Using Environment Variables with flutter_dotenv DotEnv in Flutter: Step by Step Guide
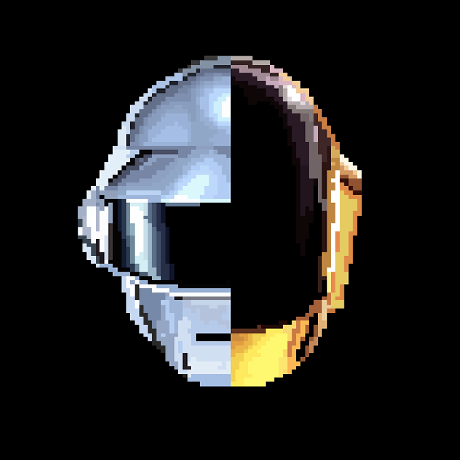
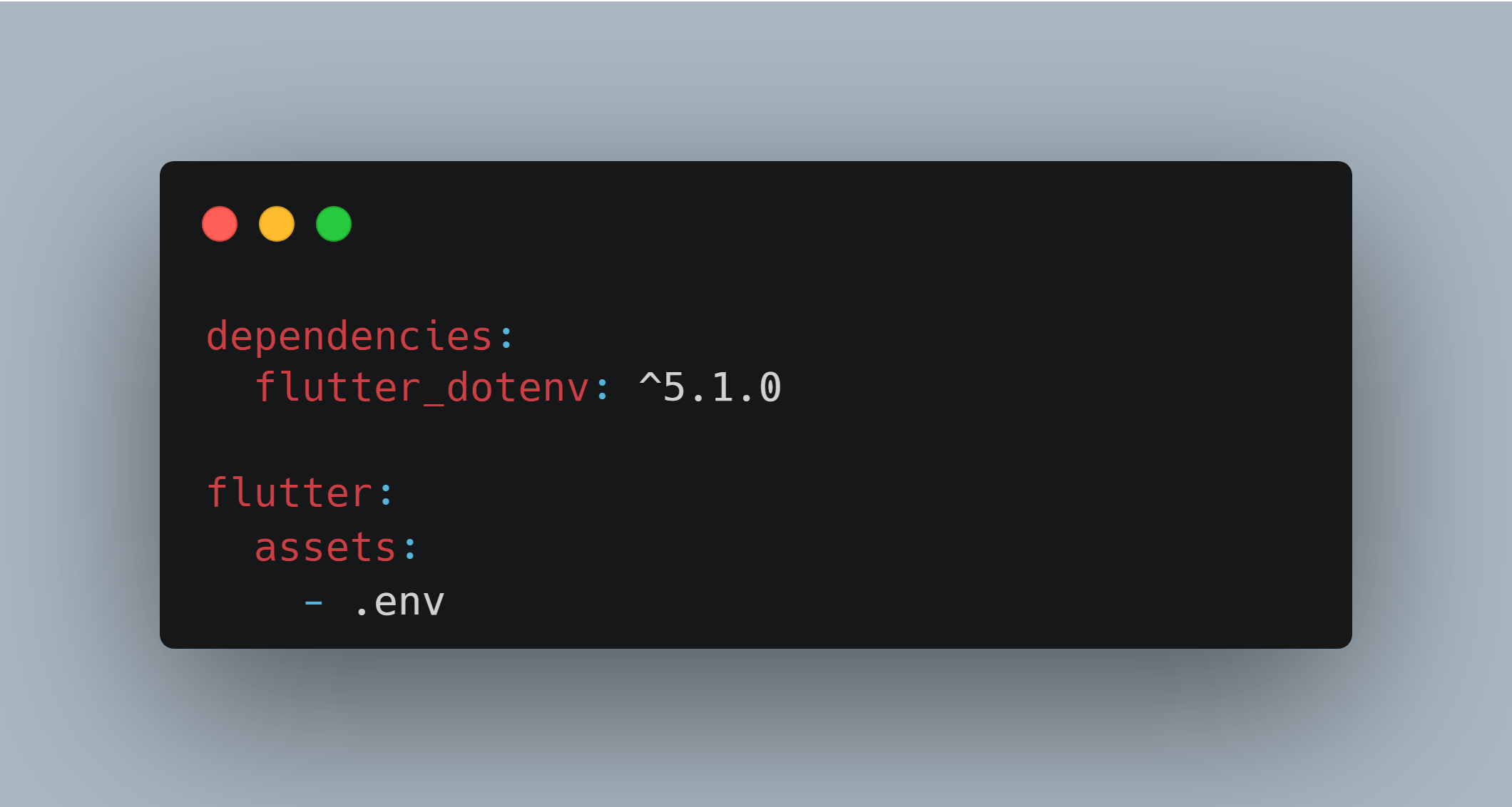
Introduction
When building Flutter applications, it's essential to manage sensitive data like API keys, database credentials, or environment-specific configurations. Hardcoding these values directly in your code is a security risk and can lead to issues when deploying your app to different environments.
That's where .env
variables come in – a simple and effective way to store and manage environment-specific configurations. In this blog, we’ll explore how to use .env
files to manage environment variables in your Flutter app, with a step-by-step guide and a practical example.
Some concepts
What Are .env
Files?
.env
files are simple text files used to store environment-specific variables. They typically contain key-value pairs, like API keys or base URLs, which can be accessed throughout your application.
Why Use .env
Files?
Separation of Concerns: Keep configuration settings separate from your code.
Security: Store sensitive information like API keys securely.
Flexibility: Easily switch between different environments (development, staging, production).
Step-by-Step Guide
Prerequisites
Before we begin, ensure you have the following:
- A Flutter project set up.
Step 1: Add the dotenv
package
First, add the dotenv
package to your pubspec.yaml
file:
dependencies:
flutter_dotenv: ^5.1.0
Step 2: Create a .env
File
Create a .env
file in the root directory of your Flutter project. This file will store your environment variables.
API_KEY=your_api_key_here
BASE_URL=https://example.com
Step 3: Update pubspec.yaml
Ensure that the .env
file is included as an asset in your project. Modify your pubspec.yaml
file to include the .env
file:
flutter:
assets:
- .env
Important: Run flutter pub get
to apply the changes.
Step 4: Load the .env
File
In your main.dart
file, import flutter_dotenv
and load the .env
file before running the app:
import 'package:flutter_dotenv/flutter_dotenv.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized(); // Ensure Flutter is initialized
try {
await dotenv.load(fileName: ".env"); // Load environment variables
} catch (e) {
throw Exception('Error loading .env file: $e'); // Print error if any
}
runApp(const MainApp()); // Runs the app
}
Ensure Flutter is initialized:
WidgetsFlutterBinding.ensureInitialized()
ensures that Flutter's framework is initialized before loading the.env
file. This is important becausedotenv.load()
relies on Flutter's file system to read the.env
file.Load environment variables:
await dotenv.load(fileName: ".env")
loads the environment variables from the.env
file. Theawait
keyword ensures that the loading process is completed before proceeding.Error handling: The
try-catch
block catches any errors that might occur while loading the.env
file. If an error occurs, it throws an exception with a meaningful error message.Run the app: Finally,
runApp(const MainApp())
runs the app.
Step 5: Access Environment Variables
You can access the environment variables using dotenv.env
:
import 'package:flutter_dotenv/flutter_dotenv.dart';
class ApiService {
final String baseUrl = dotenv.env['BASE_URL'] ?? 'default_url';
final String apiKey = dotenv.env['API_KEY'] ?? 'default_key';
// Use baseUrl and apiKey in your API calls
}
Troubleshooting
Common Issues:
File Not Found: Ensure the
.env
file is in the root directory and correctly specified inpubspec.yaml
.File Not Loading: Confirm that
await dotenv.load()
is called before running the app.
Note: Remember to add your .env
file to your .gitignore
file to avoid committing sensitive data to your version control system.
By following these steps and using flutter_dotenv
in your Flutter app, you'll be able to manage your environment-specific configurations securely and efficiently.
I hope this helps! Let me know if you have any questions.
Aditional Resources:
Topics you may be interested in:
Subscribe to my newsletter
Read articles from Mateo Ramirez Rubio directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
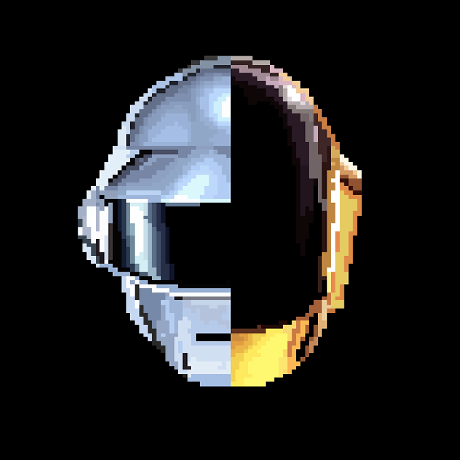