Thread Synchronisation and Coordination in Python
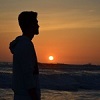
Thread synchronization and coordination are essential for developing multi-threaded applications in Python. These concepts help ensure that threads operate together smoothly, avoiding common issues such as data corruption, race conditions, and deadlocks. By mastering these techniques, you can effectively manage concurrent operations in your programs.
What is Thread Synchronization?
Thread synchronisation involves controlling the execution of multiple threads to prevent them from interfering with each other when accessing shared resources. Without proper synchronisation, threads operating on shared data can produce unpredictable results. For instance, if two threads simultaneously update the same variable, the final value could become inconsistent.
Why Synchronise Threads?
Prevent Race Conditions: A race condition arises when the outcome of operations depends on the unpredictable timing of thread execution. Synchronization ensures that threads do not conflict when accessing shared resources.
Ensure Data Consistency: Synchronization coordinates thread access to prevent threads from reading or modifying data in ways that lead to incorrect or corrupted results.
Avoid Deadlocks: Deadlocks occur when two or more threads wait indefinitely for each other to release resources. Proper synchronization helps design systems that avoid such scenarios.
Synchronization Mechanisms in Python
Python offers several mechanisms for synchronizing threads and managing their interactions. The primary tools include:
1. Locks
Locks are the simplest form of synchronization. A lock permits only one thread to access a critical section of code or a shared resource at a time. When a thread acquires a lock, other threads must wait until the lock is released, ensuring that only one thread can execute the critical section of code, thus preventing interference.
2. RLocks (Reentrant Locks)
An RLock, or reentrant lock, is a type of lock that allows the same thread to acquire it multiple times without causing a deadlock. This is useful for scenarios where a thread needs to re-enter a critical section during nested operations. RLocks offer more flexibility than regular locks and help avoid deadlocks in complex situations.
3. Semaphores
Semaphores manage access to a limited number of resources. Unlike locks, which allow only one thread at a time, semaphores permit a specified number of threads to access a resource concurrently. Semaphores maintain a counter representing the number of available resources, and threads acquire and release semaphores to control their access.
4. Conditions
Conditions are used for more complex synchronisation scenarios, such as producer-consumer problems. A condition allows threads to wait for specific conditions to be met before proceeding. For example, a thread might wait until a resource becomes available before continuing its execution. Conditions help manage the state of shared resources and coordinate thread actions.
Common Synchronisation Issues
When handling multi-threading, several issues can arise if synchronisation is not properly managed:
1. Race Conditions
A race condition occurs when the outcome of operations depends on the order in which threads execute. For example, if two threads increment a shared counter without synchronisation, the final result may be incorrect. Proper use of locks and other synchronisation tools helps prevent race conditions.
2. Deadlocks
Deadlocks occur when two or more threads wait indefinitely for resources held by each other. For instance, if Thread A holds Resource 1 and waits for Resource 2 while Thread B holds Resource 2 and waits for Resource 1, neither thread can proceed. To prevent deadlocks, avoid nested locks and carefully design resource allocation strategies.
3. Livelocks
A livelock is similar to a deadlock but happens when threads continuously change states in response to each other without making progress. For example, two threads might repeatedly release and re-acquire locks without completing their tasks. Ensuring proper exit conditions and avoiding unnecessary lock acquisition can help prevent livelocks.
Best Practices for Thread Synchronisation
To effectively manage thread synchronisation and coordination, consider these best practices:
1. Keep Critical Sections Small
Minimise the amount of code executed while holding a lock. This reduces the time other threads spend waiting for the lock and enhances overall performance.
2. Use High-Level Concurrency Tools
Where possible, use high-level concurrency tools from Python’s standard library, such as thread pools from the concurrent.futures module. These tools simplify thread management and abstract away much of the complexity involved.
3. Avoid Nested Locks
Be cautious with nested locks, as they increase the risk of deadlocks. If nested locks are necessary, ensure they are acquired in a consistent order to prevent deadlock scenarios.
4. Test Thoroughly
Multi-threaded programs can be challenging to debug due to their non-deterministic behaviour. Test your application rigorously under various conditions to ensure that synchronisation mechanisms work as intended and handle edge cases effectively.
5. Use Timeouts
When waiting for a condition or acquiring a lock, consider using timeouts to avoid indefinite waiting. Timeouts help ensure that threads do not get stuck waiting forever, providing better control and recovery from synchronisation issues.
Effective thread synchronisation and coordination are crucial for developing reliable and efficient multi-threaded applications in Python. Whether you're enhancing your skills locally or pursuing advanced online Python training in Noida, Delhi, Pune and other cities across India, mastering these synchronisation mechanisms will enable you to manage concurrent operations effectively, ensuring that your programs run smoothly and correctly.
Subscribe to my newsletter
Read articles from Sanjeet Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
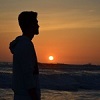
Sanjeet Singh
Sanjeet Singh
I am Sanjeet Singh, an IT professional with experience in the IT sector. I have a broad understanding of Data Analytics and proficiency across multiple layers of software development and testing, from the front end to the back end.