Implementing Real-Time Features in React: A Guide to Short Polling, Long Polling, and WebSockets
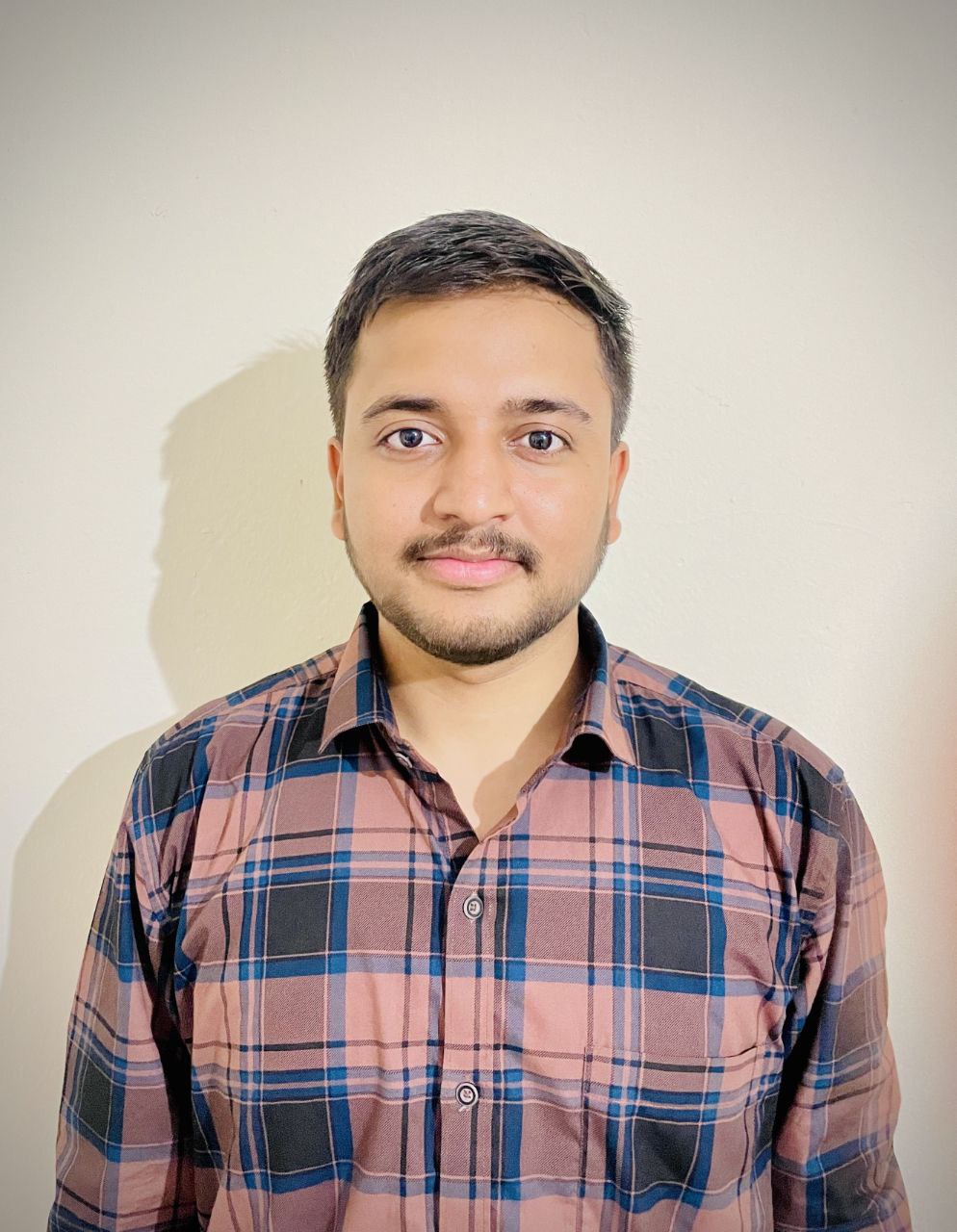
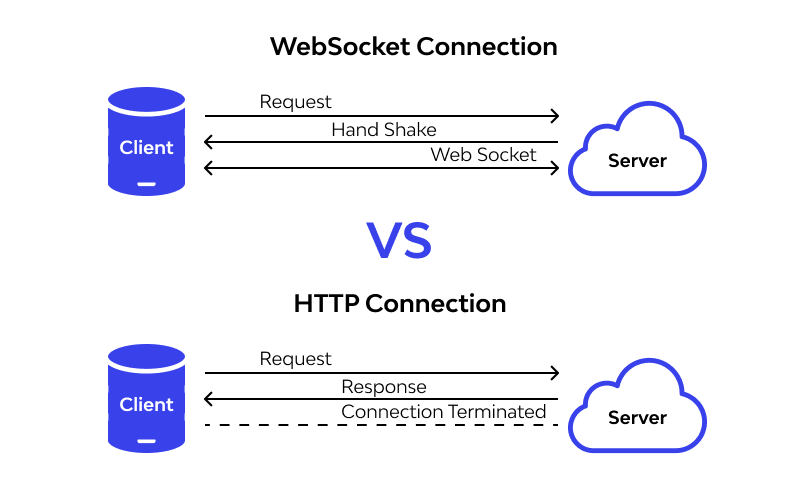
Ever wondered how those cool, real-time updates work in your favorite apps? Whether it's seeing new messages pop up in a chat app or tracking live scores in a sports app, real-time features are like magic that keeps your app fresh and exciting. But behind that magic curtain, there’s a bit of tech wizardry going on. Let’s dive into three popular methods to add real-time features to your React app: Short Polling, Long Polling, and WebSockets. Buckle up!
1. Short Polling: The “Just in Case” Approach
Imagine you’re waiting for your favorite pizza to be ready. You keep peeking into the kitchen every few minutes to check if your pizza’s done. That’s short polling.
How It Works: In short polling, your app regularly checks the server to see if there’s new data. You set a timer (using setInterval
), and your app sends a request to the server every few seconds. If there’s something new, it gets updated. If not, well, better luck next time.
Pros:
Simple to implement.
Works with standard HTTP requests.
Cons:
Can be wasteful, as it keeps asking even when there’s nothing new.
Might cause unnecessary load on the server.
Example Code:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const ShortPollingComponent = () => {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = () => {
axios.get('/api/data')
.then(response => setData(response.data))
.catch(error => console.error('Error:', error));
};
fetchData();
const interval = setInterval(fetchData, 5000); // Check every 5 seconds
return () => clearInterval(interval); // Cleanup on unmount
}, []);
return (
<div>
<h2>Short Polling Data</h2>
<ul>
{data.map(item => <li key={item.id}>{item.text}</li>)}
</ul>
</div>
);
};
export default ShortPollingComponent;
2. Long Polling: The “Hang Tight” Approach
Now, imagine you’re at a restaurant, and instead of constantly asking if your pizza’s ready, you tell the chef to come to you once it’s done. That’s long polling.
How It Works: With long polling, you make a request to the server and keep that connection open until new data is available. Once the server has new data, it sends a response, and you immediately send another request. It’s like having a continuous conversation with the server.
Pros:
More efficient than short polling since the server sends data only when there’s something new.
Reduces unnecessary server load.
Cons:
Slightly more complex to implement.
Can still cause some delays compared to WebSockets.
Example Code:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const LongPollingComponent = () => {
const [data, setData] = useState([]);
const [lastId, setLastId] = useState(null);
useEffect(() => {
const fetchData = () => {
axios.get('/api/long-polling', { params: { lastId } })
.then(response => {
if (response.data.length) {
setData(prevData => [...prevData, ...response.data]);
setLastId(response.data[response.data.length - 1].id);
}
fetchData(); // Keep the connection open
})
.catch(error => console.error('Error:', error));
};
fetchData();
return () => {}; // Cleanup if needed
}, [lastId]);
return (
<div>
<h2>Long Polling Data</h2>
<ul>
{data.map(item => <li key={item.id}>{item.text}</li>)}
</ul>
</div>
);
};
export default LongPollingComponent;
3. WebSockets: The “Always On” Approach
Imagine you’re at a party, and you have a walkie-talkie. Whenever someone has something to say, they just speak into the walkie-talkie, and everyone hears it instantly. That’s WebSockets.
How It Works: WebSockets establish a persistent connection between the client and server. Once the connection is open, data can flow freely in both directions without repeatedly opening new connections. It’s like having a continuous, real-time chat with the server.
Pros:
Real-time updates with low latency.
Efficient, with no need for repeated requests.
Cons:
Requires WebSocket support on both client and server.
More complex to implement initially.
Example Code:
import React, { useEffect, useState } from 'react';
import io from 'socket.io-client';
const WebSocketComponent = () => {
const [data, setData] = useState([]);
const socket = io('http://localhost:5000'); // Replace with your server URL
useEffect(() => {
socket.on('new-data', (newData) => {
setData(prevData => [...prevData, newData]);
});
return () => {
socket.disconnect(); // Cleanup on unmount
};
}, [socket]);
return (
<div>
<h2>WebSocket Data</h2>
<ul>
{data.map(item => <li key={item.id}>{item.text}</li>)}
</ul>
</div>
);
};
export default WebSocketComponent;
Wrapping Up
And there you have it—three robust methods for adding real-time features to your React app. Each approach—short polling, long polling, and WebSockets—has its unique advantages and use cases.
Choose the method that best fits your application's requirements and user needs. With the right implementation, you'll enhance user experience and keep your app responsive and engaging.
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Prasad Firame directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
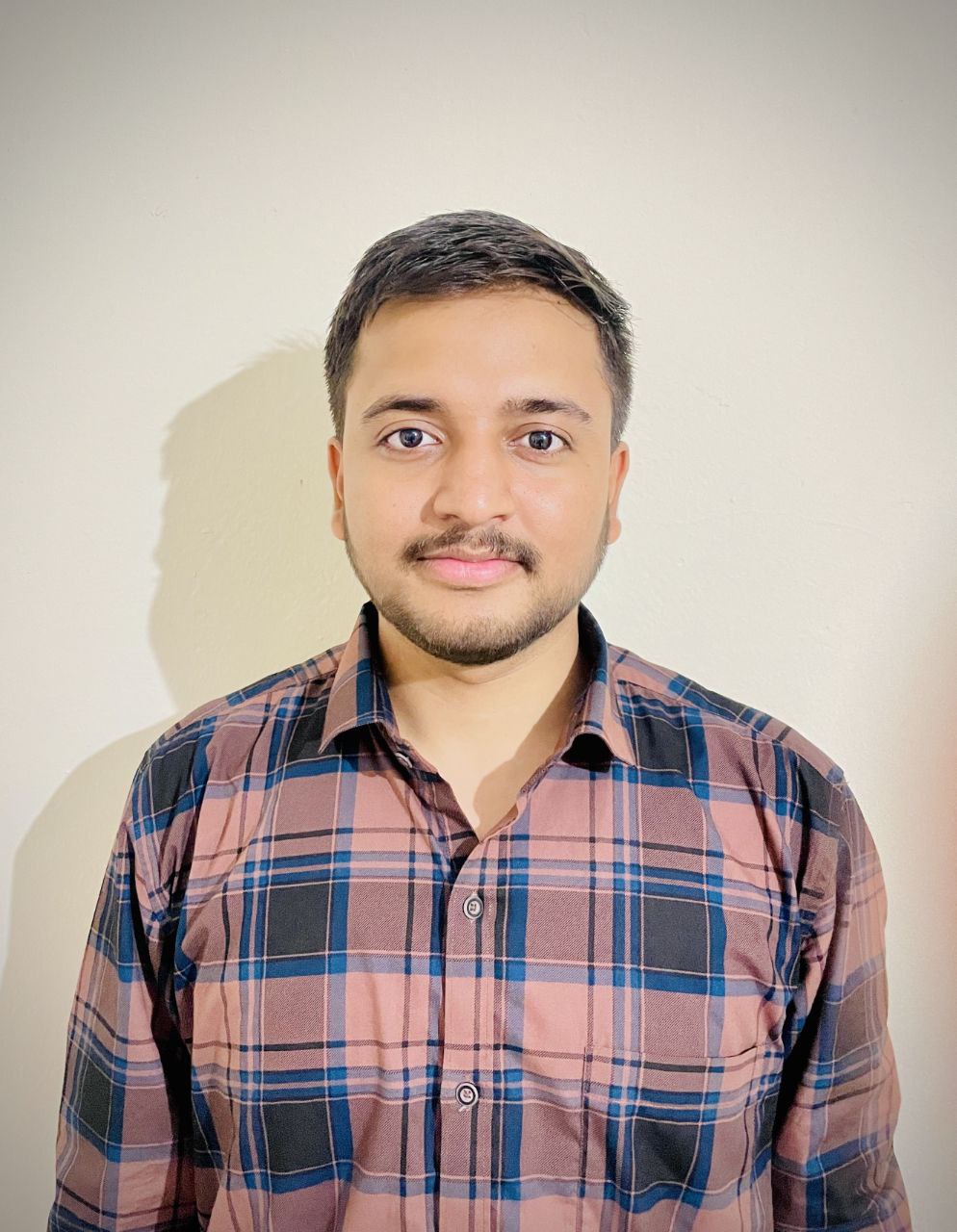
Prasad Firame
Prasad Firame
Tech Enthusiast & Tech Savvy person who's passionate about learning new technologies .