An Introduction to Abstract Data Types in Python
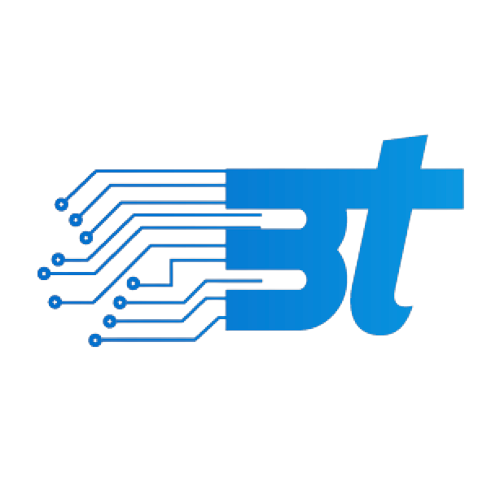
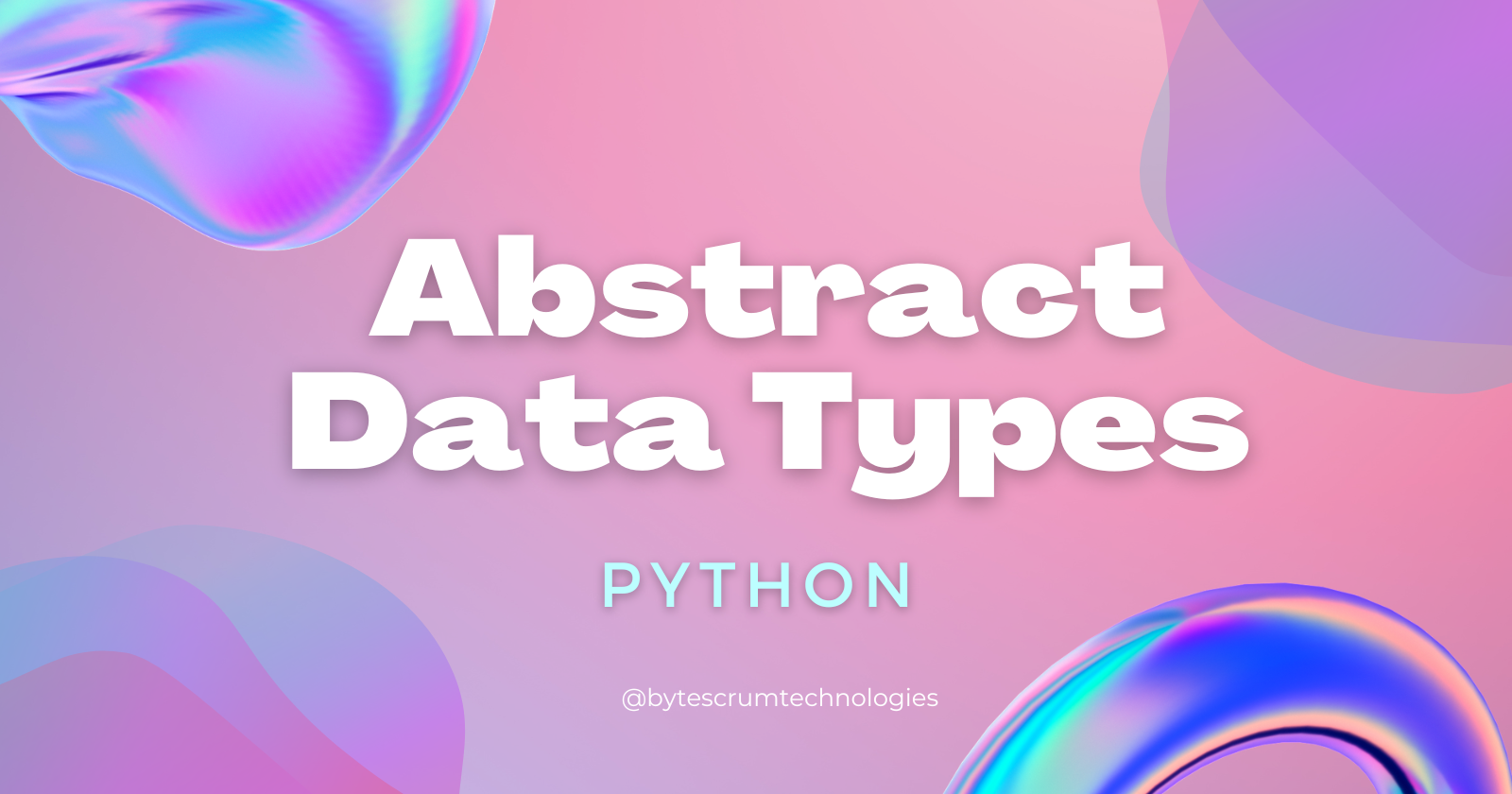
When diving into the world of programming, one of the fundamental concepts you'll encounter is Abstract Data Types (ADTs). ADTs provide a theoretical framework for organizing data, specifying the operations that can be performed on the data and the types of parameters those operations can accept. Unlike concrete data structures, ADTs do not dictate how these operations will be implemented. In Python, ADTs are crucial for writing clean, maintainable, and efficient code.
Introduction to Abstract Data Types
Abstract Data Types are mathematical models for data types where a data type is defined by its behavior from the point of view of a user, specifically in terms of possible values, possible operations on data of this type, and the behavior of these operations. ADTs are used to provide a clear specification of what a data structure does, without getting bogged down in how it does it. This separation of concerns is key in software development, allowing developers to think about high-level design without worrying about low-level details.
Common Abstract Data Types in Python
Several common ADTs are frequently used in Python:
List (Array): An ordered collection of elements.
Stack: A collection of elements with Last In, First Out (LIFO) access.
Queue: A collection of elements with First In, First Out (FIFO) access.
Set: A collection of distinct elements.
Dictionary (Map): A collection of key-value pairs.
Time and Space Complexity
Understanding the time and space complexity of operations on ADTs is crucial for writing efficient programs. Complexity analysis helps predict the behavior of an algorithm as the size of the input data grows.
List (Array)
A list is a collection of elements where each element can be accessed by its index. Lists are one of the most versatile data structures in Python and support a wide range of operations.
Access (indexing): O(1)
Search: O(n)
Insertion: O(n) (O(1) for append)
Deletion: O(n)
Space Complexity: O(n)
In a list, accessing an element by index is very efficient (O(1)) because the list is essentially an array in memory. However, searching, inserting, and deleting elements can be time-consuming, especially if the list is large, as these operations may require shifting elements.
Example:
# Creating a list
my_list = [1, 2, 3, 4, 5]
# Accessing elements
print(my_list[2]) # Output: 3
# Inserting elements
my_list.append(6) # [1, 2, 3, 4, 5, 6]
# Deleting elements
my_list.remove(3) # [1, 2, 4, 5, 6]
Stack
A stack is a collection of elements that follows the Last In, First Out (LIFO) principle. The most recently added element is the first one to be removed.
Push (insertion): O(1)
Pop (deletion): O(1)
Peek (access top element): O(1)
Search: O(n)
Space Complexity: O(n)
A stack is a specialized list where elements are added and removed from only one end, called the top. The push, pop, and peek operations are all O(1) because they involve only the top element. However, searching through a stack requires linear time, O(n).
Example:
# Implementing stack using list
stack = []
# Push operation
stack.append(1)
stack.append(2)
# Pop operation
print(stack.pop()) # Output: 2
# Peek operation
print(stack[-1]) # Output: 1
Queue
A queue operates similarly to a stack but with FIFO access. Enqueuing and dequeuing elements are O(1) operations because they occur at the ends of the queue. Searching through a queue, however, is a linear operation, O(n).
Example:
from collections import deque
# Creating a queue
queue = deque()
# Enqueue operation
queue.append(1)
queue.append(2)
# Dequeue operation
print(queue.popleft()) # Output: 1
# Peek operation
print(queue[0]) # Output: 2
Set
A set is a collection of distinct elements, meaning it cannot have duplicate elements. Sets are highly efficient for membership testing.
Insertion: O(1)
Deletion: O(1)
Search: O(1)
Space Complexity: O(n)
Sets are collections of unique elements. The primary operations on a set (insertion, deletion, and search) have an average time complexity of O(1) due to the underlying hash table implementation. This makes sets very efficient for membership testing and eliminating duplicates.
Example:
# Creating a set
my_set = {1, 2, 3, 4, 5}
# Adding elements
my_set.add(6)
# Removing elements
my_set.remove(3)
# Checking existence
print(4 in my_set) # Output: True
Dictionary (Map)
A dictionary, or map, is a collection of key-value pairs, where each key is unique and is used to store and retrieve values.
Insertion: O(1)
Deletion: O(1)
Search: O(1)
Space Complexity: O(n)
Dictionaries, or maps, store key-value pairs and also use hash tables for their implementation. The average time complexity for insertion, deletion, and search operations is O(1), making dictionaries highly efficient for associative arrays or mappings.
Example:
# Creating a dictionary
my_dict = {'a': 1, 'b': 2, 'c': 3}
# Accessing elements
print(my_dict['b']) # Output: 2
# Adding elements
my_dict['d'] = 4
# Deleting elements
del my_dict['a']
In-Depth Examples and Implementations
Let's delve deeper into the implementation and internal workings of these ADTs in Python.
Detailed List Operations
Lists in Python are implemented as dynamic arrays. This means they have an underlying array, and when the array's capacity is exceeded, a new array (usually larger) is allocated, and the old elements are copied to the new array.
# Creating a list
my_list = [1, 2, 3, 4, 5]
# Accessing elements
print(my_list[2]) # Output: 3
# Inserting elements at the end
my_list.append(6) # [1, 2, 3, 4, 5, 6]
# Inserting elements at a specific position
my_list.insert(2, 'a') # [1, 2, 'a', 3, 4, 5, 6]
# Deleting elements by value
my_list.remove(3) # [1, 2, 'a', 4, 5, 6]
# Deleting elements by index
del my_list[2] # [1, 2, 4, 5, 6]
# Iterating through the list
for element in my_list:
print(element)
Detailed Stack Operations
Stacks are often used in scenarios where you need to reverse the order of elements or manage function calls in recursion.
# Implementing stack using list
stack = []
# Push operation
stack.append(1)
stack.append(2)
stack.append(3)
# Pop operation
print(stack.pop()) # Output: 3
# Peek operation
print(stack[-1]) # Output: 2
# Checking if stack is empty
if not stack:
print("Stack is empty")
# Iterating through the stack
while stack:
print(stack.pop())
Detailed Queue Operations
Queues are useful for scenarios like scheduling tasks, managing order of execution, and breadth-first search (BFS) in graphs.
from collections import deque
# Creating a queue
queue = deque()
# Enqueue operation
queue.append(1)
queue.append(2)
queue.append(3)
# Dequeue operation
print(queue.popleft()) # Output: 1
# Peek operation
print(queue[0]) # Output: 2
# Checking if queue is empty
if not queue:
print("Queue is empty")
# Iterating through the queue
while queue:
print(queue.popleft())
Detailed Set Operations
Sets are often used for membership testing, eliminating duplicates, and performing set operations like union, intersection, and difference.
# Creating a set
my_set = {1, 2, 3, 4, 5}
# Adding elements
my_set.add(6)
# Removing elements
my_set.remove(3)
# Checking existence
print(4 in my_set) # Output: True
# Set operations
another_set = {4, 5, 6, 7}
# Union
union_set = my_set | another_set # {1, 2, 4, 5, 6, 7}
# Intersection
intersection_set = my_set & another_set # {4, 5, 6}
# Difference
difference_set = my_set - another_set # {1, 2}
# Symmetric Difference
symmetric_difference_set = my_set ^ another_set # {1, 2, 7}
Detailed Dictionary Operations
Dictionaries are widely used for storing and retrieving values based on keys, implementing caches, and counting occurrences of items.
# Creating a dictionary
my_dict = {'a': 1, 'b': 2, 'c': 3}
# Accessing elements
print(my_dict['b']) # Output: 2
# Adding elements
my_dict['d'] = 4
# Deleting elements
del my_dict['a']
# Iterating through keys and values
for key, value in my_dict.items():
print(f"{key}: {value}")
# Checking existence of a key
if 'c' in my_dict:
print("Key 'c' exists in the dictionary")
# Default value for non-existent keys
print(my_dict.get('e', 'Not found')) # Output: Not found
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
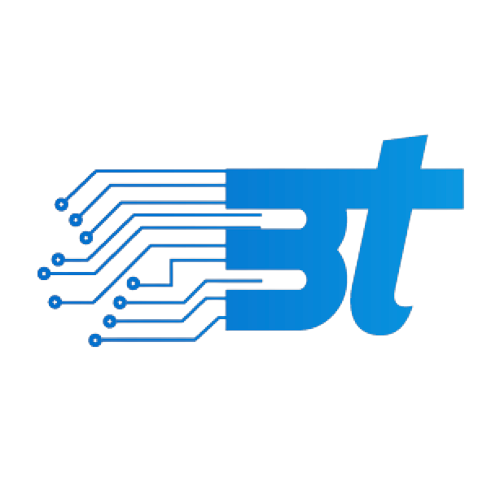
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.