What are the possible ways to create objects in JavaScript
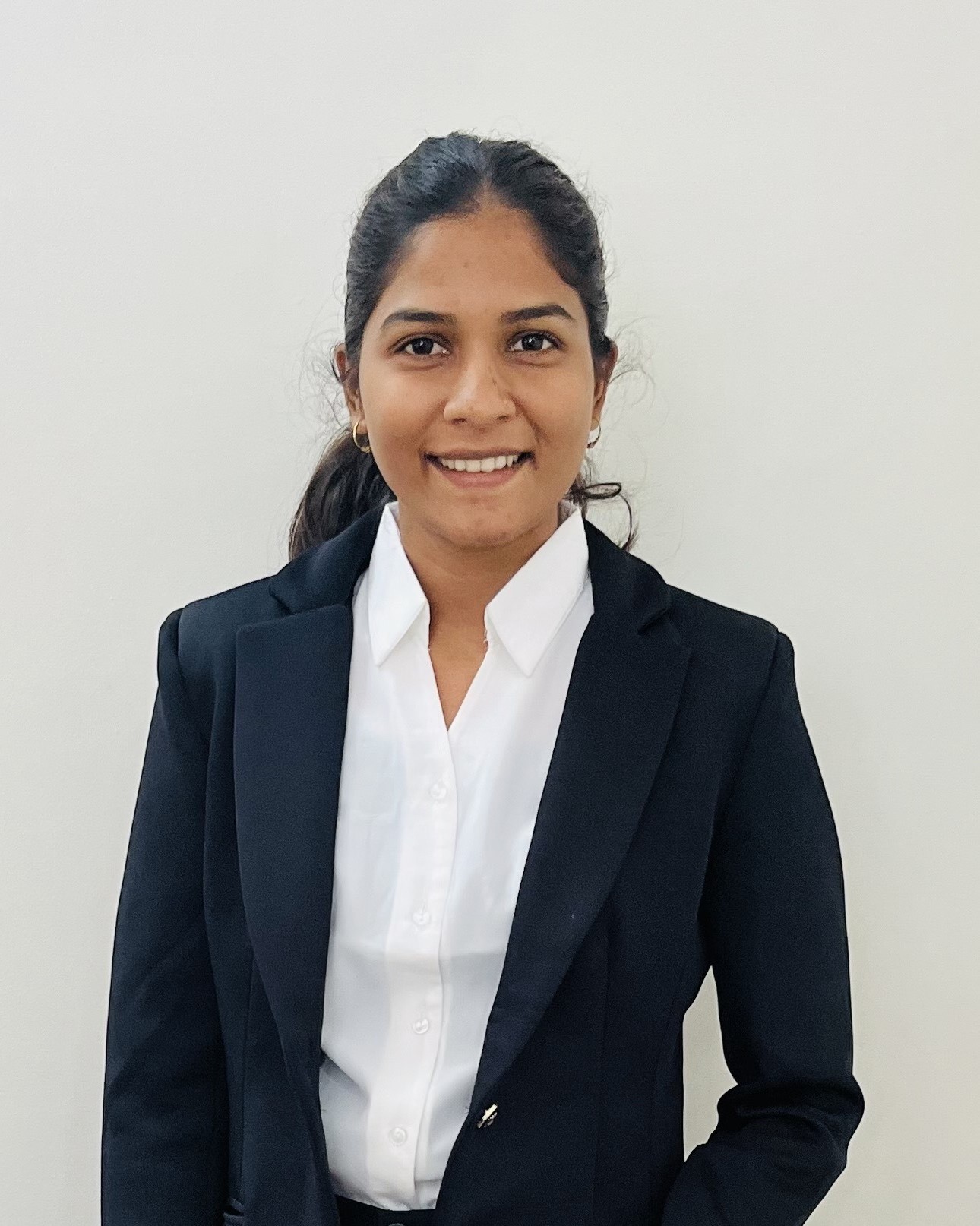
2 min read
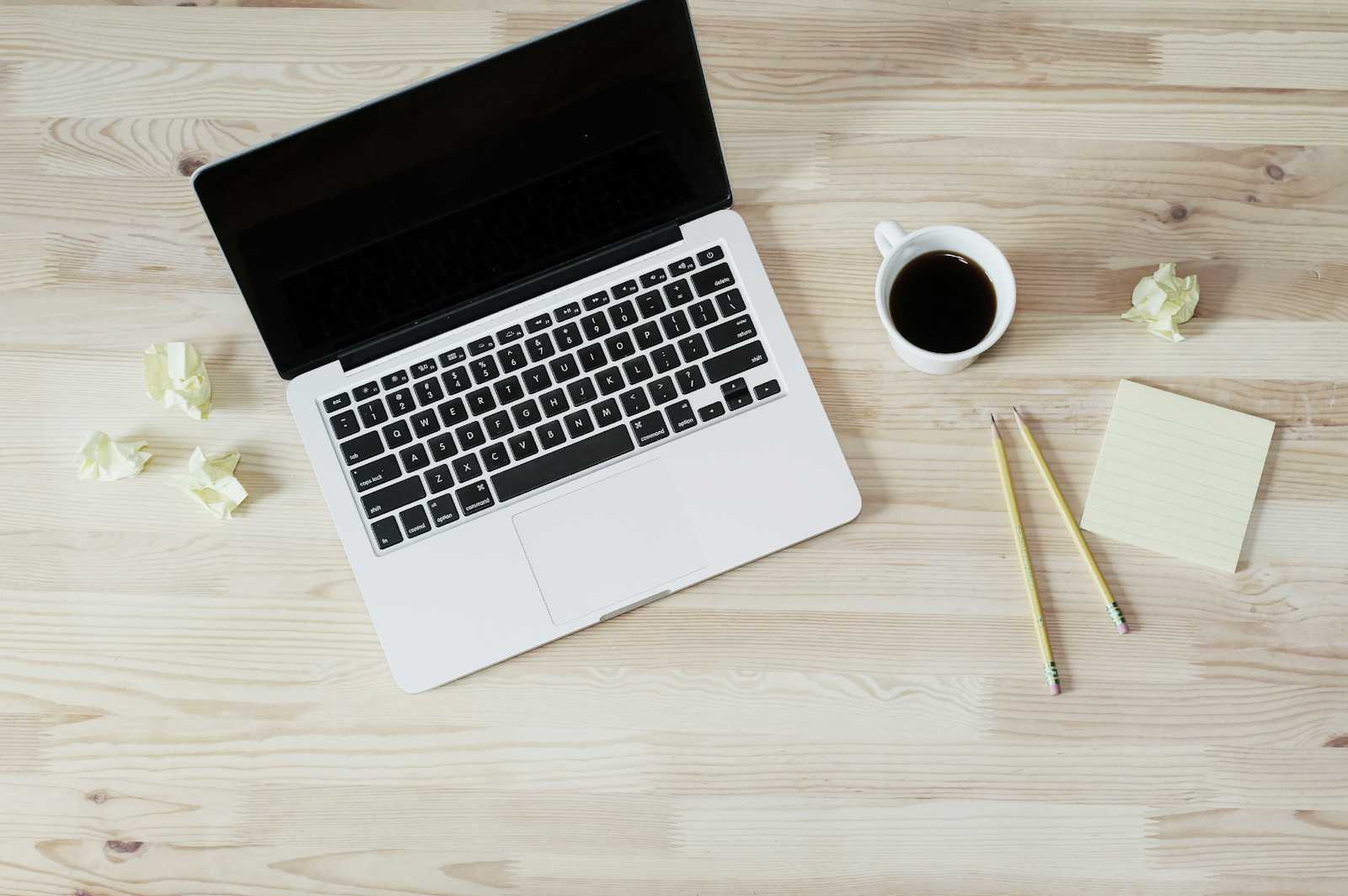
In JavaScript, you can create objects using several different methods, each with its own use case and syntax. Here are some common ways:
1. Object Literals
- The simplest and most common way to create an object is using an object literal.
const person = {
name: 'Priyanka',
age: 28
};
2. Using the new Object()
Constructor
- This method is less common but still valid.
const person = new Object();
person.name = 'Priyanka';
person.age = 28;
3. Using a Constructor Function
- You can define a constructor function and create objects using the
new
keyword.
function Person(name, age) {
this.name = name;
this.age = age;
}
const person1 = new Person('Priyanka', 28);
4. Using Object.create()
- This method allows you to create a new object with a specified prototype object and properties.
const personPrototype = {
greet: function() {
console.log('Hello, my name is ' + this.name);
}
};
const person = Object.create(personPrototype);
person.name = 'Priyanka';
person.greet();
5. Using ES6 Classes
- Classes in JavaScript provide a more straightforward and clear syntax for creating constructor functions and handling inheritance.
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
console.log(`Hello, my name is ${this.name}`);
}
}
const person = new Person('Priyanka', 28);
person.greet();
6. Using Factory Functions
- A factory function returns an object without the need to use
new
.
function createPerson(name, age) {
return {
name,
age,
greet() {
console.log(`Hello, my name is ${this.name}`);
}
};
}
const person = createPerson('Priyanka', 28);
person.greet();
7. Using Singleton Pattern
- This pattern is used when you want only one instance of an object.
const person = (function() {
const instance = {
name: 'Priyanka',
age: 28
};
return instance;
})();
8. Using the Object.fromEntries()
Method
- This method creates an object from an array of key-value pairs.
const entries = [['name', 'Priyanka'], ['age', 28]];
const person = Object.fromEntries(entries);
These different methods offer flexibility depending on the situation and the type of object creation you need.
11
Subscribe to my newsletter
Read articles from priyanka chaudhari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
2Articles1Week#LCOiwritecodecrazybloggerJavaScriptAngularinterviewTrainWithShubhamWeb Developmentcrazy bloggerJSXlambdaLaraveljsObjects
Written by
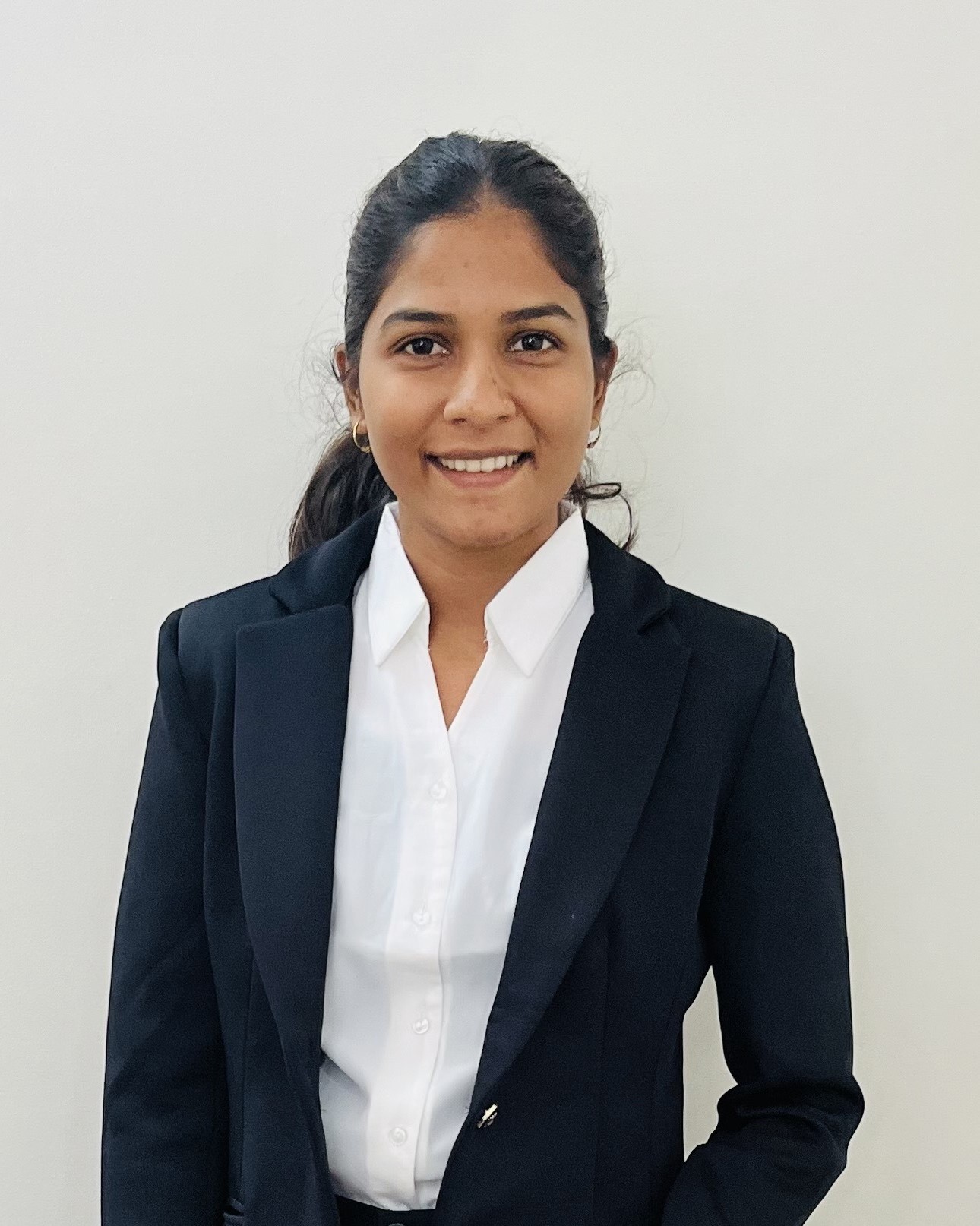
priyanka chaudhari
priyanka chaudhari
i am a full stack web developer and i write code....