Boost Software Quality Using Unit Tests and Automation
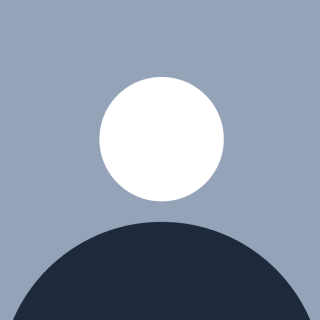
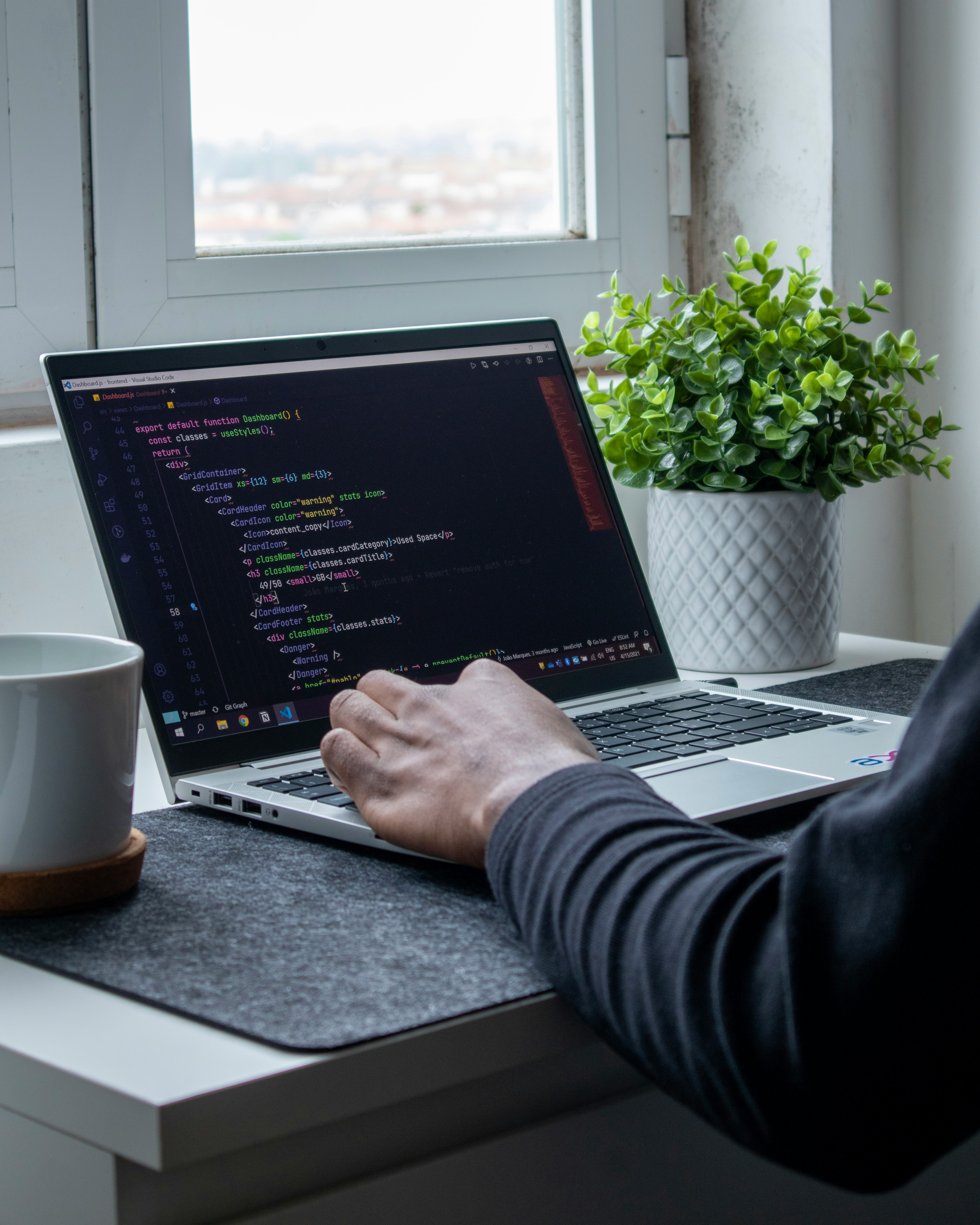
Importance of unit tests in software development
Unit tests are the foundation of a robust and reliable software development process. They play a crucial role in ensuring the quality and stability of your codebase. By writing unit tests, developers can validate the functionality of individual components or units of their software, ensuring that they work as expected and catch any potential issues early on in the development cycle.
This proactive approach to testing allows developers to identify and fix bugs quickly, reducing the time and cost associated with resolving issues later in the development process or, even worse, in production. Unit tests also serve as a safety net, enabling developers to make changes to the codebase with confidence, knowing that they can quickly verify that existing functionality remains intact.
Moreover, unit tests promote better code design and modularity. By breaking down the software into smaller, testable units, developers are encouraged to write more modular, loosely coupled, and maintainable code. This, in turn, leads to improved code readability, flexibility, and scalability, making it easier to adapt to changing requirements and collaborate effectively within a development team.
Benefits of automation in software testing
Automating the testing process is a game-changer in the world of software development. By leveraging automation, teams can significantly improve the efficiency, consistency, and coverage of their testing efforts, ultimately leading to higher-quality software.
One of the primary benefits of test automation is the ability to run tests repeatedly and consistently, without the risk of human error or fatigue. Automated tests can be executed quickly and reliably, ensuring that every change in the codebase is thoroughly validated, even in complex scenarios or edge cases that might be difficult to test manually. This not only saves time but also enhances the overall confidence in the software's quality.
Additionally, automated tests can be run at any time, including during the development process, as part of a continuous integration (CI) pipeline, or during scheduled regression testing. This allows development teams to catch issues early before they escalate and become more costly to fix. Automation also enables the execution of repetitive, time-consuming, or tedious test cases, freeing up valuable human resources to focus on more complex, exploratory testing or new feature development.
Understanding unit tests and their role in software quality
Unit tests are a fundamental component of the software testing hierarchy, focusing on the smallest testable parts of an application, known as units. These units can be individual functions, methods, or classes, and their purpose is to verify that each component works as expected in isolation, before integrating them into the larger system.
By writing unit tests, developers can ensure that their code is behaving correctly, catching bugs early in the development process and reducing the likelihood of introducing regressions. Unit tests also serve as a form of documentation, clearly communicating the expected behavior of each component and helping new team members understand the codebase more quickly.
The role of unit tests in software quality is multifaceted. They provide a safety net for developers, allowing them to refactor or modify code with confidence, knowing that existing functionality is still working as intended. Unit tests also facilitate the implementation of test-driven development (TDD) and behavior-driven development (BDD) practices, which promote a more disciplined and collaborative approach to software development. By writing tests first, developers can better understand the requirements and design their code accordingly, leading to more maintainable and extensible software.
Strategies for effective unit testing
Developing an effective unit testing strategy is crucial for ensuring the long-term success and quality of your software project. One key aspect of this strategy is to focus on writing tests that are independent, isolated, and fast-running. This means that each unit test should be self-contained, not relying on external dependencies or the state of the system, and be able to execute quickly, providing immediate feedback to the developer.
Another important consideration is the coverage of your unit tests. Aim to achieve a high level of code coverage, ensuring that your tests exercise a significant portion of your codebase. This not only helps catch more bugs but also serves as a valuable indicator of the overall health and robustness of your software. However, it's important to strike a balance between coverage and the complexity of your tests, as overly complex or brittle tests can become a maintenance burden and slow down the development process.
Equally important is the organization and structure of your unit tests. Group related tests together, using descriptive and meaningful names that communicate the intent of each test case. This makes it easier to understand the purpose of each test, facilitates debugging, and ensures that the test suite remains organized and maintainable as the codebase grows. Additionally, consider implementing a testing framework that provides a clear and consistent structure for your unit tests, such as the popular xUnit or behavior-driven development (BDD) frameworks.
Best practices for writing unit tests
Writing effective unit tests requires a certain level of discipline and attention to detail. One of the fundamental best practices is to follow the FIRST principle: tests should be Fast, Independent, Repeatable, Self-Validating, and Timely.
Ensuring that your tests are fast-running is crucial, as slow-executing tests can slow down the development process and discourage developers from running them regularly. Independence means that each test should be self-contained, not relying on the state of other tests or external dependencies. Repeatability guarantees that the tests will produce the same results every time they are executed, and self-validation ensures that the tests can determine whether a test case has passed or failed without manual intervention. Finally, timely tests are those that are written at the appropriate stage of the development lifecycle, catching issues as early as possible.
Another best practice is to write tests that are focused and specific, testing a single behavior or functionality at a time. This makes it easier to identify and fix any issues that arise, as the failure of a test case will point to the root cause. Additionally, aim to write tests that are easy to understand and maintain, using descriptive names and providing clear comments that explain the purpose and expected behavior of each test case.
Choosing the right tools for unit testing and automation
Selecting the appropriate tools for unit testing and test automation is crucial for the success of your software development efforts. The landscape of testing tools is vast, and the choice will depend on factors such as the programming language, the complexity of your application, and the specific needs of your development team.
For unit testing, popular frameworks like JUnit (Java), pytest (Python), and Jest (JavaScript) provide a solid foundation for writing and running unit tests. These frameworks offer features such as assertion libraries, test organization, and reporting, making it easier to write and maintain your unit test suite. Additionally, consider tools that integrate seamlessly with your development environment, such as Visual Studio Code's built-in unit testing support or IntelliJ IDEA's extensive testing capabilities.
When it comes to test automation, there are numerous options to choose from, each with its strengths and weaknesses. Selenium is a widely used web automation framework that allows you to write and execute automated tests for web-based applications. For mobile app testing, frameworks like Appium and Espresso provide the necessary tools and APIs. For API testing, tools like Postman, SoapUI, and RestAssured offer comprehensive solutions. Additionally, consider cloud-based testing platforms like Sauce Labs or BrowserStack, which can help you scale your testing efforts and ensure cross-browser and cross-device compatibility.
Implementing test automation frameworks
Implementing a robust test automation framework is crucial for streamlining your software testing processes and ensuring the long-term maintainability of your test suite. A well-designed automation framework should provide a structured and scalable approach to writing, executing, and managing your automated tests.
One key aspect of a successful test automation framework is the adoption of a modular and reusable design. This involves breaking down your tests into smaller, independent components that can be easily combined and reused across different test suites or even different projects. This modular approach not only promotes code reuse but also makes it easier to maintain and update your tests as the application evolves.
Another important consideration is the integration of your test automation framework with your continuous integration (CI) and continuous deployment (CD) pipelines. By seamlessly integrating your automated tests into your CI/CD workflow, you can ensure that every code change is thoroughly validated, and any regressions are caught early in the development process. This helps to reduce the risk of deploying buggy software to production and ensures that your application remains stable and reliable.
Integrating unit tests and automation into the software development lifecycle
Integrating unit tests and test automation into the software development lifecycle is a critical step in ensuring the long-term quality and sustainability of your software project. This integration should be a collaborative effort, involving developers, quality assurance (QA) engineers, and other stakeholders, to create a cohesive and efficient testing strategy.
One effective approach is to adopt a shift-left testing methodology, where testing activities are incorporated into the early stages of the development process. This means that unit tests and automated tests are written and executed alongside the development of new features, rather than being an afterthought or a separate phase. By shifting testing to the left, you can catch issues earlier, reducing the time and cost associated with resolving them later in the development cycle.
Another key aspect of this integration is the implementation of continuous integration (CI) and continuous deployment (CD) pipelines. These pipelines automate the build, test, and deployment processes, ensuring that every code change is thoroughly validated before being pushed to production. By integrating your unit tests and automated tests into these pipelines, you can ensure that the quality of your software is consistently maintained and that any regressions are quickly identified and addressed.
Conclusion
In the fast-paced world of software development, delivering high-quality applications is no longer a luxury – it's a necessity. By harnessing the power of unit tests and test automation, you can elevate the quality of your software, ensuring that your codebase is stable, reliable, and able to adapt to changing requirements.
Throughout this comprehensive guide, we have explored the importance of unit tests, the benefits of test automation, and the best practices for implementing effective testing strategies. From writing well-designed unit tests to integrating them into your software development lifecycle, we have provided you with the knowledge and tools you need to take your software quality to new heights.
Remember, the journey to software excellence is an ongoing process, and it's crucial to continuously evaluate and refine your testing practices as your codebase and team evolve. By embracing a culture of quality and continuously striving to improve your testing efforts, you can ensure that your software remains robust, scalable, and able to deliver an exceptional user experience.
So, what are you waiting for? Start leveraging the power of unit tests and automation today, and watch as your software development process transforms into a well-oiled machine, delivering high-quality applications that delight your users and keep your business ahead of the competition.
Subscribe to my newsletter
Read articles from Himanshu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by