Virtual DOM

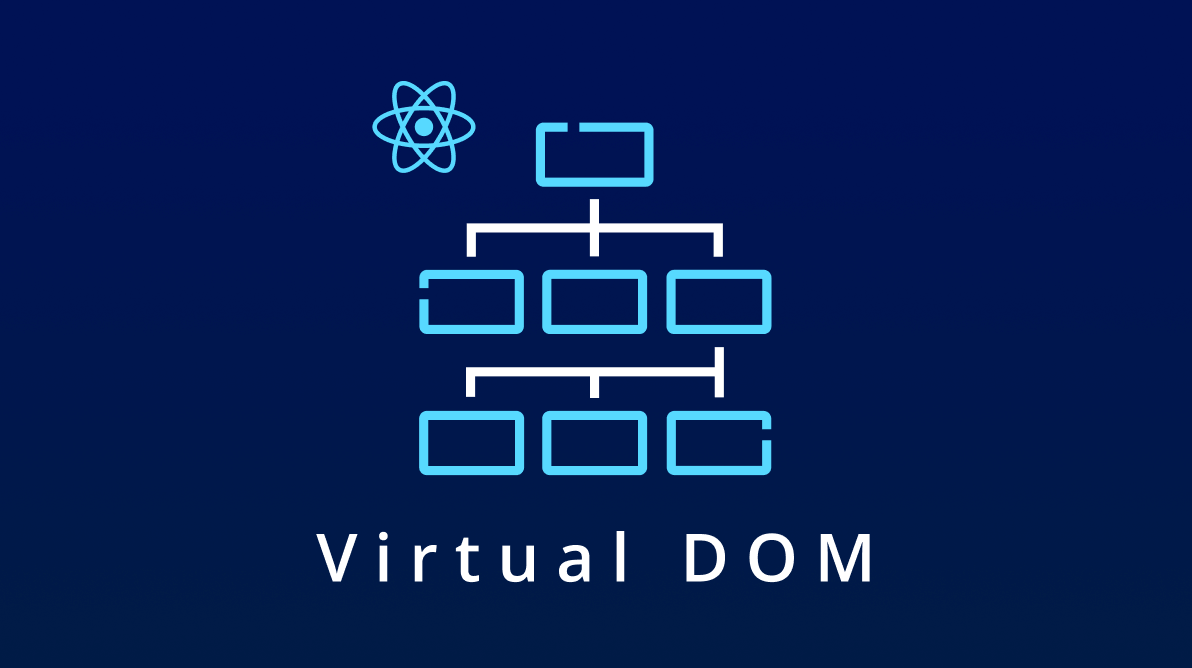
What is Virtual DOM:
The Virtual DOM is a blueprint of the Real DOM.
In React, for every DOM object, there is a corresponding Virtual DOM object.
A Virtual DOM object is a representation of a DOM object, like a lightweight copy.
A Virtual DOM object has the same properties as a Real DOM object, but it lacks the ability to directly change what's on screen.
Manipulating the Real DOM is slow, whereas manipulating the Virtual DOM is much faster.
The Virtual DOM cannot update HTML directly on the webpage, whereas the Real DOM can update HTML directly.
The memory is efficiently utilized in the Virtual DOM, whereas in the Real DOM, memory usage is less efficient.
Imagine you have a simple React component that displays a list of items:
import React, { useState } from 'react';
const ItemList = () => {
const [items, setItems] = useState(['Item 1', 'Item 2', 'Item 3']);
const addItem = () => {
setItems([...items, `Item ${items.length + 1}`]);
};
return (
<div>
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
<button onClick={addItem}>Add Item</button>
</div>
);
};
export default ItemList;
Virtual DOM Example Explanation:
Initial Rendering:
When the
ItemList
component is first rendered, React creates a Virtual DOM representation of the structure:<div> <ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> <button>Add Item</button> </div>
State Change:
When you click the "Add Item" button, the
addItem
function updates the state by adding a new item to the list.React creates a new Virtual DOM to represent the updated structure:
<div> <ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> <li>Item 4</li> </ul> <button>Add Item</button> </div>
Diffing and Patching:
React compares the new Virtual DOM with the previous one (this process is called "diffing").
React identifies that only the list item has changed.
React updates the Real DOM with the new item efficiently, without re-rendering the entire list.
Subscribe to my newsletter
Read articles from Nitin Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
