Bit at the ith Position we use AND Operator among the number and mask created by us.Mask consists of 1 at the ith position and all other vacant spots are filled with zero . To achieve this mask we use left shift operator.
public class i_th_bit_of_number {
public static int i_th_bit_of_number(int num,int position){
// ith =position
int mask=1<<(position-1);
int bitvalue=(num&mask)>0?1:0;
return bitvalue;
}
Set the ith bit to 1
Means setting 0 -> 1 and 1 -> 1 at ith position. We use OR operator , we create a mask and then OR it with the original number .
public static int set_bit_to_1(int num,int position){
int mask =1<<(position-1);
int bitvalue=(num|mask);
return bitvalue;
}
Reset the ith Bit to 0
To Reset the bit to zero we use AND operator then we create a mask where we shift 1 to the ith position , taking compliment of our mask gives us with ith position occupied with 0 and all the rest spots with 1.
public static int Reset_bit_to_0(int num,int position){
int mask=~(1<<(position-1));
int newnum =(num & mask);
return newnum;
}
Find the No. of Digits in Binary Code
Iterate over every bit of the number and rase the counter by 1 , do this till the number is equal to 0. we can also find the digits of various Number Systems.
public static int method1(int num){
int count=0;
while (num>0) {
num=num>>1;
count++;
}
return count;
}
public static int method2(int num,int base){
// base for deccimal =10 , for binary =2
int ans=(int)(Math.log(num)/Math.log(base))+1;
return ans;
}
public static void main(String[] args) {
System.out.println(method1(66));
System.out.println(method2(66, 2));
System.out.println(method2(123456789, 10));
}
}
Is Number ODD or Even ??
check the value of the Least Significant Bit .
if 0 --> EVEN else 1 --> ODD.
public static boolean isodd(int n){
return (n&1)==1;
}
Find the Single Element in Duplicate Element Array
LOGIC :when we XOR a number with itself it gives zero .
num ^ num = 0
so the only number that will be left is the number that occur only once.
public class single_elemnt_in_duplicate_element_array {
public static int findUnique(int[]arr){
int unique =0;
for(int i:arr){
unique ^=i;
}
return unique;
}
public static void main(String[] args) {
int[] arr={2,3,3,4,2,6,4};
System.out.println(findUnique(arr));
}
}
Number of 1's in Binary of a Number
counting the number of bits that stores 1 as their value. Also Known as SETBITS.
LOGIC: num = num & (num-1)
public class no_of_1_s {
public static int setBits(int num){
int count =0;
// METHOD - 1
// while(num>0){
// count++;
// num -=(num & -num);
// }
//METHOD -2
while(num>0){
count++;
num = num &(num-1);
}
return count;
}
public static void main(String[] args) {
System.out.println(setBits(45));
System.out.println(Integer.toBinaryString(45));
}
}
Calculating the Power
To find the the value of a number raised to some power we used AND Operator.
base^power = value; --> find this value.
public static int calculate_power(int base, int power){
int ans = 1;
while(power>0){
if ((power & 1)==1){
ans *= base;
}
base *=base;
power = power>>1;
}
return ans;
}
Subscribe to my newsletter
Read articles from ANSH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
