Getting Started with Webpack: A Beginner’s Guide - Part 1
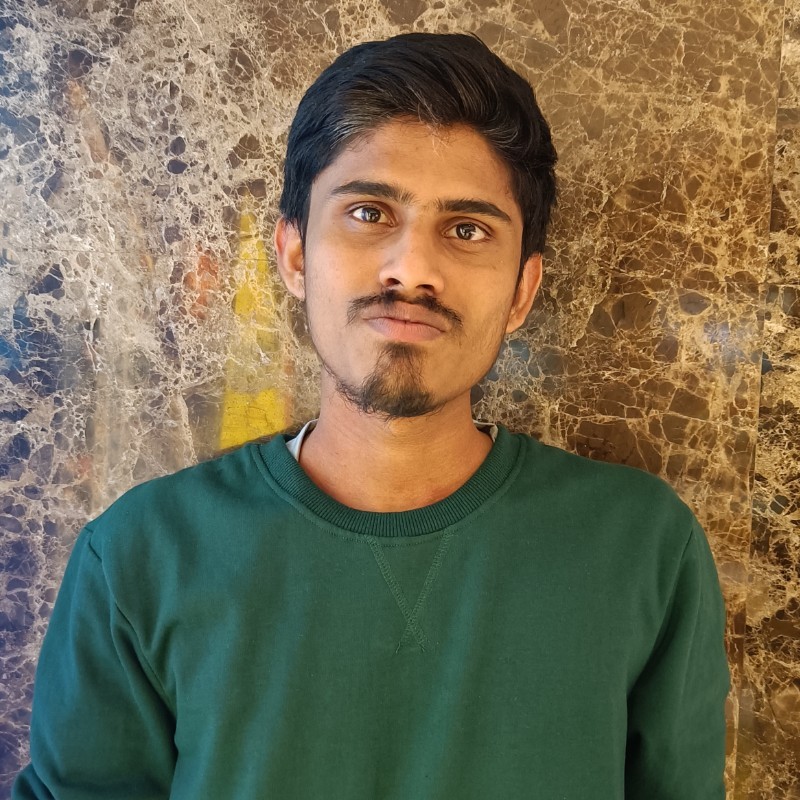
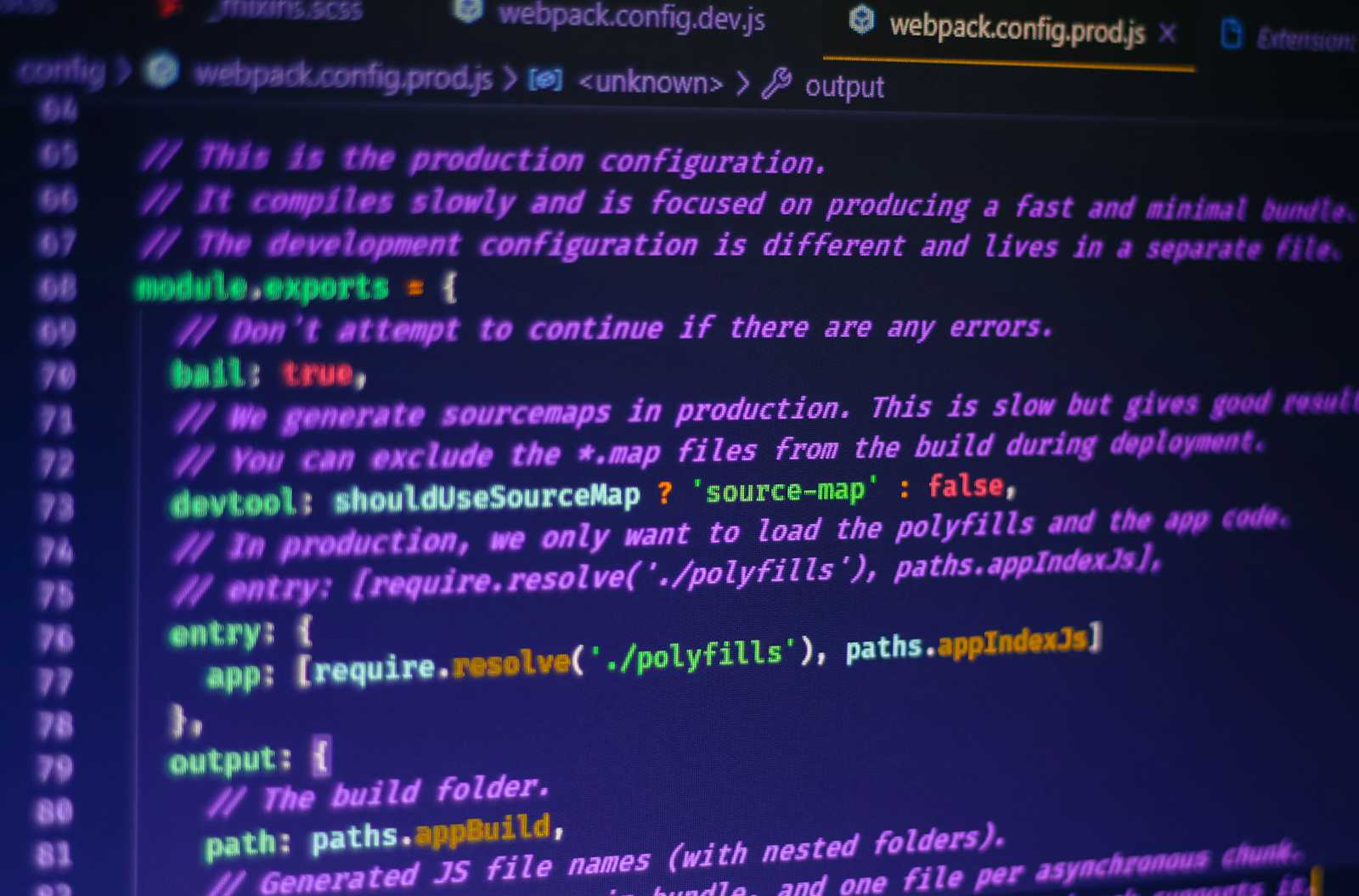
How Webpack Has Evolved
In the early days of web development, websites were pretty simple. You'd only have a few HTML, CSS, and JavaScript files. But as websites grew more complex, with lots of files and dependencies, managing them became tricky. Developers faced challenges like organising files, ensuring they load in the correct order, and reducing their number and size to make websites load faster. Then Webpack came up with a solution.
What is Webpack
Webpack is a handy tool that helps solve these problems. It takes all your scattered files and bundles them together into neat packages. This makes development easier and your website faster.
Let's check with an example.
Example: Without Webpack
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My App</title>
</head>
<body>
<script src="file1.js"></script>
<script src="file2.js"></script>
<script src="file3.js"></script>
</body>
</html>
In this case, each <script>
tag results in a separate network request for each JavaScript file.
This means:
Multiple Network Requests: The browser makes multiple requests to the server to fetch each JavaScript file.
Increased Load Time: Each request adds to the total time it takes to load the page, especially if there are many files.
Potential Performance Issues: More requests can lead to increased latency and more time spent in establishing connections.
Example: With Webpack
Webpack optimizes this process by bundling all your JavaScript files into a single file (or a few files) before serving them to the browser:
- Bundling: Webpack combines all your JavaScript files into a single bundle (or a few chunks), like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My App</title>
</head>
<body>
<script src="bundle.js"></script>
</body>
</html>
Single Network Request: The browser makes just one network request for the bundled
bundle.js
file, reducing the overhead of multiple requests.Improved Performance: By minimizing the number of network requests, you improve the page load time and reduce latency.
Let's deep dive into the core concepts of Webpack, how it works, and how to integrate it into our application.
Core Concepts of Webpack
Modules and module bundling: Think of modules as individual pieces of your project, like JavaScript, CSS, or images. Webpack takes all these pieces and combines them into a few files that are ready to be used by your website.
Entry Points: The entry point is the starting point of Webpack’s work. It’s like the front door of your project. From here, Webpack figures out which files to include and how to bundle them. A simple project might have one entry point, while a larger one might have several.
Output: The output is where Webpack saves the final bundled files. You decide where and how these files should be named and stored.
Loaders: Loaders are tools that transform files into a format that Webpack can understand. For example,
babel-loader
converts modern JavaScript into a format that works in older browsers, whilecss-loader
handles CSS files.Plugins: Plugins add extra features to Webpack. They can help with tasks like optimizing files, managing HTML, or cleaning up old files.
Some of the plugins are
HtmlWebpackPlugin: It helps to create HTML files
MiniCssExtractPlugin: It extracts CSS into separate files rather than including it in JavaScript bundles.
CleanWebpackPlugin: It clears the old build files.
Like this, we have postcss plugins likeautoprefixer
,cssnano
,postcss-pxtorem
, etc., which helps us to process the CSS while bundling. we will look into them when I give an exampleMode: Webpack can work in different modes depending on what you’re doing. In development mode, it focuses on making the build process fast and easy to debug. In production mode, it optimizes everything for better performance and smaller file sizes.
Installation & setup
Installing Webpack and Webpack CLI
npm install --save-dev webpack webpack-cli
Webpack Configuration:
Create a
webpack.config.js
file to define your configuration:const path = require('path'); module.exports = { mode: 'development' entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist') }, };
As I said before,
mode
: Determines the mode of the build (development or production). Development mode is for easy debugging, while production mode optimizes for performance.entry
: The entry point for your application.output
: Defines where and how the output files are saved.module.rules
: Specifies rules for handling different file types.
Run Webpack
We have several ways to run a webpack file. some of them are
using npx command:
npx webpack
using scripts in
package.json
: You can add Webpack commands to thescripts
section of yourpackage.json
file. For example:"scripts": { "build": "webpack" }
Global Installation: Install Webpack globally on your system with
npm install -g webpack
. Then you can run it directlywebpack
from your command line.
In the next part, we will look into some loaders like Babel, PostCSS, and plugins with examples. And how to integrate them with Webpack
References
https://webpack.js.org/concepts/
https://github.com/rajeshpolarati/Webpack-Example-Project
Subscribe to my newsletter
Read articles from Rajesh Polarati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
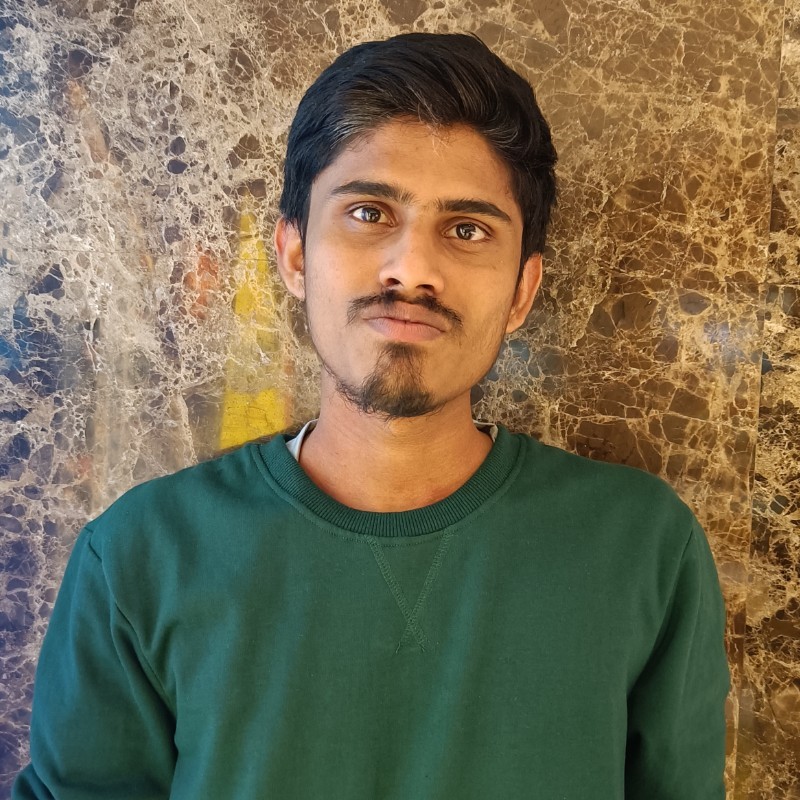
Rajesh Polarati
Rajesh Polarati
Full Stack Developer experienced in creating scalable web applications. Proficient in React, Express.js, Node.js, and SQL, MongoDB, Kafka, DSA. Skilled in team collaboration and passionate about building efficient, responsive solutions