Comprehensive Guide to Spring Data JPA
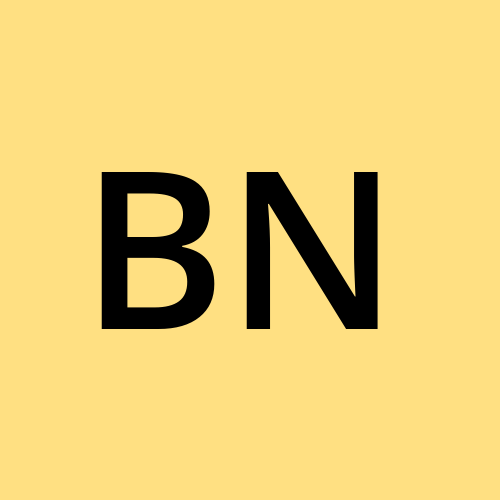
Table of contents
- 1. Introduction to Spring Data JPA
- 2. Getting Started
- 3. Repository Abstraction
- 4. Query Methods
- 5. Custom Queries
- 6. Pagination and Sorting
- 7. Auditing
- 8. Entity Relationships
- One-to-One Relationship
- One-to-Many Relationship
- Many-to-One Relationship
- Many-to-Many Relationship
- Cascade Types and Fetching Strategies
- Cascade Types
- CascadeType.PERSIST
- CascadeType.MERGE
- CascadeType.REMOVE
- CascadeType.REFRESH
- CascadeType.DETACH
- CascadeType.ALL
- Fetching Strategies
- Eager Fetching (FetchType.EAGER)
- Example
- Lazy Fetching (FetchType.LAZY)
- Example
- Choosing the Right Strategy
- Addressing the N+1 Select Problem
- Example with @EntityGraph
- 9. Criteria API
- 10. Specification API
- 11. Native Queries
- 12. Entity Graphs
- 13. Caching
- 14. Transaction Management
- 15. Projections
- 16. Error Handling and Validation
- 17. Testing
- 18. Entity Listeners and Callback Methods
- 19. Data Transfer Objects (DTOs)
- 20. Multi-Tenancy Support
- 21. Query By Example (QBE)
- 22. Optimistic and Pessimistic Locking
- 23. Event Listeners
- 24. Flyway or Liquibase with Spring Data JPA
- 25. Soft Deletes
- 26. Bulk Operations
- 27. Entity Lifecycle Management
- 28. Read-Only Entities
- 29. Composite Keys
- 30. Entity Inheritance Strategies
- 31. UUID and Other Key Generators
- 32. Advanced Query Techniques
- 32. Reactive Spring Data JPA
- 33. Asynchronous Queries
- 34. Data Encryption
- 35. Handling Large Objects (LOBs)
- 36. Integration with NoSQL Databases
- 37. Optimistic Locking with Versioning
- 38. QueryDSL with Spring Data JPA
- 39. Time-Series Data
- 40. Multi-Database Configurations
- 43. Advanced Query Techniques with Streams and Lambdas
- Conclusion
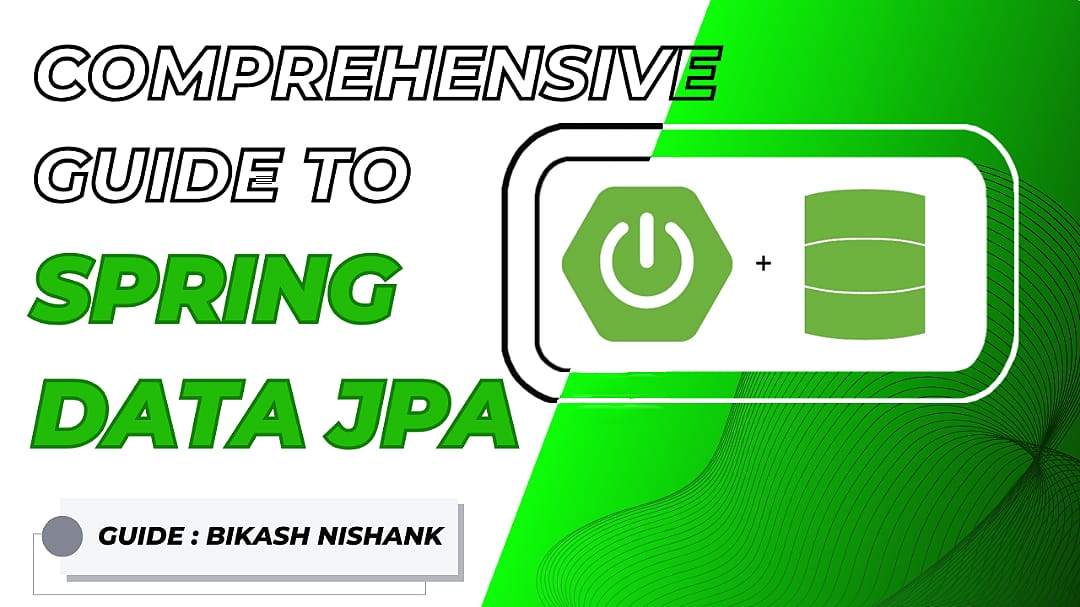
Spring Data JPA is a powerful framework that simplifies the implementation of data access layers for Java applications. Whether you're a beginner or an experienced developer, understanding the key concepts and features of Spring Data JPA can significantly enhance your productivity and enable you to build robust applications. This guide covers 50 essential topics, providing a comprehensive overview of Spring Data JPA.
1. Introduction to Spring Data JPA
Overview of Spring Data JPA
Spring Data JPA is a part of the larger Spring Data family, providing an abstraction over the JPA (Java Persistence API). It simplifies database access and data manipulation using repositories.
Benefits of Using Spring Data JPA
Reduced Boilerplate Code: Simplifies data access layer with repository interfaces.
Consistency: Encourages a consistent approach to data access.
Integration: Seamlessly integrates with Spring Framework and other Spring Data projects.
2. Getting Started
Setting Up the Environment
To get started, set up a Spring Boot project with the necessary dependencies for Spring Data JPA.
Dependencies and Configuration
Add the required dependencies in your pom.xml
or build.gradle
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
Configure the database connection in application.properties
:
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=password
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
3. Repository Abstraction
JpaRepository Interface
The JpaRepository
interface provides CRUD operations and additional JPA-specific operations.
public interface UserRepository extends JpaRepository<User, Long> {
}
CrudRepository and PagingAndSortingRepository Interfaces
CrudRepository: Basic CRUD operations.
PagingAndSortingRepository: Adds pagination and sorting capabilities.
public interface UserRepository extends CrudRepository<User, Long> {
}
public interface UserRepository extends PagingAndSortingRepository<User, Long> {
}
4. Query Methods
Derived Query Methods
Define query methods by following a naming convention:
List<User> findByLastName(String lastName);
@Query Annotation
Customise queries using the @Query
annotation:
@Query("SELECT u FROM User u WHERE u.email = ?1")
User findByEmail(String email);
Named Queries
Define queries with @NamedQuery
annotations on the entity class.
@Entity
@NamedQueries({
@NamedQuery(name = "User.findByEmail",
query = "SELECT u FROM User u WHERE u.email = :email")
})
public class User {
// ...
}
5. Custom Queries
JPQL (Java Persistence Query Language)
Use JPQL for complex queries:
@Query("SELECT u FROM User u WHERE u.age > :age")
List<User> findUsersOlderThan(@Param("age") int age);
Criteria Queries
Build dynamic queries programmatically with the Criteria API.
CriteriaBuilder cb = entityManager.getCriteriaBuilder();
CriteriaQuery<User> query = cb.createQuery(User.class);
Root<User> user = query.from(User.class);
query.select(user).where(cb.equal(user.get("lastName"), "Doe"));
List<User> result = entityManager.createQuery(query).getResultList();
6. Pagination and Sorting
Pageable Interface
Implement pagination with the Pageable
interface:
Page<User> findByLastName(String lastName, Pageable pageable);
Sorting Options
Apply sorting with the Sort
class:
List<User> findAll(Sort.by("lastName").ascending());
7. Auditing
Enabling auditing in Spring Data JPA allows you to automatically capture and store information about entity changes, such as who created or modified an entity and when these changes occurred. This can be particularly useful for tracking historical data and maintaining accountability in your application.
Steps to Enable Auditing in Spring Data JPA
Add Dependencies: Ensure you have the necessary Spring Data JPA dependencies in your
pom.xml
orbuild.gradle
file.Enable Auditing: Enable auditing in your Spring Boot application by adding the
@EnableJpaAuditing
annotation.Create an AuditorAware Implementation: Define a way to get the current user or any other auditing information.
Annotate Your Entities: Use the provided annotations to mark the fields you want to be automatically populated with auditing information.
Annotate Your Entities
Use the following annotations to mark the fields that should be automatically populated with auditing information:
@CreatedDate
: Marks the field that stores the creation timestamp.@LastModifiedDate
: Marks the field that stores the last modification timestamp.@CreatedBy
: Marks the field that stores the user who created the entity.@LastModifiedBy
: Marks the field that stores the user who last modified the entity.
Enabling Auditing in Spring Data JPA
Here's an example entity:
import org.springframework.data.annotation.CreatedBy;
import org.springframework.data.annotation.CreatedDate;
import org.springframework.data.annotation.LastModifiedBy;
import org.springframework.data.annotation.LastModifiedDate;
import org.springframework.data.jpa.domain.support.AuditingEntityListener;
import javax.persistence.*;
import java.time.LocalDateTime;
@Entity
@EntityListeners(AuditingEntityListener.class)
public class MyEntity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@CreatedBy
private String createdBy;
@CreatedDate
private LocalDateTime createdDate;
@LastModifiedBy
private String lastModifiedBy;
@LastModifiedDate
private LocalDateTime lastModifiedDate;
// other fields, getters and setters
}
Full Example
1. Add Dependencies
Add Spring Data JPA dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
2. Enable Auditing
Enable JPA auditing in your application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.data.jpa.repository.config.EnableJpaAuditing;
@SpringBootApplication
@EnableJpaAuditing
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
3. Create AuditorAware Implementation
Create an implementation of AuditorAware
:
import org.springframework.data.domain.AuditorAware;
import java.util.Optional;
public class AuditorAwareImpl implements AuditorAware<String> {
@Override
public Optional<String> getCurrentAuditor() {
// In a real application, return the current user
return Optional.of("admin");
}
}
Register the AuditorAware
implementation:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AuditConfig {
@Bean
public AuditorAware<String> auditorProvider() {
return new AuditorAwareImpl();
}
}
4. Annotate Entities
Annotate your entities to enable auditing:
import org.springframework.data.annotation.CreatedBy;
import org.springframework.data.annotation.CreatedDate;
import org.springframework.data.annotation.LastModifiedBy;
import org.springframework.data.annotation.LastModifiedDate;
import org.springframework.data.jpa.domain.support.AuditingEntityListener;
import javax.persistence.*;
import java.time.LocalDateTime;
@Entity
@EntityListeners(AuditingEntityListener.class)
public class MyEntity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@CreatedBy
private String createdBy;
@CreatedDate
private LocalDateTime createdDate;
@LastModifiedBy
private String lastModifiedBy;
@LastModifiedDate
private LocalDateTime lastModifiedDate;
// other fields, getters and setters
}
8. Entity Relationships
One-to-One Relationship
Map a one-to-one relationship using @OneToOne
annotation:
@OneToOne
@JoinColumn(name = "profile_id")
private Profile profile;
One-to-Many Relationship
Map a one-to-many relationship using @OneToMany
annotation:
@OneToMany(mappedBy = "user")
private List<Order> orders;
Many-to-One Relationship
Map a many-to-one relationship using @ManyToOne
annotation:
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
Many-to-Many Relationship
Map a many-to-many relationship using @ManyToMany
annotation:
@ManyToMany
@JoinTable(
name = "user_role",
joinColumns = @JoinColumn(name = "user_id"),
inverseJoinColumns = @JoinColumn(name = "role_id"))
private Set<Role> roles;
Cascade Types and Fetching Strategies
Cascade Types
Cascade types are used to propagate the changes made to a parent entity to its related child entities. They are specified using the cascade
attribute in a relationship annotation like @OneToMany
, @ManyToMany
, @OneToOne
, or @ManyToOne
. Here are the different types of cascade operations:
CascadeType.PERSIST
When you save the parent, the child entities are also saved.
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(cascade = CascadeType.PERSIST)
private List<Book> books = new ArrayList<>();
}
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
}
// Usage
Author author = new Author();
author.setName("John Doe");
Book book1 = new Book();
book1.setTitle("Book One");
Book book2 = new Book();
book2.setTitle("Book Two");
author.getBooks().add(book1);
author.getBooks().add(book2);
authorRepository.save(author); // This will also save book1 and book2
CascadeType.MERGE
When you update the parent, the child entities are also updated.
// Assuming author and books are already saved in the database
author.setName("Jane Doe");
author.getBooks().get(0).setTitle("Updated Book One");
authorRepository.save(author); // This will update the author's name and the book's title
CascadeType.REMOVE
When you delete the parent, the child entities are also deleted.
// Assuming author and books are already saved in the database
authorRepository.delete(author); // This will also delete all books associated with the author
CascadeType.REFRESH
When you refresh the parent, the child entities are also refreshed.
// Assuming author and books are already saved in the database and have been changed in another transaction
authorRepository.refresh(author); // This will refresh the author's data and also refresh the associated books
CascadeType.DETACH
When you detach the parent, the child entities are also detached.
// Assuming author and books are already saved in the database
entityManager.detach(author); // This will detach the author and all associated books from the persistence context
CascadeType.ALL
Applies all the above operations: PERSIST, MERGE, REMOVE, REFRESH, and DETACH.
@Entity
public class Library {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(cascade = CascadeType.ALL)
private List<Book> books = new ArrayList<>();
}
// Usage
Library library = new Library();
library.setName("Central Library");
Book book1 = new Book();
book1.setTitle("Book One");
Book book2 = new Book();
book2.setTitle("Book Two");
library.getBooks().add(book1);
library.getBooks().add(book2);
libraryRepository.save(library); // This will save the library and books
// Update example
library.setName("Updated Library");
library.getBooks().get(0).setTitle("Updated Book One");
libraryRepository.save(library); // This will update the library and the book
// Delete example
libraryRepository.delete(library); // This will delete the library and books
Fetching Strategies
Fetching strategies determine how and when related entities are loaded from the database. There are two main types of fetching strategies in JPA:
Eager Fetching (
FetchType.EAGER
)Lazy Fetching (
FetchType.LAZY
)
Eager Fetching (FetchType.EAGER
)
Definition: Related entities are loaded immediately along with the parent entity.
Use Case: Use when you always need the related entities and want to avoid additional queries.
Drawback: Can lead to performance issues if related entities have large datasets or if there are many related entities, as it loads everything in one go.
Example
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(fetch = FetchType.EAGER, cascade = CascadeType.ALL)
private List<Book> books = new ArrayList<>();
}
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
}
// Usage
Author author = authorRepository.findById(1L).get(); // Loads author and books
In this example, when you load an Author
, all the associated Book
entities are loaded immediately.
Lazy Fetching (FetchType.LAZY
)
Definition: Related entities are loaded only when they are accessed for the first time.
Use Case: Use when you don't always need the related entities immediately and want to improve performance by loading them on demand.
Drawback: Can lead to the N+1 select problem, where each related entity triggers a separate query.
Example
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(fetch = FetchType.LAZY, cascade = CascadeType.ALL)
private List<Book> books = new ArrayList<>();
}
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
}
// Usage
Author author = authorRepository.findById(1L).get(); // Loads author only
List<Book> books = author.getBooks(); // Loads books only when accessed
In this example, the Book
entities are loaded only when the getBooks()
method is called.
Choosing the Right Strategy
Eager Fetching: Suitable for relationships where related entities are always needed and will be accessed immediately.
Lazy Fetching: Generally preferred to avoid unnecessary data loading and to improve performance.
Addressing the N+1 Select Problem
The N+1 select problem occurs with FetchType.LAZY
when each related entity requires an additional query. This can be mitigated using techniques like:
JPQL Fetch Joins: Fetch related entities in a single query using JPQL.
@Query("SELECT a FROM Author a JOIN FETCH a.books WHERE a.id = :id") Author findAuthorWithBooks(@Param("id") Long id);
Entity Graphs: Define fetch plans to specify which related entities should be loaded.
@EntityGraph(attributePaths = "books") @Query("SELECT a FROM Author a WHERE a.id = :id") Author findAuthorWithBooks(@Param("id") Long id);
Batch Fetching: Load related entities in batches to reduce the number of queries.
@BatchSize(size = 10) @OneToMany(fetch = FetchType.LAZY, cascade = CascadeType.ALL) private List<Book> books = new ArrayList<>();
Example with @EntityGraph
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(fetch = FetchType.LAZY, cascade = CascadeType.ALL)
private List<Book> books = new ArrayList<>();
}
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
}
@Repository
public interface AuthorRepository extends JpaRepository<Author, Long> {
@EntityGraph(attributePaths = "books")
List<Author> findAll();
}
In this example, the @EntityGraph
annotation ensures that books
are eagerly loaded when fetching all Author
entities.
9. Criteria API
The Criteria API is a powerful and flexible way to create dynamic, type-safe queries in JPA (Java Persistence API). Unlike JPQL (Java Persistence Query Language), which is string-based, the Criteria API is object-based and provides compile-time checking of queries.
Key Features
Type-Safety: The Criteria API allows you to construct queries in a type-safe manner.(Note : The Criteria API is designed to be type-safe, meaning that the compiler checks the types of query components (such as fields, conditions, and return values) to ensure correctness. This reduces the likelihood of runtime errors that are common in string-based query languages like JPQL (Java Persistence Query Language).)
Dynamic Queries: You can build queries dynamically at runtime.
No String-Based Queries: Eliminates the need for string-based JPQL queries, reducing the risk of errors.
Basic Components
CriteriaBuilder: The starting point for creating queries.
CriteriaQuery: Represents a query object.
Root: Represents the entity in the FROM clause.
Predicate: Represents conditions in the WHERE clause.
Getting Started
Obtain a CriteriaBuilder: You get this from an
EntityManager
.Create a CriteriaQuery: Use the
CriteriaBuilder
to create aCriteriaQuery
.Define Query Roots: Specify the entities involved in the query.
Construct Query: Build the query by adding conditions and selecting fields.
Execute Query: Use the
EntityManager
to execute the query.
Example: Simple Query
Let's create a simple query to fetch all Employee
entities.
Step 1: Entity Class
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String department;
private Double salary;
// getters and setters
}
Step 2: Creating a Simple Query
import javax.persistence.*;
import javax.persistence.criteria.*;
import java.util.List;
public class CriteriaApiExample {
public static void main(String[] args) {
EntityManagerFactory emf = Persistence.createEntityManagerFactory("example-unit");
EntityManager em = emf.createEntityManager();
// Step 1: Get CriteriaBuilder
CriteriaBuilder cb = em.getCriteriaBuilder();
// Step 2: Create CriteriaQuery
CriteriaQuery<Employee> cq = cb.createQuery(Employee.class);
// Step 3: Define query root
Root<Employee> employee = cq.from(Employee.class);
// Step 4: Specify query selection
cq.select(employee);
// Step 5: Create TypedQuery and execute
TypedQuery<Employee> query = em.createQuery(cq);
List<Employee> employees = query.getResultList();
employees.forEach(emp -> System.out.println(emp.getName()));
em.close();
emf.close();
}
}
Example: Query with Conditions
Let's create a query to fetch employees with a salary greater than a certain amount.
public List<Employee> getEmployeesWithHighSalary(EntityManager em, double salary) {
CriteriaBuilder cb = em.getCriteriaBuilder();
CriteriaQuery<Employee> cq = cb.createQuery(Employee.class);
Root<Employee> employee = cq.from(Employee.class);
// Create a predicate for the salary condition
Predicate salaryCondition = cb.greaterThan(employee.get("salary"), salary);
// Apply the predicate to the query
cq.where(salaryCondition);
TypedQuery<Employee> query = em.createQuery(cq);
return query.getResultList();
}
Example: Query with Multiple Conditions
Let's create a query to fetch employees from a specific department with a salary greater than a certain amount.
public List<Employee> getEmployeesByDepartmentAndSalary(EntityManager em, String department, double salary) {
CriteriaBuilder cb = em.getCriteriaBuilder();
CriteriaQuery<Employee> cq = cb.createQuery(Employee.class);
Root<Employee> employee = cq.from(Employee.class);
// Create predicates for conditions
Predicate departmentCondition = cb.equal(employee.get("department"), department);
Predicate salaryCondition = cb.greaterThan(employee.get("salary"), salary);
// Combine predicates using AND
cq.where(cb.and(departmentCondition, salaryCondition));
TypedQuery<Employee> query = em.createQuery(cq);
return query.getResultList();
}
Example: Query with Sorting
Let's create a query to fetch employees sorted by their name.
public List<Employee> getEmployeesSortedByName(EntityManager em) {
CriteriaBuilder cb = em.getCriteriaBuilder();
CriteriaQuery<Employee> cq = cb.createQuery(Employee.class);
Root<Employee> employee = cq.from(Employee.class);
// Apply sorting
cq.orderBy(cb.asc(employee.get("name")));
TypedQuery<Employee> query = em.createQuery(cq);
return query.getResultList();
}
Example: Query with Joins
Let's create a query to fetch employees along with their departments using joins.
Assuming a Department
Entity
@Entity
public class Department {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "department")
private List<Employee> employees;
// getters and setters
}
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Double salary;
@ManyToOne
@JoinColumn(name = "department_id")
private Department department;
// getters and setters
}
Query with Joins
public List<Employee> getEmployeesWithDepartments(EntityManager em) {
CriteriaBuilder cb = em.getCriteriaBuilder();
CriteriaQuery<Employee> cq = cb.createQuery(Employee.class);
Root<Employee> employee = cq.from(Employee.class);
// Join with Department
employee.join("department");
cq.select(employee);
TypedQuery<Employee> query = em.createQuery(cq);
return query.getResultList();
}
10. Specification API
The Specification API in Spring Data JPA provides a way to create dynamic and reusable queries using a type-safe, object-oriented approach. It builds on the Criteria API, making it easier to write complex queries without using raw SQL or JPQL strings. This API is especially useful for constructing predicates (conditions) that can be combined and reused across different queries.
Key Features
Dynamic Queries: Build queries dynamically at runtime.
Reusability: Define reusable query specifications that can be combined or reused in different parts of the application.
Type-Safety: Ensures that queries are type-safe, reducing the risk of runtime errors.
Basic Components
Specification: An interface that extends
JpaSpecificationExecutor
and provides a method to construct aPredicate
based on certain criteria.JpaSpecificationExecutor: An interface provided by Spring Data JPA that allows the execution of
Specification
objects.
Example: Using the Specification API
Step-by-Step Guide
Entity Classes: Define your entity classes.
Repository Interface: Extend
JpaSpecificationExecutor
in your repository interface.Specification Implementation: Create implementations of the
Specification
interface.
Step 1: Entity Classes
Let's consider two entities, Employee
and Department
.
import javax.persistence.*;
import java.util.List;
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Double salary;
@ManyToOne
@JoinColumn(name = "department_id")
private Department department;
// getters and setters
}
@Entity
public class Department {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "department")
private List<Employee> employees;
// getters and setters
}
Step 2: Repository Interface
Extend JpaSpecificationExecutor
in your repository interface.
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.JpaSpecificationExecutor;
public interface EmployeeRepository extends JpaRepository<Employee, Long>, JpaSpecificationExecutor<Employee> {
}
Step 3: Specification Implementation
Create a specification to find employees by department and minimum salary.
import org.springframework.data.jpa.domain.Specification;
import javax.persistence.criteria.*;
public class EmployeeSpecifications {
public static Specification<Employee> hasDepartment(String departmentName) {
return (root, query, criteriaBuilder) -> {
if (departmentName == null || departmentName.isEmpty()) {
return criteriaBuilder.conjunction();
}
Join<Employee, Department> department = root.join("department");
return criteriaBuilder.equal(department.get("name"), departmentName);
};
}
public static Specification<Employee> hasSalaryGreaterThan(Double salary) {
return (root, query, criteriaBuilder) -> {
if (salary == null) {
return criteriaBuilder.conjunction();
}
return criteriaBuilder.greaterThan(root.get("salary"), salary);
};
}
}
Using Specifications in Service
Combine and use the specifications in a service method.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.jpa.domain.Specification;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class EmployeeService {
@Autowired
private EmployeeRepository employeeRepository;
public List<Employee> findEmployees(String departmentName, Double minSalary) {
Specification<Employee> spec = Specification.where(EmployeeSpecifications.hasDepartment(departmentName))
.and(EmployeeSpecifications.hasSalaryGreaterThan(minSalary));
return employeeRepository.findAll(spec);
}
}
Combining Specifications
Specifications can be combined using logical operators like and
, or
, and not
.
Specification<Employee> spec = Specification.where(EmployeeSpecifications.hasDepartment("Sales"))
.or(EmployeeSpecifications.hasSalaryGreaterThan(50000.0));
11. Native Queries
Native queries in JPA (Java Persistence API) allow you to write SQL queries directly, bypassing the abstraction provided by JPQL (Java Persistence Query Language) or the Criteria API. This can be useful when you need to execute complex queries that are not easily expressed with JPQL or when you need to take advantage of database-specific features.
Key Features of Native Queries
Direct SQL: Write raw SQL queries directly.
Database-Specific Features: Leverage database-specific functions and optimizations.
Complex Queries: Execute complex queries that might be difficult to express with JPQL or Criteria API.
Performance Optimization: Fine-tune queries for better performance.
Using Native Queries
To execute a native query, you typically use the @Query
annotation in Spring Data JPA or the EntityManager
interface.
Example: Using @Query
Annotation
You can define a native query in a Spring Data JPA repository using the @Query
annotation.
Step 1: Define the Entity
Let's use the Employee
entity.
import javax.persistence.*;
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Double salary;
// getters and setters
}
Step 2: Repository Interface with Native Query
Define a repository interface with a native query.
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.CrudRepository;
import org.springframework.data.repository.query.Param;
import java.util.List;
public interface EmployeeRepository extends CrudRepository<Employee, Long> {
@Query(value = "SELECT * FROM Employee WHERE salary > :salary", nativeQuery = true)
List<Employee> findEmployeesWithSalaryGreaterThan(@Param("salary") Double salary);
}
Using the Repository
You can now use the repository to execute the native query.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class EmployeeService {
@Autowired
private EmployeeRepository employeeRepository;
public List<Employee> getHighSalaryEmployees(Double salary) {
return employeeRepository.findEmployeesWithSalaryGreaterThan(salary);
}
}
Example: Using EntityManager
You can also use the EntityManager
to execute native queries.
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import javax.persistence.Query;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class EmployeeNativeQueryRepository {
@PersistenceContext
private EntityManager entityManager;
public List<Employee> findEmployeesWithHighSalary(Double salary) {
Query query = entityManager.createNativeQuery("SELECT * FROM Employee WHERE salary > ?", Employee.class);
query.setParameter(1, salary);
return query.getResultList();
}
}
Example: Complex Query with Database-Specific Features
Suppose you want to use a database-specific function, such as a window function in PostgreSQL.
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import javax.persistence.Query;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class EmployeeNativeQueryRepository {
@PersistenceContext
private EntityManager entityManager;
public List<Object[]> findTopSalariesByDepartment() {
String sql = "SELECT department_id, name, salary, "
+ "RANK() OVER (PARTITION BY department_id ORDER BY salary DESC) as rank "
+ "FROM Employee";
Query query = entityManager.createNativeQuery(sql);
return query.getResultList();
}
}
Handling Result Mapping
Native queries can return different types of results, such as entities, scalar values, or custom projections. You need to map these results accordingly.
Example: Mapping Scalar Results
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import javax.persistence.Query;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class EmployeeNativeQueryRepository {
@PersistenceContext
private EntityManager entityManager;
public List<Object[]> findEmployeeNamesAndSalaries() {
Query query = entityManager.createNativeQuery("SELECT name, salary FROM Employee");
return query.getResultList();
}
}
Example: Mapping to a Custom DTO
Define a DTO (Data Transfer Object) for custom projections.
public class EmployeeDTO {
private String name;
private Double salary;
public EmployeeDTO(String name, Double salary) {
this.name = name;
this.salary = salary;
}
// getters and setters
}
Modify the repository to map results to the DTO.
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import javax.persistence.Query;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class EmployeeNativeQueryRepository {
@PersistenceContext
private EntityManager entityManager;
public List<EmployeeDTO> findEmployeeDTOs() {
Query query = entityManager.createNativeQuery("SELECT name, salary FROM Employee");
List<Object[]> results = query.getResultList();
return results.stream()
.map(row -> new EmployeeDTO((String) row[0], (Double) row[1]))
.collect(Collectors.toList());
}
}
12. Entity Graphs
Entity Graphs in JPA help you control how and when related data (associations) are loaded from the database. They let you specify which parts of your data should be fetched eagerly or lazily, helping you optimize performance and avoid common issues like loading too much data.
Key Points
What is an Entity Graph?
- An entity graph is a way to define how related data should be fetched when you load an entity. It tells JPA which associations should be loaded immediately (eagerly) and which should be loaded later (lazily).
Why Use Entity Graphs?
Performance: Improve performance by controlling the amount of data loaded.
Flexibility: Customise the fetch plan for different scenarios.
Avoid Problems: Prevent issues like the N+1 select problem, where too many queries are run.
How to Use Entity Graphs
1. Define an Entity Graph with Annotations
You can define an entity graph directly in your entity class using the @NamedEntityGraph
annotation.
Example
Suppose you have Employee
and Department
entities:
@Entity
@NamedEntityGraph(name = "Employee.withDepartment",
attributeNodes = @NamedAttributeNode("department"))
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Double salary;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "department_id")
private Department department;
// getters and setters
}
@Entity
public class Department {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "department")
private List<Employee> employees;
// getters and setters
}
In this example, the Employee.withDepartment
entity graph ensures that when you load an Employee
, its Department
is also fetched immediately.
2. Use the Entity Graph in Queries
You can apply the entity graph to your queries to control how the data is fetched.
Example
Using the @Query
annotation:
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.CrudRepository;
public interface EmployeeRepository extends CrudRepository<Employee, Long> {
@Query("SELECT e FROM Employee e")
List<Employee> findAllEmployees();
}
To apply the entity graph programmatically:
import javax.persistence.EntityManager;
import javax.persistence.EntityGraph;
import javax.persistence.PersistenceContext;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class EmployeeRepository {
@PersistenceContext
private EntityManager entityManager;
public List<Employee> findEmployeesWithDepartment() {
EntityGraph<Employee> graph = entityManager.createEntityGraph(Employee.class);
graph.addAttributeNodes("department");
return entityManager.createQuery("SELECT e FROM Employee e", Employee.class)
.setHint("javax.persistence.fetchgraph", graph)
.getResultList();
}
}
13. Caching
Caching in Spring Data JPA is a technique used to improve the performance of data access operations by storing frequently accessed data in memory. This reduces the need to repeatedly fetch data from the database, which can be slower and more resource-intensive. Caching can be implemented at different levels in Spring Data JPA.
Levels of Caching
First-Level Cache (Persistence Context)
Second-Level Cache
Query Cache
1. First-Level Cache (Persistence Context)
What It Is:
- The first-level cache is associated with the Hibernate
Session
or JPAEntityManager
. It automatically caches entities within the context of a single transaction or session.
How It Works:
- When you retrieve an entity from the database, it is stored in the persistence context. If you try to retrieve the same entity again within the same session or transaction, Hibernate will return the cached instance rather than hitting the database.
Characteristics:
Automatic: Managed by the JPA provider (e.g., Hibernate) without additional configuration.
Session Bound: Limited to the scope of the session or transaction.
Example:
// Assuming an Employee entity
Employee employee1 = entityManager.find(Employee.class, 1L);
Employee employee2 = entityManager.find(Employee.class, 1L);
System.out.println(employee1 == employee2); // true, same instance
2. Second-Level Cache
What It Is:
- The second-level cache is a shared cache across multiple sessions or transactions. It stores data beyond the lifecycle of a single session.
How It Works:
Requires explicit configuration and integration with a caching provider (e.g., EHCache, Infinispan).
Entities and their associations are cached across different transactions and sessions.
Configuration:
Enable Caching: Use the
@Cacheable
annotation or configure caching inpersistence.xml
or application properties.Configure Cache Provider: Set up a caching provider like EHCache or Infinispan.
Example:
Add Dependency for EHCache:
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.9.7</version>
</dependency>
Configure Cache Provider inapplication.properties
:
spring.jpa.properties.hibernate.cache.use_second_level_cache=true
spring.jpa.properties.hibernate.cache.region.factory_class=org.hibernate.cache.jcache.JCacheRegionFactory
spring.jpa.properties.javax.persistence.sharedCache.mode=ENABLE_SELECTIVE
Annotate Entity with@Cacheable
:
import org.hibernate.annotations.Cache;
import org.hibernate.annotations.CacheConcurrencyStrategy;
@Entity
@Cacheable
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// getters and setters
}
3. Query Cache
What It Is:
- Caches the results of queries, so subsequent executions of the same query can be served from the cache.
How It Works:
- To use query caching, you need to enable it and configure your cache provider.
Configuration:
Enable Query Cache: Use
spring.jpa.properties
.hibernate.cache.use_query_cache=true
.Configure Cache Provider: Set up your cache provider to handle query caching.
Example:
Configure Query Cache inapplication.properties
:
spring.jpa.properties.hibernate.cache.use_query_cache=true
Annotate Query with@Cacheable
:
import org.springframework.cache.annotation.Cacheable;
public interface EmployeeRepository extends JpaRepository<Employee, Long> {
@Cacheable("employees")
@Query("SELECT e FROM Employee e WHERE e.name = :name")
List<Employee> findByName(@Param("name") String name);
}
14. Transaction Management
Transaction management ensures that a series of operations on the database are completed successfully together or not at all. It helps maintain data consistency and integrity.
Here's a simple breakdown of how transaction management works in Spring Data JPA:
Key Concepts
Transaction: A transaction groups multiple operations into a single unit. It either completes all operations successfully (commit) or none at all (rollback).
ACID Properties: Transactions follow these rules to ensure consistency:
Atomicity: All operations in the transaction succeed or fail together.
Consistency: The database remains in a consistent state before and after the transaction.
Isolation: Transactions do not interfere with each other.
Durability: Once a transaction is committed, its changes are permanent.
Transaction Management with Spring
Spring provides two main ways to handle transactions:
Declarative Transaction Management
Programmatic Transaction Management
1. Declarative Transaction Management
What It Is: This method uses annotations to manage transactions automatically.
How It Works:
You use the
@Transactional
annotation to mark methods that should be managed by transactions.Spring takes care of starting, committing, and rolling back transactions.
Example:
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class EmployeeService {
@Transactional
public void updateEmployeeSalary(Long employeeId, Double newSalary) {
// Find the employee
Employee employee = employeeRepository.findById(employeeId)
.orElseThrow(() -> new RuntimeException("Employee not found"));
// Update salary
employee.setSalary(newSalary);
// Save the updated employee
employeeRepository.save(employee);
// The transaction will be committed if no exception is thrown
}
}
Key Points:
Automatic: Spring handles transactions for you.
Simple: Just annotate methods with
@Transactional
.Rollback on Exceptions: If an exception occurs, the transaction is rolled back automatically.
2. Programmatic Transaction Management
What It Is: This method allows you to manually control transactions using code.
How It Works:
- You use Spring’s
TransactionTemplate
orPlatformTransactionManager
to start, commit, and roll back transactions.
Example:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.PlatformTransactionManager;
import org.springframework.transaction.TransactionDefinition;
import org.springframework.transaction.TransactionStatus;
import org.springframework.transaction.support.DefaultTransactionDefinition;
@Service
public class EmployeeService {
@Autowired
private PlatformTransactionManager transactionManager;
public void updateEmployeeSalary(Long employeeId, Double newSalary) {
DefaultTransactionDefinition def = new DefaultTransactionDefinition();
def.setPropagationBehavior(TransactionDefinition.PROPAGATION_REQUIRED);
TransactionStatus status = transactionManager.getTransaction(def);
try {
Employee employee = employeeRepository.findById(employeeId)
.orElseThrow(() -> new RuntimeException("Employee not found"));
employee.setSalary(newSalary);
employeeRepository.save(employee);
transactionManager.commit(status); // Commit the transaction
} catch (Exception e) {
transactionManager.rollback(status); // Roll back the transaction on error
throw e;
}
}
}
Key Points:
Manual Control: You explicitly start, commit, and roll back transactions.
Flexible: Useful for complex scenarios where you need more control.
15. Projections
Projections in Spring Data JPA allow you to fetch a subset of data from your entities, focusing only on the fields you need. They help improve performance and reduce the amount of data transferred from the database.
Key Concepts
What Is a Projection?
- A projection is a way to retrieve a specific subset of data from the database, rather than loading full entity objects. This is useful when you only need certain fields from an entity.
Types of Projections
Interface Projections
Class-Based Projections
1. Interface Projections
What It Is:
- Interface projections allow you to define an interface with getter methods for the fields you want to retrieve. Spring Data JPA will automatically map the query results to this interface.
How It Works:
Define an interface with methods that match the fields you want to retrieve.
Use this interface in your repository query methods.
Example:
Suppose you have an Employee
entity:
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Double salary;
// getters and setters
}
Define an interface projection:
public interface EmployeeSummary {
String getName();
Double getSalary();
}
Use the projection in a repository:
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.Query;
public interface EmployeeRepository extends JpaRepository<Employee, Long> {
@Query("SELECT e.name AS name, e.salary AS salary FROM Employee e WHERE e.id = ?1")
EmployeeSummary findEmployeeSummaryById(Long id);
}
Key Points:
Lightweight: Only fetches the fields defined in the interface.
Automatic Mapping: Spring Data JPA maps query results to the interface.
2. Class-Based Projections
What It Is:
- Class-based projections use a class with a constructor to hold the projection data. This class is used to return a specific subset of data from a query.
How It Works:
Define a class with a constructor that matches the fields you want to retrieve.
Use this class in your repository query methods.
Example:
Define a projection class:
public class EmployeeSummary {
private String name;
private Double salary;
public EmployeeSummary(String name, Double salary) {
this.name = name;
this.salary = salary;
}
// getters
}
Use the projection in a repository:
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.Query;
public interface EmployeeRepository extends JpaRepository<Employee, Long> {
@Query("SELECT new com.example.EmployeeSummary(e.name, e.salary) FROM Employee e WHERE e.id = ?1")
EmployeeSummary findEmployeeSummaryById(Long id);
}
Key Points:
Customisable: You have control over the projection class and can include any necessary fields.
Constructor Mapping: Query results are mapped to the constructor of the class.
Benefits of Using Projections
Performance Improvement: Reduce the amount of data retrieved from the database, improving query performance.
Reduced Data Transfer: Only transfer the necessary data, which can reduce network load and memory usage.
Simplified Queries: Focus queries on the fields you need, making them easier to understand and maintain.
16. Error Handling and Validation
Error handling and validation ensure that your application behaves correctly and gracefully handles issues or invalid data.
1. Error Handling
What It Is:
- Error handling is about managing and responding to errors that occur during database operations, such as when an entity is not found or a constraint is violated.
Common Errors:
Entity Not Found: Trying to access an entity that does not exist.
Data Integrity Violation: Issues like unique constraint violations or foreign key violations.
How to Handle Errors:
Using Exceptions:
EntityNotFoundException
: Thrown when an entity is not found in the database.DataIntegrityViolationException
: Thrown when a database constraint is violated (e.g., duplicate entry).
Example:
import org.springframework.dao.DataIntegrityViolationException;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Service;
import javax.persistence.EntityNotFoundException;
@Service
public class EmployeeService {
private final EmployeeRepository employeeRepository;
public EmployeeService(EmployeeRepository employeeRepository) {
this.employeeRepository = employeeRepository;
}
public Employee getEmployee(Long id) {
return employeeRepository.findById(id)
.orElseThrow(() -> new EntityNotFoundException("Employee not found with ID " + id));
}
public void updateEmployeeSalary(Long id, Double newSalary) {
try {
Employee employee = employeeRepository.findById(id)
.orElseThrow(() -> new EntityNotFoundException("Employee not found with ID " + id));
employee.setSalary(newSalary);
employeeRepository.save(employee);
} catch (DataIntegrityViolationException e) {
// Handle data integrity violations
throw new RuntimeException("Error updating employee salary: " + e.getMessage(), e);
}
}
}
Custom Exception Handling:
- You can create custom exceptions to provide more meaningful error messages.
Example:
public class CustomException extends RuntimeException {
public CustomException(String message) {
super(message);
}
}
Handling Custom Exceptions in a Controller:
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(CustomException.class)
public ResponseEntity<String> handleCustomException(CustomException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.BAD_REQUEST);
}
}
2. Validation
What It Is:
- Validation ensures that data meets certain criteria before it is processed or stored in the database. It helps prevent invalid or inconsistent data from being saved.
How to Implement Validation:
Using JSR-380 (Bean Validation 2.0):
- Annotations: Use annotations like
@NotNull
,@Size
,@Min
,@Max
, etc., to validate entity fields.
- Annotations: Use annotations like
Example:
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
@Entity
public class Employee {
@Id
private Long id;
@NotNull
@Size(min = 2, max = 50)
private String name;
@NotNull
private Double salary;
// getters and setters
}
Validating Requests in Controllers:
- Use
@Valid
to trigger validation of request bodies in controllers.
- Use
Example:
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.*;
import javax.validation.Valid;
@RestController
@RequestMapping("/employees")
@Validated
public class EmployeeController {
private final EmployeeService employeeService;
public EmployeeController(EmployeeService employeeService) {
this.employeeService = employeeService;
}
@PostMapping
public void createEmployee(@Valid @RequestBody Employee employee) {
employeeService.saveEmployee(employee);
}
}
17. Testing
Unit Testing with Spring Data JPA
Write unit tests for repository methods using mocks.
Integration Testing with H2 Database
Use H2 in-memory database for integration testing:
@RunWith(SpringRunner.class)
@DataJpaTest
public class UserRepositoryTests {
@Autowired
private UserRepository userRepository;
@Test
public void testFindByLastName() {
List<User> users = userRepository.findByLastName("Doe");
assertFalse(users.isEmpty());
}
}
18. Entity Listeners and Callback Methods
Entity Listeners and Callback Methods in Spring Data JPA help you execute custom logic before or after certain lifecycle events of your entities. This is useful for tasks like auditing, data validation, and setting default values.
Key Concepts
Entity Listeners:
Java classes that contain methods to handle specific lifecycle events of entities.
These methods are automatically invoked by the JPA provider (e.g., Hibernate) during the lifecycle of an entity.
Callback Methods:
- Methods defined within your entity class to respond to lifecycle events. They are often used to perform actions like setting default values or validating data before saving.
1. Entity Listeners
What It Is:
- An entity listener is a separate class that contains methods annotated with lifecycle event annotations (e.g.,
@PostPersist
). These methods are called automatically during the entity's lifecycle events.
How It Works:
Define a listener class with methods annotated with JPA lifecycle annotations.
Associate this listener with your entity using the
@EntityListeners
annotation.
Example:
Define an entity listener:
import javax.persistence.PostPersist;
import javax.persistence.PostRemove;
import javax.persistence.PostUpdate;
public class EmployeeEntityListener {
@PostPersist
public void afterInsert(Object o) {
System.out.println("Entity inserted: " + o);
}
@PostUpdate
public void afterUpdate(Object o) {
System.out.println("Entity updated: " + o);
}
@PostRemove
public void afterDelete(Object o) {
System.out.println("Entity deleted: " + o);
}
}
Associate the listener with an entity:
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.EntityListeners;
@Entity
@EntityListeners(EmployeeEntityListener.class)
public class Employee {
@Id
private Long id;
private String name;
private Double salary;
// getters and setters
}
Key Points:
Separation of Concerns: Keeps entity-specific logic out of the entity class itself.
Reusability: Reuse the same listener for multiple entities if needed.
2. Callback Methods
What It Is:
- Callback methods are methods defined directly within the entity class and annotated with lifecycle event annotations. They are called during specific lifecycle events of the entity.
How It Works:
- Annotate methods within your entity class with JPA lifecycle annotations to automatically invoke them at the appropriate times.
Example:
Define callback methods within an entity class:
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.PrePersist;
import javax.persistence.PreUpdate;
@Entity
public class Employee {
@Id
private Long id;
private String name;
private Double salary;
@PrePersist
public void prePersist() {
System.out.println("Before inserting the entity");
// Set default values or perform validation
if (this.salary == null) {
this.salary = 0.0;
}
}
@PreUpdate
public void preUpdate() {
System.out.println("Before updating the entity");
// Perform actions before updating
}
// getters and setters
}
Key Points:
Simplicity: Easy to implement directly within the entity class.
Automatic Invocation: Methods are automatically called at the right time by the JPA provider.
Common Lifecycle Annotations
@PrePersist
: Called before an entity is inserted into the database.@PostPersist
: Called after an entity is inserted into the database.@PreUpdate
: Called before an entity is updated in the database.@PostUpdate
: Called after an entity is updated in the database.@PreRemove
: Called before an entity is deleted from the database.@PostRemove
: Called after an entity is deleted from the database.@PostLoad
: Called after an entity is loaded from the database.
19. Data Transfer Objects (DTOs)
Data Transfer Objects (DTOs) are simple objects used to transfer data between different layers of an application or between systems. They help decouple the internal data representation of an application from the data that is exposed to clients or external systems.
Key Concepts
What Is a DTO?
- A DTO is an object that carries data between processes. It usually contains only fields (properties) and their getters and setters. It does not contain business logic or methods that manipulate the data.
Purpose of DTOs
Decoupling: Separates the internal data model of the application from the external API or data representation.
Data Aggregation: Combines multiple data sources into a single object.
Performance Optimization: Reduces the amount of data transferred over the network or between layers by including only the necessary fields.
When to Use DTOs
API Communication:
- When sending data from a backend to a frontend or from one microservice to another, DTOs ensure only the required data is exposed.
Data Aggregation:
- To aggregate data from multiple entities into a single object for reporting or complex data views.
Data Transformation:
- To transform data into a format that is more suitable for client-side use or for external systems.
20. Multi-Tenancy Support
Multi-tenancy refers to a software architecture where a single instance of an application serves multiple customers, called tenants. Each tenant's data is isolated from others, ensuring privacy and security.
Key Concepts
What Is Multi-Tenancy?
- A system where multiple tenants (customers) share the same application while keeping their data separate.
Types of Multi-Tenancy:
Database Per Tenant: Each tenant has a separate database.
Schema Per Tenant: Each tenant has a separate schema in a shared database.
Table Per Tenant: All tenants share the same tables, but each row is tagged with a tenant identifier.
Implementing Multi-Tenancy in Spring Data JPA
- Database Per Tenant
What It Is:
- Each tenant has a separate database. The application switches databases based on the current tenant.
How to Implement:
- DataSource Routing: Use a routing DataSource that determines the correct DataSource to use based on the current tenant.
Example:
Define a TenantAwareRoutingDataSource
:
import org.springframework.jdbc.datasource.lookup.AbstractRoutingDataSource;
public class TenantAwareRoutingDataSource extends AbstractRoutingDataSource {
@Override
protected Object determineCurrentLookupKey() {
return TenantContext.getCurrentTenant();
}
}
Configure multiple DataSources in a Spring configuration class:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jdbc.datasource.lookup.AbstractRoutingDataSource;
import javax.sql.DataSource;
import java.util.HashMap;
import java.util.Map;
@Configuration
public class DataSourceConfig {
@Bean
public DataSource dataSource() {
TenantAwareRoutingDataSource routingDataSource = new TenantAwareRoutingDataSource();
Map<Object, Object> dataSources = new HashMap<>();
dataSources.put("tenant1", tenant1DataSource());
dataSources.put("tenant2", tenant2DataSource());
routingDataSource.setTargetDataSources(dataSources);
routingDataSource.setDefaultTargetDataSource(tenant1DataSource());
return routingDataSource;
}
public DataSource tenant1DataSource() {
// configure tenant1 DataSource
}
public DataSource tenant2DataSource() {
// configure tenant2 DataSource
}
}
Manage the current tenant using a TenantContext
:
public class TenantContext {
private static ThreadLocal<String> currentTenant = new ThreadLocal<>();
public static void setCurrentTenant(String tenant) {
currentTenant.set(tenant);
}
public static String getCurrentTenant() {
return currentTenant.get();
}
public static void clear() {
currentTenant.remove();
}
}
2. Schema Per Tenant
What It Is:
- Each tenant has a separate schema within a shared database. The application switches schemas based on the current tenant.
How to Implement:
- Use a schema switcher to change the schema dynamically based on the current tenant.
Example:
Use Hibernate's MultiTenantConnectionProvider
and CurrentTenantIdentifierResolver
:
import org.hibernate.engine.jdbc.connections.spi.MultiTenantConnectionProvider;
import org.hibernate.service.jdbc.connections.spi.ConnectionProvider;
import org.hibernate.context.spi.CurrentTenantIdentifierResolver;
import java.sql.Connection;
import java.sql.SQLException;
public class SchemaPerTenantConnectionProvider implements MultiTenantConnectionProvider {
private final ConnectionProvider connectionProvider;
public SchemaPerTenantConnectionProvider(ConnectionProvider connectionProvider) {
this.connectionProvider = connectionProvider;
}
@Override
public Connection getAnyConnection() throws SQLException {
return connectionProvider.getConnection();
}
@Override
public Connection getConnection(String tenantIdentifier) throws SQLException {
Connection connection = getAnyConnection();
connection.createStatement().execute("SET SCHEMA '" + tenantIdentifier + "'");
return connection;
}
@Override
public void releaseAnyConnection(Connection connection) throws SQLException {
connectionProvider.closeConnection(connection);
}
@Override
public void releaseConnection(String tenantIdentifier, Connection connection) throws SQLException {
connectionProvider.closeConnection(connection);
}
// other overridden methods
}
public class SchemaPerTenantIdentifierResolver implements CurrentTenantIdentifierResolver {
@Override
public String resolveCurrentTenantIdentifier() {
return TenantContext.getCurrentTenant();
}
@Override
public boolean validateExistingCurrentSessions() {
return true;
}
}
Configure Hibernate for multi-tenancy:
import org.hibernate.cfg.Environment;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean;
import org.springframework.orm.jpa.vendor.HibernateJpaVendorAdapter;
import javax.sql.DataSource;
import java.util.HashMap;
import java.util.Map;
@Configuration
public class HibernateConfig {
@Autowired
private DataSource dataSource;
@Bean
public LocalContainerEntityManagerFactoryBean entityManagerFactory() {
LocalContainerEntityManagerFactoryBean em = new LocalContainerEntityManagerFactoryBean();
em.setDataSource(dataSource);
em.setPackagesToScan("com.example");
HibernateJpaVendorAdapter vendorAdapter = new HibernateJpaVendorAdapter();
em.setJpaVendorAdapter(vendorAdapter);
Map<String, Object> properties = new HashMap<>();
properties.put(Environment.MULTI_TENANT, MultiTenancyStrategy.SCHEMA);
properties.put(Environment.MULTI_TENANT_CONNECTION_PROVIDER, schemaPerTenantConnectionProvider());
properties.put(Environment.MULTI_TENANT_IDENTIFIER_RESOLVER, schemaPerTenantIdentifierResolver());
em.setJpaPropertyMap(properties);
return em;
}
@Bean
public SchemaPerTenantConnectionProvider schemaPerTenantConnectionProvider() {
return new SchemaPerTenantConnectionProvider(dataSource);
}
@Bean
public SchemaPerTenantIdentifierResolver schemaPerTenantIdentifierResolver() {
return new SchemaPerTenantIdentifierResolver();
}
}
3. Table Per Tenant
What It Is:
- All tenants share the same tables, but each row is tagged with a tenant identifier.
How to Implement:
Add a tenant identifier column to each table.
Use Hibernate's filters to automatically include the tenant identifier in queries.
Example:
Add a tenant identifier column to the Employee
entity:
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class Employee {
@Id
private Long id;
private String name;
private Double salary;
@Column(name = "tenant_id")
private String tenantId;
// getters and setters
}
Configure Hibernate filters:
import org.hibernate.annotations.Filter;
import org.hibernate.annotations.FilterDef;
import org.hibernate.annotations.ParamDef;
@Entity
@FilterDef(name = "tenantFilter", parameters = @ParamDef(name = "tenantId", type = "string"))
@Filter(name = "tenantFilter", condition = "tenant_id = :tenantId")
public class Employee {
@Id
private Long id;
private String name;
private Double salary;
@Column(name = "tenant_id")
private String tenantId;
// getters and setters
}
Enable the filter in the application:
import org.hibernate.Session;
import org.hibernate.engine.spi.SessionFactoryImplementor;
import org.springframework.stereotype.Service;
@Service
public class EmployeeService {
@Autowired
private SessionFactoryImplementor sessionFactory;
public void enableTenantFilter(String tenantId) {
Session session = sessionFactory.getCurrentSession();
session.enableFilter("tenantFilter").setParameter("tenantId", tenantId);
}
}
21. Query By Example (QBE)
Query By Example (QBE) is a query technique that allows you to search for entities by providing an example entity. Spring Data JPA makes it easy to use QBE to perform dynamic queries without writing complex code.
Key Concepts
What Is QBE?
- QBE is a querying approach where you create an example object that contains the properties you want to match. Spring Data JPA then uses this example object to generate the query dynamically.
When to Use QBE?
- Use QBE when you need to perform dynamic queries with varying search criteria. It is especially useful when building search forms or filtering data based on user input.
How to Use QBE in Spring Data JPA
Define an Entity:
- Create an entity class representing the data you want to query.
Example:
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class Employee {
@Id
private Long id;
private String name;
private String department;
private Double salary;
// getters and setters
}
Create a Repository:
- Extend the
JpaRepository
and includeQueryByExampleExecutor
.
- Extend the
Example:
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.repository.query.QueryByExampleExecutor;
public interface EmployeeRepository extends JpaRepository<Employee, Long>, QueryByExampleExecutor<Employee> {
}
Create an Example Object:
- Use
ExampleMatcher
to specify matching rules and create an example object.
- Use
Example:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Example;
import org.springframework.data.domain.ExampleMatcher;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class EmployeeService {
@Autowired
private EmployeeRepository employeeRepository;
public List<Employee> findByExample(Employee exampleEmployee) {
ExampleMatcher matcher = ExampleMatcher.matching()
.withIgnoreNullValues()
.withIgnorePaths("id") // Ignore the id field
.withStringMatcher(ExampleMatcher.StringMatcher.CONTAINING); // Use contains for string matching
Example<Employee> example = Example.of(exampleEmployee, matcher);
return employeeRepository.findAll(example);
}
}
Use the Example Object in Queries:
- Pass the example object to the repository's
findAll
method to perform the query.
- Pass the example object to the repository's
Example:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class EmployeeController {
@Autowired
private EmployeeService employeeService;
@GetMapping("/search")
public List<Employee> searchEmployees(@RequestParam String name, @RequestParam String department) {
Employee exampleEmployee = new Employee();
exampleEmployee.setName(name);
exampleEmployee.setDepartment(department);
return employeeService.findByExample(exampleEmployee);
}
}
ExampleMatcher Options
withIgnoreNullValues
: Ignore properties with null values.withIgnorePaths
: Ignore specific properties.withStringMatcher
: Define string matching strategies (e.g.,EXACT
,STARTING
,ENDING
,CONTAINING
).
Advantages of QBE
Simplicity: Easily create queries by providing an example object.
Flexibility: Dynamically adjust query criteria without changing the query structure.
Maintainability: Reduce the need for complex query methods in your repository.
22. Optimistic and Pessimistic Locking
Optimistic and Pessimistic Locking are techniques used to handle concurrent access to database records. They ensure data consistency and prevent conflicts when multiple transactions attempt to modify the same data simultaneously.
Key Concepts
Why Use Locking?
- Locking mechanisms prevent data corruption and ensure consistency by controlling how concurrent transactions access and modify data.
Optimistic Locking:
Assumes that conflicts are rare and proceeds without locking resources initially.
Uses versioning to detect conflicts at the time of commit.
Pessimistic Locking:
- Assumes that conflicts are likely and locks resources before performing operations to prevent other transactions from accessing the data.
Optimistic Locking
How It Works:
Each entity has a version field (usually an integer or timestamp).
When an entity is read, the version number is also read.
Before an update is committed, the version number is checked. If it has changed since the entity was read, a conflict is detected, and the transaction is rolled back.
Setting Up Optimistic Locking:
Step 1: Add a Version Field:
import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.Version; @Entity public class Employee { @Id private Long id; private String name; private Double salary; @Version private Integer version; // getters and setters }
Step 2: Enable JPA Versioning:
- The
@Version
annotation tells JPA to use this field for optimistic locking.
- The
Handling Version Conflicts:
Example:
import org.springframework.dao.OptimisticLockingFailureException; import org.springframework.stereotype.Service; import org.springframework.transaction.annotation.Transactional; @Service public class EmployeeService { @Autowired private EmployeeRepository employeeRepository; @Transactional public void updateEmployeeSalary(Long id, Double newSalary) { try { Employee employee = employeeRepository.findById(id) .orElseThrow(() -> new RuntimeException("Employee not found")); employee.setSalary(newSalary); employeeRepository.save(employee); } catch (OptimisticLockingFailureException e) { // Handle the conflict System.out.println("Optimistic lock failure. Try again."); } } }
Pessimistic Locking
How It Works:
Locks a database row or table before performing an operation to prevent other transactions from accessing the data.
The lock is held until the transaction is committed or rolled back.
Types of Pessimistic Locks:
Pessimistic Read (
PESSIMISTIC_READ
): Prevents others from obtaining a lock for writing.Pessimistic Write (
PESSIMISTIC_WRITE
): Prevents others from obtaining a lock for reading or writing.Pessimistic Force Increment (
PESSIMISTIC_FORCE_INCREMENT
): Similar toPESSIMISTIC_WRITE
but increments the version field.
Setting Up Pessimistic Locking:
Example:
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import org.springframework.transaction.annotation.Transactional; import javax.persistence.EntityManager; import javax.persistence.LockModeType; @Service public class EmployeeService { @Autowired private EmployeeRepository employeeRepository; @Autowired private EntityManager entityManager; @Transactional public void updateEmployeeSalaryWithLock(Long id, Double newSalary) { Employee employee = entityManager.find(Employee.class, id, LockModeType.PESSIMISTIC_WRITE); if (employee == null) { throw new RuntimeException("Employee not found"); } employee.setSalary(newSalary); employeeRepository.save(employee); } }
Handling Deadlocks and Lock Timeouts:
- Pessimistic locking can lead to deadlocks or lock timeouts. Handle these exceptions by retrying the transaction or using a fallback mechanism.
23. Event Listeners
Event Listeners in Spring Data JPA allow you to perform specific actions in response to changes in your entity's lifecycle. They provide a way to hook into the persistence lifecycle of an entity to execute custom logic at different stages.
Key Concepts
Entity Lifecycle Events:
- These events correspond to different stages in the lifecycle of an entity, such as creation, update, and deletion.
Types of Entity Listeners:
Entity Callbacks: Methods annotated in the entity class itself.
Entity Listeners: Separate listener classes that contain callback methods.
Common Entity Lifecycle Events
PrePersist: Before the entity is persisted (saved for the first time).
PostPersist: After the entity is persisted.
PreUpdate: Before the entity is updated.
PostUpdate: After the entity is updated.
PreRemove: Before the entity is removed (deleted).
PostRemove: After the entity is removed.
PostLoad: After the entity is loaded from the database.
Using Entity Callbacks
Define Callbacks in the Entity Class:
- Annotate methods in your entity class to listen for lifecycle events.
Example:
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.PrePersist;
import javax.persistence.PreUpdate;
import java.time.LocalDateTime;
@Entity
public class Employee {
@Id
private Long id;
private String name;
private Double salary;
private LocalDateTime createdDate;
private LocalDateTime updatedDate;
@PrePersist
public void prePersist() {
createdDate = LocalDateTime.now();
System.out.println("PrePersist called");
}
@PreUpdate
public void preUpdate() {
updatedDate = LocalDateTime.now();
System.out.println("PreUpdate called");
}
// getters and setters
}
Using Entity Listeners
Define a Separate Listener Class:
- Create a separate class with callback methods.
Example:
import javax.persistence.PrePersist;
import javax.persistence.PreUpdate;
public class EmployeeListener {
@PrePersist
public void prePersist(Employee employee) {
employee.setCreatedDate(LocalDateTime.now());
System.out.println("EmployeeListener PrePersist called");
}
@PreUpdate
public void preUpdate(Employee employee) {
employee.setUpdatedDate(LocalDateTime.now());
System.out.println("EmployeeListener PreUpdate called");
}
}
Register the Listener in the Entity Class:
- Use the
@EntityListeners
annotation to register the listener class.
- Use the
Example:
import javax.persistence.Entity;
import javax.persistence.EntityListeners;
import javax.persistence.Id;
import java.time.LocalDateTime;
@Entity
@EntityListeners(EmployeeListener.class)
public class Employee {
@Id
private Long id;
private String name;
private Double salary;
private LocalDateTime createdDate;
private LocalDateTime updatedDate;
// getters and setters
}
Advanced Usage
Using Multiple Listeners:
- You can register multiple listener classes with the
@EntityListeners
annotation.
- You can register multiple listener classes with the
Example:
@Entity
@EntityListeners({EmployeeListener.class, AnotherListener.class})
public class Employee {
// Entity fields and methods
}
Using Spring Components as Listeners:
- You can use Spring components (beans) as entity listeners by registering them in the entity class.
Example:
import org.springframework.stereotype.Component;
import javax.persistence.PrePersist;
import javax.persistence.PreUpdate;
@Component
public class EmployeeSpringListener {
@PrePersist
public void prePersist(Employee employee) {
employee.setCreatedDate(LocalDateTime.now());
System.out.println("EmployeeSpringListener PrePersist called");
}
@PreUpdate
public void preUpdate(Employee employee) {
employee.setUpdatedDate(LocalDateTime.now());
System.out.println("EmployeeSpringListener PreUpdate called");
}
}
Register the Spring component as a listener in the entity class:
@Entity
@EntityListeners(EmployeeSpringListener.class)
public class Employee {
// Entity fields and methods
}
24. Flyway or Liquibase with Spring Data JPA
Flyway and Liquibase are popular database migration tools used to manage and version your database schema changes. They integrate seamlessly with Spring Data JPA, allowing you to automate and manage schema migrations in a consistent and reliable manner.
Key Concepts
Database Migration Tools:
These tools help track and apply schema changes to your database over time.
They ensure that your database schema is in sync with your application code.
Flyway:
A lightweight, open-source database migration tool.
Uses SQL scripts or Java classes to define migrations.
Tracks migrations using a special
flyway_schema_history
table in your database.
Liquibase:
A more feature-rich, open-source database migration tool.
Uses XML, YAML, JSON, or SQL to define migrations.
Tracks migrations using a
DATABASECHANGELOG
table in your database.
25. Soft Deletes
Soft Deletes are a way to "delete" records in a database without actually removing them. Instead of physically deleting the data, a flag is set to mark the record as deleted. This allows the data to be easily restored or retained for historical purposes.
Key Concepts
Why Use Soft Deletes?
Data Retention: Keep records for auditing, reporting, or recovery.
Logical Deletion: Mark records as inactive instead of permanently removing them.
Undo Capability: Easily restore "deleted" records if needed.
How Soft Deletes Work:
Add a
deleted
(or similar) field to your entity.Use this field to filter out "deleted" records in queries.
Implementing Soft Deletes
Add a
deleted
Field to Your Entity:Example:
import javax.persistence.Entity; import javax.persistence.Id; @Entity public class Employee { @Id private Long id; private String name; private String department; private Double salary; private Boolean deleted = false; // getters and setters }
Override Repository Methods:
- Override methods to filter out deleted records in your repository.
Example:
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.Query;
import java.util.List;
public interface EmployeeRepository extends JpaRepository<Employee, Long> {
@Query("SELECT e FROM Employee e WHERE e.deleted = false")
List<Employee> findAllActive();
@Query("SELECT e FROM Employee e WHERE e.id = ?1 AND e.deleted = false")
Employee findByIdAndNotDeleted(Long id);
}
Handle Soft Deletes in Service Layer:
- Update the service layer to handle soft deletes.
Example:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class EmployeeService {
@Autowired
private EmployeeRepository employeeRepository;
public List<Employee> getAllActiveEmployees() {
return employeeRepository.findAllActive();
}
public Employee getActiveEmployeeById(Long id) {
return employeeRepository.findByIdAndNotDeleted(id);
}
@Transactional
public void softDeleteEmployee(Long id) {
Employee employee = employeeRepository.findById(id).orElseThrow(() -> new RuntimeException("Employee not found"));
employee.setDeleted(true);
employeeRepository.save(employee);
}
}
Querying Soft Deleted Records
Find Active Records:
- Use custom queries to find only non-deleted records.
Example:
public List<Employee> findAllActiveEmployees() {
return employeeRepository.findAllActive();
}
Include Deleted Records if Needed:
- If you need to access soft-deleted records, add appropriate queries.
Example:
@Query("SELECT e FROM Employee e WHERE e.deleted = true")
List<Employee> findAllDeleted();
Advanced Configuration
Using
@SQLDelete
and@Where
Annotations:- Hibernate provides annotations to automate soft delete functionality.
Example:
import org.hibernate.annotations.SQLDelete;
import org.hibernate.annotations.Where;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
@SQLDelete(sql = "UPDATE employee SET deleted = true WHERE id = ?")
@Where(clause = "deleted = false")
public class Employee {
@Id
private Long id;
private String name;
private String department;
private Double salary;
private Boolean deleted = false;
// getters and setters
}
Use Global Filters:
- Configure global filters in Hibernate to automatically exclude deleted records from queries.
Example:
import org.hibernate.annotations.Filter;
import org.hibernate.annotations.FilterDef;
import org.hibernate.annotations.ParamDef;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
@FilterDef(name = "deletedFilter", parameters = @ParamDef(name = "isDeleted", type = "boolean"))
@Filter(name = "deletedFilter", condition = "deleted = :isDeleted")
public class Employee {
@Id
private Long id;
private String name;
private String department;
private Double salary;
private Boolean deleted = false;
// getters and setters
}
In your configuration:
import org.springframework.boot.autoconfigure.orm.jpa.HibernatePropertiesCustomizer;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.Map;
@Configuration
public class HibernateConfig {
@Bean
public HibernatePropertiesCustomizer hibernatePropertiesCustomizer() {
return (properties) -> properties.put("hibernate.default_filter", "deletedFilter");
}
}
26. Bulk Operations
Performing Bulk Inserts, Updates, and Deletes
Perform bulk operations efficiently:
@Modifying
@Query("UPDATE User u SET u.status = :status WHERE u.lastLogin < :date")
int updateStatusForInactiveUsers(@Param("status") String status, @Param("date") LocalDate date);
Considerations for Bulk Operations
Consider performance and transactional implications of bulk operations.
27. Entity Lifecycle Management
Entity Lifecycle Management in Spring Data JPA refers to the various states an entity goes through from its creation to its removal, and the specific events or callbacks that can be triggered during these transitions. Understanding these lifecycle events helps you manage entity state transitions effectively.
Key Concepts
Entity States:
New/Transient: An entity instance not yet associated with a persistence context.
Managed/Persistent: An entity instance that is associated with a persistence context.
Detached: An entity instance that was once associated with a persistence context but is now detached.
Removed: An entity instance marked for removal from the database.
Lifecycle Events:
PrePersist: Before the entity is inserted into the database.
PostPersist: After the entity is inserted into the database.
PreUpdate: Before the entity is updated in the database.
PostUpdate: After the entity is updated in the database.
PreRemove: Before the entity is removed from the database.
PostRemove: After the entity is removed from the database.
PostLoad: After the entity has been loaded from the database.
28. Read-Only Entities
Read-Only Entities are entities meant only for reading, not modifying. They're useful for static or rarely changing data like reference tables, configuration settings, or historical records.
Key Concepts
Why Use Read-Only Entities?
Performance: Avoids unnecessary checks for changes.
Data Integrity: Ensures certain data remains unchanged.
Simplified Code: Reduces complexity by avoiding transaction management for read-only data.
How to Implement Read-Only Entities
- Add a Read-Only Entity:
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class Country {
@Id
private Long id;
private String name;
private String code;
// getters and setters
}
- Mark Repository Methods as Read-Only:
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.transaction.annotation.Transactional;
public interface CountryRepository extends JpaRepository<Country, Long> {
@Override
@Transactional(readOnly = true)
List<Country> findAll();
@Override
@Transactional(readOnly = true)
Optional<Country> findById(Long id);
}
- Service Layer Configuration:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class CountryService {
@Autowired
private CountryRepository countryRepository;
@Transactional(readOnly = true)
public List<Country> getAllCountries() {
return countryRepository.findAll();
}
@Transactional(readOnly = true)
public Country getCountryById(Long id) {
return countryRepository.findById(id).orElseThrow(() -> new RuntimeException("Country not found"));
}
}
Benefits
Performance Optimization: No need to track changes, reducing overhead.
Data Integrity: Ensures data remains unchanged.
Simplified Code: Clear indication of immutable data
29. Composite Keys
Composite Keys are primary keys that consist of more than one column. They are used to uniquely identify a record in a table using a combination of multiple fields. This is common in scenarios where a single field is not sufficient to ensure uniqueness.
Key Concepts
Why Use Composite Keys?
Uniqueness: Ensures records are uniquely identified by a combination of fields.
Relational Integrity: Useful in join tables or many-to-many relationships.
How Composite Keys Work:
Define a class to represent the composite key.
Annotate the entity with the composite key class.
Implementing Composite Keys
Define the Composite Key Class:
Use the
@Embeddable
annotation to mark it as a composite key class.Implement
hashCode()
andequals()
methods.
Example Composite Key Class:
import java.io.Serializable;
import java.util.Objects;
import javax.persistence.Embeddable;
@Embeddable
public class EmployeeId implements Serializable {
private Long departmentId;
private Long employeeNumber;
public EmployeeId() {}
public EmployeeId(Long departmentId, Long employeeNumber) {
this.departmentId = departmentId;
this.employeeNumber = employeeNumber;
}
// getters and setters
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
EmployeeId that = (EmployeeId) o;
return Objects.equals(departmentId, that.departmentId) &&
Objects.equals(employeeNumber, that.employeeNumber);
}
@Override
public int hashCode() {
return Objects.hash(departmentId, employeeNumber);
}
}
Annotate the Entity with the Composite Key:
Use
@EmbeddedId
to mark the composite key field in the entity.Use
@MapsId
to map the fields in the composite key class to the entity fields.
Example Entity:
import javax.persistence.*;
@Entity
public class Employee {
@EmbeddedId
private EmployeeId id;
private String name;
private String role;
// getters and setters
public EmployeeId getId() {
return id;
}
public void setId(EmployeeId id) {
this.id = id;
}
// other getters and setters
}
Repository Interface:
- Define the repository interface for the entity using the composite key class.
Example Repository:
import org.springframework.data.jpa.repository.JpaRepository;
public interface EmployeeRepository extends JpaRepository<Employee, EmployeeId> {
}
Benefits of Composite Keys
Uniqueness Across Multiple Columns: Ensures uniqueness when a single field is not sufficient.
Relational Integrity: Useful for composite foreign keys in join tables.
Logical Grouping: Combines multiple related fields to form a unique key.
30. Entity Inheritance Strategies
Entity Inheritance allows you to map an inheritance hierarchy of classes to database tables. This is useful when you have a base class with common properties and several subclasses with specific properties.
Key Concepts
Why Use Entity Inheritance?
Code Reusability: Share common fields and methods in a base class.
Polymorphism: Work with entities polymorphically.
Database Design: Reflect object-oriented inheritance in the database.
Inheritance Strategies:
Single Table (Default): All classes in the hierarchy are mapped to a single table.
Joined: Each class in the hierarchy is mapped to its own table, with relationships between them.
Table per Class: Each class is mapped to its own table with all fields from the base and subclasses.
Implementing Inheritance Strategies
Single Table Strategy:
All classes share a single table.
Use
@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
on the base class.Use
@DiscriminatorColumn
to distinguish between subclasses.
Example:
import javax.persistence.*;
@Entity
@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
@DiscriminatorColumn(name = "type")
public abstract class Vehicle {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String manufacturer;
// getters and setters
}
@Entity
@DiscriminatorValue("Car")
public class Car extends Vehicle {
private int numberOfDoors;
// getters and setters
}
@Entity
@DiscriminatorValue("Bike")
public class Bike extends Vehicle {
private boolean hasPedals;
// getters and setters
}
Joined Strategy:
Each class has its own table.
Use
@Inheritance(strategy = InheritanceType.JOINED)
on the base class.
Example:
import javax.persistence.*;
@Entity
@Inheritance(strategy = InheritanceType.JOINED)
public abstract class Vehicle {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String manufacturer;
// getters and setters
}
@Entity
public class Car extends Vehicle {
private int numberOfDoors;
// getters and setters
}
@Entity
public class Bike extends Vehicle {
private boolean hasPedals;
// getters and setters
}
Table per Class Strategy:
Each class has its own table with all fields from the base and subclasses.
Use
@Inheritance(strategy = InheritanceType.TABLE_PER_CLASS)
on the base class.
Example:
import javax.persistence.*;
@Entity
@Inheritance(strategy = InheritanceType.TABLE_PER_CLASS)
public abstract class Vehicle {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String manufacturer;
// getters and setters
}
@Entity
public class Car extends Vehicle {
private int numberOfDoors;
// getters and setters
}
@Entity
public class Bike extends Vehicle {
private boolean hasPedals;
// getters and setters
}
Benefits of Inheritance Strategies
Single Table: Simple schema, efficient queries but can have sparse columns.
Joined: Normalized schema, reduces redundancy but can have complex joins.
Table per Class: Independent tables, no joins but can be less efficient with redundant columns.
31. UUID and Other Key Generators
Using UUIDs as Primary Keys
Use UUIDs as primary keys for entities:
@Entity
public class User {
@Id
@GeneratedValue
private UUID id;
}
Custom Key Generators
Implement custom key generators for unique identifiers.
32. Advanced Query Techniques
Spring Data JPA provides robust support for creating and executing advanced queries. Some advanced techniques include using window functions, complex SQL queries, and Common Table Expressions (CTEs) through native queries.
Window Functions
Window functions perform calculations across a set of table rows related to the current row. Unlike aggregate functions, they do not collapse rows into a single output row, allowing you to perform operations like running totals, ranking, and moving averages.
Example: Ranking Employees by Salary
SELECT
id,
name,
salary,
RANK() OVER (ORDER BY salary DESC) as salary_rank
FROM
employee;
Implementing in Spring Data JPA:
You can use the @Query
annotation with native SQL:
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.CrudRepository;
public interface EmployeeRepository extends CrudRepository<Employee, Long> {
@Query(value = "SELECT id, name, salary, RANK() OVER (ORDER BY salary DESC) as salary_rank FROM employee", nativeQuery = true)
List<Object[]> findEmployeeSalaryRanks();
}
Complex SQL Queries
Complex SQL queries may include multiple joins, subqueries, and other advanced SQL features.
Example: Finding Departments with Average Salary Above a Threshold
SELECT
d.id,
d.name
FROM
department d
JOIN
employee e ON d.id = e.department_id
GROUP BY
d.id, d.name
HAVING
AVG(e.salary) > 50000;
Implementing in Spring Data JPA:
public interface DepartmentRepository extends CrudRepository<Department, Long> {
@Query(value = "SELECT d.id, d.name FROM department d JOIN employee e ON d.id = e.department_id GROUP BY d.id, d.name HAVING AVG(e.salary) > :threshold", nativeQuery = true)
List<Object[]> findDepartmentsWithHighAvgSalary(@Param("threshold") double threshold);
}
Common Table Expressions (CTEs)
CTEs allow you to define temporary result sets that can be referenced within a SELECT
, INSERT
, UPDATE
, or DELETE
statement.
Example: Using a CTE to Find Employees in Departments with High Average Salaries
WITH HighSalaryDepartments AS (
SELECT
department_id
FROM
employee
GROUP BY
department_id
HAVING
AVG(salary) > 50000
)
SELECT
e.id, e.name, e.salary
FROM
employee e
WHERE
e.department_id IN (SELECT department_id FROM HighSalaryDepartments);
Implementing in Spring Data JPA:
public interface EmployeeRepository extends CrudRepository<Employee, Long> {
@Query(value = "WITH HighSalaryDepartments AS (SELECT department_id FROM employee GROUP BY department_id HAVING AVG(salary) > :threshold) SELECT e.id, e.name, e.salary FROM employee e WHERE e.department_id IN (SELECT department_id FROM HighSalaryDepartments)", nativeQuery = true)
List<Object[]> findEmployeesInHighSalaryDepartments(@Param("threshold") double threshold);
}
32. Reactive Spring Data JPA
Reactive Spring Data JPA is a part of the Spring ecosystem that supports building reactive, non-blocking applications using the reactive programming paradigm. It leverages Project Reactor to provide asynchronous data access with backpressure support.
Key Concepts
Reactive Programming:
Non-Blocking: Operations do not block the execution thread.
Backpressure: Ability to handle flow control, ensuring the system is not overwhelmed with data.
Reactive Repositories:
- Similar to traditional Spring Data repositories but return reactive types like
Mono
andFlux
.
- Similar to traditional Spring Data repositories but return reactive types like
Why Use Reactive Spring Data JPA?
Scalability: Better performance and resource utilization for high-concurrency environments.
Responsiveness: Improved application responsiveness due to non-blocking I/O operations.
Resource Efficiency: Efficiently handles a large number of concurrent connections with minimal threads.
Implementing Reactive Spring Data JPA
Dependencies:
- Add the necessary dependencies for Spring Data JPA and Reactive support in
pom.xml
.
- Add the necessary dependencies for Spring Data JPA and Reactive support in
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-r2dbc</artifactId>
</dependency>
<dependency>
<groupId>io.r2dbc</groupId>
<artifactId>r2dbc-postgresql</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
Configure Database Connectivity:
- Set up your application properties for R2DBC connectivity.
spring.r2dbc.url=r2dbc:postgresql://localhost:5432/mydatabase
spring.r2dbc.username=myuser
spring.r2dbc.password=mypassword
Reactive Entity and Repository:
- Define your entity class as usual.
Example Entity:
import org.springframework.data.annotation.Id;
import org.springframework.data.relational.core.mapping.Table;
@Table("employees")
public class Employee {
@Id
private Long id;
private String name;
private String department;
private Double salary;
// getters and setters
}
Reactive Repository Interface:
- Use
ReactiveCrudRepository
for reactive repositories.
- Use
Example Repository:
import org.springframework.data.repository.reactive.ReactiveCrudRepository;
import reactor.core.publisher.Flux;
public interface EmployeeRepository extends ReactiveCrudRepository<Employee, Long> {
Flux<Employee> findByDepartment(String department);
}
Service Layer:
- Use reactive types like
Mono
andFlux
in your service layer.
- Use reactive types like
Example Service:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
@Service
public class EmployeeService {
@Autowired
private EmployeeRepository employeeRepository;
public Mono<Employee> getEmployeeById(Long id) {
return employeeRepository.findById(id);
}
public Flux<Employee> getEmployeesByDepartment(String department) {
return employeeRepository.findByDepartment(department);
}
public Mono<Employee> saveEmployee(Employee employee) {
return employeeRepository.save(employee);
}
public Mono<Void> deleteEmployee(Long id) {
return employeeRepository.deleteById(id);
}
}
Controller Layer:
- Create a reactive REST controller.
Example Controller:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
@RestController
@RequestMapping("/employees")
public class EmployeeController {
@Autowired
private EmployeeService employeeService;
@GetMapping("/{id}")
public Mono<Employee> getEmployeeById(@PathVariable Long id) {
return employeeService.getEmployeeById(id);
}
@GetMapping("/department/{department}")
public Flux<Employee> getEmployeesByDepartment(@PathVariable String department) {
return employeeService.getEmployeesByDepartment(department);
}
@PostMapping
public Mono<Employee> createEmployee(@RequestBody Employee employee) {
return employeeService.saveEmployee(employee);
}
@DeleteMapping("/{id}")
public Mono<Void> deleteEmployee(@PathVariable Long id) {
return employeeService.deleteEmployee(id);
}
}
33. Asynchronous Queries
Asynchronous Queries allow you to execute database operations without blocking the execution thread. This can improve the responsiveness and scalability of your application, especially when dealing with I/O-bound tasks like database access.
Key Concepts
Asynchronous Programming:
Non-blocking: Operations do not block the execution thread, allowing other tasks to proceed.
Concurrency: Multiple operations can be performed concurrently, improving throughput.
CompletableFuture:
Java's
CompletableFuture
is used to handle asynchronous results in Spring Data JPA.Methods return
CompletableFuture
instead of the actual result.
Why Use Asynchronous Queries?
Improved Responsiveness: Keep the application responsive by not blocking threads on I/O operations.
Better Resource Utilization: Efficiently utilize system resources, allowing for higher concurrency.
Scalability: Handle more requests concurrently, making your application more scalable.
Implementing Asynchronous Queries
Enable Async Support:
- Add
@EnableAsync
to your main application class or a configuration class.
- Add
Example:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableAsync;
@SpringBootApplication
@EnableAsync
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Define Asynchronous Repository Methods:
- Use
@Async
on repository methods and returnCompletableFuture
.
- Use
Example Repository:
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.scheduling.annotation.Async;
import java.util.concurrent.CompletableFuture;
public interface EmployeeRepository extends JpaRepository<Employee, Long> {
@Async
CompletableFuture<Employee> findById(Long id);
@Async
CompletableFuture<List<Employee>> findByDepartment(String department);
}
Service Layer:
- Call the asynchronous repository methods in your service layer.
Example Service:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.concurrent.CompletableFuture;
@Service
public class EmployeeService {
@Autowired
private EmployeeRepository employeeRepository;
public CompletableFuture<Employee> getEmployeeById(Long id) {
return employeeRepository.findById(id);
}
public CompletableFuture<List<Employee>> getEmployeesByDepartment(String department) {
return employeeRepository.findByDepartment(department);
}
}
Controller Layer:
- Use
@Async
to handle asynchronous responses in your controller.
- Use
Example Controller:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
import java.util.concurrent.CompletableFuture;
@RestController
@RequestMapping("/employees")
public class EmployeeController {
@Autowired
private EmployeeService employeeService;
@GetMapping("/{id}")
public CompletableFuture<Employee> getEmployeeById(@PathVariable Long id) {
return employeeService.getEmployeeById(id);
}
@GetMapping("/department/{department}")
public CompletableFuture<List<Employee>> getEmployeesByDepartment(@PathVariable String department) {
return employeeService.getEmployeesByDepartment(department);
}
@PostMapping
public CompletableFuture<Employee> createEmployee(@RequestBody Employee employee) {
return employeeService.saveEmployee(employee);
}
@DeleteMapping("/{id}")
public CompletableFuture<Void> deleteEmployee(@PathVariable Long id) {
return employeeService.deleteEmployee(id);
}
}
Benefits of Asynchronous Queries
Enhanced Performance: Non-blocking operations allow handling more requests simultaneously.
Reduced Latency: Faster response times by not waiting for I/O operations to complete.
Better User Experience: Improved responsiveness leads to a smoother user experience.
34. Data Encryption
Data Encryption is the process of converting data into a secure format that is not readable without proper authorization. It is crucial for protecting sensitive information from unauthorized access or breaches.
Key Concepts
Encryption:
Symmetric Encryption: Uses the same key for both encryption and decryption. Fast and suitable for large volumes of data.
Asymmetric Encryption: Uses a pair of keys (public and private). Slower but provides a higher level of security, typically used for encrypting small pieces of data like keys.
Why Use Data Encryption?:
Data Security: Protect sensitive information from unauthorized access.
Compliance: Meet regulatory requirements (e.g., GDPR, HIPAA).
Integrity: Ensure data has not been tampered with.
Implementing Data Encryption in Spring Data JPA
Encryption Libraries:
- Use libraries like Jasypt or Spring Security Crypto for easy integration.
Basic Example with Jasypt:
Add Jasypt Dependency:
<dependency> <groupId>org.jasypt</groupId> <artifactId>jasypt-spring-boot-starter</artifactId> <version>3.0.4</version> </dependency>
Configure Jasypt:
Add configuration properties to your
application.properties
orapplication.yml
.jasypt.encryptor.password=your-secret-key
Encrypt Data:
Use
@EncryptablePropertySource
to encrypt sensitive data.import org.jasypt.encryption.StringEncryptor; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; @Service public class EncryptionService { @Autowired private StringEncryptor stringEncryptor; public String encrypt(String plaintext) { return stringEncryptor.encrypt(plaintext); } public String decrypt(String ciphertext) { return stringEncryptor.decrypt(ciphertext); } }
Encrypt Entity Fields:
Encrypt sensitive fields in your entity before persisting.
import javax.persistence.*; import org.jasypt.encryption.StringEncryptor; @Entity public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; @Transient private String password; private String encryptedPassword; @PostLoad @PostPersist @PostUpdate public void encryptPassword() { if (password != null) { this.encryptedPassword = encryptor.encrypt(password); } } @PrePersist @PreUpdate public void decryptPassword() { if (encryptedPassword != null) { this.password = encryptor.decrypt(encryptedPassword); } } // getters and setters }
Basic Example with Spring Security Crypto:
Add Dependency:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency>
Encrypt Data:
Use
Cipher
fromjavax.crypto
for encryption and decryption.import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; import java.util.Base64; public class EncryptionUtil { private static final String ALGORITHM = "AES"; private static final String TRANSFORMATION = "AES"; public static String encrypt(String plainText, SecretKey key) throws Exception { Cipher cipher = Cipher.getInstance(TRANSFORMATION); cipher.init(Cipher.ENCRYPT_MODE, key); byte[] encryptedBytes = cipher.doFinal(plainText.getBytes()); return Base64.getEncoder().encodeToString(encryptedBytes); } public static String decrypt(String cipherText, SecretKey key) throws Exception { Cipher cipher = Cipher.getInstance(TRANSFORMATION); cipher.init(Cipher.DECRYPT_MODE, key); byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(cipherText)); return new String(decryptedBytes); } public static SecretKey generateKey() throws Exception { KeyGenerator keyGenerator = KeyGenerator.getInstance(ALGORITHM); keyGenerator.init(128); return keyGenerator.generateKey(); } }
Benefits of Data Encryption
Data Protection: Ensures that even if data is accessed by unauthorized parties, it remains unreadable.
Regulatory Compliance: Helps meet legal and regulatory requirements for data protection.
Integrity and Confidentiality: Maintains the confidentiality and integrity of sensitive information.
35. Handling Large Objects (LOBs)
Storing and Retrieving Large Binary and Text Data
Handle large binary and text data with the @Lob
annotation:
@Lob
private byte[] data;
@Lob Annotation Usage
Use the @Lob
annotation for large objects in your entities.
36. Integration with NoSQL Databases
Using Spring Data JPA with NoSQL Databases like MongoDB
Integrate Spring Data JPA with NoSQL databases such as MongoDB.
Polyglot Persistence Strategies
Implement polyglot persistence strategies for diverse data needs.
37. Optimistic Locking with Versioning
Optimistic Locking with Versioning in Spring Data JPA uses a version field to manage concurrent updates to entities. When an entity is updated, JPA checks that the version has not changed since it was last read. If a conflict is detected (i.e., the version has changed), an OptimisticLockException
is thrown.
Define Version Field: Use
@Version
to annotate the version field in your entity.Handle Exceptions: Manage
OptimisticLockException
to address concurrent modification issues.Benefits: Reduces lock contention, improves performance, and scales well with high read-to-write ratios.
Implementing Versioning for Concurrency Control
Use versioning for concurrency control with the @Version
annotation:
@Version
private Long version;
38. QueryDSL with Spring Data JPA
QueryDSL is a framework that provides a type-safe way to construct queries in Java. It integrates with Spring Data JPA to build queries dynamically using a fluent API, avoiding the need for string-based JPQL or SQL queries.
Key Concepts
Type-Safe Queries:
Avoid runtime errors due to incorrect query syntax by leveraging the type safety provided by QueryDSL.
Provides compile-time checking of query structure.
Fluent API:
- Build queries using a fluent API, making it easier to construct and manage complex queries.
QueryDSL Components:
QueryDSL JPA: For JPA-based querying.
QueryDSL SQL: For SQL-based querying.
QueryDSL MongoDB: For MongoDB querying.
Setting Up QueryDSL with Spring Data JPA
Add Dependencies:
- Include the QueryDSL dependencies in your
pom.xml
.
- Include the QueryDSL dependencies in your
Example Dependencies:
<dependency>
<groupId>com.querydsl</groupId>
<artifactId>querydsl-jpa</artifactId>
<version>5.0.0</version>
</dependency>
<dependency>
<groupId>com.querydsl</groupId>
<artifactId>querydsl-apt</artifactId>
<version>5.0.0</version>
<scope>provided</scope>
</dependency>
Configure the Annotation Processor:
- Add the QueryDSL annotation processor to your
pom.xml
to generate the Q-type classes.
- Add the QueryDSL annotation processor to your
Example Configuration:
<build>
<plugins>
<plugin>
<groupId>com.mysema.maven</groupId>
<artifactId>apt-maven-plugin</artifactId>
<version>1.1.3</version>
<executions>
<execution>
<goals>
<goal>process</goal>
</goals>
</execution>
</executions>
<configuration>
<outputDirectory>target/generated-sources/java</outputDirectory>
</configuration>
</plugin>
</plugins>
</build>
Generate Q-Types:
- QueryDSL generates Q-types (e.g.,
QEmployee
) for your entities. These are used to build type-safe queries.
- QueryDSL generates Q-types (e.g.,
Use QueryDSL in Repositories:
- Create a repository that uses QueryDSL for dynamic querying.
Example Repository:
import com.querydsl.core.BooleanBuilder;
import com.querydsl.jpa.impl.JPAQueryFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import javax.persistence.EntityManager;
import java.util.List;
@Repository
public class EmployeeRepositoryCustomImpl implements EmployeeRepositoryCustom {
@Autowired
private EntityManager entityManager;
@Override
public List<Employee> findEmployeesByCriteria(String name, Double salary) {
JPAQueryFactory queryFactory = new JPAQueryFactory(entityManager);
QEmployee qEmployee = QEmployee.employee;
BooleanBuilder builder = new BooleanBuilder();
if (name != null) {
builder.and(qEmployee.name.eq(name));
}
if (salary != null) {
builder.and(qEmployee.salary.gt(salary));
}
return queryFactory.selectFrom(qEmployee)
.where(builder)
.fetch();
}
}
Custom Repository Interface:
import java.util.List;
public interface EmployeeRepositoryCustom {
List<Employee> findEmployeesByCriteria(String name, Double salary);
}
Regular Repository Interface:
import org.springframework.data.jpa.repository.JpaRepository;
public interface EmployeeRepository extends JpaRepository<Employee, Long>, EmployeeRepositoryCustom {
}
Integrate with Spring Data JPA:
- Extend
JpaRepository
and include your custom repository interface to use QueryDSL.
- Extend
Benefits of Using QueryDSL
Type Safety: Avoids errors related to incorrect query syntax.
Dynamic Queries: Easily build complex, dynamic queries using a fluent API.
Maintainability: Easier to refactor and maintain queries.
39. Time-Series Data
Time-Series Data is a sequence of data points collected or recorded at regular time intervals. It's commonly used in various fields such as finance, IoT, healthcare, and more, to analyze trends, patterns, and changes over time.
Key Concepts
Data Points:
Individual measurements or observations collected at specific time intervals.
Examples: stock prices, temperature readings, or server response times.
Time Intervals:
- The time gap between consecutive data points, which can be seconds, minutes, hours, days, etc.
Time Index:
- Each data point is associated with a timestamp that indicates when it was recorded.
Common Uses of Time-Series Data
Financial Markets:
- Track stock prices, trading volumes, and other financial indicators over time.
IoT and Sensors:
- Monitor temperature, humidity, or equipment performance continuously.
Healthcare:
- Record patient vitals like heart rate or blood pressure over time.
Web Analytics:
- Analyze website traffic, user interactions, and system performance.
Key Features and Challenges
Trends:
Long-term movements or patterns in the data.
Example: a steady increase in a company’s stock price over several years.
Seasonality:
Repeating patterns or cycles that occur at regular intervals.
Example: increased sales during holiday seasons.
Noise:
Random variations or fluctuations that do not reflect actual trends or patterns.
Example: daily fluctuations in stock prices due to market noise.
Anomalies:
Unexpected deviations from typical patterns.
Example: a sudden spike in server response time due to a system outage.
40. Multi-Database Configurations
Multi-Database Configurations involve using multiple databases within a single Spring Boot application. This setup can be useful for various reasons, such as integrating with legacy systems, handling different types of data, or separating read and write operations.
Key Concepts
Data Sources:
Primary Data Source: The main database used for most operations.
Secondary Data Sources: Additional databases used for specific purposes, such as read replicas or different data stores.
Multiple
EntityManagerFactory
:- Each data source requires a separate
EntityManagerFactory
, which is responsible for creatingEntityManager
instances.
- Each data source requires a separate
Transaction Management:
- Transactions need to be managed across multiple data sources, which may require custom configurations.
Configuration Steps
1. Add Dependencies
Ensure you have the necessary dependencies in your pom.xml
or build.gradle
for Spring Data JPA and the specific databases you are using.
Example (Maven):
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
</dependency>
<dependency>
<groupId>com.mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
2. Define Configuration Classes
Create configuration classes for each data source. Each configuration class will define a DataSource
, EntityManagerFactory
, and TransactionManager
.
Example Configuration for Primary Database:
@Configuration
@EnableTransactionManagement
public class PrimaryDataSourceConfig {
@Bean
@Primary
@ConfigurationProperties(prefix = "spring.datasource.primary")
public DataSource primaryDataSource() {
return DataSourceBuilder.create().build();
}
@Bean
@Primary
public LocalContainerEntityManagerFactoryBean primaryEntityManagerFactory(
EntityManagerFactoryBuilder builder, @Qualifier("primaryDataSource") DataSource dataSource) {
return builder
.dataSource(dataSource)
.packages("com.example.primary") // Packages to scan for entities
.persistenceUnit("primary")
.build();
}
@Bean
@Primary
public PlatformTransactionManager primaryTransactionManager(
@Qualifier("primaryEntityManagerFactory") EntityManagerFactory entityManagerFactory) {
return new JpaTransactionManager(entityManagerFactory);
}
}
Example Configuration for Secondary Database:
@Configuration
@EnableTransactionManagement
public class SecondaryDataSourceConfig {
@Bean
@ConfigurationProperties(prefix = "spring.datasource.secondary")
public DataSource secondaryDataSource() {
return DataSourceBuilder.create().build();
}
@Bean
public LocalContainerEntityManagerFactoryBean secondaryEntityManagerFactory(
EntityManagerFactoryBuilder builder, @Qualifier("secondaryDataSource") DataSource dataSource) {
return builder
.dataSource(dataSource)
.packages("com.example.secondary") // Packages to scan for entities
.persistenceUnit("secondary")
.build();
}
@Bean
public PlatformTransactionManager secondaryTransactionManager(
@Qualifier("secondaryEntityManagerFactory") EntityManagerFactory entityManagerFactory) {
return new JpaTransactionManager(entityManagerFactory);
}
}
3. Configure Application Properties
Define properties for each data source in your application.properties
or application.yml
.
Example (application.properties
):
# Primary DataSource
spring.datasource.primary.url=jdbc:postgresql://localhost:5432/primarydb
spring.datasource.primary.username=primaryuser
spring.datasource.primary.password=primarypassword
spring.datasource.primary.driver-class-name=org.postgresql.Driver
# Secondary DataSource
spring.datasource.secondary.url=jdbc:mysql://localhost:3306/secondarydb
spring.datasource.secondary.username=secondaryuser
spring.datasource.secondary.password=secondarypassword
spring.datasource.secondary.driver-class-name=com.mysql.cj.jdbc.Driver
4. Define Repositories
Create repository interfaces for entities associated with each data source.
Example Repository for Primary Database:
public interface PrimaryEntityRepository extends JpaRepository<PrimaryEntity, Long> {
}
Example Repository for Secondary Database:
public interface SecondaryEntityRepository extends JpaRepository<SecondaryEntity, Long> {
}
5. Use@Transactional
Use the @Transactional
annotation to manage transactions. You can specify which transaction manager to use by providing the transactionManager
attribute.
Example:
@Service
public class MyService {
@Autowired
private PrimaryEntityRepository primaryRepository;
@Autowired
private SecondaryEntityRepository secondaryRepository;
@Transactional("primaryTransactionManager")
public void performPrimaryDatabaseOperation() {
// Perform operations on the primary database
}
@Transactional("secondaryTransactionManager")
public void performSecondaryDatabaseOperation() {
// Perform operations on the secondary database
}
}
43. Advanced Query Techniques with Streams and Lambdas
Using Java 8 Streams and Lambdas with Spring Data JPA
Leverage Java 8 streams and lambdas for advanced query techniques:
List<User> users = userRepository.findAll().stream()
.filter(user -> user.getAge() > 30)
.collect(Collectors.toList());
Functional Programming Concepts in Spring Data JPA
Apply functional programming concepts in your data access layer.
Conclusion
Spring Data JPA is an indispensable framework for Java developers looking to streamline the implementation of data access layers in their applications. By providing a comprehensive abstraction over JPA, it significantly reduces boilerplate code, ensures consistency, and integrates seamlessly with the broader Spring ecosystem. This guide has covered a wide array of essential topics, from basic setup and repository abstractions to advanced features like custom queries, auditing, entity relationships, and multi-tenancy support. Whether you're dealing with complex query requirements, optimizing performance with caching and projections, or ensuring data integrity with transaction management and locking mechanisms, Spring Data JPA offers robust solutions. By leveraging these features, developers can build scalable, maintainable, and high-performance applications, making Spring Data JPA a powerful tool in the modern Java development landscape.
Subscribe to my newsletter
Read articles from Bikash Nishank directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
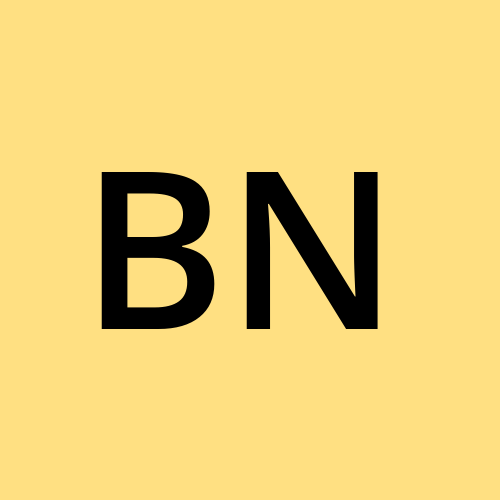