Understanding Pointers, Reference and Value Semantics in Go
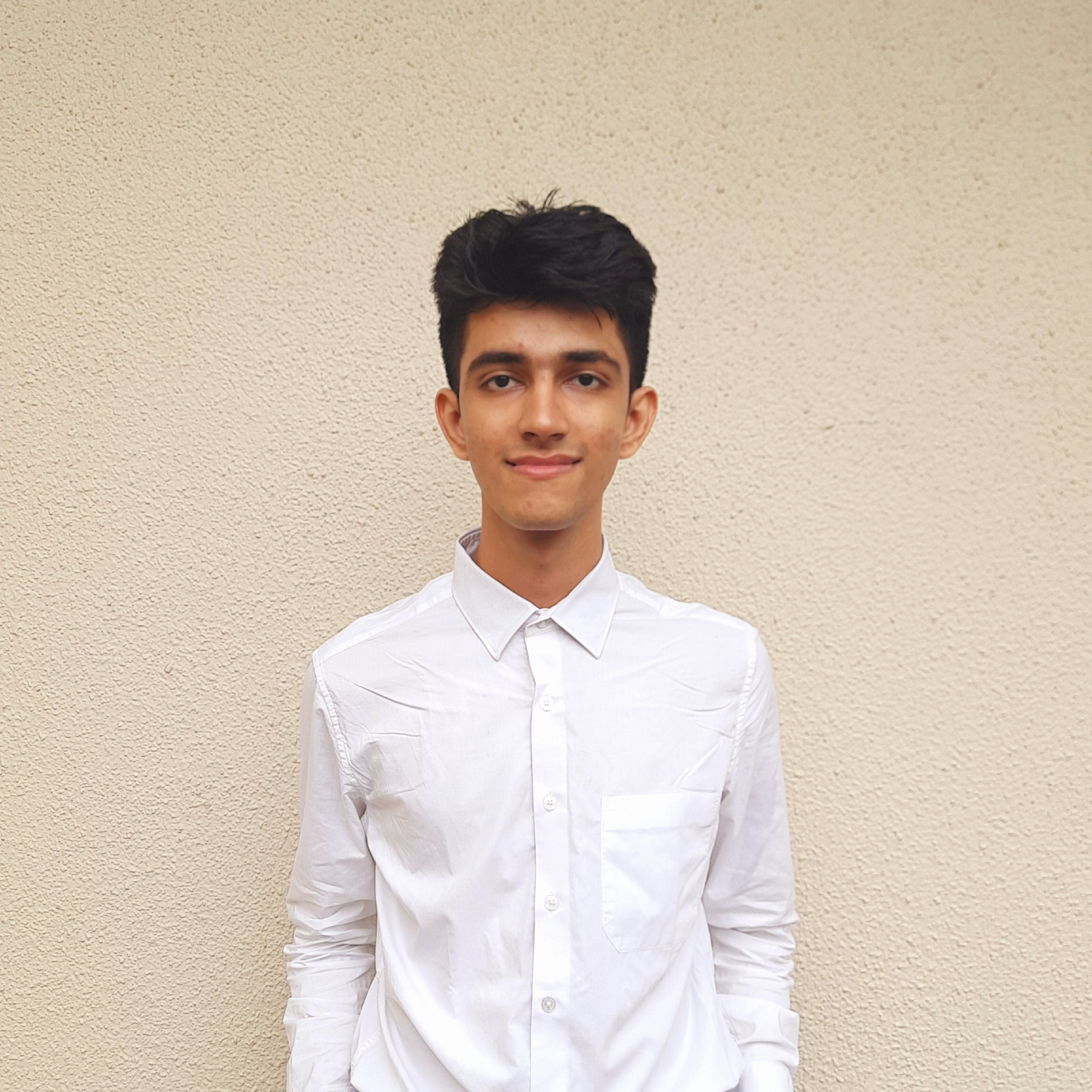
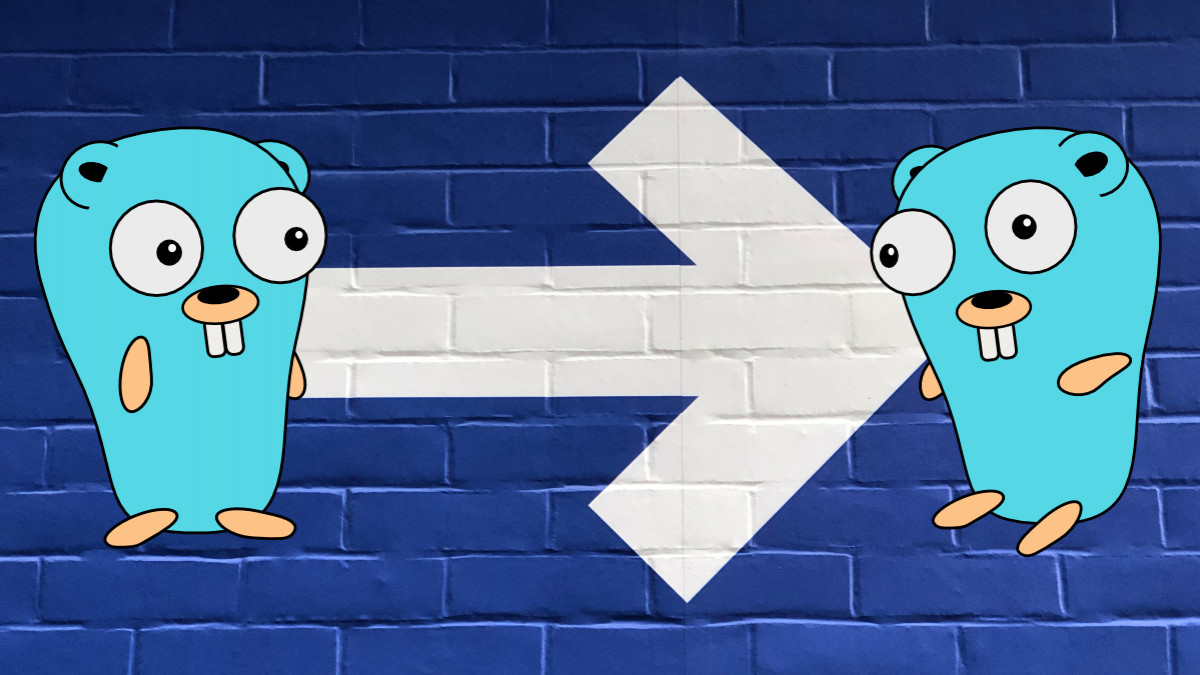
Understanding the distinction between pointer and value semantics in Go is crucial for writing efficient, maintainable, and bug-free code. In this blog post, we’ll delve into some special cases in Go where understanding reference and value semantics becomes crucial, particularly focusing on how these concepts affect loops and slices. Using code examples, we'll dive deep into when and why to use pointers versus values, exploring common pitfalls and practical examples to illustrate key concepts.
The Basics of Pointers and Values
In Go, a pointer holds the memory address of a value. When you use a pointer, you share the actual data, allowing different parts of your program to modify it. Conversely, when you use values, you create copies, which means changes to one copy do not affect others.
When to Use Pointers
Sharing Data: When multiple parts of your program need to modify the same data.
Efficiency: For large structures, copying can be expensive.
Non-Copyable Types: Some types, like those containing a mutex, cannot be copied.
When to Use Values
Immutability: When you want to ensure data isn't shared or modified unexpectedly.
Concurrency: To avoid issues with shared data, prefer not sharing at all.
Practical Examples
Example 1: Sharing Data with Pointers
Suppose we have an Employee
struct:
type Employee struct {
Name string
Age int
mu sync.Mutex
}
If multiple functions need to update the Employee
's details, using pointers is essential.
func updateAge(e *Employee, newAge int) {
e.mu.Lock()
defer e.mu.Unlock()
e.Age = newAge
}
func main() {
emp := &Employee{Name: "John", Age: 30}
updateAge(emp, 31)
fmt.Println(emp.Age) // Output: 31
}
Here, emp
is a pointer to Employee
. Functions that modify Employee
use this pointer to ensure all changes reflect across the program.
Example 2: Efficiency with Large Structures
For large structures, copying can significantly impact performance. Consider a large data block:
type LargeBlock struct {
Data [4096]byte
}
func processBlock(lb *LargeBlock) {
// Process the block
}
func main() {
lb := &LargeBlock{}
processBlock(lb)
}
Passing the pointer to LargeBlock
avoids the overhead of copying the entire 4KB block.
Example 3: Non-Copyable Types
Certain types, like those involving mutexes, cannot be copied. Let's consider a SyncMap
:
type SyncMap struct {
data map[string]string
mu sync.Mutex
}
func updateMap(sm *SyncMap, key, value string) {
sm.mu.Lock()
defer sm.mu.Unlock()
sm.data[key] = value
}
func main() {
sm := &SyncMap{data: make(map[string]string)}
updateMap(sm, "foo", "bar")
fmt.Println(sm.data["foo"]) // Output: bar
}
Passing a SyncMap
by value would result in copying the mutex, rendering it ineffective.
Value Semantics and Immutability
In some scenarios, especially with small structures, value semantics can be beneficial and safe.
Example 4: Small Structs
For small structures, copying is often efficient and ensures immutability:
type Point struct {
X, Y int
}
func move(p Point, dx, dy int) Point {
p.X += dx
p.Y += dy
return p
}
func main() {
p := Point{X: 1, Y: 2}
p = move(p, 2, 3)
fmt.Println(p) // Output: {3 5}
}
Here, Point
is small enough that copying it is efficient. This ensures the original Point
remains unchanged.
Consistency in Function Signatures
Consistency in using pointers or values is vital to avoid bugs. Consider a chain of function calls:
func relocateEmployee(e *Employee) {
function1(e)
function2(e)
eCopy := *e
function3(eCopy)
function4(&eCopy)
}
func function3(e Employee) {
e.Age += 1
}
func function4(e *Employee) {
e.Age += 2
}
func main() {
emp := &Employee{Name: "Jane", Age: 25}
relocateEmployee(emp)
fmt.Println(emp.Age) // Unexpected Output: 27
}
In this example, function3
works with a copy, meaning changes are not reflected outside. This inconsistency can lead to subtle bugs.
Allocation and Performance
Stack vs. Heap Allocation
Go attempts to allocate variables on the stack for efficiency but uses escape analysis to determine if they should be on the heap.
func createEmployee(name string, age int) *Employee {
e := Employee{Name: name, Age: age}
return &e // e escapes to heap
}
Here, e
is allocated on the heap because it escapes the function scope.
Efficient Memory Access
Dense data structures (e.g., slices) are generally more efficient due to better cache utilization:
type DenseData struct {
values []int
}
func process(data *DenseData) {
for i := range data.values {
data.values[i] *= 2
}
}
func main() {
d := &DenseData{values: []int{1, 2, 3, 4}}
process(d)
fmt.Println(d.values) // Output: [2 4 6 8]
}
Reference vs. Value Semantics
Reference semantics means that a variable holds a reference to an actual value stored elsewhere in memory. Changes to this variable affect the original value. Value semantics means that a variable holds a copy of the value. Changes to this variable do not affect the original value.
Understanding these semantics is vital, especially when working with loops and slices in Go.
For Loops and Copying
In Go, when using the range operator in a for loop, the variable receives a copy of the element from the slice or array. This behavior can lead to issues if you intend to mutate the original elements.
Example: Modifying Elements in a Slice
Consider the following example where we attempt to modify elements of a slice within a loop:
type Thing struct {
Field int
}
func main() {
things := []Thing{{1}, {2}, {3}}
for _, thing := range things {
thing.Field = 42
}
fmt.Println(things) // Output: [{1} {2} {3}]
}
In this case, thing
is a copy of each element in things
, so modifications to thing
do not affect the original slice. To make changes visible outside the loop, use the index:
func main() {
things := []Thing{{1}, {2}, {3}}
for i := range things {
things[i].Field = 42
}
fmt.Println(things) // Output: [{42} {42} {42}]
}
By using the index, we directly modify the original elements in the slice.
Appending to Slices
When using append
in Go, it’s crucial to reassign the result back to the original slice variable, as append
might cause the slice to be reallocated if it grows beyond a certain limit.
Example: Appending to a Slice
func main() {
nums := []int{1, 2, 3}
nums = append(nums, 4)
fmt.Println(nums) // Output: [1 2 3 4]
}
In this example, failing to reassign nums
after appending would result in the addition being lost.
Functions Modifying Slices
If a function modifies a slice, it’s essential to return the modified slice to ensure any reallocations are reflected outside the function.
Example: Modifying a Slice in a Function
func appendValue(slice []int, value int) []int {
slice = append(slice, value)
return slice
}
func main() {
nums := []int{1, 2, 3}
nums = appendValue(nums, 4)
fmt.Println(nums) // Output: [1 2 3 4]
}
Here, appendValue
returns the modified slice, which is reassigned to nums
in the main function.
Slices and Pointers
Taking the address of an element in a slice can be risky because slices can reallocate, invalidating the pointer.
Example: Taking the Address of a Slice Element
type User struct {
Count int
}
func addToCount(user *User) {
user.Count++
}
func main() {
users := []User{{Count: 1}, {Count: 2}}
alice := &users[0]
users = append(users, User{Count: 3})
addToCount(alice)
fmt.Println(users) // Output: [{1} {2} {3}]
}
In this example, alice
points to the original slice, which may become invalid after reallocation. To avoid this issue, ensure the pointer is taken after any potential reallocations.
Conclusion
Choosing between pointers and values in Go depends on factors like data sharing, efficiency, and immutability. Understanding reference and value semantics is crucial for writing correct and performant code. When working with loops and slices, being mindful of how Go handles copies and references can help avoid common pitfalls and bugs. By using indexes for direct modification, returning modified slices from functions, and carefully managing pointers, you can ensure your code behaves as expected. Consistently applying these principles will help you write robust and efficient Go programs.
Subscribe to my newsletter
Read articles from Arya M. Pathak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
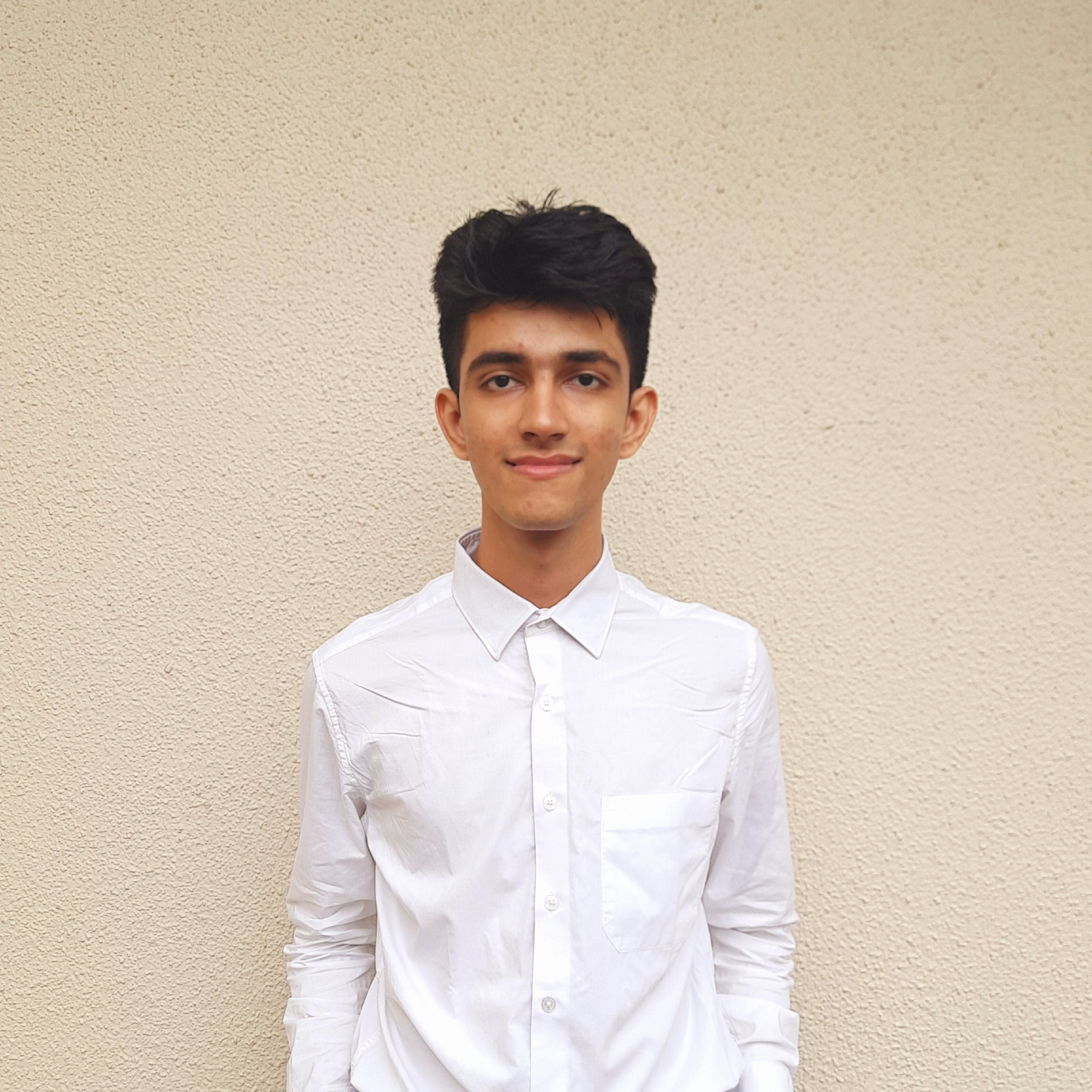
Arya M. Pathak
Arya M. Pathak
Backend Dev | VIT Pune '26 | 🐈Cat Connoisseur /ᐠ。ꞈ。ᐟ\