Python For DevOps: Part 1

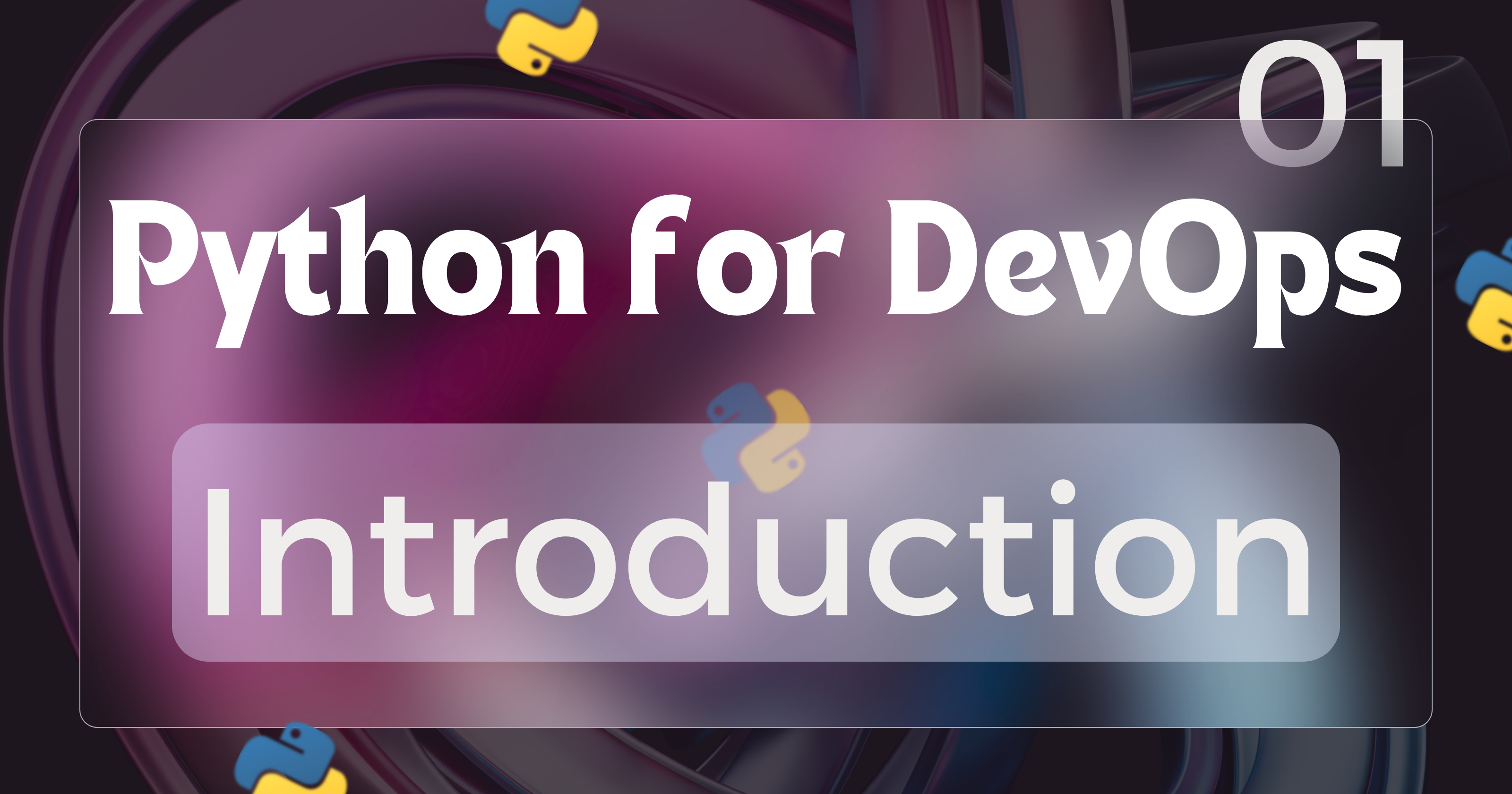
Introduction
Python is a programming language which is:
Easy to learn
simple syntax
easy to setup
Large ecosystem
many libraries
large community
Flexible
- you are not limited to language specifics
What Python is used for:
Web Development
Data Science
Machine Learning
Artificial Intelligence
Web Scrapping
Automation
Like automating DevOps tasks
Automating general tasks
Why Python with DevOps?
As an approach to culture, platform, and automation, Python is an important language for DevOps engineers or teams working to automate repetitive tasks, infrastructure management, provisioning, API driven deployment.
Setup python locally in Ubuntu
Head over to the official Python website and download the latest version of it according to your operating system.
Check that the Python is downloaded to your machine:
# this comamand is for Linux users
python3
# Checking the Python Path
which python3
Python Fundamentals:
Print Function
The print()
function in Python is used to output text or other data to the console.
objects: Any number of objects to be printed. Multiple objects are separated by a space by default.
sep: A string inserted between each object. The default is a space.
end: A string appended after the last object. The default is a newline.
file: A file-like object (stream). The default is
sys.stdout
.
# Basic usage
print("Hello, world!")
# Printing multiple items
print("The answer is", 42)
# Changing the separator
print("apple", "banana", "cherry", sep=", ")
# Changing the end character
print("Hello", end=" ")
print("world!")
# Printing to a file
with open("output.txt", "w") as f:
print("Hello, file!", file=f)
# This is the Output: Hello, file!
# Using formatted strings aka f-strings
name = "Alice"
age = 30
print(f"My name is {name} and I am {age} years old.")
Literals
A literal is a notation for representing a fixed value directly in the source code. Here is an example of a literal:
# Integer Literal:
93
# Floating-point Literal:
3.14
# String Literal:
"Hello, Suraj!"
# Boolean Literal
True
# None Literal: This represents the absesce of a value or null value
None
# Lits Literal:
[1,2,3,4]
# Simple program for literals
integer_literal = 42
string_literal = "Hello"
print(integer_literal, string_literal)
Variable
A variable is a named storage location in memory that holds a value that can be changed during program execution.
Variable Rules in Python
Names can contain letters, digits, and underscores: e.g.,
my_variable1
.Names must start with a letter or an underscore: e.g.,
_variable
orvariable1
.Names cannot start with a digit: e.g.,
1variable
is invalid.Names are case-sensitive: e.g.,
Variable
andvariable
are different.Cannot use Python reserved keywords: e.g.,
class
,def
,if
, etc.
Naming Convention:
Naming conventions is a convention(generally agreed scheme) for naming variables.
- Use lowercase with words separated with Underscores
# Simple program using variables
my_number1 = 10
my_number2 = 20
print("First No.", my_number1, "Second No.", my_number2)
Keywords
Keywords in Python are reserved words that have special meaning and cannot be used as identifiers (variable names, function names, etc.). You don't need to remember all these keywords
I just put it over here for reference.
Keyword | Description |
False | Boolean false value. |
None | Represents the absence of a value or a null value. |
True | Boolean true value. |
and | Logical AND operator. |
as | Used to create an alias. |
assert | Used for debugging purposes to test if a condition is true. |
async | Defines an asynchronous function (coroutine). |
await | Waits for the completion of an asynchronous function. |
break | Exits a loop prematurely. |
class | Defines a class. |
continue | Skips the rest of the current loop iteration and moves to the next iteration. |
def | Defines a function. |
del | Deletes an object. |
elif | Else if condition in control statements. |
else | Else condition in control statements. |
except | Catches exceptions in a try block. |
finally | Executes code after a try block, regardless of whether an exception occurred. |
for | Starts a for loop. |
from | Imports specific parts of a module. |
global | Declares a global variable. |
if | Starts an if condition. |
import | Imports a module. |
in | Check if a value is present in a sequence. |
is | Tests for object identity. |
lambda | Creates an anonymous function. |
nonlocal | Declares a non-local variable. |
not | Logical NOT operator. |
or | Logical OR operator. |
pass | A null statement; does nothing. |
raise | Raises an exception. |
return | Exits a function and returns a value. |
try | Starts a try block for exception handling. |
while | Starts a while loop. |
with | Used to simplify exception handling. |
yield | Pauses a function and returns an intermediate value; used in generators. |
Expressions and statements
Expressions are code segments that evaluate a value, while statements are instructions that perform an action in a program. A program is made up of several statements.
# These are the exmaple of Expressions:
# 1. Arithmetic expression:
2+3
# 2. String concatination
"Hello, "+" World!"
# 3. Function call expression:
len("example")
# 4. Boolean expression:
5>2
# List indexing:
my_list[1]
# Simple program with expressions and statements
a = 5 # this is a statement (assignment statement with an expression on the right side)
b = 10 # this is a statement (assignment statement with an expression on the right side)
result = a + b # this is a statement (assignment statement with an expression on the right side)
print("Result:", result) # this is a statement (function call statement with an expression as an argument)
Comments
Comments in Python are used to explain code logic, making it easier for others to understand what the code does. It also helps to document the code’s purpose, usage, and functionality and enhances maintainability by making the code simpler to manage and update.
Comments are of two types:
- Simple Line Comments that start with '#' and below is an example.
x = 10 # Assigning 10 to x
print(x) # Printing the value of x
- Multi-line Comments: that uses triple quotes (
'''
or"""
).
"""
This is a multi-line comment.
Hi there suraj this side.
"""
Indentation in Python
Indentation means spacing in statements in Python is very important. For example, in below code snippet below, you can see the print function has a lot more space from its original position which will give an IndentationError: unexpected indent
.
# This program will give indentation error
name = "Suraj"
print(name)
Data Types
Different data types can do different things: We will see two immutable data types in this blog rest of all we'll learn in the journey...
Number Data Types
# Simple program with number data types
pi = 3.14
print("Pi:", pi)
# Output: 3.14
Strings Data Types
# Simple program with strings
message = "Hello, Python!"
print(message)
String Concatenation
Adding strings together.
# String Concatenation program
print("Hello Suraj" + "Are you learning Python?") # we concatenate using '+' symbol
Instead of writing above style, we can usef-string
:
# This is a formatted string literal, introduced in Python 3.6
print(f"Hello Suraj, are you {21} years old?")
# Output:
Hello Suraj, are you 21 years old?
String Methods
String methods...
upper(): Used to convert all characters in a string to uppercase.
# Convert to uppercase message = "hello, python!" print(message.upper()) # Output: HELLO, PYTHON!
lower(): Convert all characters in a string to lowercase.
# Convert to lowercase message = "HELLO, PYTHON!" print(message.lower()) # Output: hello, python!
strip(): Remove leading and trailing whitespace.
# Remove whitespace message = " hello, python! " print(message.strip()) # Output: hello, python!
replace(): Replace a substring with another substring.
# Replace substring message = "hello, python!" print(message.replace("python", "world")) # Output: hello, world!
split(): Split a string into a list of substrings.
# Split string into list message = "hello, python!" words = message.split() print(words) # Output: ['hello,', 'python!']
join(): Join elements of a list into a string.
# Join list into string words = ["hello", "python"] message = " ".join(words) print(message) # Output: hello python
find(): Find the first occurrence of a substring.
# Find substring message = "hello, python!" position = message.find("python") print("Position of 'python':", position) # Output: Position of 'python': 7
count(): Count the number of occurrences of a substring.
# Count occurrences of substring message = "hello, python! python is fun!" count = message.count("python") print("Occurrences of 'python':", count) # Output: Occurrences of 'python': 2
capitalize(): Capitalize the first character of the string.
# Capitalize first character message = "hello, python!" print(message.capitalize()) # Output: Hello, python!
title(): Capitalize the first character of each word.
# Capitalize first character of each word message = "hello, python!" print(message.title()) # Output: Hello, Python!
swapcase(): Swap the case of all characters in the string.
# Swap case of characters message = "Hello, Python!" print(message.swapcase())
Escaping Characters
Escaping characters in Python allows you to include special characters in strings that would otherwise be difficult or impossible to include directly. This is done using a backslash (\
) followed by the character you want to escape.
# 1. Using a newline character(\n)
print("Hello\nSuraj")
#Output:
Hello
Suraj
# 2. Tab Character(\t)
print("Hell0\tSuraj")
# Output:
Hello Wolrd
# 3. Backslash Character(\\)
print("This is a backslash: \\")
# Output:
This is a backslash: \ # This is the output because, The \\ sequence inserts a single backslash.
# 4. Single Qoute(\') and Double Qoute(\")
print('I said, \'This is single qoute!\'')
print("I said, \"This is double qoute!\"")
# Output:
He said, 'Hello, World!'
He said, "Hello, World!"
# 5. Carriage Return(\r)
print("Hello\rBro")
# Output:
Bro # The \r character moves the cursor to the beginning of the line, so "Bro" overwrites "Hello.
# 6. Bell/Alert(\a)
print("Hello\aBro")
# Output: You might hear a beep sound, and the text "HelloBro" will be printed. BecauseThe \a character triggers the system bell (alert).
String Characters & Slicing
A string is a sequence of characters. Each character in a string can be accessed using its index. Python strings are zero-indexed, meaning the first character has an index 0
, the second character has an index 1
, and so on.
text = "Python"
print(text[0]) # Output: P
print(text[1]) # Output: y
print(text[-1]) # Output: n (negative indexing starts from the end)
String Slicing
String slicing allows you to extract a portion of a string by specifying a start and end index. The syntax for slicing is: string[start:stop:step]
Examples of Slicing
# Basic Slicing
text = "Python"
print(text[1:4]) # Output: yth
# Omitting Start or Stop
text = "Python"
print(text[:4]) # Output: Pyth (Omits the start index, starts from the beginning)
print(text[2:]) # Output: thon (Omits the end index, goes till the end)
# Using Step
text = "Python"
print(text[::2]) # Output: Pto (Takes every 2nd character)
# Negative Indices and Slicing
text = "Python"
print(text[-5:-1]) # Output: ytho (From index -5 to -2, -1 is not included)
print(text[::-1]) # Output: nohtyP (Reverses the string)
# Reversing a String
text = "Python"
reversed_text = text[::-1]
print(reversed_text) # Output: nohtyP
Booleans
# Simple program with booleans
is_active = True
print("Is active:", is_active)
# Output:
Build-In Functions
# Simple program using built-in functions
message = "Hello, Python!"
print("Length of message:", len(message))
# Output:
Enums
from enum import Enum
# Define an enum for days of the week
class Day(Enum):
MONDAY = 1
TUESDAY = 2
WEDNESDAY = 3
THURSDAY = 4
FRIDAY = 5
SATURDAY = 6
SUNDAY = 7
# Accessing enum members
print(Day.MONDAY) # Output: Day.MONDAY
print(Day.MONDAY.name) # Output: MONDAY
print(Day.MONDAY.value) # Output: 1
# Iterating over enum members
for day in Day:
print(day.name, day.value)
# this is the Output:
"""
MONDAY 1
TUESDAY 2
WEDNESDAY 3
THURSDAY 4
FRIDAY 5
SATURDAY 6
SUNDAY 7
"""
User input
# Simple program with user input
name = input("Enter your name: ")
print(f"Hello, {name}")
# Output:
# Enter your name: Suraj # In console
# Hello, Suraj # actual output after entring name
Logic and Bit operators
Operators
# Simple program with operators
result = 10 + 5
print("Result of addition:", result)Logic and Bit operators
Operators
# Simple program with operators
result = 10 + 5
print("Result of addition:", result)
# Output:
Arithmetic Operators
# Simple program with arithmetic operators
quotient = 10 / 2
print("Quotient:", quotient)
# Output:
Comparison Operators
# Simple program with comparison operators
is_equal = (10 == 10)
print("10 is equal to 10:", is_equal)
# Output:
Boolean Operators
# Simple program with boolean operators
result = True and False
print("True and False:", result)
# Output:
Bitwise Operators
# Simple program with bitwise operators
bitwise_result = 5 & 3
print("5 AND 3:", bitwise_result)
# Output:
is & in Operators
# Simple program with 'is' and 'in' operators
x = 5
y = 5
is_same = (x is y)
contains = 'a' in 'apple'
print("x is y:", is_same)
print("'a' in 'apple':", contains)
# Output:
Ternary Operator
# Simple program with ternary operator
x = 10
result = "Even" if x % 2 == 0 else "Odd"
print("x is:", result)
# Output
Control Flow - Conditional Blocks & Loops:
Conditional Statements:
if Statement: Executes a block of code if a specified condition is true.
# Simple if statement
x = 10
if x > 0:
print("Positive")
# Output:
Positive
if-else Statement: Executes one block of code if a condition is true, and another block of code if the condition is false.
# Simple if-else statement
x = -5
if x > 0:
print("Positive")
else:
print("Non-positive")
# Output
Non-positive
if-elif-else Statement: Executes different blocks of code based on multiple conditions.
# Simple if-elif-else statement
x = 0
if x > 0:
print("Positive")
elif x < 0:
print("Negative")
else:
print("Zero")
# Output:
Zero
Loops:
while Loop: Repeats a block of code as long as a specified condition is true.
# Simple while loop
count = 1
while count <= 5:
print("Count:", count)
count += 1
# Output:
Count: 1
Count: 2
Count: 3
Count: 4
Count: 5
for Loop: Iterates over a sequence (such as a list, tuple, or string).
# Simple for loop
for i in range(5):
print("Iteration:", i)
# Output:
Iteration: 0
Iteration: 1
Iteration: 2
Iteration: 3
Iteration: 4
Arithmetic Operators
# Simple program with arithmetic operators
quotient = 10 / 2
print("Quotient:", quotient)
Comparison Operators
# Simple program with comparison operators
is_equal = (10 == 10)
print("10 is equal to 10:", is_equal)
Boolean Operators
# Simple program with boolean operators
result = True and False
print("True and False:", result)
Bitwise Operators
# Simple program with bitwise operators
bitwise_result = 5 & 3
print("5 AND 3:", bitwise_result)
# Output:
is & in Operators
# Simple program with 'is' and 'in' operators
x = 5
y = 5
is_same = (x is y)
contains = 'a' in 'apple'
print("x is y:", is_same)
print("'a' in 'apple':", contains)
# Output:
Ternary Operator
# Simple program with ternary operator
x = 10
result = "Even" if x % 2 == 0 else "Odd"
print("x is:", result)
# Output
Control Flow - Conditional Blocks & Loops:
Conditional Statements:
if Statement: Executes a block of code if a specified condition is true.
# Simple if statement x = 10 if x > 0: print("Positive") # Output: Positive
if-else Statement: Executes one block of code if a condition is true, and another block of code if the condition is false.
# Simple if-else statement x = -5 if x > 0: print("Positive") else: print("Non-positive") # Output Non-positive
if-elif-else Statement: Executes different blocks of code based on multiple conditions.
# Simple if-elif-else statement x = 0 if x > 0: print("Positive") elif x < 0: print("Negative") else: print("Zero") # Output: Zero
Loops:
while Loop: Repeats a block of code as long as a specified condition is true.
# Simple while loop count = 1 while count <= 5: print("Count:", count) count += 1 # Output: Count: 1 Count: 2 Count: 3 Count: 4 Count: 5
for Loop: Iterates over a sequence (such as a list, tuple, or string).
# Simple for loop
for i in range(5):
print("Iteration:", i)
# Output:
Iteration: 0
Iteration: 1
Iteration: 2
Iteration: 3
Iteration: 4
Thanks for reading...
Subscribe to my newsletter
Read articles from Suraj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Suraj
Suraj
I'm a Developer from India with a passion for DevOps ♾️ and open-source 🧑🏻💻. I'm always striving for the best code quality and seamless workflows. Currently, I'm exploring AI/ML and blockchain technologies. Alongside coding, I write Technical blogs at Hashnode, Dev.to & Medium 📝. In my free time, I love traveling, reading books