Understanding Null in JavaScript: A Primitive or an Object?
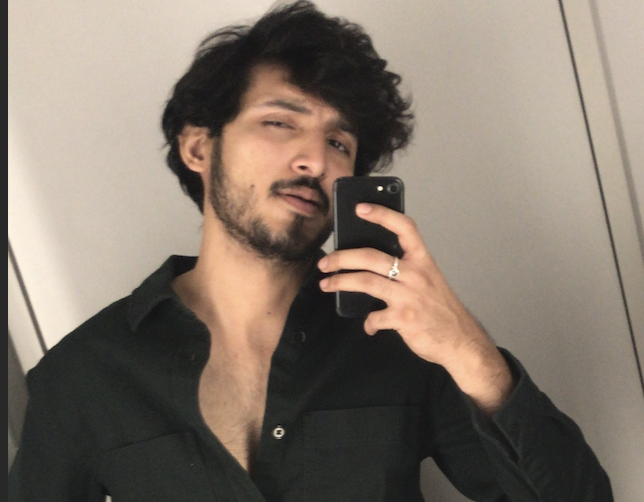
JavaScript is full of quirks, and one of the most puzzling is the treatment of null
. While the official ECMAScript specification defines null
as a primitive value, using the typeof
operator reveals a surprising result: it returns "object"
. This apparent contradiction has left many developers scratching their heads. In this article, we will explore the nature of null
in JavaScript, clarify its type, and uncover the historical reasons behind this behavior.
The typeof
Operator and Null
In JavaScript, the typeof
operator is a useful tool for determining the type of a variable. When applied to various data types, it produces expected results:
javascripttypeof 42 // "number"
typeof "hello" // "string"
typeof true // "boolean"
typeof undefined // "undefined"
However, when we check the type of null
, we encounter an unexpected outcome:
javascripttypeof null // "object"
This result seems contradictory, especially given the ECMAScript specification that categorizes null
as a primitive value. So, why does typeof null
return "object"
?
Checking with instanceof
To further investigate this anomaly, we can use the instanceof
operator to see if null
is an instance of the Object
type:
javascriptnull instanceof Object // false
This confirms that null
is not an object, despite what the typeof
operator suggests. This discrepancy has led many to label it as a bug in the JavaScript language.
The Historical Context
The reason behind the typeof null
returning "object"
can be traced back to the early days of JavaScript. In its initial version, JavaScript stored values in 32-bit units. The first three bits were designated for the data type, while the remaining bits represented the actual value.For all object types, the first three bits were always set to "000"
. Null
, being a special value that represents "nothing," was also represented by all zero bits. Thus, when the JavaScript engine encounters null
, it sees the first three bits as "000"
and incorrectly identifies it as an object.
This behavior was recognized as a bug, but it has been preserved for the sake of backwards compatibility. Changing this behavior now could break existing code that relies on the current implementation.
Subscribe to my newsletter
Read articles from Prince Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
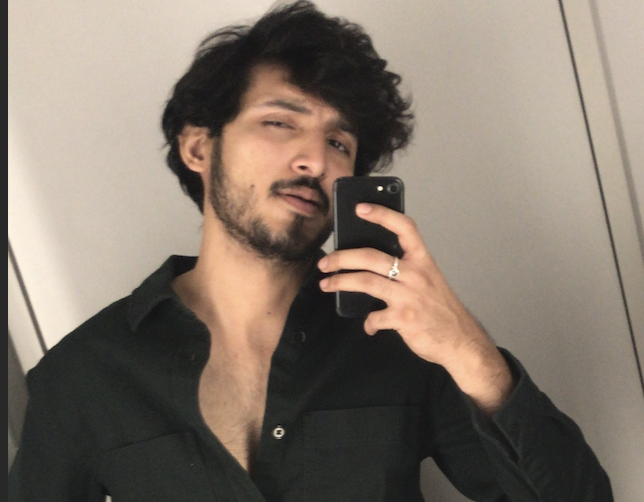
Prince Patel
Prince Patel
A passionate and enthusiastic programmer, having an appetite to learn and explore new things.I have experience working as a Developer in a Startup environment. Also, I am well versed in javascript and have hands-on experience working on full-stack projects using (RESTful API).