Understanding Model Diagnostics in Machine Learning: A Comprehensive Guide
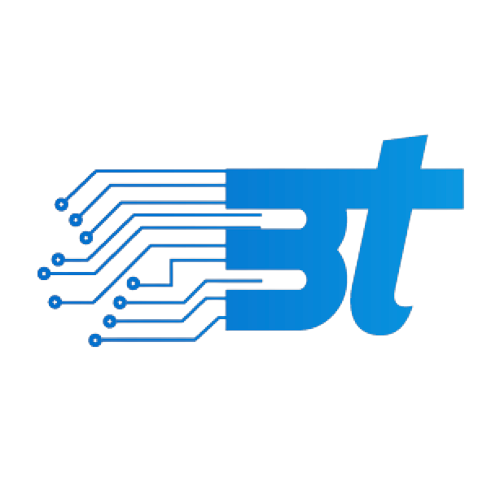
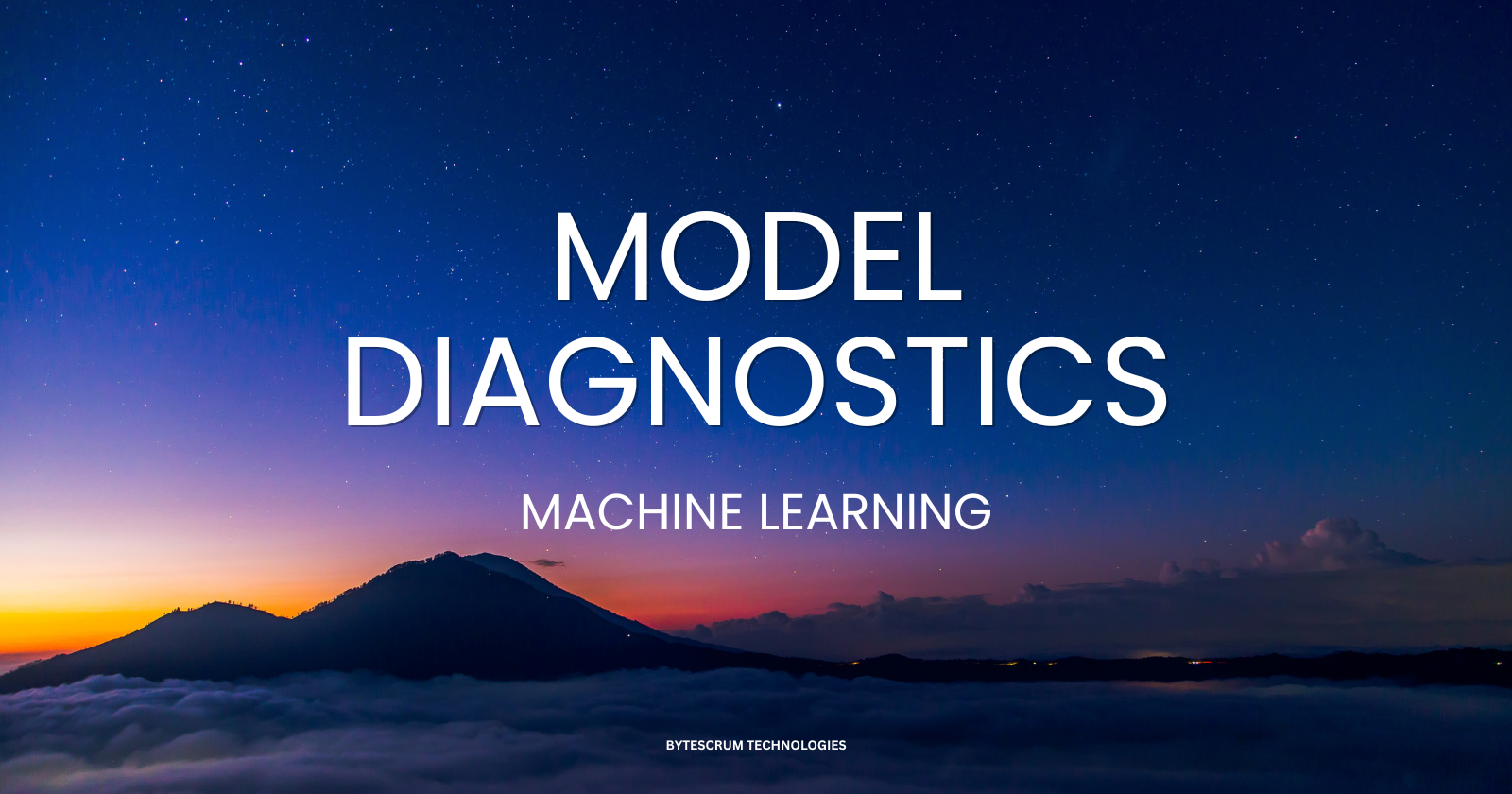
In machine learning, building a model is just one part of the process. Ensuring that the model performs well and diagnosing potential issues are equally important. Model diagnostics help in understanding how well the model is performing and identifying areas for improvement. This blog will delve into the various aspects of model diagnostics, providing detailed examples to illustrate key concepts.
Importance of Model Diagnostics
Model diagnostics are crucial for several reasons:
Performance Assessment: Determine how well the model is performing on training and unseen data.
Overfitting and Underfitting: Identify if the model is too complex (overfitting) or too simple (underfitting).
Model Comparison: Compare different models to select the best one.
Feature Importance: Understand the contribution of different features to the model's predictions.
Error Analysis: Analyze errors to improve model accuracy.
Key Metrics for Model Diagnostics
Classification Metrics
- Accuracy: The ratio of correctly predicted observations to the total observations. Suitable for balanced datasets.
$$Accuracy= Total Number of Predictions/ Number of Correct Predictions$$
Precision, Recall, and F1-Score: Useful for imbalanced datasets.
Precision: The ratio of correctly predicted positive observations to the total predicted positives.
$$Precision= True Positives + False Positives /True Positives$$
Recall (Sensitivity): The ratio of correctly predicted positive observations to all observations in the actual class.
$$Recall= True Positives + False Negatives /True Positives$$
F1-Score: The harmonic mean of Precision and Recall.
$$F1-Score=2× Precision + Recall /Precision×Recall$$
ROC Curve and AUC (Area Under the Curve): Evaluate the trade-off between True Positive Rate and False Positive Rate.
- AUC: A single scalar value to compare models. Higher AUC indicates a better model.
Confusion Matrix: A table used to describe the performance of a classification model.
- True Positives (TP), True Negatives (TN), False Positives (FP), False Negatives (FN).
Example:
from sklearn.metrics import confusion_matrix
import seaborn as sns
cm = confusion_matrix(y_test, y_pred)
sns.heatmap(cm, annot=True, fmt='d', cmap='Blues')
plt.xlabel('Predicted')
plt.ylabel('Actual')
plt.title('Confusion Matrix')
plt.show()
Regression Metrics
- Mean Absolute Error (MAE): The average of absolute differences between predicted and actual values.
$$MAE= n 1 i=1 ∑ n ∣y i − y ^ i$$
- Mean Squared Error (MSE): The average of squared differences between predicted and actual values.
$$MSE= n 1 i=1 ∑ n (y i − y ^ i ) 2$$
- Root Mean Squared Error (RMSE): The square root of MSE, providing an error metric in the same units as the target variable.
$$RMSE= √MSE$$
- R-squared (R²): The proportion of variance in the dependent variable that is predictable from the independent variables.
$$R 2 =1− ∑ i=1 n (y i − y ˉ ) 2 ∑ i=1 n (y i − y ^ i ) 2$$
Example:
from sklearn.metrics import mean_absolute_error, mean_squared_error, r2_score
mae = mean_absolute_error(y_test, y_pred)
mse = mean_squared_error(y_test, y_pred)
rmse = np.sqrt(mse)
r2 = r2_score(y_test, y_pred)
print(f'MAE: {mae}')
print(f'MSE: {mse}')
print(f'RMSE: {rmse}')
print(f'R-squared: {r2}')
Diagnostic Techniques
1. Residual Analysis
For regression models, residual analysis helps in diagnosing issues. Residuals are the differences between observed and predicted values.
- Plotting Residuals: Residuals vs. Fitted values plot can help identify non-linearity, heteroscedasticity, and outliers.
Example:
import matplotlib.pyplot as plt
import seaborn as sns
import numpy as np
# Assuming y_test and y_pred are the true and predicted values
residuals = y_test - y_pred
plt.figure(figsize=(10, 6))
sns.scatterplot(x=y_pred, y=residuals)
plt.axhline(y=0, color='r', linestyle='--')
plt.xlabel('Predicted Values')
plt.ylabel('Residuals')
plt.title('Residuals vs Predicted Values')
plt.show()
Learning Curves
Learning curves plot the training and validation error as a function of the training set size. They help in diagnosing overfitting and underfitting.
Overfitting: High training accuracy but low validation accuracy.
Underfitting: Both training and validation accuracy are low.
Example:
from sklearn.model_selection import learning_curve
import numpy as np
train_sizes, train_scores, test_scores = learning_curve(model, X, y, cv=5, scoring='accuracy', n_jobs=-1, train_sizes=np.linspace(0.1, 1.0, 10))
train_mean = np.mean(train_scores, axis=1)
train_std = np.std(train_scores, axis=1)
test_mean = np.mean(test_scores, axis=1)
test_std = np.std(test_scores, axis=1)
plt.figure(figsize=(10, 6))
plt.plot(train_sizes, train_mean, 'o-', color='blue', label='Training score')
plt.plot(train_sizes, test_mean, 'o-', color='green', label='Cross-validation score')
plt.fill_between(train_sizes, train_mean - train_std, train_mean + train_std, alpha=0.1, color='blue')
plt.fill_between(train_sizes, test_mean - test_std, test_mean + test_std, alpha=0.1, color='green')
plt.xlabel('Training Set Size')
plt.ylabel('Accuracy')
plt.title('Learning Curves')
plt.legend(loc='best')
plt.show()
3. Cross-Validation
Cross-validation helps in understanding model performance on unseen data. It involves splitting the data into k subsets and training the model k times, each time using a different subset as the validation set.
- K-Fold Cross-Validation: Commonly used technique.
Example:
from sklearn.model_selection import cross_val_score
scores = cross_val_score(model, X, y, cv=5, scoring='accuracy')
print("Cross-validation scores:", scores)
print("Mean accuracy:", scores.mean())
Feature Importance
Understanding which features contribute the most to the model's predictions can help in refining the model.
Tree-based models: Provide built-in feature importance scores.
Permutation Importance: Assess the impact of shuffling a feature on model performance.
Example (Tree-based models):
importances = model.feature_importances_
indices = np.argsort(importances)[::-1]
plt.figure(figsize=(10, 6))
plt.title("Feature Importances")
plt.bar(range(X.shape[1]), importances[indices], align='center')
plt.xticks(range(X.shape[1]), [X.columns[i] for i in indices], rotation=90)
plt.show()
Partial Dependence Plots (PDPs)
Partial Dependence Plots show the relationship between a feature and the predicted outcome, marginalizing over the values of other features. They help in understanding the effect of a feature on the model's predictions.
Example:
from sklearn.inspection import plot_partial_dependence
features = [0, 1] # Indices of features to plot
plot_partial_dependence(model, X, features)
plt.show()
Error Analysis
Error analysis involves examining the types and sources of errors made by the model. This can involve:
Misclassified Instances: Reviewing instances where the model made incorrect predictions.
Error Distribution: Understanding the distribution of errors to identify patterns.
Case Study: Conducting a detailed analysis of specific errors to understand their cause.
Example:
misclassified = X_test[y_test != y_pred]
print("Misclassified instances:")
print(misclassified)
Hyperparameter Tuning
Hyperparameter tuning involves optimizing the hyperparameters of the model to improve its performance. Techniques include Grid Search and Random Search.
Grid Search: Exhaustive search over specified parameter values.
Random Search: Randomly sampling parameter values.
Example (Grid Search):
from sklearn.model_selection import GridSearchCV
param_grid = {'n_estimators': [100, 200], 'max_depth': [10, 20]}
grid_search = GridSearchCV(model, param_grid, cv=5, scoring='accuracy')
grid_search.fit(X, y)
print("Best parameters:", grid_search.best_params_)
print("Best cross-validation score:", grid_search.best_score_)
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
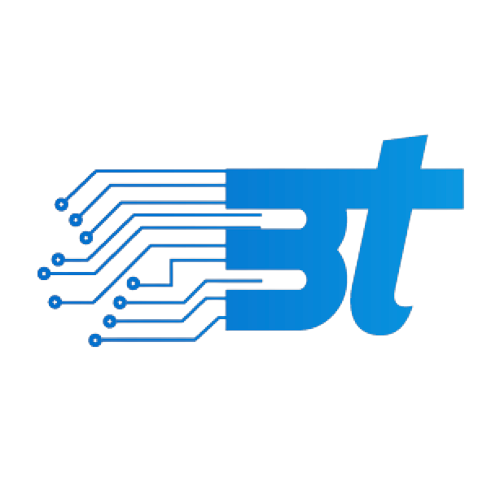
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.