Essential Python Data Types Explained for New Programmers
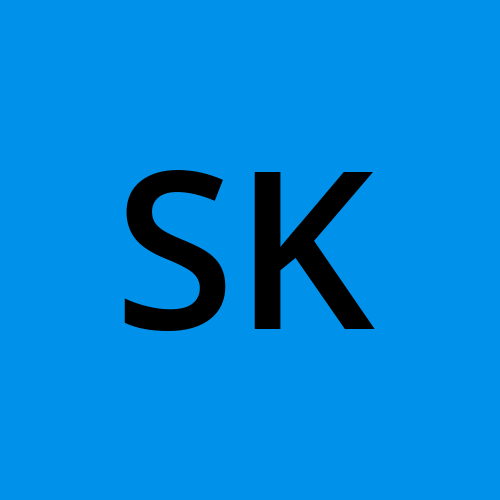
Python ek versatile aur powerful programming language hai jo alag-alag data types ko support karti hai. Yeh blog post Python ke primary data types ke baare me batayegi, including complex numbers, strings, booleans, lists, tuples, dictionaries, aur sets. Hum indentation, conventions aur best practices bhi discuss karenge jo aapko readable aur maintainable code likhne me madad karengi. Saath hi, typecasting aur redundancy ko handle karne ke tarike bhi dekhenge.
Python Data Types
1. Complex Numbers
Complex numbers mathematics me imaginary numbers ko represent karte hain. Python me complex numbers ko complex
data type use karke represent kiya jata hai.
Example: Quadratic Equations
# Quadratic equation: ax^2 + bx + c = 0
import cmath
a = 1
b = 2
c = 3
# Discriminant
d = (b**2) - (4*a*c)
# Roots
root1 = (-b - cmath.sqrt(d)) / (2*a)
root2 = (-b + cmath.sqrt(d)) / (2*a)
print(f"Root 1: {root1}")
print(f"Root 2: {root2}")
2. String
Strings text data ko represent karte hain. Python me strings ko single ya double quotes me likha jata hai.
Example:
name = "John Doe"
greeting = 'Hello, ' + name
print(greeting)
3. Boolean
Booleans logical values ko represent karte hain. Python me True
aur False
values ko boolean data type kaha jata hai.
Example:
is_valid = True
print(is_valid)
4. List
Lists ordered collection of items ko represent karte hain. Lists mutable hoti hain, yaani unki values ko badla ja sakta hai.
Example:
fruits = ["apple", "banana", "cherry"]
fruits.append("date")
print(fruits)
5. Tuple
Tuples ordered collection of items ko represent karte hain lekin yeh immutable hoti hain, yaani inki values ko change nahi kiya ja sakta.
Example:
colors = ("red", "green", "blue")
print(colors)
6. Dictionary
Dictionaries unordered collection of key-value pairs ko represent karte hain. Keys unique hoti hain aur unhe values se map kiya jata hai.
Example:
person = {"name": "John", "age": 30}
print(person["name"])
7. Set
Sets unordered collection of unique items ko represent karte hain. Sets me duplicate values allowed nahi hoti.
Example:
unique_numbers = {1, 2, 3, 3, 4}
print(unique_numbers) # Output: {1, 2, 3, 4}
Typecasting
Typecasting ek process hai jisme ek data type ko dusre data type me convert kiya jata hai.
Example:
# String to int
num_str = "100"
num_int = int(num_str)
print(num_int)
# List to set
numbers = [1, 2, 2, 3, 4]
unique_numbers = set(numbers)
print(unique_numbers)
Handling Duplicates and Redundancy
Python me sets aur dictionaries naturally duplicates ko handle karte hain kyunki sets me duplicate values allowed nahi hoti aur dictionaries me unique keys hoti hain.
Example:
# Handling duplicates with set
numbers = [1, 2, 2, 3, 4]
unique_numbers = set(numbers)
print(unique_numbers) # Output: {1, 2, 3, 4}
# Removing duplicates from a list
numbers = [1, 2, 2, 3, 4]
unique_numbers_list = list(set(numbers))
print(unique_numbers_list)
Conventions and Best Practices
Indentation: Python me indentation kaafi important hai. Har nested block ko 4 spaces se indent karein.
if True: print("Hello, World!")
Naming Conventions: Variables, functions ko
snake_case
me naam dein aur classes koCamelCase
me.def my_function(): pass class MyClass: pass
Comments and Documentation: Code ko readable banane ke liye comments aur docstrings ka use karein.
# This is a comment def add(a, b): """This function adds two numbers""" return a + b
Consistent Style: PEP 8 style guide ko follow karein taaki code consistent rahe.
Conclusion
Python me alag-alag data types use karne se hum data ko effectively manage kar sakte hain. Is blog post me humne complex numbers, strings, booleans, lists, tuples, dictionaries, aur sets ko discuss kiya. Typecasting aur redundancy handle karne ke methods bhi dekhe. Conventions aur best practices ko follow karke hum apne code ko readable, maintainable aur error-free bana sakte hain. Python ki flexibility aur powerful features isse beginners aur experienced programmers ke liye ek preferred language banate hain.
Happy coding!
Subscribe to my newsletter
Read articles from Sandhya Kondmare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
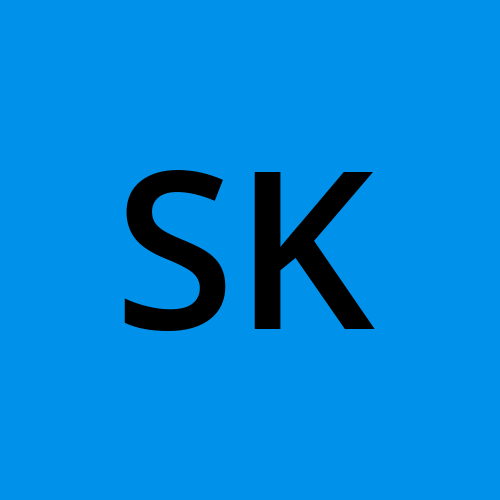
Sandhya Kondmare
Sandhya Kondmare
Aspiring DevOps Engineer with 2 years of hands-on experience in designing, implementing, and managing AWS infrastructure. Proven expertise in Terraform for infrastructure as code, automation tools, and scripting languages. Adept at collaborating with development and security teams to create scalable and secure architectures. Hands-on in AWS, GCP, Azure and Terraform best practices.