Understanding JavaScript's forEach, Filter, Map, and Reduce Methods
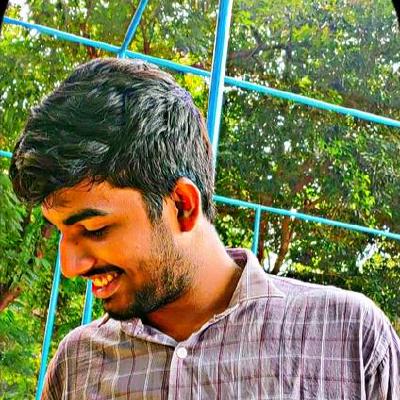
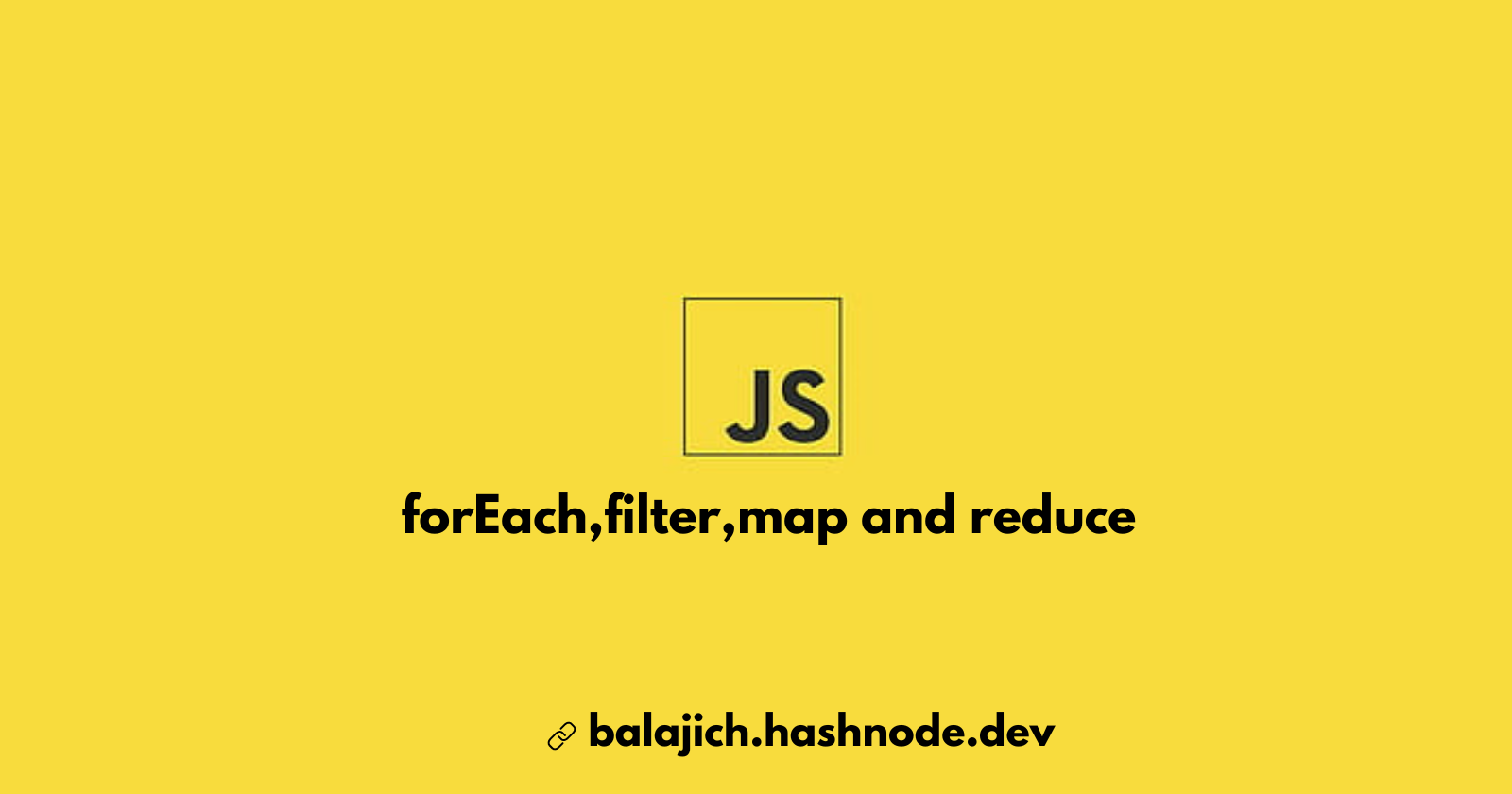
So basically any js developer who has ever worked in js must have come across arrays, objects, maps, etc. These are all popular data structures used in js to store and retrieve data, Actually these are the most frequently used data structures in js and as all these ds use key value kind of storing data the ecma script foundation has decided gradually to design purpose specific loops to address each and every data structure's usecase and the most popular loops that actually are used in js community are forEach, filter, map and reduce. So I will try to breakdown and explain every loop and it's use in this article.
forEach()
This is just a standard for loop provided as a method for an array. This method takes a callback function as a parameter and if you wonder what is a callback() then simply think of it as a function which calls itself until it reaches the end of the array. Now let's have a look at this forEach method.
let a=[1,5,9,2,4];
a.forEach((value)=>console.log(value+1));
Now if you try to execute the above code you will get an output of each element being printed out with an increment of 1, And if you are thinking how did that just work well have a look at the code block below.
let a=[1,5,9,2,4];
for(let i=0;i<a.length;i++){
console.log(a[i]+1);
}
Now did you get it, forEach is just a simplified version of standard for loop which takes a callback function as an input which provides you with 3 parameters namely value at that index in array, index and complete array, you can just put your logic inside the callback function and just relax javascript just takes care of all incrementing,iterators and stuff.
filter()
It is another loop kind of method but this is slightly different instead of letting you edit an existing array it let's you have a new array with a particular filter over the existing array. So simply forEach is an alternative to a forloop whereas filter is an advancement of forEach which let's you get a brand new array out of an existing one. For better understanding take a look at below code block.
let a=[1,5,9,2,4];
let b=a.filter((value)=>value>4);
console.log(b);
Now what we have just done is we have looped through filtered out all values greater than 4 and stored them in b and console logged b and to understand why this filter() exists just take a look at the standard approach without filter to achieve same result.
let a=[1,5,9,2,4],b=[];
for(let i=0;i<a.length;i++){
if(a[i]>4)
b.push(a[i]);
else
continue;
}
console.log(b);
map()
map is an advancement to forEach loop, map is just used to return an array but this array is obtained by performing some operations over another array, like creating a new array that wants an incremented version of an existing array. Take a look at code block below.
let a=[3,6,9,12,15,18];
let b=a.map((value)=>value+1);
console.log(b);
This has same parameters as filter and forEach and returns anew array similar to filter.
reduce()
This is slightly an odd one out of this loop family and how is that, well reduce takes a callback() similar to it's siblings and an initial value but the parameters it has are a bit different, it takes two values as parameters one is an accumulator and two is current value parameter, So basically I will tell how reduce works, first it takes an accumulator which is just a value that takes initially an input value which is a parameter to reduce() and then updates it's value with the value returned by the callback() in each iteration and current value is just the value in array at current iteration. Take a look at code block below.
let a=[1,3,5,7];
let b=a.reduce((acc,val)=>acc=acc+val,0);
console.log(b);
What the above reduce code does is it takes two params a callback() and an initial value 0 which is assigned to acc and after
first iteration acc will become 1 which is a result of 0+1 and in
second iteration acc becomes 4 which is result of 1+4 and in
third iteration acc becomes 9 which is result of 4+5 and in
fourth and final iteration acc becomes 16 which is result of 9+7.
This reduce() is used to do tasks like giving the total of all items in your basket in an ecommerce website, etc.
Conclusion
That's it for this article, subscribe to my news letter to explore may such interesting js, java and python articles.
Subscribe to my newsletter
Read articles from Balaji Chennupati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
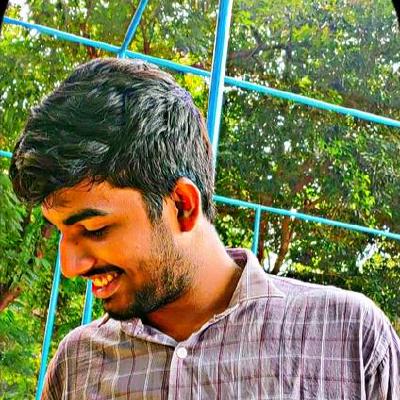
Balaji Chennupati
Balaji Chennupati
Passionate about web dev, java and devops, crafting solutions one line of code at a time.