Exploring Spring Data: An In-Depth Guide
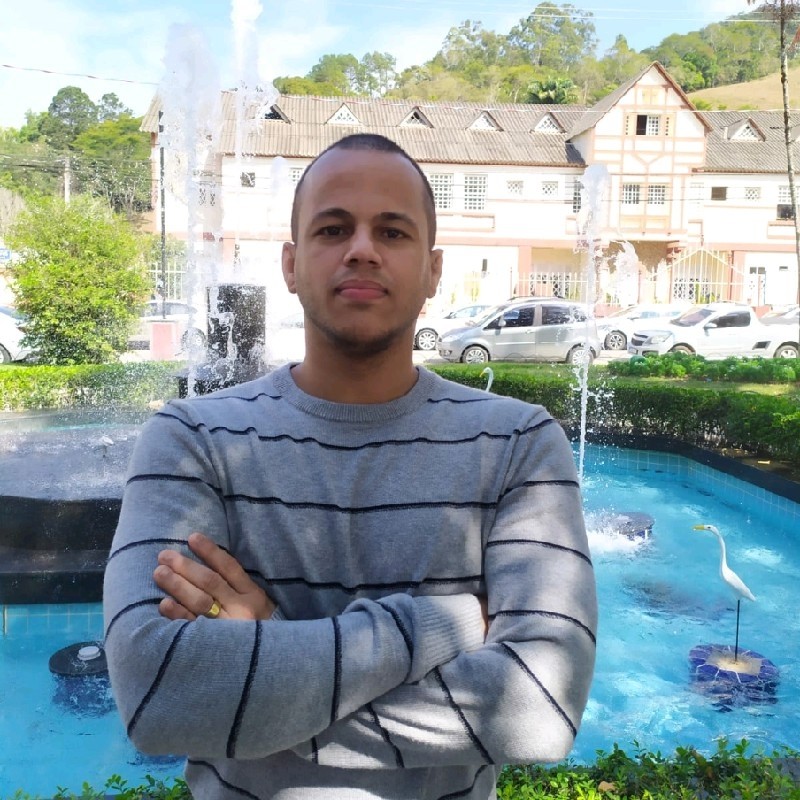
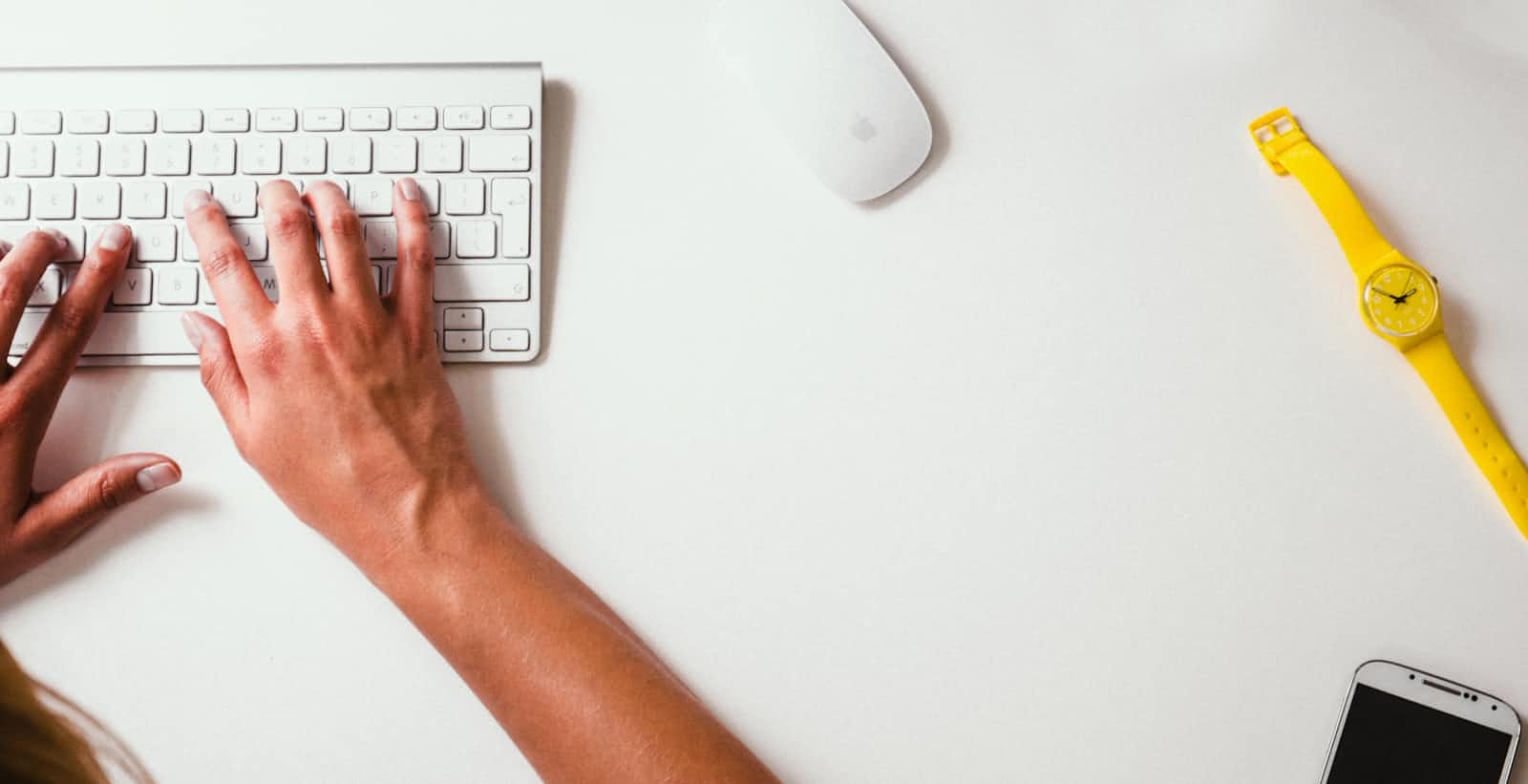
Introduction to Spring Data
Spring Data is a part of the larger Spring Framework that simplifies the interaction with databases. It provides a consistent, Spring-based programming model for data access, while still retaining the special traits of the underlying data store. Spring Data's goal is to significantly reduce the amount of boilerplate code required for database operations, thereby making data access easy, consistent, and more robust.
Key Annotations in Spring Data
Spring Data comes with several annotations that make database operations straightforward and efficient. Here are some of the most important ones:
@Repository
The @Repository
annotation is a specialization of the @Component
annotation, indicating that the class is a Data Access Object (DAO). This annotation translates data access-related exceptions into Spring's DataAccessException
.
Detailed Explanation:
Purpose: Indicates that the class is a repository, which will be used for CRUD operations.
Exception Translation: Automatically translates persistence exceptions into Spring's unified
DataAccessException
.
Example:
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
// Custom query methods can be defined here
}
@Query
The @Query
annotation is used to define custom queries using JPQL or SQL.
Detailed Explanation:
JPQL: Java Persistence Query Language, which is database-independent.
Native Query: Allows the use of native SQL queries for complex operations.
Example:
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT u FROM User u WHERE u.email = ?1")
User findByEmail(String email);
@Query(value = "SELECT * FROM users WHERE email = ?1", nativeQuery = true)
User findByEmailNative(String email);
}
@Modifying
The @Modifying
annotation is used in conjunction with @Query
for update and delete operations.
Detailed Explanation:
Purpose: Indicates that the query method modifies the database (update or delete).
Transactional: Often used with
@Transactional
to ensure atomicity.
Example:
public interface UserRepository extends JpaRepository<User, Long> {
@Modifying
@Query("UPDATE User u SET u.status = ?2 WHERE u.id = ?1")
int updateUserStatus(Long id, String status);
}
@Transactional
The @Transactional
annotation ensures that the method or class is executed within a transactional context.
Detailed Explanation:
Purpose: Manages transactions to ensure data integrity.
Propagation: Defines how transactions are propagated (e.g., REQUIRED, REQUIRES_NEW).
Example:
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public void updateUserStatus(Long id, String status) {
userRepository.updateUserStatus(id, status);
}
}
@EnableJpaRepositories
The @EnableJpaRepositories
annotation is used to enable JPA repositories. It is typically added to a configuration class.
Detailed Explanation:
Purpose: Scans the specified package for repository interfaces.
Configuration: Specifies base packages and other configurations.
Example:
@Configuration
@EnableJpaRepositories(basePackages = "com.example.repository")
public class JpaConfig {
// Additional JPA configuration
}
How Spring Data Works Behind the Scenes
Spring Data abstracts the data access layer, allowing developers to focus on business logic rather than boilerplate code. Here's how it works:
Repository Interfaces: You define repository interfaces that extend Spring Data interfaces like
JpaRepository
,CrudRepository
, etc.Dynamic Proxy: Spring generates a dynamic proxy that implements the repository interface and provides the implementation at runtime.
Query Derivation: Spring Data derives queries from method names defined in the repository interface.
Custom Queries: For more complex queries, you can use the
@Query
annotation.
Integration with Hibernate
Spring Data integrates seamlessly with Hibernate, a popular ORM framework. Here's how the integration works:
Configuration: Configure the
EntityManagerFactory
andTransactionManager
beans.Repositories: Define repository interfaces that extend
JpaRepository
.Entity Mapping: Use JPA annotations (
@Entity
,@Table
,@Id
, etc.) to map Java classes to database tables.Transactions: Manage transactions using
@Transactional
.
Examples
Simple Examples
Example 1: Basic CRUD Operations
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// Getters and setters
}
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public User saveUser(User user) {
return userRepository.save(user);
}
public User getUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
}
Example 2: Custom Query
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT u FROM User u WHERE u.email = :email")
User findByEmail(@Param("email") String email);
}
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public User getUserByEmail(String email) {
return userRepository.findByEmail(email);
}
}
Complex Examples
Example 1: Pagination and Sorting
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT u FROM User u WHERE u.status = :status")
Page<User> findByStatus(@Param("status") String status, Pageable pageable);
}
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public Page<User> getUsersByStatus(String status, int page, int size) {
Pageable pageable = PageRequest.of(page, size, Sort.by("name").ascending());
return userRepository.findByStatus(status, pageable);
}
}
Example 2: Transactional Service with Multiple Repositories
@Entity
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String product;
private int quantity;
// Getters and setters
}
@Repository
public interface OrderRepository extends JpaRepository<Order, Long> {
}
@Entity
public class Customer {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// Getters and setters
}
@Repository
public interface CustomerRepository extends JpaRepository<Customer, Long> {
}
@Service
public class OrderService {
@Autowired
private OrderRepository orderRepository;
@Autowired
private CustomerRepository customerRepository;
@Transactional
public void placeOrder(Order order, Long customerId) {
Customer customer = customerRepository.findById(customerId).orElseThrow();
// Business logic
orderRepository.save(order);
// More business logic
}
}
Conclusion
Spring Data simplifies data access in Spring applications, reducing boilerplate code and providing a consistent programming model. By leveraging its powerful annotations and integrating seamlessly with Hibernate, developers can focus more on business logic and less on plumbing code. This article covered the main annotations, how Spring Data works behind the scenes, its integration with Hibernate, and provided simple and complex examples to illustrate its capabilities.
Spring Data is an indispensable tool for any Spring developer, making database operations straightforward and efficient. Whether you're building a simple application or a complex enterprise system, Spring Data has the features and flexibility to meet your needs.
Subscribe to my newsletter
Read articles from André Felipe Costa Bento directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
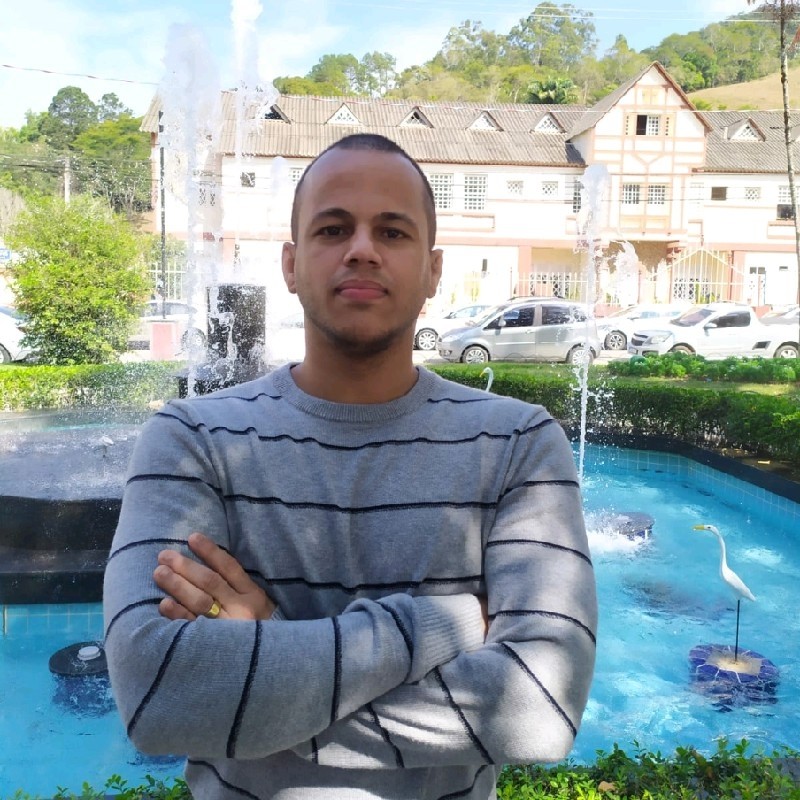
André Felipe Costa Bento
André Felipe Costa Bento
Fullstack Software Engineer.