π The Magic of React Fiber: A Deep Dive into React's Reconciliation Algorithm
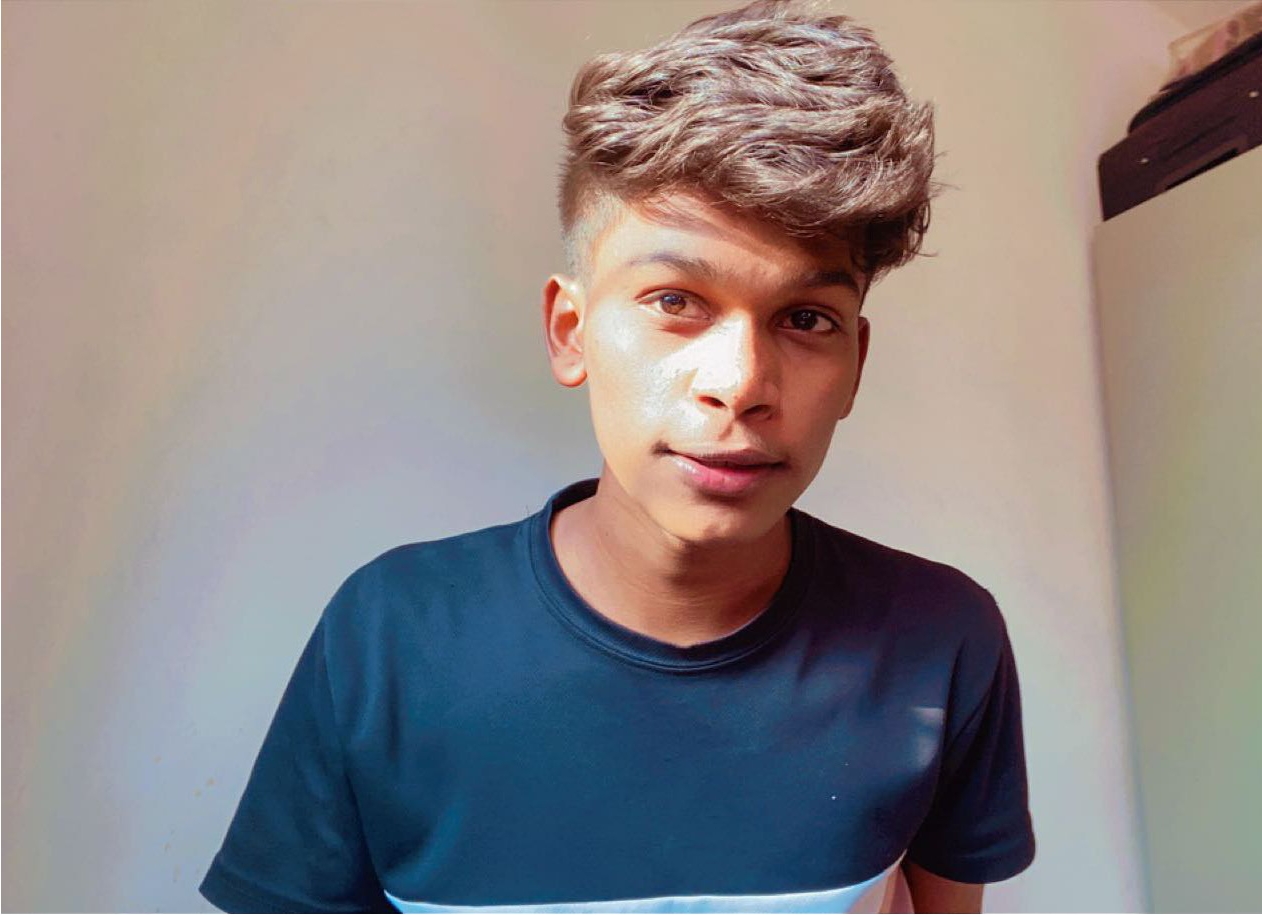
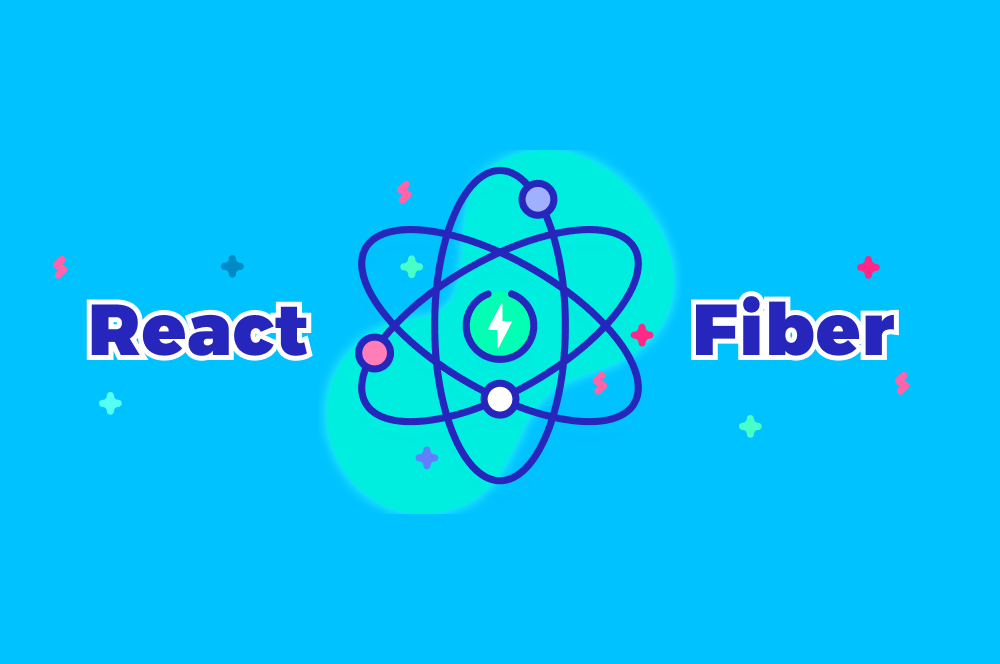
π Introduction
React has been a cornerstone of modern web development, empowering developers to build dynamic, responsive user interfaces. One of the most significant updates to React is the introduction of React Fiber, a complete rewrite of the React reconciliation algorithm. This update allows for more efficient rendering, better user experience, and enhanced performance. In this blog, weβll explore what React Fiber is, how it works, and why itβs a game-changer for React developers. ππ
π What is React Fiber?
React Fiber is the reimplementation of the core algorithm in React that is responsible for diffing and reconciliation. It was introduced in React 16 to address limitations in the original React architecture, known as the Stack Reconciler. React Fiber improves the efficiency of rendering, especially in complex applications, by breaking down the rendering process into incremental units of work. This allows React to pause, resume, and prioritize tasks, leading to smoother and more responsive UIs. πβ‘
π The Evolution of React: From Stack to Fiber
The Stack Reconciler
Before Fiber, React used the Stack Reconciler, which was a synchronous and depth-first approach to rendering. This approach had several limitations:
Blocking: Rendering could block the main thread, leading to unresponsive UIs during complex updates.
Lack of Prioritization: All updates were treated equally, without prioritization for more important tasks.
Poor Performance: Inefficient handling of large trees and complex state changes.
Enter React Fiber
React Fiber was designed to overcome these limitations by introducing an asynchronous and incremental rendering process. Key improvements include:
Interruptible Rendering: Allows React to pause and resume rendering tasks, ensuring the UI remains responsive.
Prioritized Updates: Enables React to prioritize updates, focusing on more critical tasks first.
Concurrency: Supports concurrent rendering, making it possible to handle multiple tasks simultaneously. ππ§΅
π‘ How React Fiber Works
React Fiber works by breaking down the rendering process into small units of work called "fibers." Each fiber represents a part of the UI, such as a component or a subtree. The main concepts behind Fiber include:
Incremental Rendering: Fiber breaks down rendering into small chunks, allowing React to work on tasks in pieces and pause if needed.
Prioritization: Fiber assigns priority levels to updates, ensuring that critical updates are processed first.
Reconciliation: Fiber diffing algorithm efficiently updates the UI by comparing the current and previous state of components.
Concurrency: Fiber allows multiple updates to be processed concurrently, improving performance and responsiveness. ππ
π Key Benefits of React Fiber
Enhanced Performance: Incremental rendering and prioritization lead to more efficient updates and better performance, especially in complex applications. πβ‘
Improved User Experience: By keeping the UI responsive and smoothly handling updates, Fiber enhances the overall user experience. ππ±οΈ
Concurrent Rendering: Support for concurrent rendering allows multiple tasks to be processed simultaneously, improving efficiency. π§΅π
Error Handling: Improved error boundaries make it easier to handle and recover from errors in the UI. β οΈπ§
Better Animations: Fiber's ability to pause and resume work makes it easier to create smooth animations and transitions. π¨π
π οΈ Practical Examples
Example 1: Prioritizing Updates with Fiber
import React, { useState, useEffect } from 'react';
function App() {
const [count, setCount] = useState(0);
const [text, setText] = useState('');
// High-priority update
useEffect(() => {
setCount((prevCount) => prevCount + 1);
}, []);
// Low-priority update
useEffect(() => {
setTimeout(() => {
setText('Hello, Fiber!');
}, 3000);
}, []);
return (
<div>
<div>Count: {count}</div>
<div>Text: {text}</div>
</div>
);
}
export default App;
In this example, React prioritizes the count update over the text update, ensuring that critical updates are processed first.
Example 2: Smooth Animations with Fiber
import React, { useState, useEffect } from 'react';
function AnimationComponent() {
const [position, setPosition] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setPosition((prev) => (prev + 1) % 100);
}, 16);
return () => clearInterval(interval);
}, []);
return <div style={{ transform: `translateX(${position}px)` }}>I move smoothly!</div>;
}
export default AnimationComponent;
React Fiber's ability to handle incremental rendering ensures that the animation remains smooth and responsive. π¨π
βοΈ Challenges and Considerations
π Benefits
Enhanced Performance: React Fiber brings significant performance improvements to complex applications. π
Improved User Experience: Users enjoy smoother and more responsive UIs. π
Concurrent Rendering: Multiple updates are handled concurrently, boosting efficiency. π§΅
β οΈ Challenges
Learning Curve: Developers need to understand the new rendering paradigms introduced by Fiber. π
Experimental Features: Some features of Fiber are still experimental and may change. β οΈ
Compatibility Issues: Ensure third-party libraries are compatible with Fiber. π§
π Conclusion
React Fiber is a transformative update that brings significant improvements to the React ecosystem. By introducing incremental and prioritized rendering, Fiber enhances performance, user experience, and efficiency in handling complex applications. While there are challenges to consider, the benefits make it a compelling choice for modern web development. ππ
Subscribe to my newsletter for more insights on React, advanced techniques, and the latest trends in web development! π¬π
Did you find this article helpful? Share it with your network or leave a comment below! ππ¬
Subscribe to my newsletter
Read articles from Aditya Dhaygude directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
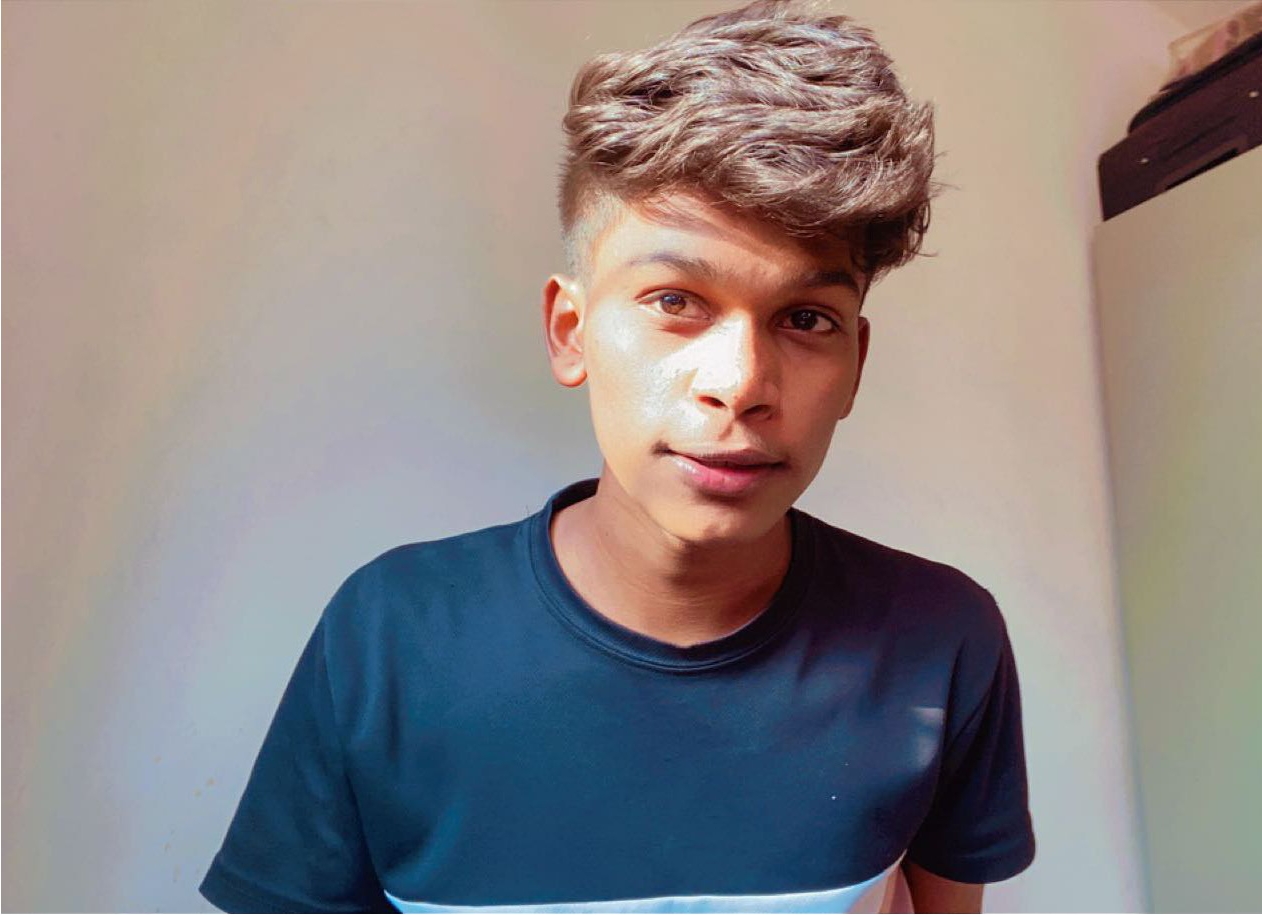
Aditya Dhaygude
Aditya Dhaygude
i Build Stuff