Mastering Concurrency Control with UnitOfWork Pattern in EF Core
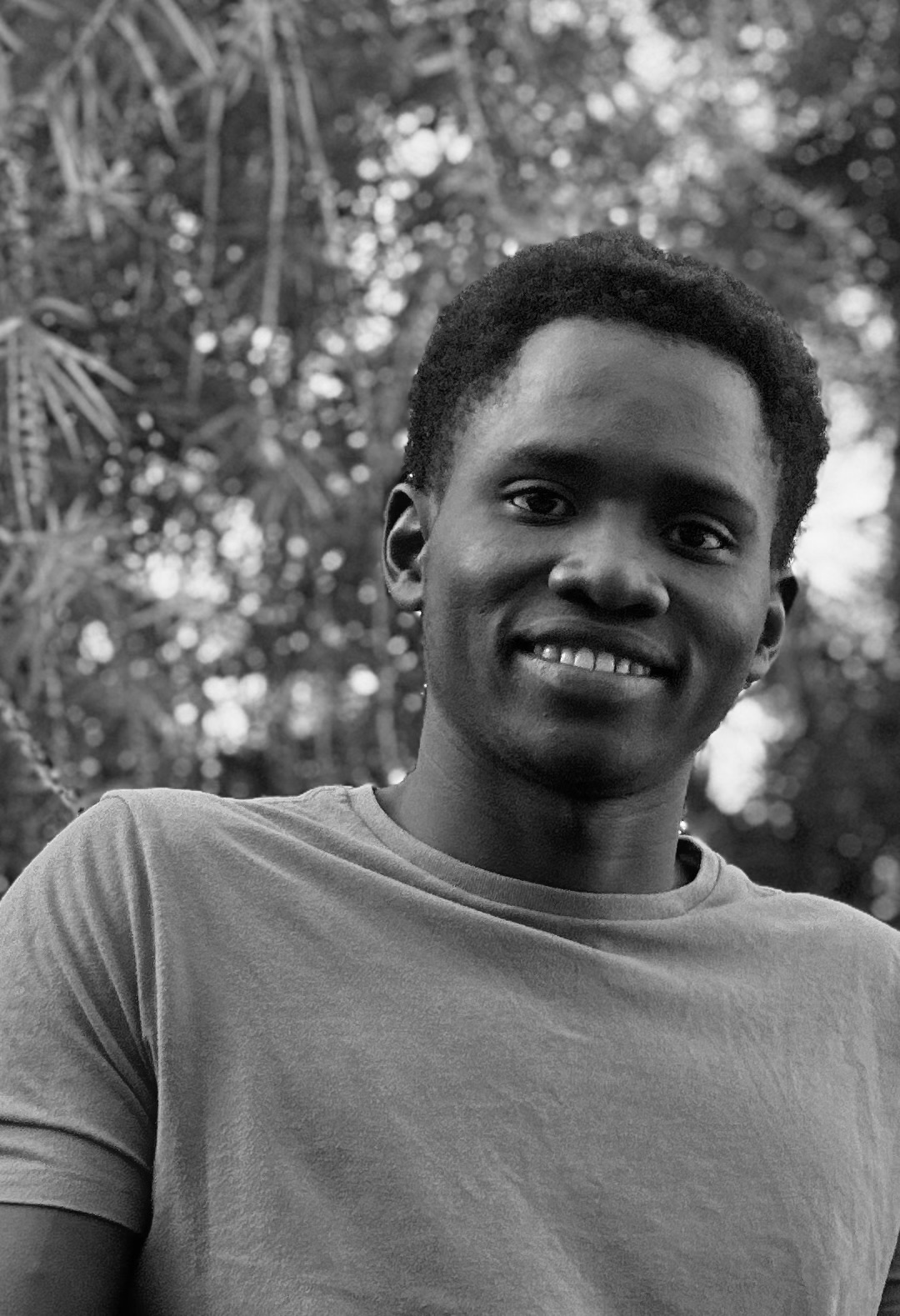
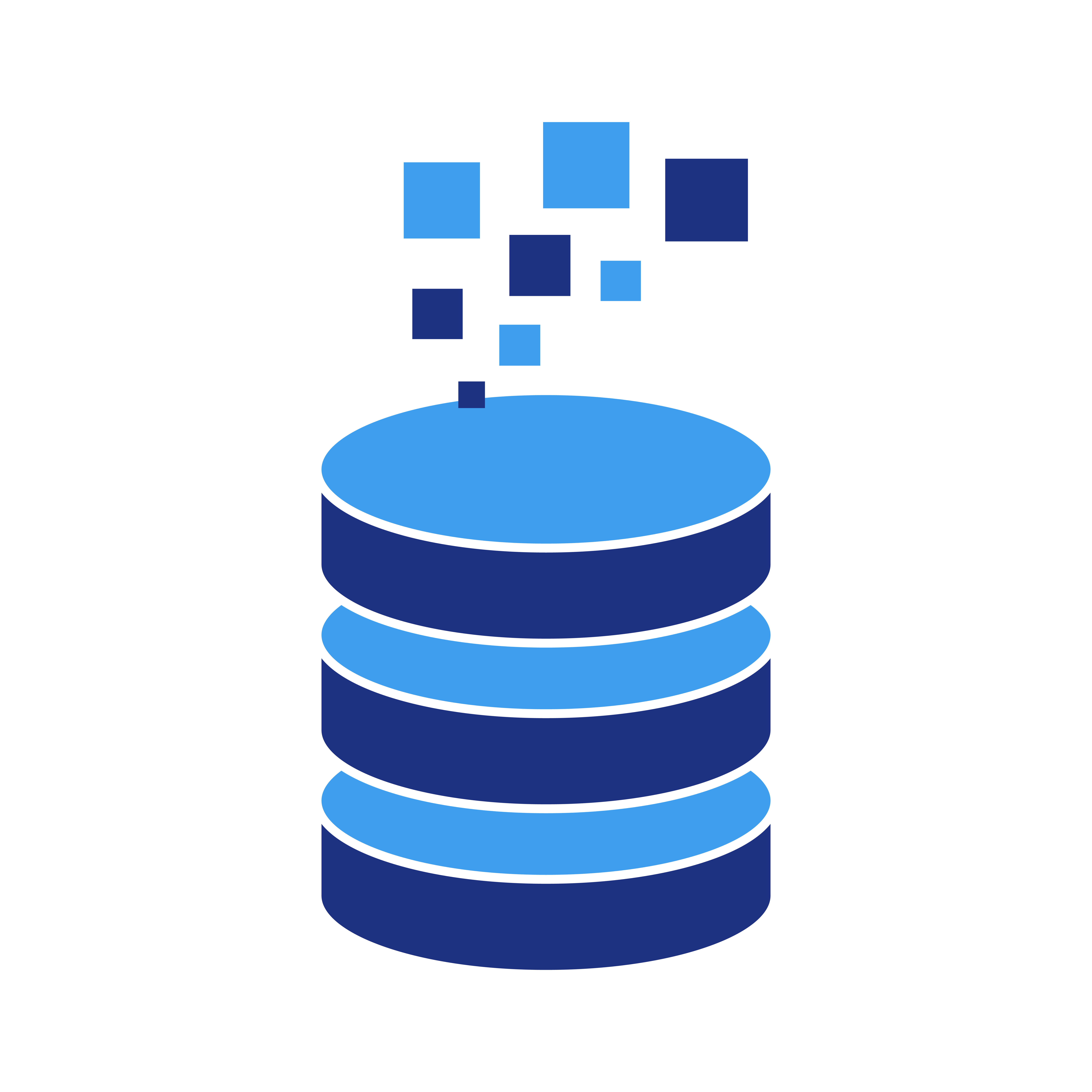
Introduction
In today's fast-paced web applications, ensuring data integrity amidst concurrent operations is paramount. Entity Framework Core (EF Core) provides a robust framework for data access, but handling concurrency requires careful consideration. This article introduces the UnitOfWork
pattern, a powerful technique for managing transactions and ensuring data integrity in EF Core applications.
Understanding Concurrency Issues
Before diving into the solution, let's understand the problem. Imagine a scenario where multiple users update the same record simultaneously. Without proper concurrency control, one user's changes might overwrite another's, leading to lost updates and frustrated users.
The UnitOfWork Pattern
The UnitOfWork
pattern encapsulates the operations related to a single logical unit of work, such as a transaction, within a single class. This pattern is particularly useful for managing transactions across multiple repositories or services, ensuring atomicity and consistency.
Defining IUnitOfWork
First, we define an interface IUnitOfWork
that outlines the necessary methods for managing transactions.
public interface IUnitOfWork : IDisposable
{
Task<int> SaveChangesAsync();
Task BeginTransactionAsync();
Task CommitTransactionAsync();
Task RollbackTransactionAsync();
}
Implementing UnitOfWork
Next, we implement the IUnitOfWork
interface in a class named UnitOfWork
.
public class UnitOfWork : IUnitOfWork
{
private readonly MyDbContext _context;
private bool _disposed = false;
public UnitOfWork(MyDbContext context)
{
_context = context;
}
public async Task<int> SaveChangesAsync()
{
return await _context.SaveChangesAsync();
}
public async Task BeginTransactionAsync()
{
await _context.Database.BeginTransactionAsync();
}
public async Task CommitTransactionAsync()
{
await _context.Database.CommitTransactionAsync();
}
public async Task RollbackTransactionAsync()
{
await _context.Database.RollbackTransactionAsync();
}
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
if (!_disposed && disposing)
{
if (_context != null)
{
_context.Dispose();
_context = null;
}
_disposed = true;
}
}
}
Applying UnitOfWork in Practice
With the UnitOfWork
implemented, let's see how it can be applied in a real-world scenario. Suppose we have a blogging platform where posts can be updated concurrently. Using UnitOfWork
, we can ensure that updates to a post are atomic and consistent.
public class BlogPostService
{
private readonly IUnitOfWork _unitOfWork;
private readonly ILogger<BlogPostService> _logger;
public BlogPostService(IUnitOfWork unitOfWork, ILogger<BlogPostService> logger)
{
_unitOfWork = unitOfWork;
_logger = logger;
}
public async Task UpdateBlogPostAsync(int postId, string title, string content)
{
try
{
await _unitOfWork.BeginTransactionAsync();
try
{
var post = await _unitOfWork.Posts.FindAsync(postId);
if (post != null)
{
post.Title = title;
post.Content = content;
await _unitOfWork.SaveChangesAsync();
}
await _unitOfWork.CommitTransactionAsync();
_logger.LogInformation("Blog post updated successfully.");
}
catch (Exception ex)
{
_logger.LogError(ex, "Failed to update blog post.");
await _unitOfWork.RollbackTransactionAsync();
throw;
}
}
finally
{
_unitOfWork.Dispose();
}
}
}
Conclusion
The UnitOfWork
pattern, combined with EF Core's transaction management features, provides a robust solution for handling concurrency in web applications. By encapsulating transaction logic within a dedicated class, we can ensure data integrity and consistency, even in highly concurrent environments. This approach not only improves the reliability of our applications but also enhances developer productivity by abstracting away low-level transaction management details.
Subscribe to my newsletter
Read articles from Bezyl Mophat Otieno directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
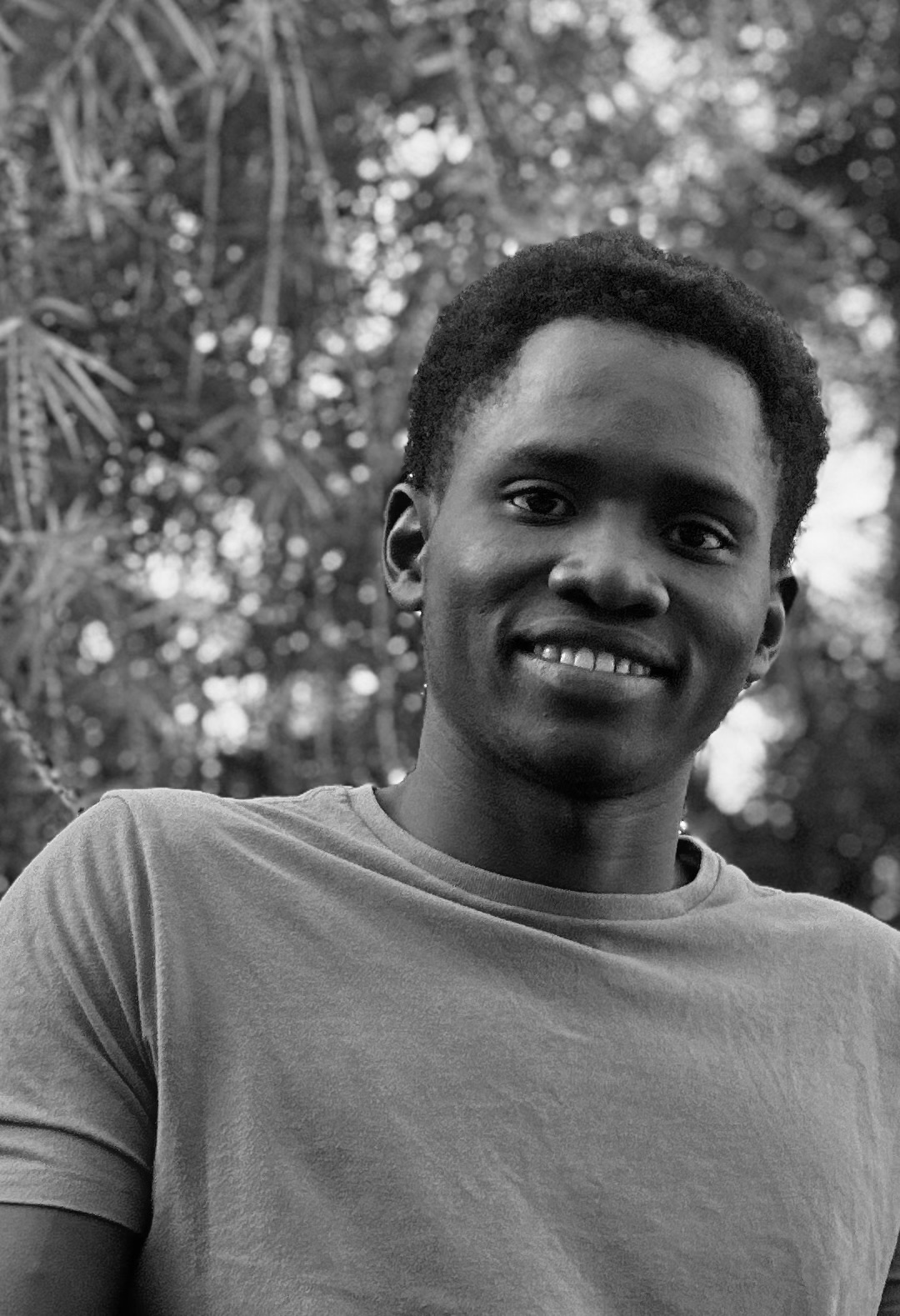
Bezyl Mophat Otieno
Bezyl Mophat Otieno
Tech-Savvy | Software Engineer | Enthusiastic Learner | Creative Problem Solver with a Dash of Humor | AI & Cutting-Edge Tech Enthusiast | Passionate Technical Article Writer.