๐ Master Data Validation with Zod: A TypeScript-First Approach to Schema Declaration

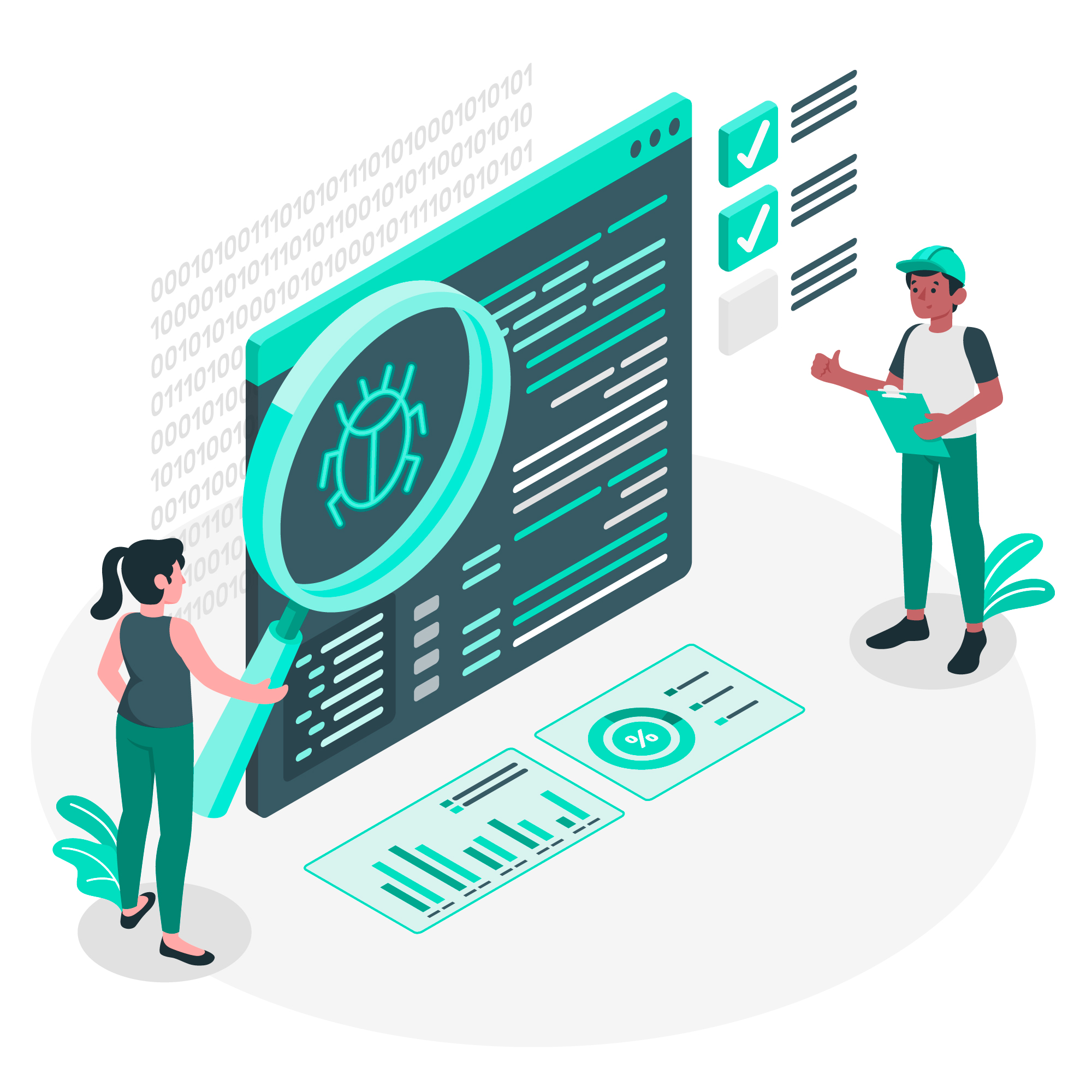
In the world of data validation, Zod stands out as a TypeScript-first schema declaration and validation library that promises simplicity and efficiency. Whether youโre dealing with simple strings or complex nested objects, Zod streamlines the process with minimal overhead and zero dependencies. In this blog post, we'll explore how Zod can transform your data validation approach, emphasizing its developer-friendly features and practical benefits. ๐ ๏ธ๐
1. What is Zod?
Zod is a schema declaration and validation library tailored for TypeScript but also functional in plain JavaScript. It provides a streamlined approach to defining and validating data structures, ranging from basic types to intricate nested objects. With Zod, you declare a validator once, and it automatically infers the static TypeScript type, making your code more maintainable and less prone to type mismatches. ๐๐
2. Key Features of Zod
TypeScript-First Approach
Zodโs TypeScript-first design means that schemas are not only easy to define but also automatically inferred. This eliminates the need for redundant type declarations, ensuring that your TypeScript code remains clean and type-safe. You define your schema, and Zod handles the type inference. ๐๐ ๏ธ
Zero Dependencies
With no external dependencies, Zod is lightweight and easy to integrate into any project. Itโs a standalone library that doesnโt bloat your application with unnecessary packages. ๐๐ชถ
Compact and Efficient
Zod is designed to be tiny, with a minified + zipped size of just 8kb. Despite its small footprint, it packs a punch with a comprehensive set of features. Efficiency is a priority, ensuring that your application remains performant and responsive. ๐๐
Immutable and Chainable
The methods in Zod, such as .optional()
, return new instances rather than modifying the original schema. This immutability ensures that your schema definitions remain intact and predictable. Additionally, Zodโs chainable interface allows for concise and readable code. ๐๐
Functional Approach
Zod uses a functional approach where you parse data rather than validate it. This paradigm shift simplifies the validation process and aligns with functional programming principles, leading to clearer and more predictable code. ๐งฉ๐
Cross-Platform Compatibility
Zod works seamlessly in Node.js, modern browsers, and plain JavaScript environments. Whether youโre building a Node.js server or a client-side application, Zod fits right in without requiring additional setup. ๐๐ป
3. Getting Started with Zod
Hereโs a step-by-step guide to getting started with Zod in your TypeScript or JavaScript project:
Installation
To add Zod to your project, simply install it via npm or yarn:
npm install zod
# or
yarn add zod
Basic Schema Definition
Define a basic schema for a simple object:
import { z } from 'zod';
const UserSchema = z.object({
name: z.string().min(1),
age: z.number().int().positive(),
});
Validating Data
Use Zod to validate data against your schema:
const result = UserSchema.safeParse({ name: 'Alice', age: 30 });
if (!result.success) {
console.error(result.error.format());
} else {
console.log('Valid data:', result.data);
}
Advanced Features
Explore advanced schema features such as nested objects and custom validations:
const AddressSchema = z.object({
street: z.string(),
city: z.string(),
zipCode: z.string().length(5),
});
const UserWithAddressSchema = z.object({
user: UserSchema,
address: AddressSchema,
});
4. Practical Applications
Form Validation
Implement Zod schemas to validate form inputs, ensuring users provide valid and correctly formatted data. Display informative error messages to guide users in correcting their inputs. ๐๐ง
API Data Validation
Validate data received from or sent to APIs, ensuring that it adheres to the required schemas. This helps maintain data integrity and consistency across your application. ๐๐
Configuration Validation
Use Zod to validate configuration files or environment variables, ensuring that your application operates with the correct settings. โ๏ธ๐
5. Best Practices
Leverage TypeScript Features: Utilize Zodโs TypeScript-first approach to benefit from type inference and autocompletion, enhancing code safety and productivity. ๐ก๐ง
Customize Error Handling: Tailor error messages to provide clear and actionable feedback to users and developers. Customize error handling to align with your applicationโs needs. ๐๐ ๏ธ
Optimize for Performance: When working with large or complex data structures, optimize your Zod schemas and validation logic to ensure efficient performance. โก๐
6. Conclusion
Zod simplifies data validation with its TypeScript-first approach, zero dependencies, and lightweight design. By integrating Zod into your projects, you can streamline schema declaration, enhance type safety, and maintain clean, efficient code. Whether youโre building a TypeScript application or working with plain JavaScript, Zod offers a robust solution for all your data validation needs. Dive into Zod today and experience a new level of validation efficiency! ๐๐
7. Learn More
For detailed documentation and advanced usage of Zod, visit the official Zod Documentation. Explore the full range of features and get started with creating powerful schemas and validations for your projects. ๐๐
8. Additional Resources
For a practical demonstration and in-depth walkthrough, check out Hitesh Sirโs video on Zod Overview and Practical Usage at Chai aur Code Youtube Channel. This video provides valuable insights and coding examples to help you get started with Zod effectively. ๐น๐จโ๐ป
Subscribe to my newsletter
Read articles from Parth Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Parth Chauhan
Parth Chauhan
I'm Parth Chauhan, a versatile web developer with expertise in both frontend and backend technologies. My passion lies in crafting clean, minimalistic designs and implementing effective branding strategies to create engaging and user-centric experiences. I am proficient in Java, Python, C, C++, SQL, MongoDB, computer networks, OS, NoSQL, and DSA. I thrive on bringing ideas to life by building solutions from scratch and am always excited to explore and master new technologies. My approach is centered around coding with precision and creativity, ensuring each project is both functional and aesthetically pleasing. Currently, I'm open to freelance opportunities and eager to collaborate on new ventures. Letโs connect to discuss how we can work together to enhance your project and achieve outstanding results.