RxDart 101: Your First Steps into Reactive Programming with Flutter

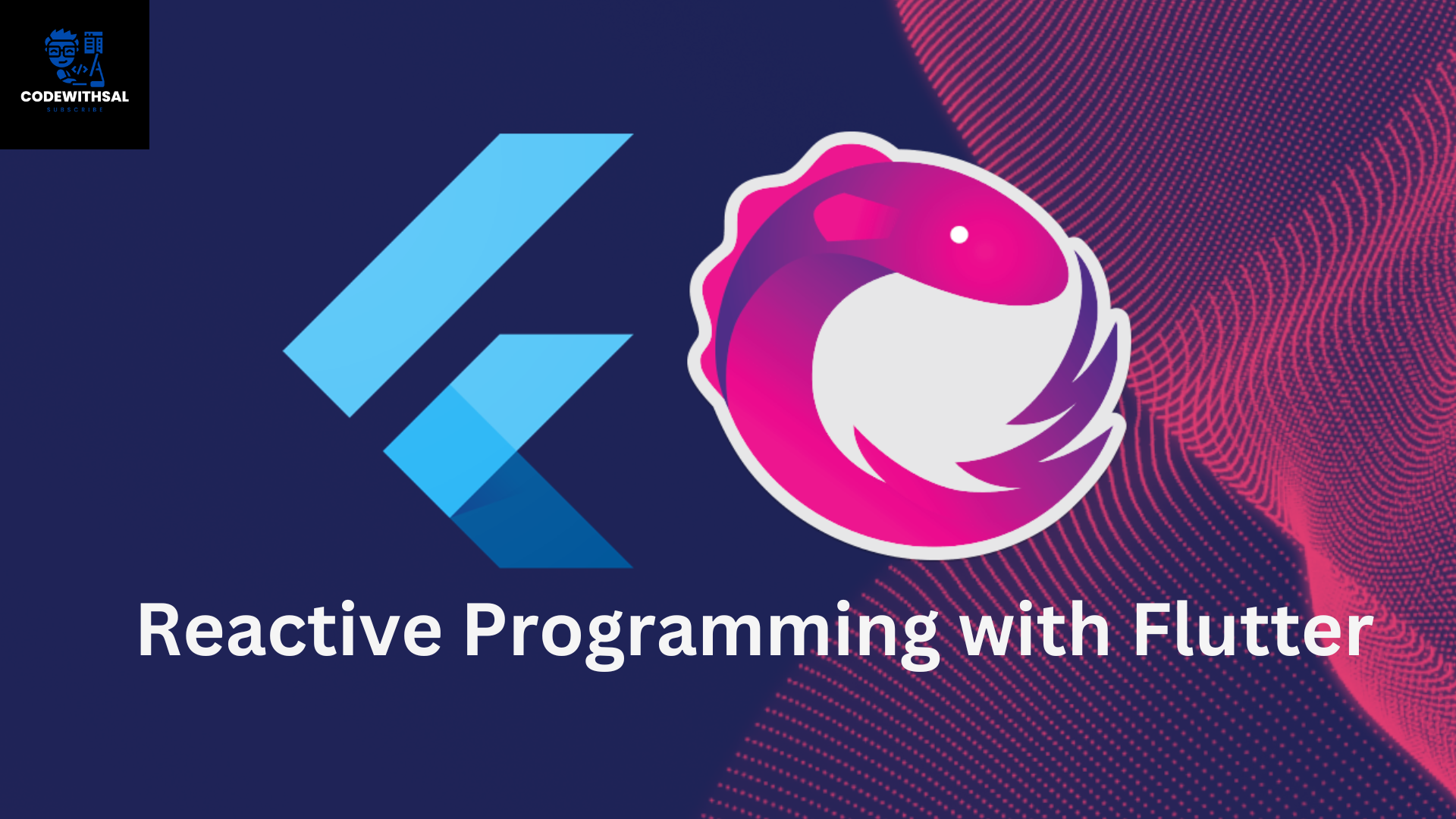
Welcome to the exciting world of reactive programming in Flutter! If you're new to Flutter development or have been hearing buzz about something called "RxDart," you're in the right place. This comprehensive beginner's guide will introduce you to the fundamental concepts of reactive programming using RxDart in Flutter. By the end of this article, you'll have a solid understanding of the basics and be ready to add some reactive magic to your apps.
What is RxDart? RxDart is an extension of Dart's Stream class, providing more powerful and flexible ways to work with asynchronous data streams. Think of it as a supercharged version of Dart's built-in streaming capabilities. RxDart is built on top of ReactiveX, a popular library for composing asynchronous and event-based programs using observable sequences.
Why Should You Care About Reactive Programming? Before we dive into the technical details, let's explore why reactive programming and RxDart are worth your time:
More responsive UIs: React to data changes instantly, creating fluid and dynamic user experiences.
Easier async operations: Simplify complex asynchronous tasks, making your code more readable and maintainable.
Cleaner code: Write more declarative and functional code, reducing the chances of bugs and improving overall code quality.
Better state management: Handle app state more efficiently, especially in complex applications.
Improved testability: Reactive code is often easier to test, leading to more robust applications.
Getting Started with RxDart: To begin our journey into reactive programming, let's first add RxDart to your Flutter project. Open your pubspec.yaml file and add the following dependency:
dependencies:
rxdart: ^0.28.0
Run flutter pub get
to fetch the package. Now you're ready to import RxDart in your Dart files:
import 'package:rxdart/rxdart.dart';
Core Concepts of RxDart:
- Observables: An Observable is the cornerstone of RxDart. It's essentially a Stream that emits data over time. Observables can emit zero or more events, followed by either a done event or an error.
Example:
final myObservable = Observable.just('Hello, RxDart!');
myObservable.listen(
(data) => print(data),
onError: (error) => print('Error: $error'),
onDone: () => print('Done'),
);
// Output: Hello, RxDart!
// Done
- Subjects: Subjects are special types of Observables that allow you to add data to the stream. They act as both an Observable (which you can subscribe to) and an Observer (which you can add data to).
RxDart provides several types of Subjects:
a. PublishSubject: Emits new events to all listeners.
b. BehaviorSubject: Emits the most recent event to new subscribers.
c. ReplaySubject: Emits a specified number of past events to new subscribers.
Example using BehaviorSubject:
final subject = BehaviorSubject<String>();
// Add some initial data
subject.add('First value');
// Subscribe to the subject
subject.listen((value) => print('Listener 1: $value'));
// Add more data
subject.add('Second value');
// Add another listener
subject.listen((value) => print('Listener 2: $value'));
// Output:
// Listener 1: First value
// Listener 1: Second value
// Listener 2: Second value
- Operators: RxDart provides a wide array of operators to transform and combine streams. These operators allow you to manipulate the data flowing through your streams in powerful ways.
Some common operators include:
a. map: Transform each event emitted by the source Observable.
b. where: Filter events based on a condition.
c. debounceTime: Emit an event only after a specified duration has passed without another event.
Example:
final numbers = Observable.fromIterable([1, 2, 3, 4, 5]);
numbers
.map((n) => n * 2)
.where((n) => n > 5)
.listen(print);
// Output: 6, 8, 10
A Simple Example:
Countdown Timer
Let's put these concepts together to create a simple countdown timer using RxDart:
import 'package:rxdart/rxdart.dart';
class CountdownBloc {
final _countdownController = BehaviorSubject<int>();
Stream<int> get countdown => _countdownController.stream;
void startCountdown(int start) {
Observable.periodic(Duration(seconds: 1), (tick) => start - tick - 1)
.take(start)
.listen(
_countdownController.add,
onDone: () => _countdownController.add(0),
);
}
void dispose() {
_countdownController.close();
}
}
Using the CountdownBloc in a Flutter widget:
class CountdownWidget extends StatelessWidget {
final bloc = CountdownBloc();
@override
Widget build(BuildContext context) {
bloc.startCountdown(10);
return StreamBuilder<int>(
stream: bloc.countdown,
builder: (context, snapshot) {
if (snapshot.hasData) {
return Text('Countdown: ${snapshot.data}');
} else {
return CircularProgressIndicator();
}
},
);
}
}
Best Practices for Beginners: As you start your journey with RxDart, keep these best practices in mind:
Always dispose of your subjects and cancel subscriptions to avoid memory leaks.
Use
distinct()
to prevent unnecessary UI updates when values haven't changed.Leverage
switchMap()
for operations that can be cancelled, like API calls.Start with simple use cases and gradually incorporate more complex RxDart features as you become comfortable.
Congratulations! You've taken your first steps into the world of reactive programming with RxDart. We've covered the fundamental concepts of Observables, Subjects, and Operators, and even created a simple countdown timer using these principles.
As you continue your Flutter journey, you'll find RxDart to be a powerful tool for handling asynchronous data and creating responsive UIs. The concepts we've explored here are just the tip of the iceberg – RxDart offers a wealth of advanced features and patterns that you can explore as you grow more comfortable with reactive programming.
Remember, practice makes perfect. Try incorporating these concepts into your next Flutter project and watch how it simplifies your code and improves your app's responsiveness. Don't be afraid to experiment and make mistakes – that's how you'll truly master RxDart.
In future articles, we'll explore more advanced topics like combining streams, handling errors, and implementing complex UI patterns using RxDart. Until then, happy coding, and welcome to the reactive world of Flutter development!
@gideonsalamii - X (formerly twitter)
Subscribe to my newsletter
Read articles from Gideon Salami directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gideon Salami
Gideon Salami
A skilled software engineer with expertise in Flutter and other frameworks, I have a passion for developing robust and efficient applications. With experience in both web and mobile app development, I am able to bring a unique perspective to each project.