Props In React
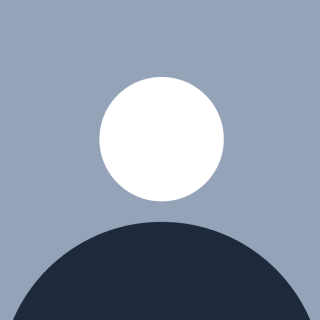
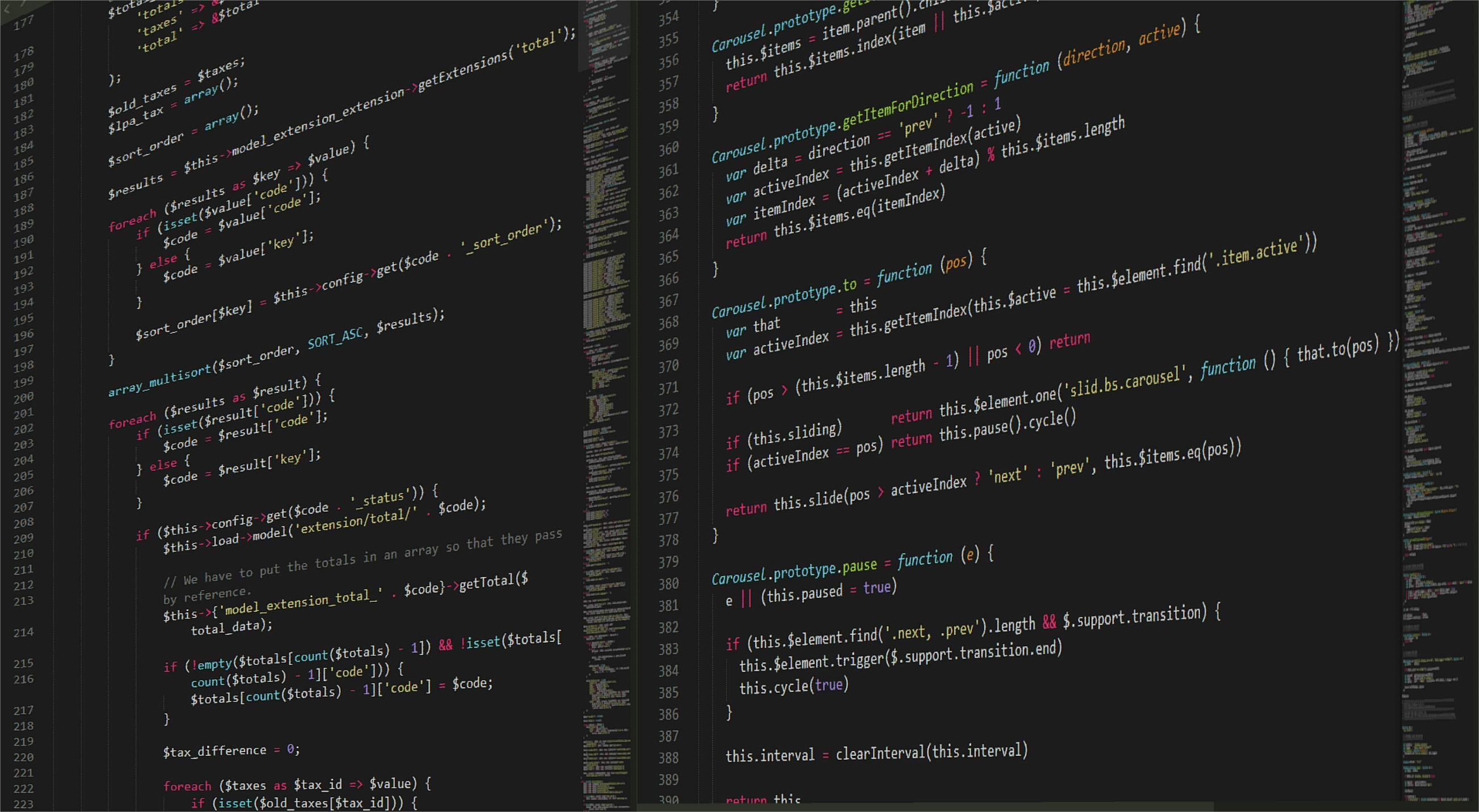
What is PROPS IN REACT? According to Mozilla Developer Network (MDN) Web docs, PROPS allows for the accessibility of the components. Props allow information to be passed from the top-level component (Parent) to another or the second-level component (child component).
The question is why use Props?
Props is used when the component is not specified or the initial values are defined.
Props is used in place of import i.e.(There is no need to keep importing data).
Props can hold any kind of data (strings, numbers, Booleans, objects, even functions!)
How do you use props?
You pass the props to the child component: e.g.: function ChildComponent(props) {}
The syntax to create props for a react component is :
function ParentComponent() {
// passing prop to ChildComponent
return <ChildComponent text="Hello!" number={2} />;
}
function ChildComponent(props) {
// using the values of the text and number of props
return (
<div>
{props.text} {props.number} // this is the syntax.
</div>
);
}
Things to remember about props:
Props are strings that allow the parent component to return the child component by passing data. The component being returned is the child component, and the component returning that child is the parent component, according to Flatiron School.
The props can be accessed in the child component via an object that is passed into our component function as an argument: function Comment(props) {}
One thing to note is that components can be re-usable. You can use one component and pass it multiple times to different components.
More on Props:
Check out Passing Props to a Component. : https://react.dev/learn/passing-props-to-a-component
Mozilla Developer Network (MDN): https://developer.mozilla.org/en-US/docs/Learn/Tools_and_testing/Client-side_JavaScript_frameworks/Svelte_variables_props#working_with_props
Subscribe to my newsletter
Read articles from Sherlyne Ochieng directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by