Emotion Detection Using Convolutional and Recurrent Neural Networks
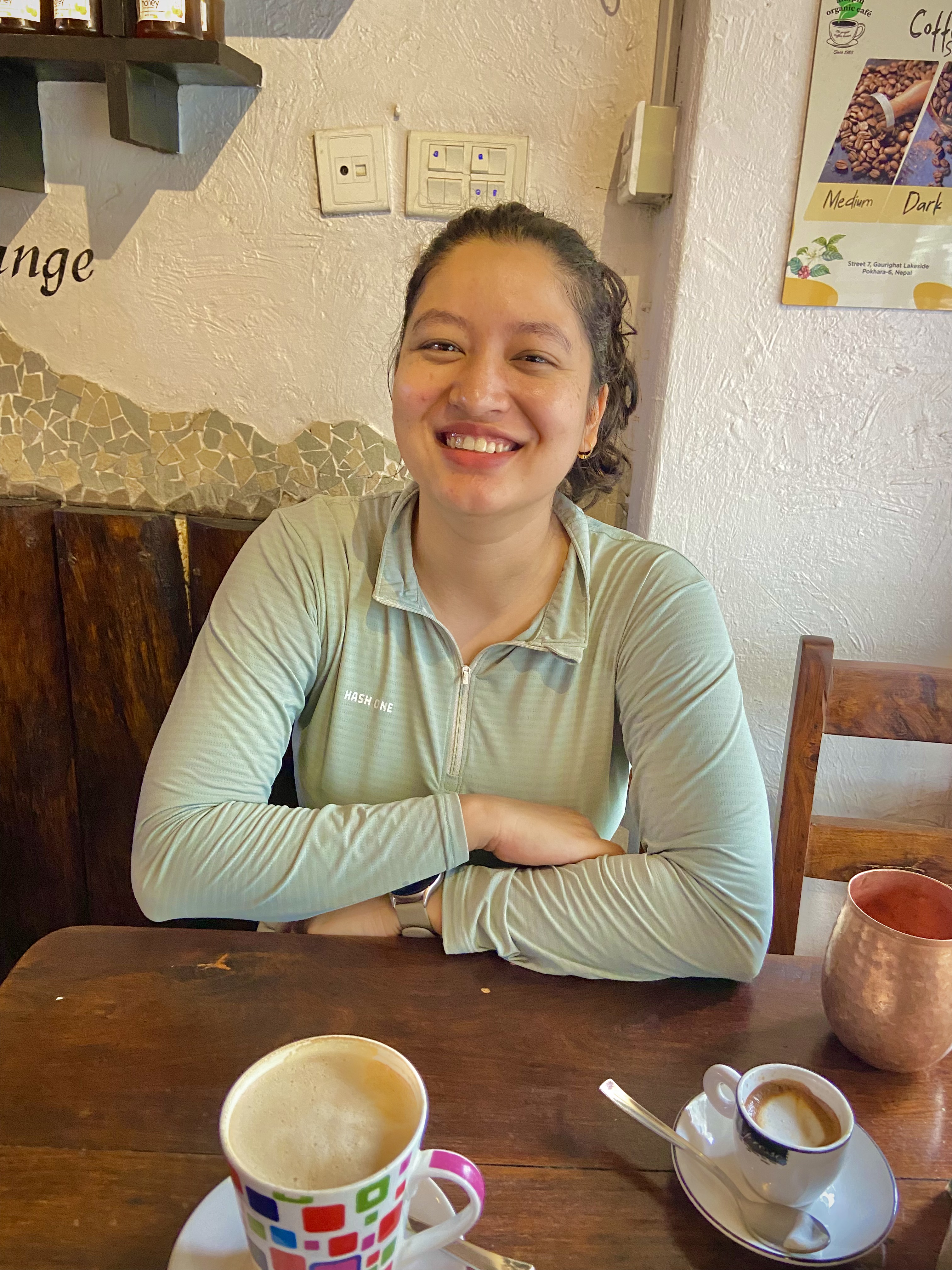
Table of contents
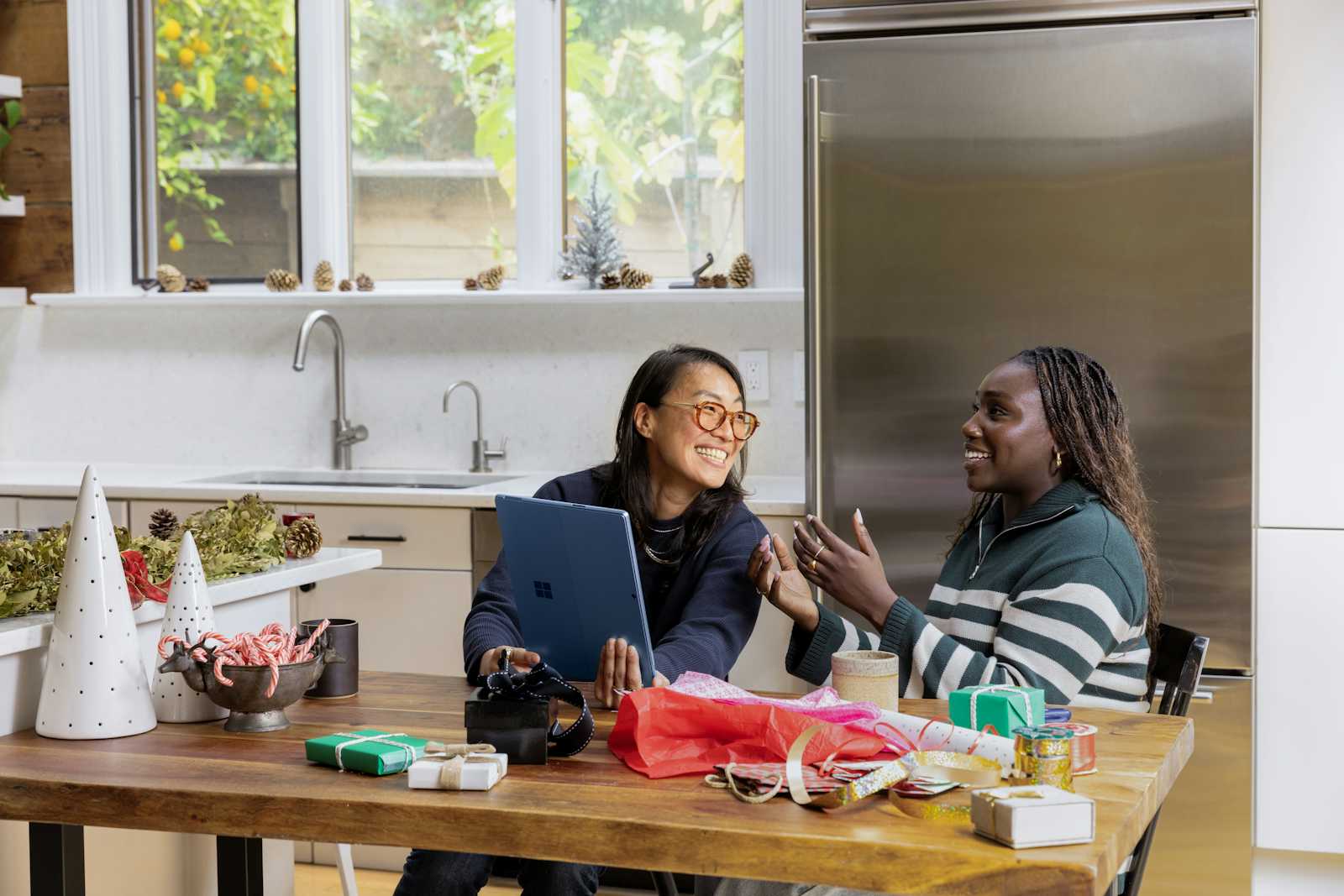
Introduction
Emotion detection from speech and text is an important problem in affective computing with applications in human-computer interaction, sentiment analysis, mental health monitoring, and more. In recent years, deep learning approaches using convolutional neural networks (CNNs) and recurrent neural networks (RNNs) like long short-term memory (LSTM) networks have shown great promise for this task. This article provides an in-depth look at how CNNs and RNNs/LSTMs can be used for emotion detection, including the theory behind these models, practical implementation details, and examples of state-of-the-art architectures.
Overview of Emotion Detection
Emotion detection aims to automatically identify the emotional state of a person from their speech, facial expressions, text, or other modalities. The most common approach is to classify emotions into discrete categories like happy, sad, angry, etc. Some systems also aim to detect emotion intensity or emotional dimensions like valence and arousal.
The main steps in an emotion detection pipeline are:
Data collection and preprocessing
Feature extraction
Model training and evaluation
Inference on new data
For speech-based emotion detection, the raw audio waveform is typically preprocessed to extract acoustic features like mel-frequency cepstral coefficients (MFCCs), pitch, energy, etc. For text, the raw text is tokenized and encoded.
Traditional machine learning approaches used hand-crafted features and classifiers like support vector machines. Deep learning methods can learn features automatically from raw data or low-level features.
Convolutional Neural Networks for Emotion Detection
CNNs have proven very effective for emotion detection, especially from speech and images. They can automatically learn hierarchical features from the input data.
Architecture
A typical CNN architecture for emotion detection consists of:
Input layer
Multiple convolutional layers
Pooling layers
Fully connected layers
Output layer
For speech emotion detection, the input is usually a spectrogram or other time-frequency representation. For text, it could be word embeddings.
The convolutional layers apply filters to extract features. Early layers capture low-level features while deeper layers learn more abstract representations. Pooling layers downsample the feature maps. The fully connected layers combine the learned features for classification.
Here's a simple CNN architecture for speech emotion detection:
model = Sequential([
Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(128, 128, 1)),
MaxPooling2D(pool_size=(2, 2)),
Conv2D(64, kernel_size=(3, 3), activation='relu'),
MaxPooling2D(pool_size=(2, 2)),
Conv2D(128, kernel_size=(3, 3), activation='relu'),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dense(num_emotions, activation='softmax')
])
This model takes a 128x128 spectrogram as input and outputs probabilities for each emotion class.
Feature Learning
The key advantage of CNNs is their ability to automatically learn useful features from raw data. The convolutional layers learn filters that activate for different patterns in the input.
For speech, early layers may learn to detect simple acoustic patterns like onsets or pitch changes. Deeper layers combine these to recognize more complex patterns associated with different emotions.
For text, the convolutional filters can capture n-gram patterns and local context information relevant for emotion.
Advantages
Some key advantages of CNNs for emotion detection:
Automatic feature learning
Capture local patterns and context
Translation invariance
Parameter sharing reduces overfitting
CNNs work particularly well for speech and image data where local patterns are important. They can capture spectro-temporal patterns in speech that are indicative of emotions.
Recurrent Neural Networks for Emotion Detection
RNNs are designed to work with sequential data, making them well-suited for speech and text emotion detection. They can capture long-range dependencies and context.
Architecture
A basic RNN processes the input sequence step-by-step, maintaining a hidden state that is updated at each step. However, basic RNNs suffer from the vanishing gradient problem.
Long Short-Term Memory (LSTM) networks solve this by introducing gating mechanisms to control information flow. A typical LSTM architecture for emotion detection includes:
Input layer
Embedding layer (for text)
One or more LSTM layers
Fully connected layer(s)
Output layer
Here's an example LSTM model for text emotion detection:
model = Sequential([
Embedding(vocab_size, 100, input_length=max_length),
LSTM(128, return_sequences=True),
LSTM(64),
Dense(64, activation='relu'),
Dense(num_emotions, activation='softmax')
])
This model takes a sequence of word indices as input, embeds them, passes them through two LSTM layers, and outputs emotion probabilities.
Sequential Processing
The key feature of RNNs/LSTMs is their ability to process sequential data. At each time step, the model takes the current input and the previous hidden state to produce an output and update the hidden state.
This allows the model to maintain context information over long sequences. For emotion detection, this is crucial for capturing the emotional trajectory over time in speech or text.
Advantages
Some key advantages of RNNs/LSTMs for emotion detection:
Can handle variable-length sequences
Capture long-range dependencies and context
Maintain temporal information
Well-suited for time-series data like speech
RNNs excel at tasks where the order and context of the input are important. They can model the dynamics of emotional expression over time.
Combining CNNs and RNNs
Many state-of-the-art emotion detection models combine CNNs and RNNs to leverage the strengths of both architectures. Some common approaches:
CNN-LSTM: Use CNN layers to extract features, followed by LSTM layers to model temporal dynamics.
Parallel CNN-LSTM: Apply CNN and LSTM in parallel and combine their outputs.
ConvLSTM: Use convolutional operations within the LSTM cell.
Here's an example of a CNN-LSTM model for speech emotion detection:
input = Input(shape=(128, 128, 1))
x = Conv2D(32, kernel_size=(3, 3), activation='relu')(input)
x = MaxPooling2D(pool_size=(2, 2))(x)
x = Conv2D(64, kernel_size=(3, 3), activation='relu')(x)
x = MaxPooling2D(pool_size=(2, 2))(x)
x = Conv2D(128, kernel_size=(3, 3), activation='relu')(x)
x = MaxPooling2D(pool_size=(2, 2))(x)
x = Reshape((-1, 128))(x)
x = LSTM(64, return_sequences=True)(x)
x = LSTM(32)(x)
output = Dense(num_emotions, activation='softmax')(x)
model = Model(inputs=input, outputs=output)
This model applies CNN layers to extract features from the spectrogram, reshapes the output, and passes it through LSTM layers to model temporal dynamics.
Data Preprocessing
Proper data preprocessing is crucial for effective emotion detection. The exact preprocessing steps depend on the input modality and model architecture.
Speech Preprocessing
For speech emotion detection, common preprocessing steps include:
Resampling to a consistent sample rate (e.g. 16 kHz)
Silence removal
Voice activity detection
Normalization
Framing (splitting into short frames, e.g. 25ms)
Windowing (applying window function to frames)
Feature extraction (e.g. computing spectrograms or MFCCs)
Here's an example of preprocessing speech data to compute spectrograms:
import librosa
import numpy as np
def preprocess_audio(file_path, sr=16000, n_mels=128, n_fft=2048, hop_length=512):
# Load audio file
y, sr = librosa.load(file_path, sr=sr)
# Compute mel spectrogram
S = librosa.feature.melspectrogram(y=y, sr=sr, n_mels=n_mels,
n_fft=n_fft, hop_length=hop_length)
# Convert to log scale
S_db = librosa.power_to_db(S, ref=np.max)
return S_db
# Preprocess all audio files
spectrograms = [preprocess_audio(file) for file in audio_files]
# Pad/trim to fixed length
max_len = max(spec.shape[1] for spec in spectrograms)
spectrograms_padded = [librosa.util.fix_length(spec, size=max_len, axis=1)
for spec in spectrograms]
# Convert to numpy array
X = np.array(spectrograms_padded)[:, :, :, np.newaxis]
This code loads each audio file, computes its mel spectrogram, converts to log scale, pads all spectrograms to the same length, and stacks them into a 4D numpy array suitable for input to a CNN.
Text Preprocessing
For text emotion detection, common preprocessing steps include:
Lowercasing
Removing punctuation and special characters
Tokenization
Removing stop words
Stemming or lemmatization
Encoding (e.g. converting to word indices)
Here's an example of text preprocessing:
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
from nltk.stem import PorterStemmer
nltk.download('punkt')
nltk.download('stopwords')
def preprocess_text(text):
# Lowercase
text = text.lower()
# Remove punctuation
text = ''.join([char for char in text if char.isalnum() or char.isspace()])
# Tokenize
tokens = word_tokenize(text)
# Remove stop words
stop_words = set(stopwords.words('english'))
tokens = [token for token in tokens if token not in stop_words]
# Stemming
stemmer = PorterStemmer()
tokens = [stemmer.stem(token) for token in tokens]
return tokens
# Preprocess all texts
preprocessed_texts = [preprocess_text(text) for text in texts]
# Create vocabulary
vocab = set(token for text in preprocessed_texts for token in text)
word_to_index = {word: i+1 for i, word in enumerate(vocab)}
# Convert to word indices
X = [[word_to_index[token] for token in text] for text in preprocessed_texts]
# Pad sequences
from keras.preprocessing.sequence import pad_sequences
X_padded = pad_sequences(X, maxlen=max_length)
This code tokenizes each text, removes stop words, applies stemming, converts to word indices based on a vocabulary, and pads all sequences to the same length.
Model Training
Training deep learning models for emotion detection involves several key considerations:
Loss Function
For multi-class emotion classification, categorical cross-entropy is typically used as the loss function. For multi-label classification (where multiple emotions can be present simultaneously), binary cross-entropy is used.
For regression tasks (e.g. predicting emotion intensity or valence/arousal values), mean squared error or mean absolute error can be used.
Optimization
Stochastic gradient descent (SGD) or adaptive optimizers like Adam are commonly used. Learning rate scheduling can help improve convergence.
Regularization
To prevent overfitting, techniques like dropout, L2 regularization, and early stopping are often employed.
Data Augmentation
Data augmentation can help improve generalization, especially when training data is limited. For speech, techniques like adding noise, changing speed/pitch, and time stretching can be used. For text, techniques like synonym replacement and back-translation can be effective.
Here's an example of training a CNN model for speech emotion detection:
from keras.callbacks import EarlyStopping, ReduceLROnPlateau
# Define model (see CNN architecture above)
model = ...
# Compile model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Define callbacks
early_stopping = EarlyStopping(monitor='val_loss', patience=10, restore_best_weights=True)
lr_scheduler = ReduceLROnPlateau(monitor='val_loss', factor=0.5, patience=5)
# Train model
history = model.fit(X_train, y_train,
validation_data=(X_val, y_val),
epochs=100,
batch_size=32,
callbacks=[early_stopping, lr_scheduler])
# Evaluate on test set
test_loss, test_acc = model.evaluate(X_test, y_test)
print(f"Test accuracy: {test_acc}")
This code compiles the model with the Adam optimizer and categorical cross-entropy loss, defines callbacks for early stopping and learning rate scheduling, trains the model on the training data while monitoring validation loss, and finally evaluates on the test set.
Evaluation Metrics
Common evaluation metrics for emotion detection include:
Accuracy: Proportion of correctly classified samples
Precision, Recall, F1-score: Useful for imbalanced datasets
Confusion matrix: Shows misclassifications between emotion classes
Mean Absolute Error (MAE): For regression tasks
Concordance Correlation Coefficient (CCC): For dimensional emotion recognition
For multi-class classification, it's important to look at per-class metrics as well as overall metrics, as performance may vary significantly between emotion classes.
Here's an example of computing evaluation metrics:
from sklearn.metrics import classification_report, confusion_matrix
import seaborn as sns
import matplotlib.pyplot as plt
# Get predictions
y_pred = model.predict(X_test)
y_pred_classes = np.argmax(y_pred, axis=1)
y_true_classes = np.argmax(y_test, axis=1)
# Print classification report
print(classification_report(y_true_classes, y_pred_classes, target_names=emotion_labels))
# Plot confusion matrix
cm = confusion_matrix(y_true_classes, y_pred_classes)
plt.figure(figsize=(10,8))
sns.heatmap(cm, annot=True, fmt='d', cmap='Blues', xticklabels=emotion_labels, yticklabels=emotion_labels)
plt.xlabel('Predicted')
plt.ylabel('True')
plt.show()
This code generates predictions on the test set, computes per-class precision, recall, and F1-score, and plots a confusion matrix to visualize misclassifications between emotion classes.
State-of-the-Art Architectures
Several advanced architectures have been proposed for emotion detection in recent years. Here are a few notable examples:
- Attention-based Models
Attention mechanisms allow models to focus on the most relevant parts of the input for emotion detection. For example, self-attention can help capture long-range dependencies in text or speech.
An example of an attention-based model for text emotion detection:
from keras.layers import Input, Embedding, Bidirectional, LSTM, Dense, Dropout, Attention
def attention_model(vocab_size, max_length, embedding_dim, num_emotions):
inputs = Input(shape=(max_length,))
embedding = Embedding(vocab_size, embedding_dim, input_length=max_length)(inputs)
lstm = Bidirectional(LSTM(128, return_sequences=True))(embedding)
attention = Attention()([lstm, lstm])
lstm_attention = Bidirectional(LSTM(64))(attention)
dropout = Dropout(0.5)(lstm_attention)
outputs = Dense(num_emotions, activation='softmax')(dropout)
model = Model(inputs=inputs, outputs=outputs)
return model
This model uses a bidirectional LSTM with self-attention to capture important words or phrases for emotion detection.
- Transfer Learning
Transfer learning from pre-trained models has shown great success in emotion detection. For speech, models pre-trained on large speech recognition datasets can be fine-tuned for emotion detection. For text, language models like BERT can be used.
Example of fine-tuning BERT for text emotion detection:
from transformers import BertTokenizer, TFBertForSequenceClassification
# Load pre-trained BERT model and tokenizer
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
model = TFBertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=num_emotions)
# Tokenize and encode the text data
encodings = tokenizer(texts, truncation=True, padding=True, max_length=128)
# Convert to TensorFlow dataset
import tensorflow as tf
dataset = tf.data.Dataset.from_tensor_slices((
dict(encodings),
tf.keras.utils.to_categorical(labels, num_classes=num_emotions)
))
# Fine-tune the model
model.compile(optimizer=tf.keras.optimizers.Adam(learning_rate=5e-5),
loss=tf.keras.losses.CategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
model.fit(dataset.shuffle(1000).batch(16), epochs=3)
This code loads a pre-trained BERT model, tokenizes the input texts, and fine-tunes the model on the emotion detection task.
- Multimodal Models
Combining multiple modalities (e.g., speech and text) can improve emotion detection performance. These models typically use separate networks for each modality and then fuse the features.
Here's a simplified example of a multimodal model combining speech and text:
from keras.layers import Input, Conv2D, MaxPooling2D, LSTM, Dense, Concatenate
def multimodal_model(speech_input_shape, text_input_shape, num_emotions):
# Speech branch
speech_input = Input(shape=speech_input_shape)
x = Conv2D(32, kernel_size=(3, 3), activation='relu')(speech_input)
x = MaxPooling2D(pool_size=(2, 2))(x)
x = Conv2D(64, kernel_size=(3, 3), activation='relu')(x)
x = MaxPooling2D(pool_size=(2, 2))(x)
x = Flatten()(x)
speech_features = Dense(128, activation='relu')(x)
# Text branch
text_input = Input(shape=text_input_shape)
y = Embedding(vocab_size, 100, input_length=text_input_shape[0])(text_input)
y = LSTM(128)(y)
text_features = Dense(128, activation='relu')(y)
# Fusion
combined = Concatenate()([speech_features, text_features])
z = Dense(128, activation='relu')(combined)
output = Dense(num_emotions, activation='softmax')(z)
model = Model(inputs=[speech_input, text_input], outputs=output)
return model
This model processes speech and text inputs separately and then concatenates the features before final classification.
- Ensemble Methods
Ensemble methods combine predictions from multiple models to improve overall performance. This can be particularly effective for emotion detection, where different models may excel at detecting different emotions.
Here's an example of a simple ensemble:
def ensemble_predict(models, X):
predictions = [model.predict(X) for model in models]
return np.mean(predictions, axis=0)
# Train multiple models
model1 = create_cnn_model()
model2 = create_lstm_model()
model3 = create_attention_model()
models = [model1, model2, model3]
for model in models:
model.fit(X_train, y_train, validation_data=(X_val, y_val), epochs=100)
# Make ensemble prediction
ensemble_pred = ensemble_predict(models, X_test)
This code trains multiple models with different architectures and then averages their predictions.
Challenges and Future Directions
While deep learning has significantly advanced the field of emotion detection, several challenges remain:
Data Scarcity: Collecting large-scale, high-quality emotion datasets is challenging and time-consuming. This is particularly true for spontaneous, real-world emotions.
Class Imbalance: Some emotions (e.g., neutral, happy) are typically overrepresented in datasets, while others (e.g., fear, disgust) are underrepresented.
Context Dependency: Emotions are highly context-dependent, and models often struggle to capture contextual information effectively.
Cultural and Individual Differences: Emotional expressions can vary significantly across cultures and individuals, making it challenging to create universal emotion detection models.
Temporal Dynamics: Emotions evolve over time, and capturing these dynamics accurately remains a challenge.
Multimodal Fusion: While multimodal approaches show promise, effectively fusing information from different modalities is still an open problem.
Future research directions to address these challenges include:
Few-shot and Zero-shot Learning: Developing models that can recognize new emotions or adapt to new domains with minimal labeled data.
Continual Learning: Creating models that can continuously learn and adapt to new emotional expressions over time.
Explainable AI: Developing interpretable models that can explain their emotion predictions, which is crucial for applications in healthcare and other sensitive domains.
Cross-cultural Emotion Detection: Building models that can generalize across different cultures and languages.
Personalized Emotion Detection: Developing models that can adapt to individual differences in emotional expression.
Emotion Generation: In addition to detection, generating emotionally expressive speech or text is an emerging area of research.
Conclusion
Convolutional Neural Networks (CNNs) and Recurrent Neural Networks (RNNs), particularly Long Short-Term Memory (LSTM) networks, have revolutionized the field of emotion detection from speech and text. CNNs excel at capturing local patterns and hierarchical features, while RNNs are adept at modeling sequential data and long-term dependencies. Combining these architectures, along with attention mechanisms and transfer learning, has led to state-of-the-art performance on various emotion detection tasks.
The success of these deep learning approaches stems from their ability to automatically learn relevant features from raw data, capturing subtle emotional cues that may be difficult to specify manually. This has enabled more accurate and robust emotion detection systems that can handle the complexity and variability of human emotional expressions.
However, challenges remain, particularly in dealing with data scarcity, class imbalance, and capturing the context-dependent and dynamic nature of emotions. Future research directions, such as few-shot learning, continual learning, and personalized emotion detection, promise to address these challenges and further advance the field.
As emotion detection systems continue to improve, they will enable a wide range of applications, from more empathetic virtual assistants and improved human-computer interaction to advanced mental health monitoring tools and emotionally intelligent robots. The ongoing research in this field will play a crucial role in developing AI systems that can better understand and respond to human emotions, ultimately leading to more natural and effective human-AI interaction.
Subscribe to my newsletter
Read articles from Monika Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
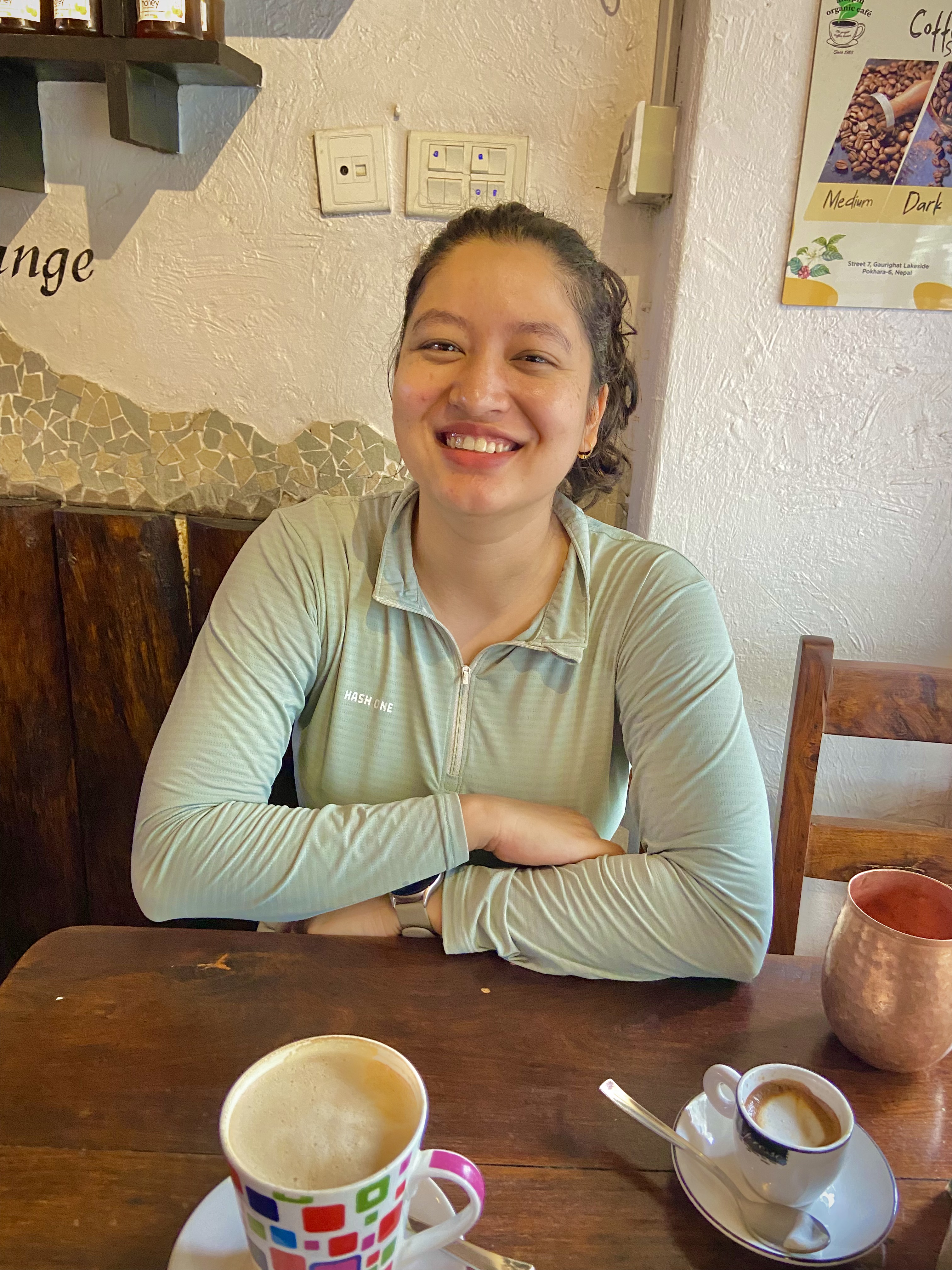