From Concept to Code: Solving pattern problems using JavaScript
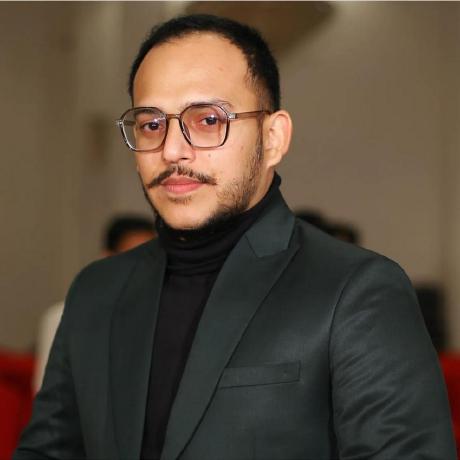
Table of contents
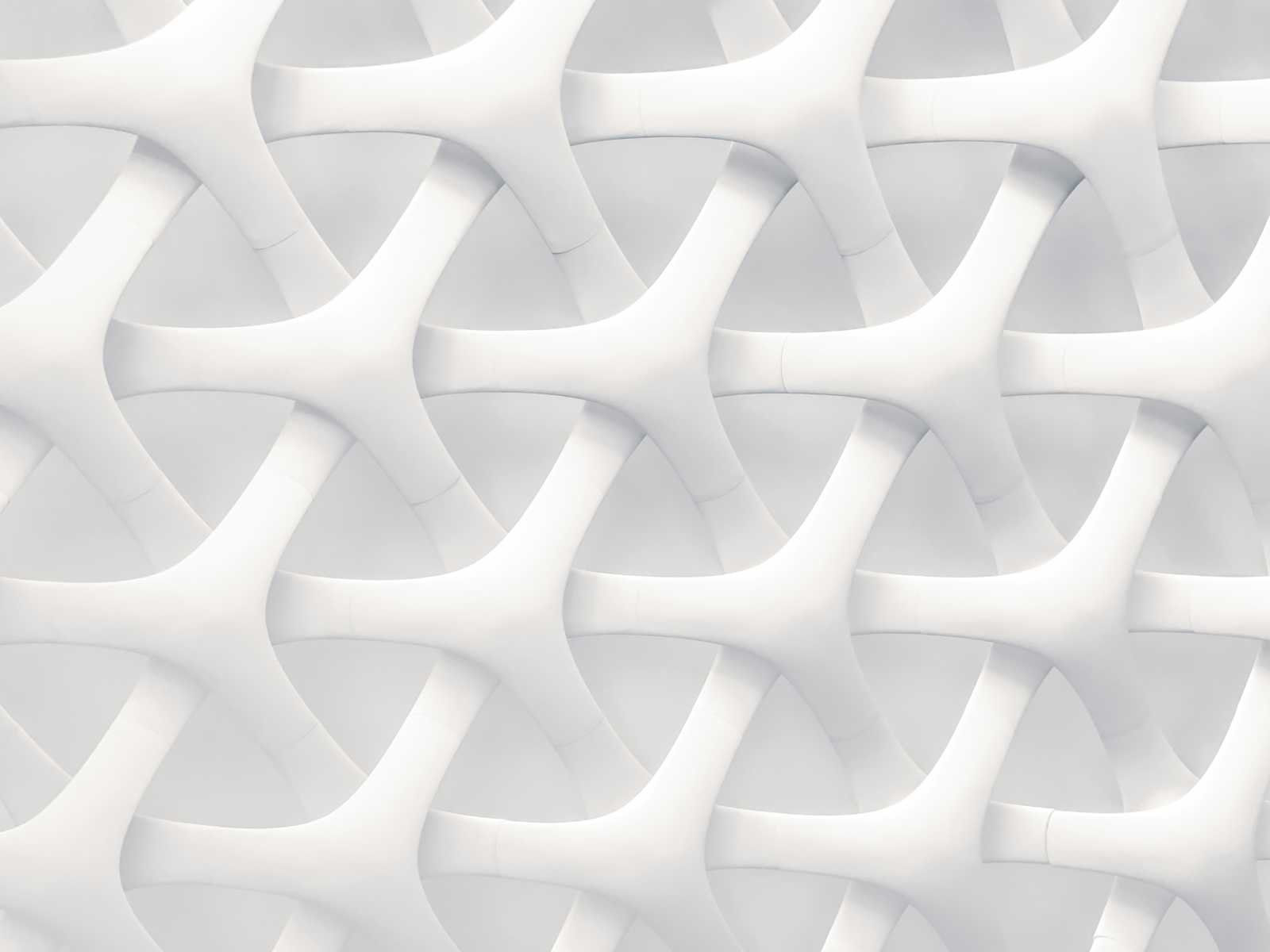
A pattern is a recurring sequence or repetition. Practicing pattern is highly recommended to master the looping, nested looping in JavaScript.
Mastering patterns is the most common used approach while learning the basics of JavaScript. At least, a good practice of patterns in JavaScript helps us quickly figure out the program's execution flow and improve logical thinking. While patterns might not be directly applied in everyday JavaScript development, they offer numerous practical use cases and insights.
Patterns in JavaScript
Let's go through various examples of number, star, and character patterns and understand their approach in depth.
When a series of numbers or characters are arranged to create a pattern or a particular shape, like pyramids, triangles etc.. it forms a pattern.
Triangle Pattern-1
Let's try printing our first triangle pattern.
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
We can see that we need to run/iterrate a loop for row number of times which will also print the number in that row according to the row number.
Example: If the loop is in its first iteration 1 then it shall print numeric 1 in row 1, if it is in 2nd iteration then it shall print numeric 1 and 2 in the 2nd row and so on till the last row is reached.
let rows = 5, num = "";
for (let count = 1; count <= rows; count++) {
num += count + " ";
console.log(num);
}
In the above code, initially we are defining the number of rows we want to have in pattern & variable num is initially empty because when the loop starts and iterates, the next numeric value will be added to it according to the row number.
When the loop starts first it starts with row 1 and at the same time it also adds the numeric value 1 in the same row.
Loop then iterates everytime incrementing the the row number and the numeric value to the previous numeric value(in line 3) untill reaches the condition i,e. row\=5.
Finally, when the loop terminates we are printing the pattern using
console.log
.
Triangle Pattern-2
Let's try printing our next triangle pattern.
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
In this pattern, we need to run/iterrate an external loop for row number of times & an internal loop which will also print the numeric value row times in the row, according to the row number.
Example: If the loop is in its first iteration 1 then it shall print numeric 1, row times, i,e. 1 time in the row 1, if it is in 2nd iteration then it shall print numeric 2, row times, i,e. 2 times in the row 2 and so on till the last row is reached.
let row =5, pattern="";
for (let count = 1; count <= row; count++) {
for (let num = 1; num <=count; num++) {
pattern += count + " ";
}
pattern +='\n';
}
console.log(pattern);
In the above code, initially we are defining the number of rows we want to have in pattern & variable pattern is initially empty because when the loop starts and iterates, the numeric value will be added to the row according to the row number.
When the loop starts first it starts with row= 1 and at the same time it also adds the numeric value 1, row times i,e 1 time in the same row.
Loop then iterates everytime incrementing the the row number and the numeric will now change according to row number, row times.(in line 4) untill reaches the condition i,e. row\=5.
In the line 5 of code, every single value of pattern that is generated after the internal loop will now move to the next line because of new line tag
"\n"
.Finally, when the loop terminates we are printing the pattern using
console.log
.
Triangle Pattern-3
Let's try printing our next triangle pattern which is also called Floyd's Triangle.
1
2 3
4 5 6
7 8 9 10
In this pattern type, we need to run/iterrate an external loop for row number of times & an internal loop which will also print the numeric value in the row, according to the row number. In this loop, numeric value will be defined initially as 1 and at every iteration, the numeric will be incremented and be added to the numeric value of the previous iteration.
Example: If the loop is in its first iteration 1 then it shall print numeric 1 as defined initially in the row 1. In 2nd iteration, it shall increment numeric value from 1 to 2, and add it the to the numeric value of the last iteration i,e,. 1(last iteration numeric value) + 2(current incremented numeric value)=3 and prints the current incremented numerice value(2) and added sum of last interation numeric value & current incremented numeric value(3) in row 2 & so on.
let row = 4, number = 1, pattern = "";
for (let count = 1; count <= row; count++) {
for (let num = 1; num <= count; num++) {
pattern = pattern + number + " ";
number++;
}
console.log(pattern);
}
In the above code, initially we are defining the number of rows we want to have in pattern, variable pattern is initially empty & the number is initially defined as 1 because when the loop starts and iterates, the defined number value will be added to the row according to the row number.
When the outer loop starts, first it starts with row= 1 and at the same time it also the inner loop that adds the defined number value 1 and stores it in variable pattern(in line 4), in row 1 and atlast increments the number value from 1 to 2 by
number++
(in line 5).Both the loops iterates everytime incrementing the the row number and then previously stored pattern value will be added to current number value i,e 2 it will be printed in row 2. Atlast, number is again incremented in the inner loop by
number++
(in line 5). This operation repeates untill reaches the condition of outer loop i,e. row\=4.Finally, when the loop terminates we are printing the pattern using
console.log
.
The examples above showcase various pattern problems in JavaScript. There are many more patterns like "*"-pyramid, Hollow Triangle etc.. We'll be adding more patterns logic over time, so keep an eye on the blog for new updates.
Subscribe to my newsletter
Read articles from Rizwan Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
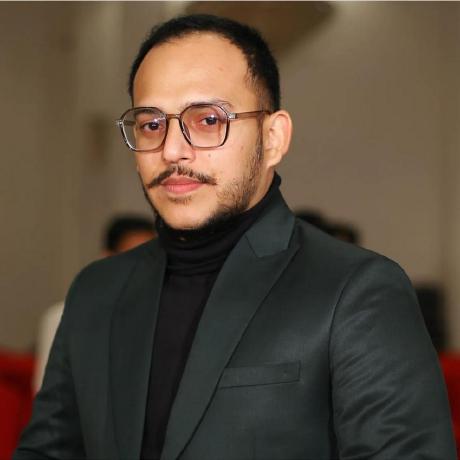