Mastering Matrix Multiplication in C with Dynamic Memory Allocation
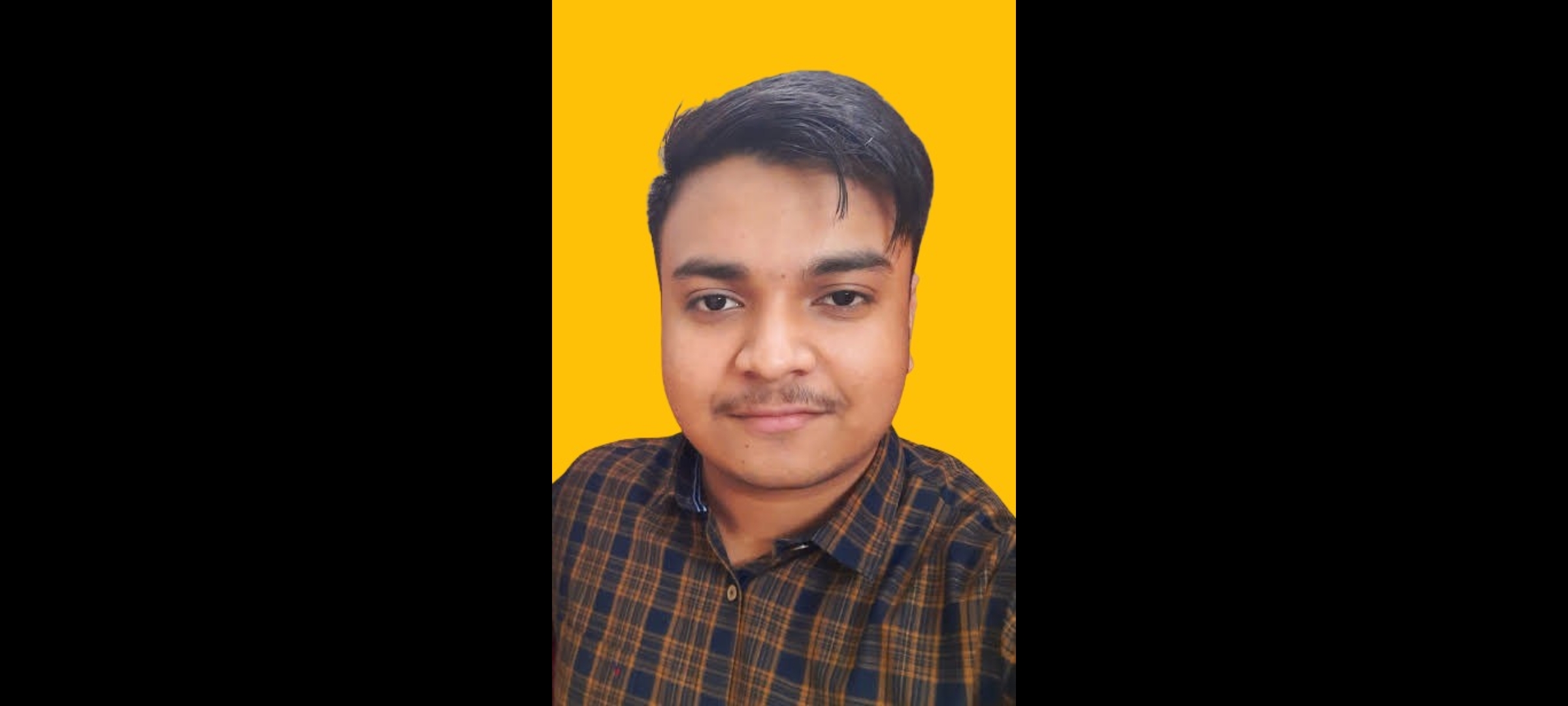
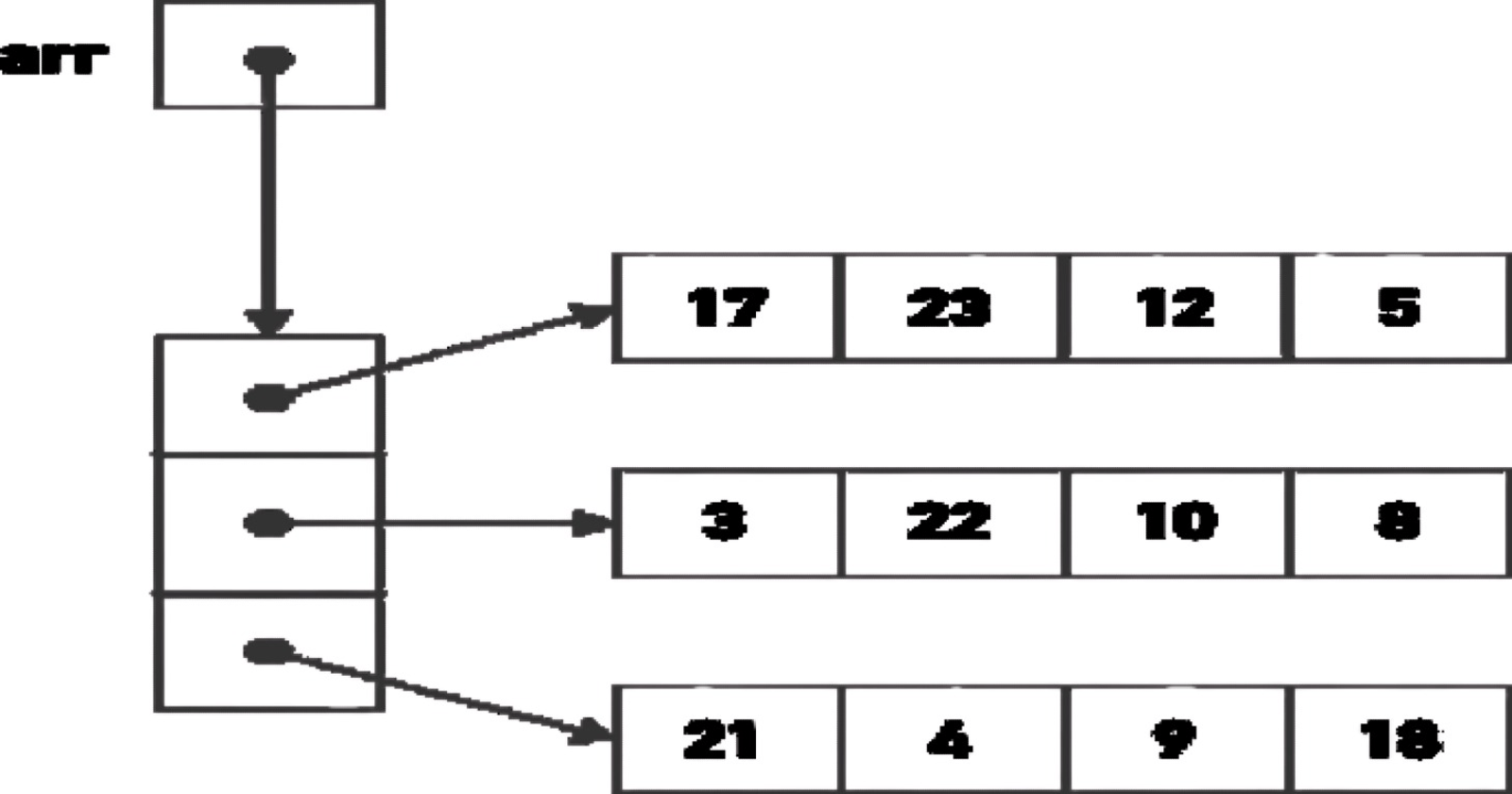
Matrix multiplication is like a mathematical dance where two matrices join forces to create a brand-new matrix. But how do we manage this in C, especially when we don’t know the matrix sizes ahead of time? That’s where dynamic memory allocation comes in! Let’s dive into how you can multiply matrices in C using dynamic memory allocation, and make it fun and easy.
Why Dynamic Memory Allocation?
In C, dynamic memory allocation lets you create and manage matrices whose sizes aren’t known until runtime. This means you can handle any matrix size, which is super handy for a lot of applications.
Matrix Multiplication 101
Before we get our hands dirty, let’s recall the basics of matrix multiplication. Given two matrices:
Matrix A of size
r1 x c1
Matrix B of size
r2 x c2
For these two to dance together, the number of columns in Matrix A (c1
) must match the number of rows in Matrix B (r2
). The result will be a new matrix with dimensions r1 x c2
.
How to Do It in C
Here’s a step-by-step guide, and we’ll use dynamic memory allocation to handle matrices flexibly.
1. Setup the Stage
First, we need to ask for the matrix dimensions and check if they’re compatible.
printf("Enter the order of first matrix (rows columns): \n");
scanf("%d%d", &r1, &c1);
printf("Enter the order of second matrix (rows columns): \n");
scanf("%d%d", &r2, &c2);
if (c1 != r2) {
printf("Matrix multiplication is not possible!\n");
return 1;
}
2. Allocate Memory
Now we allocate memory for our matrices using malloc
.
[malloc
allocates a block of memory of a specified size and returns a pointer to it, allowing dynamic management of memory during program execution.]
int **mat1 = (int **)malloc(r1 * sizeof(int *));
for (int i = 0; i < r1; i++) {
mat1[i] = (int *)malloc(c1 * sizeof(int));
}
int **mat2 = (int **)malloc(r2 * sizeof(int *));
for (int i = 0; i < r2; i++) {
mat2[i] = (int *)malloc(c2 * sizeof(int));
}
int **result = (int **)malloc(r1 * sizeof(int *));
for (int i = 0; i < r1; i++) {
result[i] = (int *)malloc(c2 * sizeof(int));
}
3. Fill the Matrices
Next, let’s fill our matrices with some data.
printf("Enter the elements of the first matrix:\n");
for (int i = 0; i < r1; i++) {
for (int j = 0; j < c1; j++) {
scanf("%d", &mat1[i][j]);
}
}
printf("Enter the elements of the second matrix:\n");
for (int i = 0; i < r2; i++) {
for (int j = 0; j < c2; j++) {
scanf("%d", &mat2[i][j]);
}
}
4. Perform the Multiplication
Here's where the magic happens. We compute the result matrix.
for (int i = 0; i < r1; i++) {
for (int j = 0; j < c2; j++) {
result[i][j] = 0;
for (int k = 0; k < c1; k++) {
result[i][j] += mat1[i][k] * mat2[k][j];
}
}
}
5. Show the Results
Finally, let’s display our result matrix.
printf("The resultant matrix is:\n");
for (int i = 0; i < r1; i++) {
for (int j = 0; j < c2; j++) {
printf("%d ", result[i][j]);
}
printf("\n");
}
6. Clean Up
Don’t forget to free up the memory we used.
for (int i = 0; i < r1; i++) {
free(mat1[i]);
free(result[i]);
}
for (int i = 0; i < r2; i++) {
free(mat2[i]);
}
free(mat1);
free(mat2);
free(result);
Wrapping Up
And that’s it! You’ve just learned how to multiply matrices using dynamic memory allocation in C. It’s like magic, but with code! Now you can handle matrices of any size and keep your programs flexible and efficient. For more fun with matrices and dynamic memory, keep exploring C programming and enjoy the journey!
"Stay curious, keep learning, and let your code make a difference!"
~ Sushil Kumar Mishra
Subscribe to my newsletter
Read articles from Sushil Kumar Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
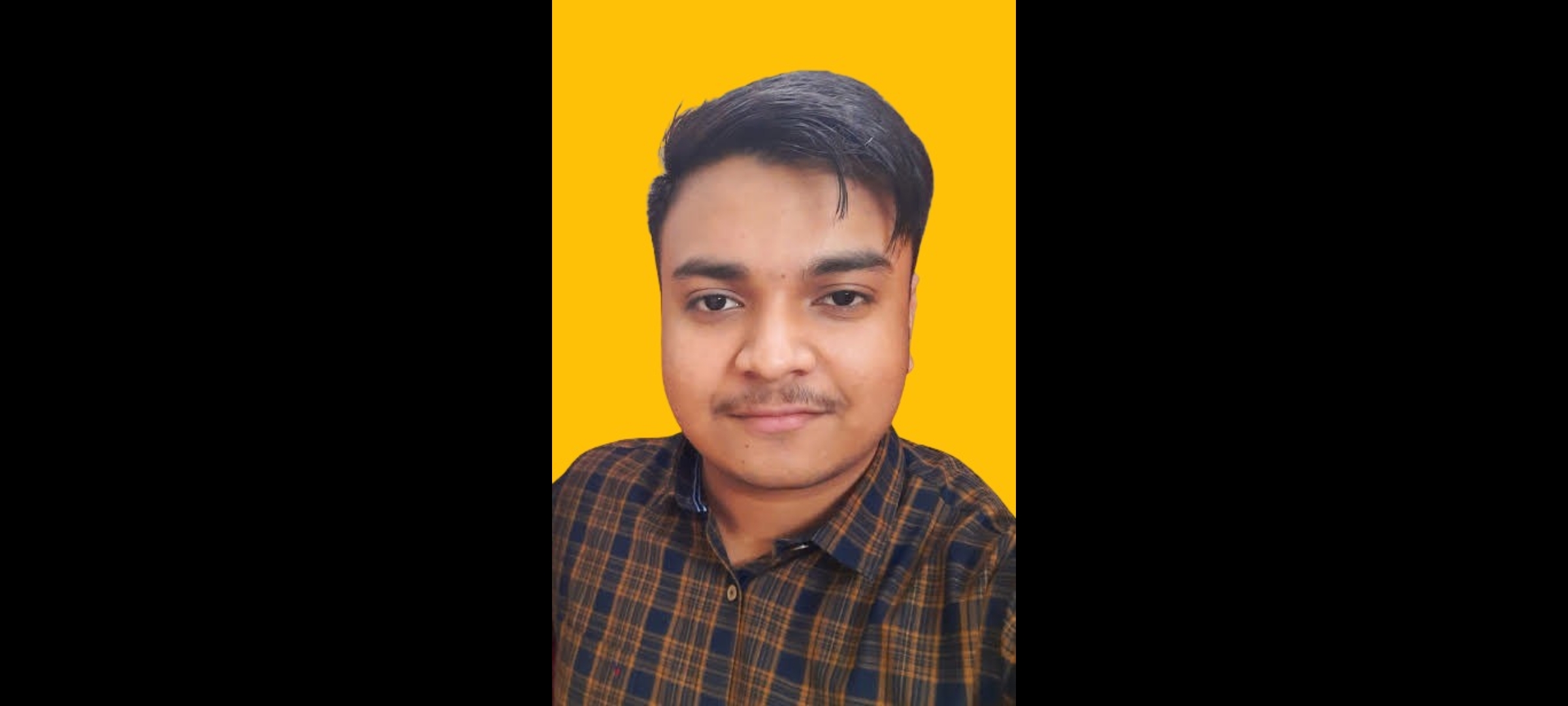