Retrofit API Call with Clean Architecture in Kotlin: A Step-by-Step Guide
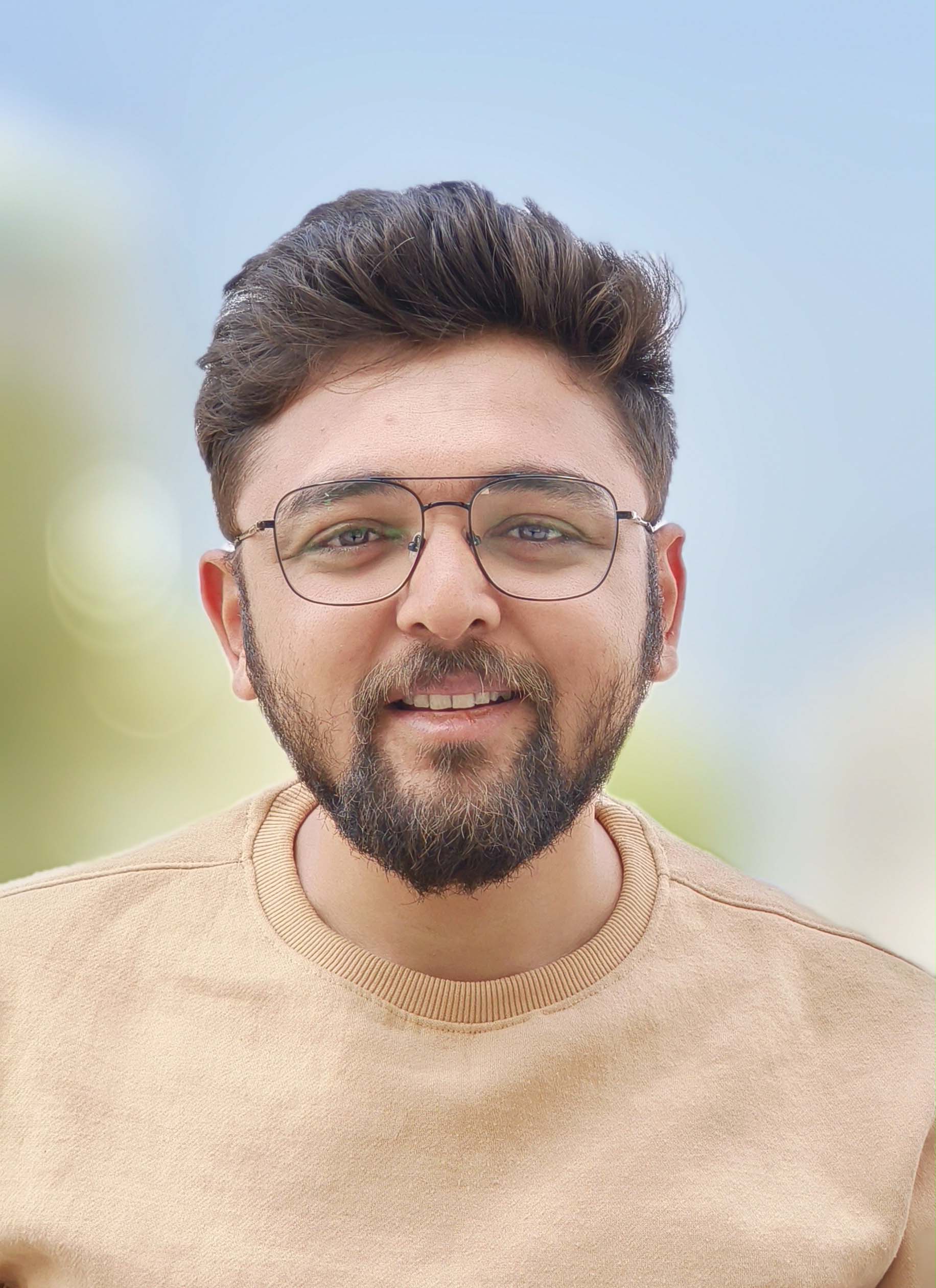
Table of contents
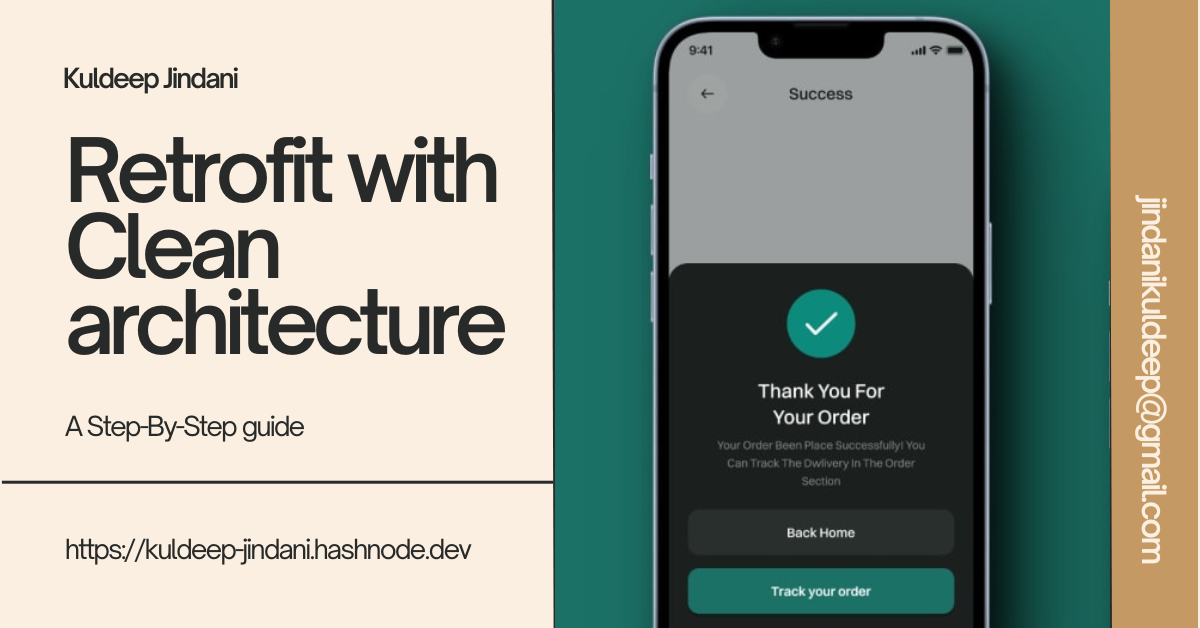
Implementing Retrofit with Clean Architecture in Kotlin involves several steps to ensure a modular and maintainable codebase. Here's a step-by-step guide:
Here we are using Kotlin as a coding language and Clean Architecture, Hilt as a dependency injection. So Let's start ๐๐๐
Implementing Retrofit with Clean Architecture in Kotlin involves setting up dependencies, defining data models and API interfaces, creating a Retrofit instance, defining use cases, managing UI-related data with ViewModel, and setting up dependency injection using Hilt. This approach ensures a modular and maintainable codebase.
Setup Dependencies:
Add Retrofit and related dependencies to your build.gradle
file:
implementation("com.squareup.retrofit2:retrofit:2.9.0")
implementation("com.squareup.retrofit2:converter-gson:2.9.0")
implementation("org.jetbrains.kotlinx:kotlinx-coroutines-core:1.5.2")
Define Data Models:
Create data classes representing your API responses:
data class User(val id: Int, val name: String)
Create API Interface:
- Define your API endpoints using Retrofit annotations:
interface ApiService {
@GET("users")
suspend fun getUsers(): List<User>
}
Implement Retrofit Instance:
- Set up the Retrofit instance in a singleton pattern
object RetrofitClient {
private const val BASE_URL = "https://api.example.com/"
val instance: ApiService by lazy {
Retrofit.Builder()
.baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.build()
.create(ApiService::class.java)
}
}
Define Use Cases
Create use cases to handle business logic:
class GetUsersUseCase(private val apiService: ApiService) {
suspend fun execute(): List<User> = apiService.getUsers()
}
Create ViewModel:
- Integrate with ViewModel to manage UI-related data
class UserViewModel(private val getUsersUseCase: GetUsersUseCase) : ViewModel() {
private val _users = MutableLiveData<List<User>>()
val users: LiveData<List<User>> get() = _users
fun fetchUsers() {
viewModelScope.launch {
_users.value = getUsersUseCase.execute()
}
}
}
Set Up Dependency Injection:
- Use Dagger or Hilt to manage dependencies:
@Module
@InstallIn(SingletonComponent::class)
object AppModule {
@Provides
fun provideApiService(): ApiService = RetrofitClient.instance
@Provides
fun provideGetUsersUseCase(apiService: ApiService): GetUsersUseCase =
GetUsersUseCase(apiService)
}
By following these steps, you can integrate Retrofit into your Kotlin application using Clean Architecture, ensuring a well-structured and scalable codebase.
Subscribe to my newsletter
Read articles from Kuldeep Jindani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
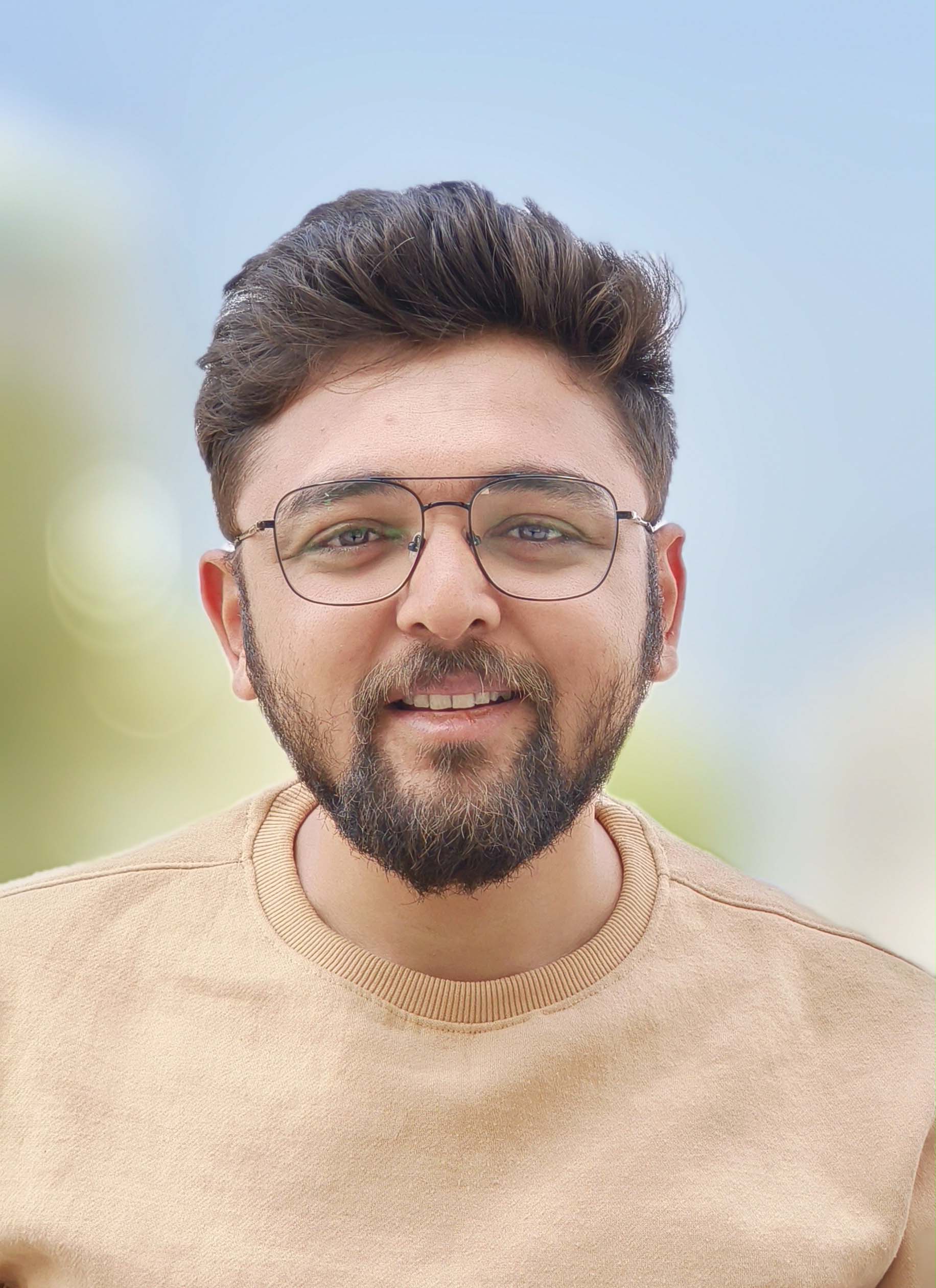
Kuldeep Jindani
Kuldeep Jindani
I have hands-on 6 years of experience in Android development with Java + Kotlin, Experience in most trending Android functionalities, UI Designs, Reusable Components, MVVM & MVI architectures, Testing, Bug Fixes, and self & quick learning skills. My expertise includes: Proficiency in Kotlin & Java Both Strong understanding of app architecture Like MVVM, Clean Architecture Experience with Jetpack Compose and coroutines and RxJava Collaboration with designers and product managers Commitment to delivering high-quality, testable code TECHNICAL SKILL SET Kotlin | Java | Jetpack Compose | Kotlin Multiplatform Platform (KMP) | Kotlin Flow | Firebase | Sqlite | Room/Realm database | Kotlin Coroutine | REST API | Web Service | MVVM/MVI/ Clean Architecture | Multiple screen support | JUnit/Mockito Unit Testing | Espresso UI Testing | Instrumental Testing | Version Controlling | Git | Jenkins CI/CD | Retrofit | Dagger/Hilt | BLE | CameraX | IOT