Ansible Role, Playbook, and Inventory: A Comprehensive Guide

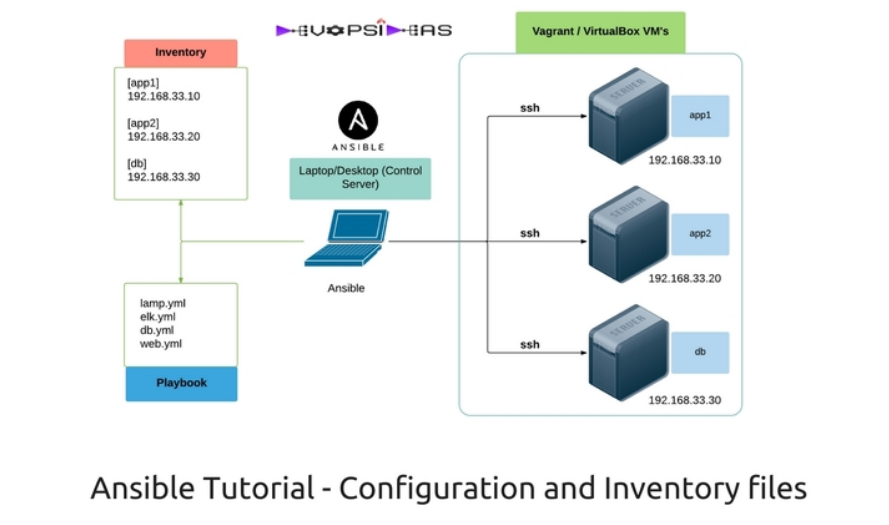
Introduction
Hello, fellow tech enthusiasts and software engineers! If you're looking to automate your infrastructure and streamline your workflows, you've probably heard of Ansible. Ansible is a powerful automation tool that simplifies the management of IT environments. In this guide, we'll dive into the core components of Ansible: Roles, Playbooks, and Inventory. Whether you're new to Ansible or looking to deepen your understanding, this friendly guide will walk you through everything you need to know. Let's get started!
Main Body
1. Introduction to Ansible
Before we dive into Roles, Playbooks, and Inventory, let's take a moment to understand what Ansible is and why it's such a valuable tool for IT automation.
What is Ansible?
Ansible is an open-source automation tool used for configuration management, application deployment, and task automation. It uses simple, human-readable YAML syntax to describe automation jobs, making it easy to learn and use.
Why Use Ansible?
Simplicity: Ansible's simple syntax makes it accessible to both beginners and experienced users.
Agentless: Ansible doesn't require any agents to be installed on managed nodes, reducing overhead.
Idempotent: Ansible ensures that tasks are idempotent, meaning they produce the same result regardless of how many times they are run.
Suggested Illustration: Create a diagram showing Ansible at the center, connecting to various managed nodes (e.g., servers, cloud instances, network devices) to illustrate the concept of agentless automation.
2. Understanding Ansible Roles
Ansible Roles are a way to organize playbooks and tasks in a structured manner. Roles allow you to break down complex automation tasks into reusable components.
Step-by-Step Guide to Creating an Ansible Role:
Install Ansible:
Ensure Ansible is installed on your control node. You can install Ansible using pip:
shCopy codepip install ansible
Create a New Role:
Use the
ansible-galaxy
command to create a new role:shCopy codeansible-galaxy init my_role
Role Directory Structure:
The
ansible-galaxy
command generates a directory structure for your role:pythonCopy codemy_role/ ├── defaults ├── files ├── handlers ├── meta ├── tasks ├── templates └── vars
Each directory has a specific purpose (e.g.,
tasks
contains the main tasks to be executed,templates
holds Jinja2 templates).
Define Tasks:
Open the
tasks/main.yml
file and define the tasks for your role:yamlCopy code--- - name: Install Nginx apt: name: nginx state: present - name: Start Nginx service: name: nginx state: started enabled: true
Use the Role in a Playbook:
Create a playbook that uses the role:
yamlCopy code--- - hosts: webservers roles: - my_role
Suggested Illustration: Create an image showing the directory structure of an Ansible role, highlighting the purpose of each directory (e.g., tasks, templates, handlers).
3. Writing Ansible Playbooks
Ansible Playbooks are YAML files that define a series of tasks to be executed on a group of hosts. Playbooks are the core of Ansible automation, allowing you to describe your desired state and actions.
Step-by-Step Guide to Writing an Ansible Playbook:
Create a Playbook File:
Create a new YAML file for your playbook:
shCopy codetouch my_playbook.yml
Define Playbook Structure:
A playbook consists of one or more plays. Each play targets a group of hosts and defines tasks to be executed:
yamlCopy code--- - name: Ensure Nginx is installed and running hosts: webservers become: yes tasks: - name: Install Nginx apt: name: nginx state: present - name: Start Nginx service: name: nginx state: started enabled: true
Use Variables:
Playbooks can use variables to make them more flexible and reusable:
yamlCopy code--- - name: Ensure Nginx is installed and running hosts: webservers become: yes vars: nginx_package: nginx tasks: - name: Install Nginx apt: name: "{{ nginx_package }}" state: present - name: Start Nginx service: name: nginx state: started enabled: true
Include Handlers:
Handlers are tasks that are triggered by other tasks. They are typically used to restart services after a configuration change:
yamlCopy code--- - name: Ensure Nginx is installed and running hosts: webservers become: yes tasks: - name: Install Nginx apt: name: nginx state: present notify: Restart Nginx - name: Copy Nginx config template: src: nginx.conf.j2 dest: /etc/nginx/nginx.conf notify: Restart Nginx handlers: - name: Restart Nginx service: name: nginx state: restarted
Suggested Illustration: Create an image showing the structure of an Ansible playbook, highlighting the different sections (e.g., hosts, tasks, variables, handlers).
4. Managing Inventory in Ansible
Ansible Inventory defines the hosts and groups of hosts on which Ansible tasks are executed. Inventory can be static or dynamic and is a crucial part of managing your infrastructure with Ansible.
Step-by-Step Guide to Managing Ansible Inventory:
Create a Static Inventory File:
Create a new inventory file:
shCopy codetouch inventory
Define Hosts and Groups:
Add hosts and groups to your inventory file:
iniCopy code[webservers] webserver1 ansible_host=192.168.1.10 webserver2 ansible_host=192.168.1.11 [dbservers] dbserver1 ansible_host=192.168.1.20 dbserver2 ansible_host=192.168.1.21
Use Inventory with Playbooks:
Specify the inventory file when running a playbook:
shCopy codeansible-playbook -i inventory my_playbook.yml
Dynamic Inventory:
Dynamic inventory allows you to generate inventory from external sources, such as cloud providers.
Use a dynamic inventory script or plugin to generate inventory dynamically:
shCopy codeansible-playbook -i dynamic_inventory.py my_playbook.yml
Suggested Illustration: Create an image showing a sample inventory file with hosts and groups, highlighting the structure and usage in playbooks.
5. Putting It All Together
Now that we have a good understanding of Roles, Playbooks, and Inventory, let's see how they work together in a real-world scenario.
Step-by-Step Guide to Using Roles, Playbooks, and Inventory Together:
Create a Role:
Create a role to install and configure Nginx:
shCopy codeansible-galaxy init nginx_role
Define tasks in
nginx_role/tasks/main.yml
:yamlCopy code--- - name: Install Nginx apt: name: nginx state: present - name: Copy Nginx config template: src: nginx.conf.j2 dest: /etc/nginx/nginx.conf notify: Restart Nginx - name: Start Nginx service: name: nginx state: started enabled: true
Create a Playbook:
Create a playbook that uses the role:
yamlCopy code--- - name: Deploy Nginx hosts: webservers become: yes roles: - nginx_role
Define Inventory:
Create an inventory file with webserver hosts:
iniCopy code[webservers] webserver1 ansible_host=192.168.1.10 webserver2 ansible_host=192.168.1.11
Run the Playbook:
Execute the playbook using the inventory:
shCopy codeansible-playbook -i inventory deploy_nginx.yml
Suggested Illustration: Create a comprehensive diagram showing the interaction between roles, playbooks, and inventory, highlighting the flow from defining tasks to executing the playbook on specified hosts.
Conclusion
Ansible is a powerful tool for automating your infrastructure, and understanding Roles, Playbooks, and Inventory is key to leveraging its full potential. By following this guide, you now have a solid foundation to create reusable roles, write effective playbooks, and manage your inventory
Subscribe to my newsletter
Read articles from Deepak parashar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
