Kickstarting My DevOps Adventure: Week 1 Recap
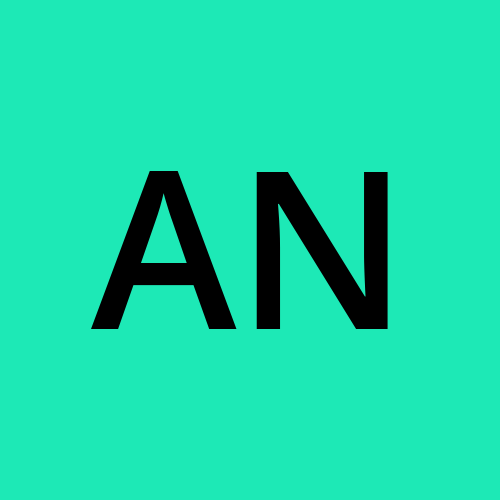
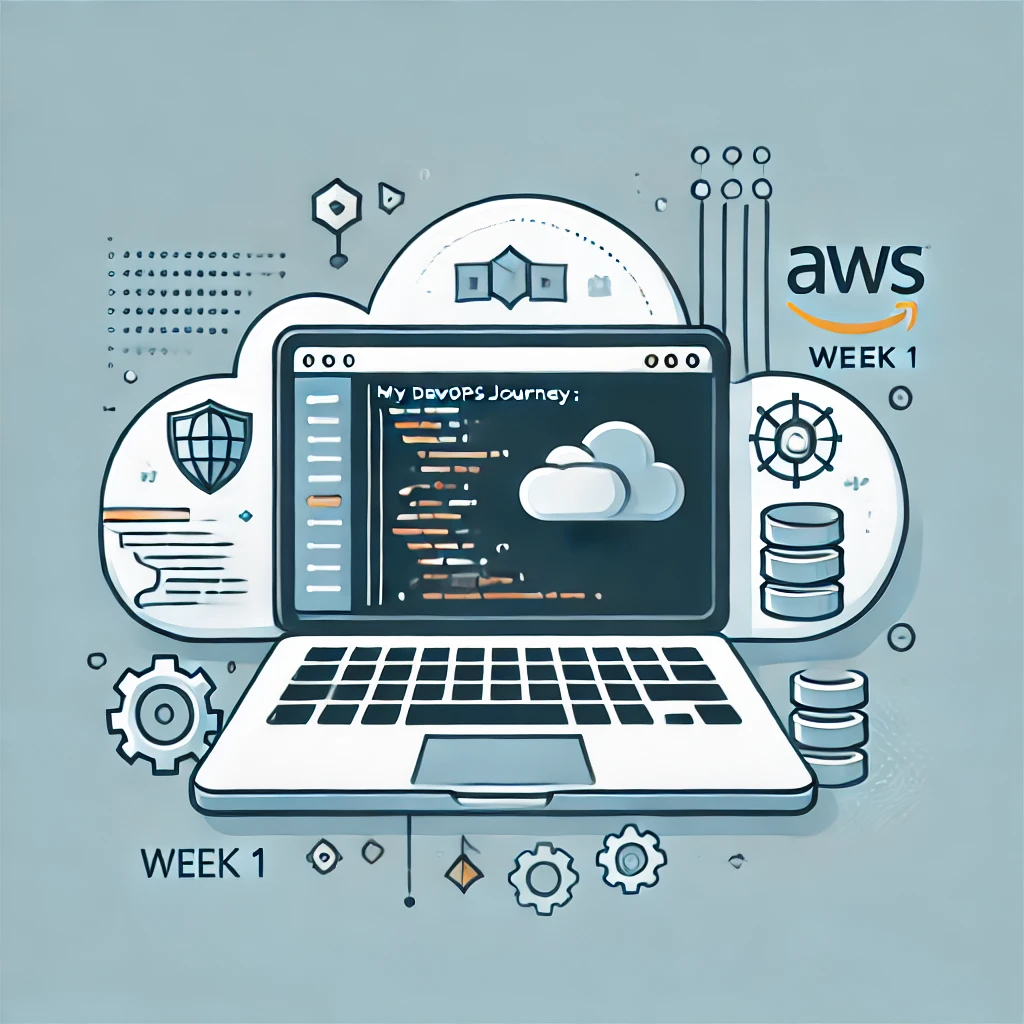
Introduction
Starting a journey in DevOps can be both exciting and daunting. With countless resources available, it’s essential to find a structured path that can guide you through the complexities of the field. For my journey, I’ve been following the "DevOps Zero to Hero" playlist on YouTube, created by Abhishek Veeramalla. This week, I completed the first 11 videos, covering essential topics and shell scripting basics. Here’s a summary of my learnings and the scripts I practiced.
Learning Shell Scripting
One of the key skills in DevOps is shell scripting. It allows for automation and efficient management of system operations. Here are some of the scripts I’ve learned and practiced during my first week.
AWS Resource Tracker Script
This script helps in reporting AWS resource usage, including S3 buckets, EC2 instances, Lambda functions, and IAM users.
#!/bin/bash
###############################
# Author: Adarsh
# Date: 5th Aug 2024
#
# Version: v1
#
# This script will report the AWS resource usage
###############################
# AWS S3
# AWS EC2
# AWS Lambda
# AWS IAM Users
# list S3 buckets
echo "Print list of S3 buckets"
aws s3 ls > ResourceTracker # Redirect the output to a file named ResourceTracker
# list EC2 instances
echo "Print EC2 instances"
aws ec2 describe-instances | jq '.Reservations[].Instances[].InstanceId' # Use jq to filter and print only instance IDs
# list lambda functions
echo "Print lambda functions"
aws lambda list-functions >> ResourceTracker # Append the output to the file ResourceTracker
# list IAM users
echo "Print all IAM users"
aws iam list-users
Simple For Loop
A basic script demonstrating the use of a for loop in bash.
#!/bin/bash
for i in {1..10}; do echo $i;
done
If-Else Conditional
This script checks if one variable is greater than another and prints the result.
#!/bin/bash
a=4
b=10
if [ $a > $b ]
then
echo "a is greater than b"
else
echo "b is greater than a"
fi
Node Health Check
A script that outputs the health status of a node, including disk space, memory, and CPU usage.
#!/bin/bash
##########################
# Author: Adarsh
# Date: 4th Aug 2024
#
# This script outputs the node health
#
# Version: V1
##########################
set -x # debug mode
set -o # exit the script when there is an error
set -o # exit when there is an error even when a pipe is present
# could've written in 1 line as set -exo pipeline
df -h # prints all details of disk space
free -g # prints all the free memory present
nproc # processors used or available
Key Takeaways
Shell Scripting Basics: I learned the basics of shell scripting, including loops, conditionals, and system health checks.
AWS CLI Usage: Gained practical experience with AWS CLI to manage and report AWS resources.
Debugging and Error Handling: Understood the importance of debugging and handling errors in scripts for robust automation.
Acknowledgements
I want to extend my heartfelt gratitude to Abhishek Veeramalla for creating the "DevOps Zero to Hero" playlist. His comprehensive and engaging tutorials have been instrumental in my learning process. Additionally, I’d like to thank savinder puri for his highly inspirational video on building a successful career in DevOps. His insights have not only motivated me but also introduced me to the Hashnode platform for blogging.
Next Steps
In the coming weeks, I plan to explore more advanced DevOps topics based on the "DevOps Zero to Hero" playlist, specifically covering:
AWS CodeCommit: Setting up Git repositories and managing version control for collaborative projects.
AWS CodePipeline: Creating CI/CD pipelines to automate the software release process.
AWS CodeBuild: Configuring and running build projects, defining build specifications, and integrating testing processes.
AWS CodeDeploy: Implementing deployment strategies, including Blue/Green deployments, to ensure zero-downtime and easy rollback options.
AWS CloudWatch: Monitoring AWS resources by setting up alarms, notifications, and collecting metrics to gain insights into the health and performance of the infrastructure.
Stay tuned for more updates as I continue my journey from zero to hero in DevOps!
Connect with Me
Follow my journey and connect with me on LinkedIn for more updates on my DevOps learning path. Feel free to share your thoughts, suggestions, and experiences.
Resources
Subscribe to my newsletter
Read articles from Adarsh N directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
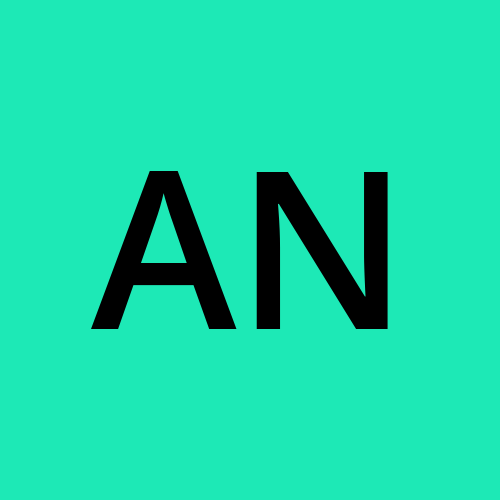
Adarsh N
Adarsh N
DevOps Enthusiast | Continuous Learner | Sharing My Journey Passionate about all things DevOps. Documenting my journey with tips and insights on automation, CI/CD, and cloud infrastructure.