Null vs Undefined in JavaScript

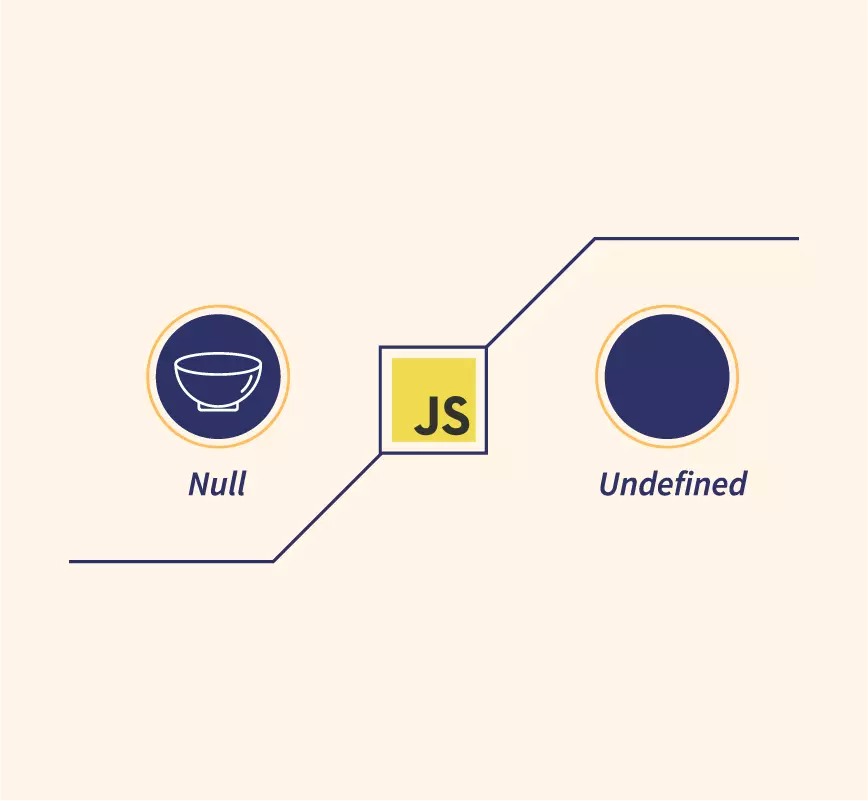
Definition and Usage
In JavaScript, null
is an assignment value that represents the intentional absence of any object value. It essentially denotes that a variable has been explicitly set to have no value. It can be thought of as a placeholder indicating that a deliberate decision has been made to leave a variable empty.
Behavior and Initial Value
When a variable is assigned null
, it is set to point to no object. Contrary to undefined
, assigning null
to a variable typically means the developer was aware the variable needed to exist but, at that moment, does not have any meaningful value. This explicit nullification is a key aspect of managing memory, particularly in more complex applications where the state of variables may dynamically change.
Type Checking Null
To determine if a variable is null
, developers should use the strict equality operator (===
). Notably, using the typeof
operator on null
returns "object," which is historically considered a bug in JavaScript but remains for compatibility reasons. Hence, a proper check is to use:
if (variable === null) {
// variable is null
}
Understanding Undefined in JavaScript
Definition and Usage
undefined
is a primitive value used when a variable is declared but not yet assigned a value. It signifies that a variable has been created, but its value has not been initialized. This can naturally occur when accessing uninitialized variables or missing function arguments.
Common Scenarios for Undefined
Variables will be undefined
if they:
Have been declared but not assigned a value.
Are function parameters that were not provided by the caller.
Are properties that do not exist on an object.
Have the return value of a function that does not explicitly return a value.
Type Checking Undefined
The typeof
operator returns "undefined" when evaluated with an undefined
variable. To check explicitly if a variable is undefined
, a strict equality operator check is recommended:
if (variable === undefined) {
// variable is undefined
}
Key Differences Between Null and Undefined
Intentional Absence vs Uninitialized
null
is employed to signify the intentional absence of an object value, assigned deliberately by developers. In contrast, undefined
typically represents a variable that has been declared but not yet assigned a value, often implying an uninitialized or unknown state.
Type and Equality Comparisons
From a type perspective, null
is an object (typeof null // "object"
), whereas undefined
is its own type (typeof undefined // "undefined"
). For equality comparisons, using the double equals (==
) operator might lead to confusion:
null == undefined // true
null === undefined // false
The strict equality (===
) should be used to avoid such issues, wherein it confirms that the values and types must match, thereby providing more predictable behavior.
Practical Examples
Consider the following code snippets:
let example1 = null;
let example2;
console.log(example1); // null
console.log(example2); // undefined
console.log(typeof example1); // "object"
console.log(typeof example2); // "undefined"
In these examples, you can observe the different behavior and type returns when handling null
and undefined
.
Best Practices for Using Null and Undefined
When to Use Null
Use null
when you want to explicitly indicate that a variable should be empty or devoid of value. This is useful for resetting or clearing objects and variables:
let user = { name: "Alex" };
user = null; // The user object is explicitly set to no value
When to Use Undefined
undefined
should typically result from the natural behavior of JavaScript, such as when variables are not initialized. Avoid manually setting variables to undefined
. Instead, use it to check if an expected variable, parameter, or return value is missing:
function checkParam(param) {
if (param === undefined) {
console.log("Parameter not provided!");
}
}
By understanding the distinct purposes and uses of null
and undefined
, developers can write clearer and more maintainable code, effectively managing unintentional errors and improving data handling consistency.
Subscribe to my newsletter
Read articles from ajayi odunayo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
