Leetcode Problem 9 Solution: Checking Palindrome Numbers

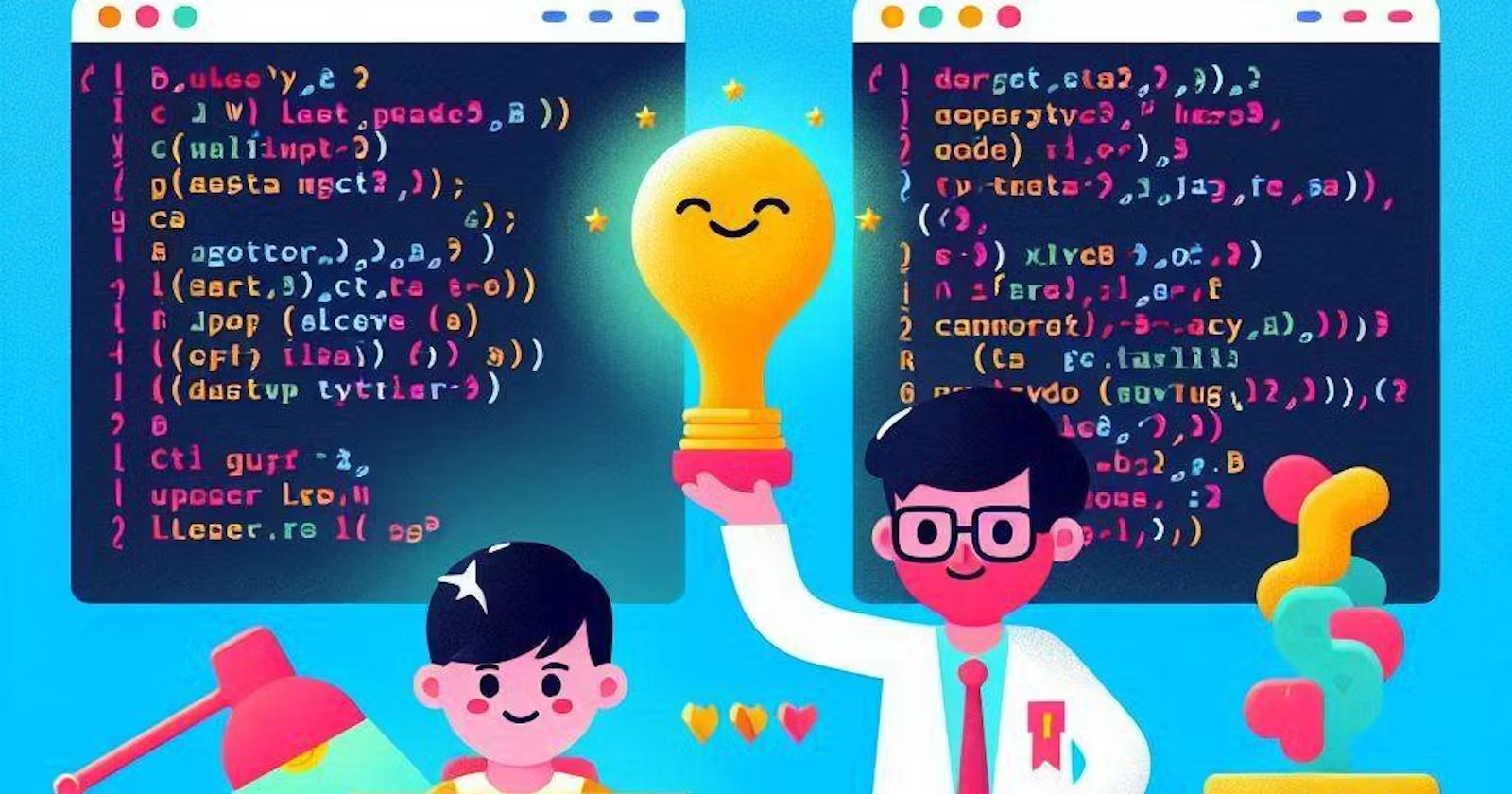
Given an integer x
, return true
if x
is a palindrome and false
otherwise.
Example 1:
Input: x = 121
Output: true
Explanation: 121 reads as 121 from left to right and from right to left.
Example 2:
Input: x = -121
Output: false
Explanation: From left to right, it reads -121. From right to left,
it becomes 121-. Therefore it is not a palindrome.
Example 3:
Input: x = 10
Output: false
Explanation: Reads 01 from right to left. Therefore it is not a palindrome.
Constraints:
-2<sup>31</sup> <= x <= 2<sup>31</sup> - 1
Follow up:Could you solve it without converting the integer to a string?
Approach 1: Converting Number into String
function isPalindrome(x: number): boolean {
//Step 1: Convert number into the string
let strX = x.toString();
let startIndex = 0;
let endIndex = strX.length - 1;
//Step 2: Handle less than Zero value
if (x < 0) {
return false;
}
//Step 3: Reverse string and check if it is palidrom
while (endIndex > startIndex) {
if (strX[startIndex] != strX[endIndex]) {
return false
}
endIndex--;
startIndex++;
}
return true;
};
Explanation:
The isPalindrome
function performs the following:
Converts the integer to a string.
Checks if the number is negative (returning
false
for negatives).Compares characters from the start and end of the string, moving towards the center.
Returns
true
if all characters matched (indicating a palindrome), otherwisefalse
.
The time complexity of the function is O(n), where n is the number of digits in the integer. The space complexity of the function is O(n), where n is the number of digits in the integer.
Approach 2: Without converting number into String
function isPalindrome(x: number): boolean {
// Step 1: Check for negative numbers
if (x < 0) {
return false;
}
// Step 2: Handle zero explicitly
if (x === 0) {
return true;
}
// Step 3: Reverse the number
let original = x;
let reversed = 0;
while (original > 0) {
const digit = original % 10;
reversed = reversed * 10 + digit;
original = Math.floor(original / 10);
}
// Step 4: Check if the reversed number is equal to the original number
return x === reversed;
}
Explanation:
Negative Numbers: Return
false
for negative numbers as they cannot be palindromes.Zero: Return
true
for zero, which is a palindrome.Reversing the Number: Use a loop to extract digits from the original number and build the reversed number.
Comparison: Finally, compare the reversed number with the original number to determine if it's a palindrome.
This solution has a time complexity of O(log10n) due to the number of digits in the integer and a space complexity of O(1) since it only uses a few extra variables.
This article provides two approaches to determine if an integer x is a palindrome. The first approach converts the integer to a string and checks the reversed string for equality, while the second approach reverses the integer directly without string conversion. Both methods are analyzed for their time and space complexities.
Subscribe to my newsletter
Read articles from Vishad Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
