Mastering JPA and ORM: An In-Depth Guide to Best Practices, Transactional Handling, Lazy Loading, Bean Scopes, and ORM Mapping💻🤖🚀
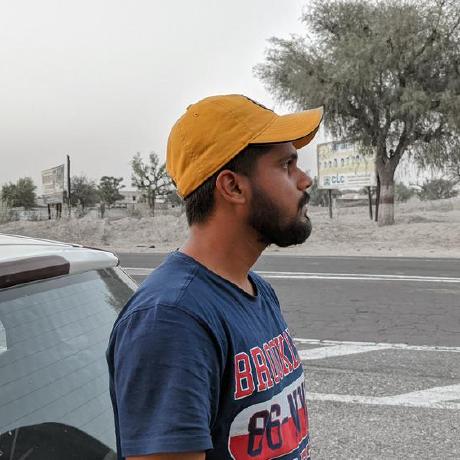
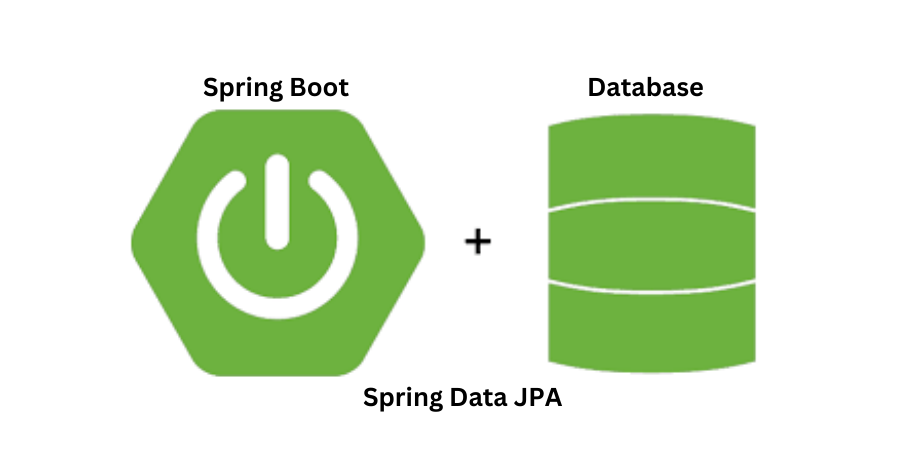
It's impossible to think of any real-time scenario of enterprise software or a product where there is no data involved. As the world is generating billions of TBs of data every second. The need for efficient data management is paramount for building robust and scalable applications. Java Persistence API (JPA) is a key player in this domain, simplifying the process of mapping Java objects to relational database tables and handling transactions. In This comprehensive guide, I will try to explore JPA and Object-Relational Mapping (ORM) in detail, covering best practices and considerations along with in-depth discussions on managing string data, transactional handling and various ORM mapping methods.
And, if you stay with me till the very end, you get two additional topics to be covered at the end. So, let's dive into these concepts and practices to enhance your understanding and application of JPA and ORM.
Introduction to JPA and ORM
The Java Persistence API (JPA) is a specification for object-relational mapping (ORM) in Java applications. ORM is a technique that allows developers to map Java objects to relational database tables, simplifying data access and manipulation. JPA provides a standardized approach to this mapping, allowing developers to focus on business logic rather than database interactions.
Core Concepts
Entities: Java classes annotated with
@Entity
that represent database tables.Entity Manager: A class responsible for managing the lifecycle of entities and handling CRUD operations.
Persistence Context: A context that holds a set of managed entity instances.
Here’s a basic example of an entity class:
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENITY)
private Long id;
@Column(length = 100, nullable = false)
private String name;
@Column(length =500)
private String email;
@Lob
private String postContent;
// Getters and setters
}
In this example, User
is an entity that maps to a database table with columns corresponding to the fields id
, name
, and email
and postContent.
String Data Management in JPA
Managing string data effectively is crucial for applications that handle a variety of textual information. JPA provides several ways to map and manage string data in your entities.
The @Column
annotation defines various attributes that the Strings can have, for example, in the field name, the length of the name can be uptill 100 characters, and the values can't be null, as the attribute nullable = false.
Similarly, for storing large amounts of contents such as 5000 words blog post in a String, we can use the @Lob
annotation, it indicates that the field postContent should be treated as a large object, which is generally suitable for storing extensive text or binary data.
Encoding and Localization
When dealing with string data, especially in applications that support multiple languages, consider encoding and localization:
Character Encoding: Ensure that your database and application use consistent character encoding (e.g., UTF-8) to handle special characters and international text correctly.
Localization: Use libraries or frameworks to manage translations and locale-specific data, ensuring your application can support various languages and formats.
ORM Mapping Methods
Object-Relational Mapping (ORM) is a technique that maps Java objects to relational database tables. JPA offers various methods for mapping entities and managing relationships.
Basic ORM Mapping Methods
- @Entity: Marks a class as a JPA entity, representing a table in the database.
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class User {
@Id
private Long id;
private String name;
private String email;
// Getters and setters
}
- @Id: Defines the primary key of the entity.
@Id
private Long id;
- @Column: Maps a field to a database column. It allows customization of column attributes, such as length and nullable constraints.
@Column(length = 100, nullable = false)
private String name;
- @Table: Customizes the table name and schema for the entity.
@Table(name = "users", schema = "public")
public class User {
// Fields and methods
}
Relationship Mapping
JPA provides several annotations for defining relationships between entities:
- @OneToOne: Defines a one-to-one relationship between entities.
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.OneToOne;
@Entity
public class Person {
@Id
private Long id;
@OneToOne
private Address address;
// Getters and setters
}
- @OneToMany: Defines a one-to-many relationship between entities.
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.OneToMany;
import java.util.Set;
@Entity
public class Author {
@Id
private Long id;
@OneToMany(mappedBy = "author")
private Set<Book> books;
// Getters and setters
}
- @ManyToOne: Defines a many-to-one relationship between entities.
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.ManyToOne;
@Entity
public class Book {
@Id
private Long id;
@ManyToOne
private Author author;
// Getters and setters
}
- @ManyToMany: Defines a many-to-many relationship between entities.
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.ManyToMany;
import java.util.Set;
@Entity
public class Student {
@Id
private Long id;
@ManyToMany
private Set<Course> courses;
// Getters and setters
}
Advanced Mapping Techniques
- @MappedSuperclass: Defines a superclass whose properties are inherited by its subclasses.
import javax.persistence.MappedSuperclass;
@MappedSuperclass
public abstract class BaseEntity {
private Long id;
// Common fields and methods
}
- @Embeddable: Defines a class whose properties can be embedded in other entities.
import javax.persistence.Embeddable;
@Embeddable
public class Address {
private String street;
private String city;
private String zipCode;
// Getters and setters
}
- @Embedded: Embeds an
@Embeddable
class within an entity.
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.Embedded;
@Entity
public class Customer {
@Id
private Long id;
@Embedded
private Address address;
// Getters and setters
}
Customizing ORM Mapping
- @AttributeOverride: Customizes the mapping of attributes in an embedded class.
import javax.persistence.AttributeOverride;
import javax.persistence.AttributeOverrides;
import javax.persistence.Column;
import javax.persistence.Embeddable;
@Embeddable
public class Address {
@Column(name = "street_name")
private String street;
@Column(name = "city_name")
private String city;
@Column(name = "postal_code")
private String zipCode;
// Getters and setters
}
- @JoinColumn: Specifies the column used for joining an entity relationship.
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
@Entity
public class Order {
@Id
private Long id;
@ManyToOne
@JoinColumn(name = "customer_id")
private Customer customer;
// Getters and setters
}
- @JoinTable: Customizes the join table for many-to-many relationships.
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.JoinTable;
import javax.persistence.ManyToMany;
import javax.persistence.JoinColumn;
@Entity
public class Student {
@Id
private Long id;
@ManyToMany
@JoinTable(name = "student_course",
joinColumns = @JoinColumn(name = "student_id"),
inverseJoinColumns = @JoinColumn(name = "course_id"))
private Set<Course> courses;
// Getters and setters
}
Additional tip here for managing unique records such as primary keys 🔑
The GenerationType enum in the JPA, provides different types of generation strategies for primary keys. They are:
- Auto - As you can see in the below code snippet, the GenerationType.AUTO strategy is one of the four types of generation strategies used in JPA for managing primary keys generation in the Relational Databases. This particular strategy lets the JPA decide which strategy to choose from the other three, namely SEQUENCE, IDENTITY and TABLE.
The main benefit of this strategy is it is ideal for implementing a beginner friendly project in a faster way, but generally I would not recommend this as it might throw non-unique primary keys sometimes.
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@Column(length = 100, nullable = false)
private String name;
@Column(length =500)
private String email;
@Lob
private String postContent;
// Getters and setters
}
IDENTITY - This is the typical auto-increment generation strategy for the primary key, here the field id will be auto-incremented whenever there is a suitable call to the database to create any particular entry related to the mapped JPA entity's object User.
The main use case is when using MySQL as a database(because MySql supports auto-incremental columns)
import javax.persistence.Entity; import javax.persistence.Id; @Entity public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(length = 100, nullable = false) private String name; @Column(length =500) private String email; @Lob private String postContent; // Getters and setters }
Sequence - This is the most used way to generate and manage the primary keys because Sequence is the standard way used to generate unique values in many RDBMS. In the below example, in attribute generator is "my_seq" and the annotation
@SequenceGenerator
is being used to map the sequence object "my_sequence" in the database for this primary key generation.It generally, requires a sequence object to be defined in the database.
@Id @GeneratedValue(strategy = GenerationType.SEQUENCE, generator = "my_seq") @SequenceGenerator(name = "my_seq", sequenceName = "my_sequence", allocationSize = 1) private Long id;
Table - This generation strategy works across all databases but the performance is less comparative to other strategies.
@Id @GeneratedValue(strategy = GenerationType.TABLE, generator = "my_table_gen") @TableGenerator(name = "my_table_gen", table = "my_table", pkColumnName = "gen_name", valueColumnName = "gen_value", pkColumnValue = "id_gen", allocationSize = 1) private Long id;
Transactional Management in JPA
Transactions are essential for maintaining data integrity and consistency. JPA provides mechanisms for managing transactions, both programmatically and declaratively.
Declarative Transactions
The @Transactional
annotation is used to define transaction boundaries declaratively in a Spring application:
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class OrderService {
@Transactional
public void placeOrder(Order order) {
// Business logic for placing an order
}
}
In this example, the placeOrder
method is executed within a transactional context. If an exception occurs, the transaction will be automatically rolled back.
Programmatic Transactions
For more control over transactions, you can manage them programmatically using the JPA EntityTransaction
:
import javax.persistence.EntityManager;
import javax.persistence.EntityTransaction;
public class OrderService {
private EntityManager entityManager;
public OrderService(EntityManager entityManager) {
this.entityManager = entityManager;
}
public void placeOrder(Order order) {
EntityTransaction transaction = entityManager.getTransaction();
try {
transaction.begin();
// Business logic for placing an order
transaction.commit();
} catch (Exception e) {
transaction.rollback();
throw e;
}
}
}
Programmatic transactions provide finer control but require manual management of transaction boundaries and exception handling.
Transactional Attributes
JPA and Spring support various transactional attributes to customize transaction behavior:
Isolation Levels: Define the level of isolation between transactions (e.g.,
READ_COMMITTED
,SERIALIZABLE
).Propagation Types: Specify how transactions are propagated (e.g.,
REQUIRED
,REQUIRES_NEW
).more details
The transactional attributes come in handy to customize transaction behavior using transactional attributes, specifically isolation levels and propagation types. These attributes help ensure data integrity and consistency while optimizing performance based on the requirements of your application.
Lazy Loading of Beans
Lazy loading defers the initialization of a bean or entity until it is actually needed. This can improve application performance by reducing unnecessary database access and resource usage.
Lazy Loading with JPA
In JPA, lazy loading is often used with associations between entities:
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.OneToMany;
import javax.persistence.FetchType;
import java.util.Set;
@Entity
public class Customer {
@Id
private Long id;
@OneToMany(fetch = FetchType.LAZY, mappedBy = "customer")
private Set<Order> orders;
// Getters and setters
}
In this example, the orders
collection is loaded lazily, meaning it is only fetched from the database when accessed for the first time.
Managing Lazy Loading
While lazy loading can optimize performance, it also introduces challenges:
LazyInitializationException: Occurs if the session is closed before the lazy-loaded data is accessed. To avoid this, ensure the session remains open or use techniques like fetch joins.
N+1 Select Problem: This issue arises when accessing a collection of entities lazily, resulting in multiple SQL queries. Use fetch joins or batch fetching to mitigate this problem.
Alternative Strategies
- Eager Loading: Fetches associated entities immediately with the initial query. Use with caution as it may lead to performance issues if overused.
@OneToMany(fetch = FetchType.EAGER, mappedBy = "customer")
private Set<Order> orders;
- Batch Fetching: Retrieves multiple entities in a single query, reducing the number of database round-trips.
@BatchSize(size = 10)
@OneToMany(fetch = FetchType.LAZY, mappedBy = "customer")
private Set<Order> orders;
Best Practices and Considerations for the coding wizard in you 🧙
Optimizing Performance
Use Indexes: Ensure that database indexes are applied to columns frequently used in queries to improve performance.
Optimize Fetch Strategies: Choose between eager and lazy loading based on the specific needs of your application. Avoid eager loading for collections that are not always needed.
Monitor SQL Queries: Use logging and profiling tools to monitor SQL queries generated by JPA. Optimize queries to reduce overhead and improve response times.
Managing Transactions Effectively
Define Transaction Boundaries Clearly: Ensure that transaction boundaries are well-defined to avoid partial updates and inconsistent states.
Handle Exceptions Gracefully: Ensure that exceptions are properly handled and transactions are rolled back in case of failures to maintain data consistency.
Use Appropriate Isolation Levels: Choose isolation levels that match your application's requirements for consistency and concurrency control.
Efficient Data Access
Batch Processing: Use batch processing techniques to handle large volumes of data efficiently. This reduces the number of database interactions and improves performance.
Caching: Implement caching strategies to reduce the number of database queries and improve response times. Use second-level cache providers like Ehcache or Redis.
Advanced Topics 💻🤖
Customizing Queries
JPA allows for advanced querying using JPQL (Java Persistence Query Language) and Criteria API:
JPQL: A powerful query language for JPA entities that resembles SQL but operates on entities and their attributes.
@Query("SELECT u FROM User u WHERE u.email = :email") User findByEmail(@Param("email") String email);
Criteria API: A type-safe way to create queries programmatically.
CriteriaBuilder cb = entityManager.getCriteriaBuilder(); CriteriaQuery<User> query = cb.createQuery(User.class); Root<User> user = query.from(User.class); query.select(user).where(cb.equal(user.get("email"), email)); TypedQuery<User> typedQuery = entityManager.createQuery(query); User result = typedQuery.getSingleResult();
Database Schema Management
Managing the database schema efficiently is crucial for application development:
Schema Generation: Use JPA properties to control schema generation during development.
spring.jpa.hibernate.ddl-auto=update
Database Migration: Use tools like Flyway or Liquibase for versioned database migrations, ensuring that schema changes are applied consistently across different environments.
Handling Large Datasets
When working with large datasets, consider the following strategies:
Pagination: Use pagination to retrieve data in chunks, reducing memory consumption and improving performance.
@Query("SELECT u FROM User u") List<User> findUsers(Pageable pageable);
Streaming: For extremely large result sets, use streaming to process data without loading everything into memory.
@Query("SELECT u FROM User u") Stream<User> streamAllUsers();
Integration with Other Frameworks
JPA can be integrated with various other frameworks and tools to enhance functionality:
Spring Data JPA: Provides additional features and abstractions for working with JPA, simplifying data access and repository management.
Hibernate: A popular JPA implementation that offers advanced features like caching, lazy loading, and query optimization.
Microservices: Use JPA in microservices architectures, ensuring proper transaction management and data consistency across services.
On the ending note,
Please feel free to share you thoughts on what topic should I bring on blogs on next. I am just trying to learn everyday and put out valuable content.
Vivek Tak
Subscribe to my newsletter
Read articles from Vivek Tak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
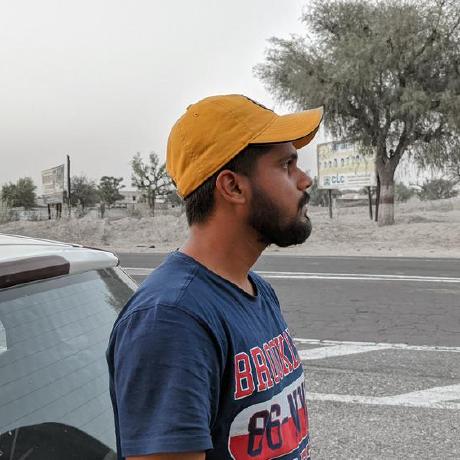
Vivek Tak
Vivek Tak
I am a developer from India. I like to learn everyday about new technologies. Interest Areas: AI, FinTech I am actively looking for learning Golang and DevOps