Today’s JavaScript Discovery: slice vs. splice - What’s the Difference?

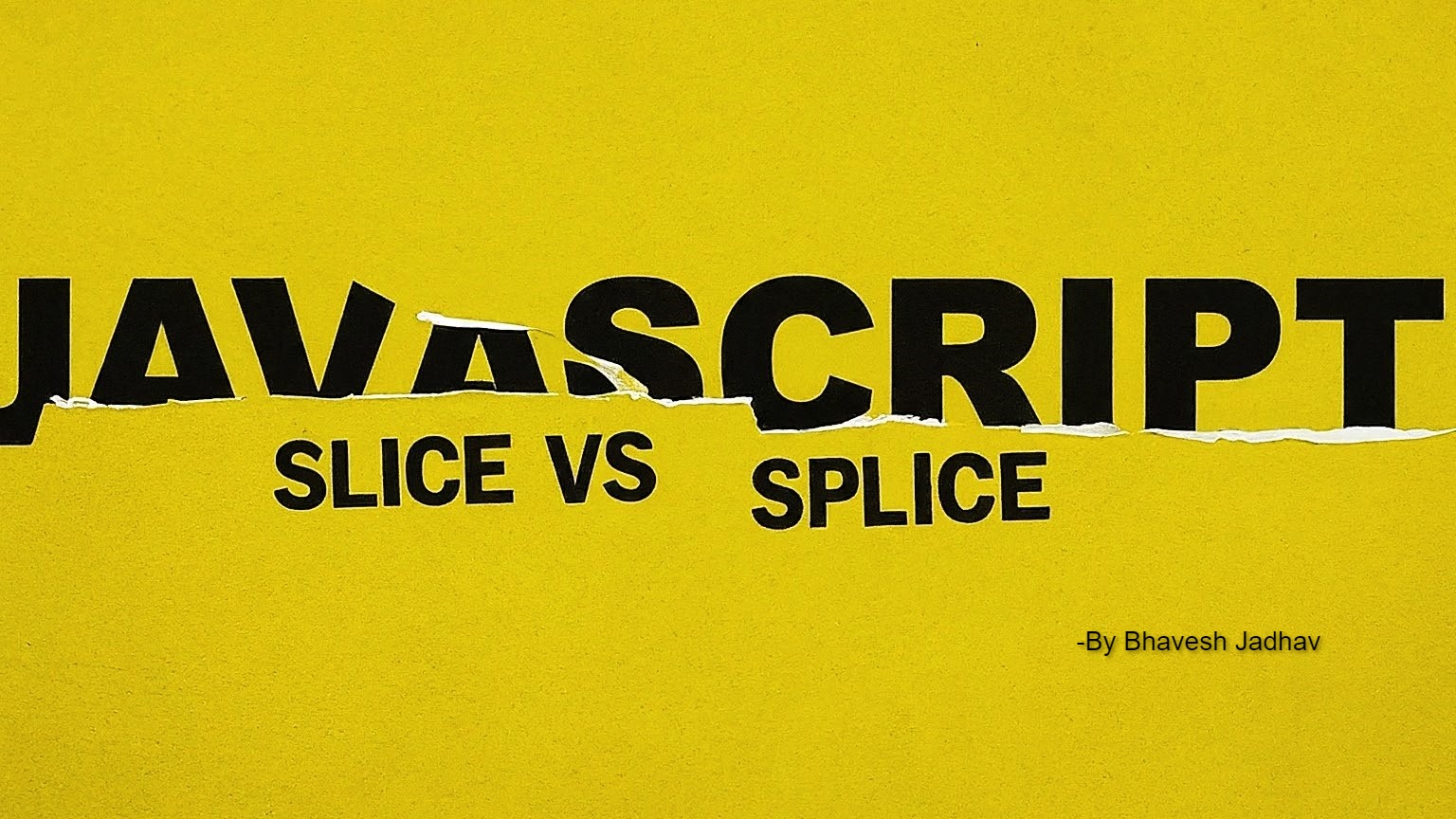
Hey folks,
I had a bit of a "Eureka!" moment today while working with JavaScript arrays. If you’ve ever used slice
and splice
, you might have wondered what exactly sets them apart. Well, I dug into it and thought I’d share what I found.
Let’s dive right in. Here’s a quick snippet of code I was experimenting with:
let arr = [32, 43, 54, 56, 34, 12, 54, 76];
console.log(typeof(arr)); // object
console.log("A ", arr);
// slice creates a new array by copying elements from the start index up to, but not including, the end index. It does not modify the original array.
console.log("using slice ", arr.slice(1, 4)); // [43, 54, 56]
console.log("B ", arr); // [32, 43, 54, 56, 34, 12, 54, 76]
// splice takes the first parameter as the starting index and the second parameter as the count of elements to remove. It returns the removed elements.
// Any additional parameters (from the third parameter onwards) are optional and are added to the array starting from the position where the elements were removed.
console.log("using splice ", arr.splice(1, 4, 9999, 6666)); // [43, 54, 56, 34]
console.log("C ", arr); // [32, 9999, 6666, 12, 54, 76]
The slice
Method
The slice
method is your go-to when you need to extract a portion of an array without modifying the original. It returns a new array containing the selected elements. For example:
arr.slice(1, 4);
This will give you a new array [43, 54, 56]
, leaving the original array untouched. So if you need a snapshot of a part of your array, slice
is your friend.
The splice
Method
On the other hand, splice
is a bit more dramatic. It modifies the original array by removing or adding elements. Here’s what happened when I used splice
:
arr.splice(1, 4, 9999, 6666);
This line removed four elements starting from index 1 and then inserted 9999
and 6666
in their place. The result was that arr
changed to [32, 9999, 6666, 12, 54, 76]
, and the removed elements were [43, 54, 56, 34]
.
Key Takeaways
slice
: Non-destructive, returns a new array.splice
: Destructive, modifies the original array and returns the removed elements.
So there you have it! If you’re ever unsure whether to use slice
or splice
, just remember what you need: a new array or modifications to the existing one. Happy coding!
Catch you later with more discoveries!
Subscribe to my newsletter
Read articles from Bhavesh Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bhavesh Jadhav
Bhavesh Jadhav
I am a passionate web developer from India, currently pursuing an MCA from Government College of Engineering, Aurangabad. With a strong foundation in HTML, CSS, JavaScript, and expertise in frameworks like React and Node.js, I create dynamic and responsive web applications. My skill set extends to backend development with PHP, MySQL, and MongoDB. I also have experience with AJAX, jQuery, and I am proficient in Python and Java. As an AI enthusiast, I enjoy leveraging AI tools and LLMs to enhance my projects. Eager to expand my horizons, I am delving into DevOps to optimize development workflows and improve deployment processes. Always keen to learn and adapt, I strive to integrate cutting-edge technologies into my work. Let's connect and explore the world of technology together!