Discover Python's Potential: Key Features and Practical Uses
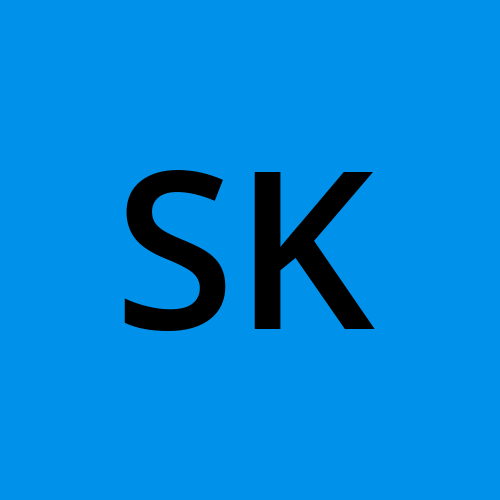
Python has emerged as one of the most popular programming languages due to its simplicity, versatility, and powerful capabilities. In this blog post, we’ll explore some of Python’s key features and demonstrate their practical applications with real-world examples. We'll also delve into some important concepts and best practices for beginners.
1. Simple and Readable Syntax
Python’s syntax is designed to be intuitive and readable, making it easy for beginners to learn and for developers to write and maintain code efficiently.
Example:
# Simple program to calculate the area of a rectangle
length = 5
width = 3
area = length * width
print(f"The area of the rectangle is {area}")
Real-World Application: Python’s readable syntax is ideal for data analysis, where clear and concise code is crucial for handling large datasets and performing complex calculations.
2. Extensive Standard Library
Python comes with a vast standard library that includes modules and packages for various tasks such as web development, data manipulation, and machine learning.
Example:
import os
# List all files and directories in the current directory
files_and_directories = os.listdir('.')
print(files_and_directories)
Real-World Application: The os
module can be used in automation scripts for managing files and directories, making it a powerful tool for system administrators.
3. Dynamic Typing
Python is dynamically typed, meaning that variables do not need to be declared with a specific type, and their type can change at runtime.
Example:
# Dynamic typing in Python
a = 5
print(type(a)) # Output: <class 'int'>
a = 'Hello'
print(type(a)) # Output: <class 'str'>
Real-World Application: Dynamic typing makes Python a flexible and versatile language, particularly useful in rapid prototyping and development where quick iteration is key.
4. Print and Type Functions
The print()
function is used to output data to the screen, and the type()
function is used to check the data type of a variable. These functions are essential for debugging and understanding code behavior.
Example:
# Using print and type functions
a = 10
print(a) # Output: 10
print(type(a)) # Output: <class 'int'>
Real-World Application: The print()
function is used extensively in development for logging and debugging, while the type()
function helps in type checking and debugging.
5. Case Sensitivity in Variable Names
Python is case-sensitive, meaning that variable names with different cases are considered distinct.
Example:
# Case sensitivity in Python
Variable = 10
variable = 20
print(Variable) # Output: 10
print(variable) # Output: 20
Real-World Application: Understanding case sensitivity is crucial to avoid bugs in your code. Using consistent naming conventions can help prevent such issues.
6. Naming Conventions and Identifiers
In Python, variable names should be descriptive and follow certain conventions. Avoid using Python keywords and built-in function names as identifiers.
Example:
# Proper naming conventions
total_price = 100
is_valid = True
# Avoid using keywords or built-in functions
# if = 10 # Incorrect
# print = "Hello" # Incorrect
Real-World Application: Following naming conventions and avoiding reserved words improve code readability and maintainability, making it easier for others (and yourself) to understand and modify your code.
7. Interpreted Language
Python is an interpreted language, meaning that code is executed line by line, which makes debugging easier and allows for rapid development and testing.
Example:
print("Hello, World!")
Real-World Application: The interpreted nature of Python is particularly useful in development environments where code needs to be tested frequently and quickly, such as in scientific computing and data analysis.
8. Large Community and Active Development
Python has a large and active community of developers who contribute to a wide range of libraries and frameworks, ensuring continuous improvement and support.
Real-World Application: The large community provides extensive resources, tutorials, and forums, making it easier for developers to find solutions and collaborate on projects. Popular platforms like Stack Overflow and GitHub are filled with Python-related discussions and projects.
9. Integration Capabilities
Python can easily integrate with other languages and technologies, making it a versatile choice for a wide range of applications.
Example:
import subprocess
# Run a shell command from Python
subprocess.run(['ls', '-l'])
Real-World Application: Python’s integration capabilities allow it to be used in diverse environments, from web development (integrating with JavaScript) to scientific computing (interfacing with C/C++ libraries).
10. Interactive Mode
Python’s interactive mode allows developers to test snippets of code quickly, making it a great tool for learning and experimentation.
Example:
>>> a = 10
>>> b = 20
>>> a + b
30
Real-World Application: Interactive mode is beneficial for data analysis and educational purposes, where immediate feedback is crucial for understanding and experimenting with new concepts.
11. Exception Handling
Python provides robust exception-handling capabilities, allowing developers to write code that can gracefully handle errors and unexpected situations.
Example:
try:
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
Real-World Application: Exception handling is essential for building reliable applications, from web servers that need to handle various user inputs to data processing pipelines that must manage diverse datasets.
Static vs Dynamic Typing
Static typing requires explicit declaration of variable types, whereas dynamic typing allows variables to change types at runtime.
Example of Static Typing (in a statically typed language like Java):
int a = 5;
String b = "Hello";
Example of Dynamic Typing (in Python):
a = 5
print(type(a)) # Output: <class 'int'>
a = 'Hello'
print(type(a)) # Output: <class 'str'>
Real-World Application: Dynamic typing in Python makes it easier to write flexible and quick-to-develop code, especially useful in data analysis and rapid prototyping. However, static typing (used in languages like Java) provides more type safety and can prevent certain types of bugs.
Conclusion
Python's simplicity, versatility, and powerful features make it an excellent choice for both beginners and experienced developers. Whether you’re building a web application, analyzing data, or automating tasks, Python has the tools and libraries to help you succeed. Start exploring Python today and unlock the potential of this incredible language!
Subscribe to my newsletter
Read articles from Sandhya Kondmare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
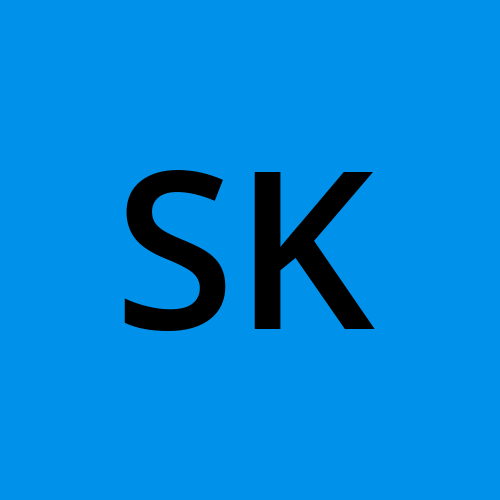
Sandhya Kondmare
Sandhya Kondmare
Aspiring DevOps Engineer with 2 years of hands-on experience in designing, implementing, and managing AWS infrastructure. Proven expertise in Terraform for infrastructure as code, automation tools, and scripting languages. Adept at collaborating with development and security teams to create scalable and secure architectures. Hands-on in AWS, GCP, Azure and Terraform best practices.