π Simplify Your Code with TypeScriptβs Nullish Coalescing Operator
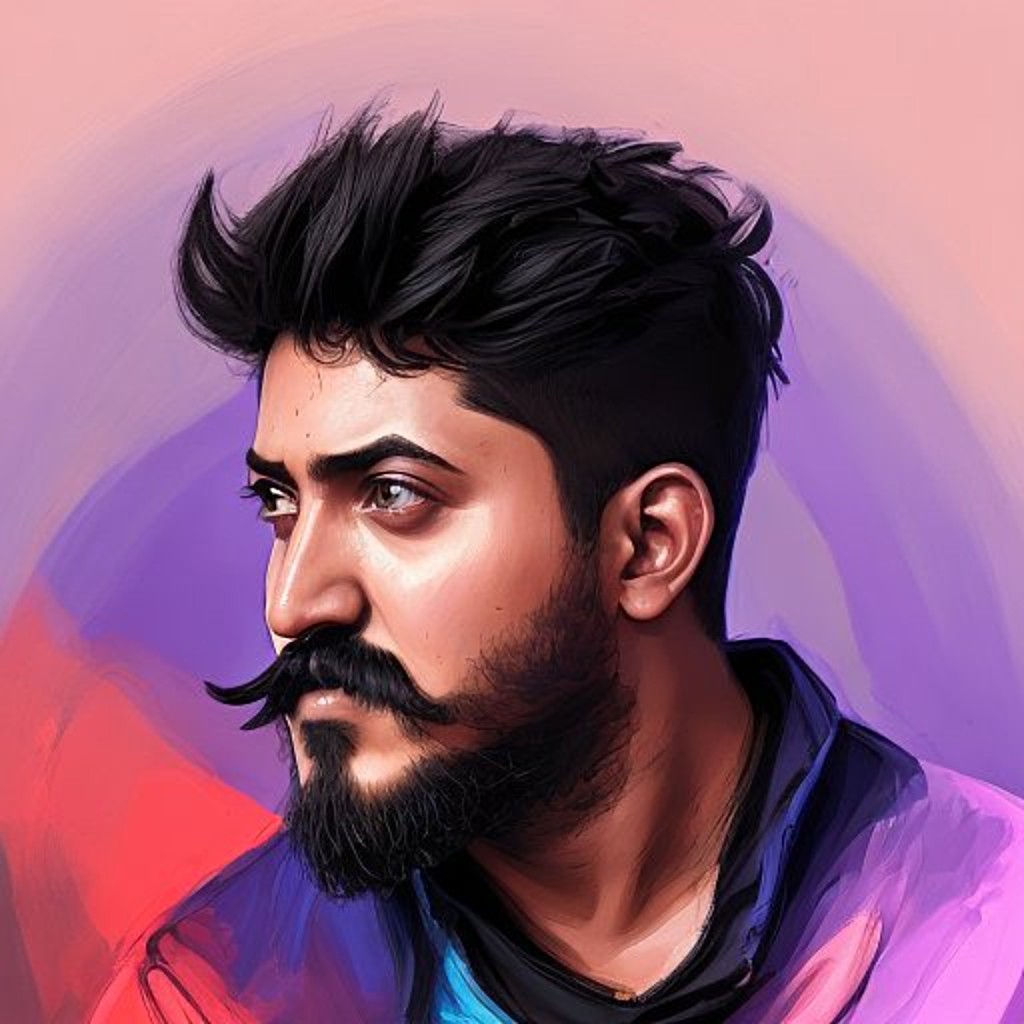
π Introduction
Have you ever found yourself dealing with values that can be null
or undefined
and needing to provide a default? If so, you're in luck! TypeScript's Nullish Coalescing Operator (??
) offers a clean and concise way to handle these situations. In this post, we'll explore what this operator is, how it works, and why you should consider using it in your TypeScript projects.
π What is Nullish Coalescing?
The Nullish Coalescing Operator (??
) is a logical operator that returns its right-hand operand when its left-hand operand is null
or undefined
, and otherwise returns its left-hand operand. This operator is especially useful when dealing with default values in your code.
π Syntax
let result = value ?? defaultValue;
value
: The variable or expression you are checking.defaultValue
: The value to use ifvalue
isnull
orundefined
.
βοΈ Before and After: Logical OR vs. Nullish Coalescing
Using Logical OR (||
) π
Before the introduction of the Nullish Coalescing Operator, developers often used the logical OR (||
) to provide default values. However, this approach can lead to unexpected results because it considers all falsy values (e.g., 0
, ""
, false
) as needing a default.
Case 1: User Input is an Empty String
let userInput = ""; // User input is an empty string
let username = userInput || "Guest";
console.log(username); // Output: "Guest" (not desired) β
Case 2: User Input is undefined
let userInput = undefined; // User input is undefined
let username = userInput || "Guest";
console.log(username); // Output: "Guest" (desired) β
Case 3: User Input has a Value
let userInput = "John"; // User input is "John"
let username = userInput || "Guest";
console.log(username); // Output: "John" (desired) β
Using Nullish Coalescing (??
) π
With the Nullish Coalescing Operator, only null
or undefined
trigger the default value, making the code more predictable.
Case 1: User Input is an Empty String
let userInput = ""; // User input is an empty string
let username = userInput ?? "Guest";
console.log(username); // Output: "" (desired) β
Case 2: User Input is undefined
let userInput = undefined; // User input is undefined
let username = userInput ?? "Guest";
console.log(username); // Output: "Guest" (desired) β
Case 3: User Input has a Value
let userInput = "John"; // User input is "John"
let username = userInput ?? "Guest";
console.log(username); // Output: "John" (desired) β
π Real-World Example:
Configuration Settings
Consider a scenario where you have an application with optional configuration settings. Using the Nullish Coalescing Operator, you can provide default values only when necessary.
function initializeSettings(config) {
let settings = {
theme: config.theme ?? "light",
debugMode: config.debugMode ?? false,
maxItems: config.maxItems ?? 10
};
console.log(settings);
}
// User configuration
let userConfig = {
theme: "dark",
debugMode: null,
maxItems: 0
};
initializeSettings(userConfig);
// Output: { theme: "dark", debugMode: false, maxItems: 0 } β
π Handling Edge Cases
One of the key benefits of the Nullish Coalescing Operator is that it only considers null
or undefined
, unlike the logical OR operator, which also considers falsy values. This distinction is crucial in scenarios where 0
, false
, or ""
are valid values that should not be replaced by a default.
let quantity = 0;
let defaultQuantity = quantity ?? 10;
console.log(defaultQuantity); // Output: 0 (desired) β
π― Benefits of Using Nullish Coalescing
The Nullish Coalescing Operator brings several advantages:
Clarity: It clearly expresses the intent to provide a default value only when dealing with
null
orundefined
.Reliability: It avoids unintended replacements of valid falsy values (
0
,""
,false
).Conciseness: It reduces the need for verbose conditional checks.
π Conclusion
The Nullish Coalescing Operator (??
) is a powerful addition to TypeScript, making it easier to handle default values in a clear and reliable manner. Whether you're dealing with user inputs, configuration settings, or any other nullable values, this operator can simplify your code and reduce potential bugs.
Give it a try in your next TypeScript project and experience the benefits firsthand. If you have any questions or examples to share, feel free to leave a comment below!
Happy coding! π
Subscribe to my newsletter
Read articles from Jobin Mathew directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
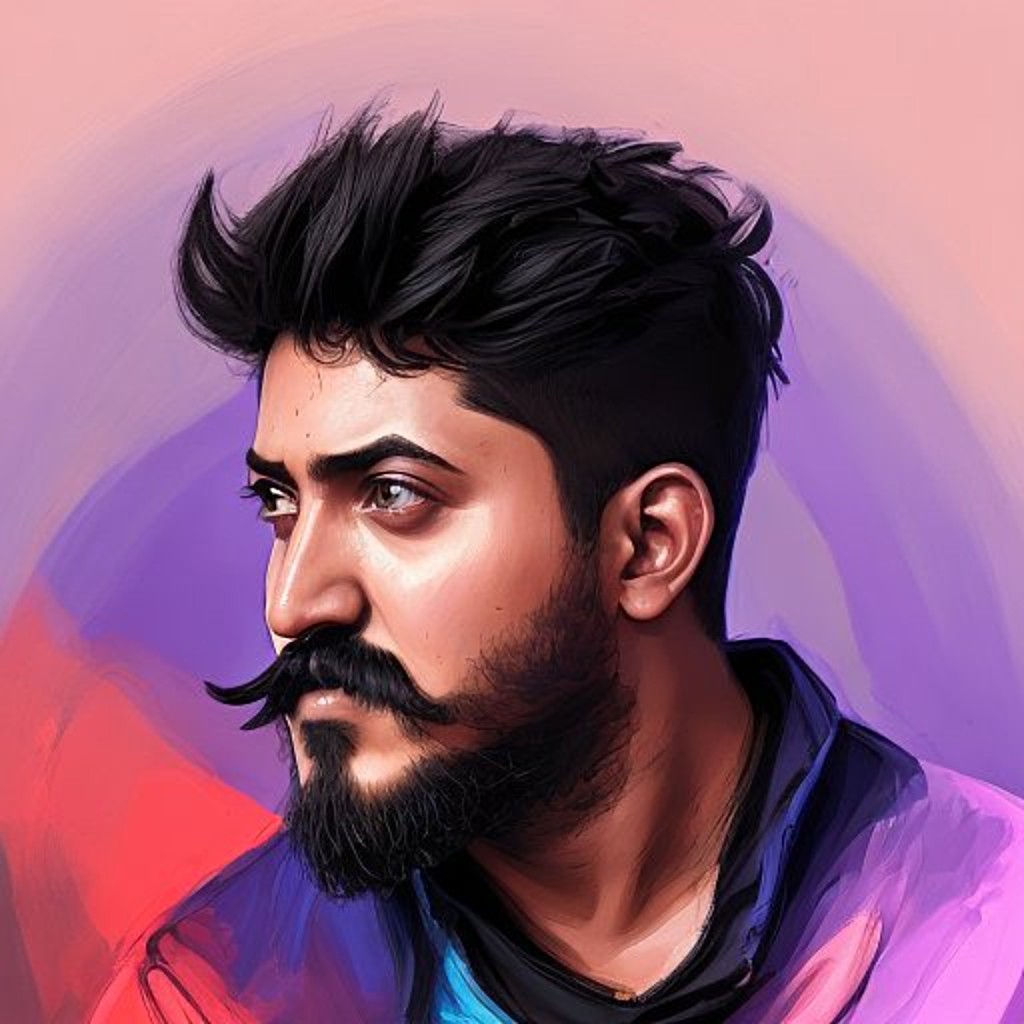
Jobin Mathew
Jobin Mathew
Hey there! I'm Jobin Mathew, a passionate software developer with a love for Node.js, AWS, SQL, and NoSQL databases. When I'm not working on exciting projects, you can find me exploring the latest in tech or sharing my coding adventures on my blog. Join me as I decode the world of software development, one line of code at a time!