Angular's Magical Feature

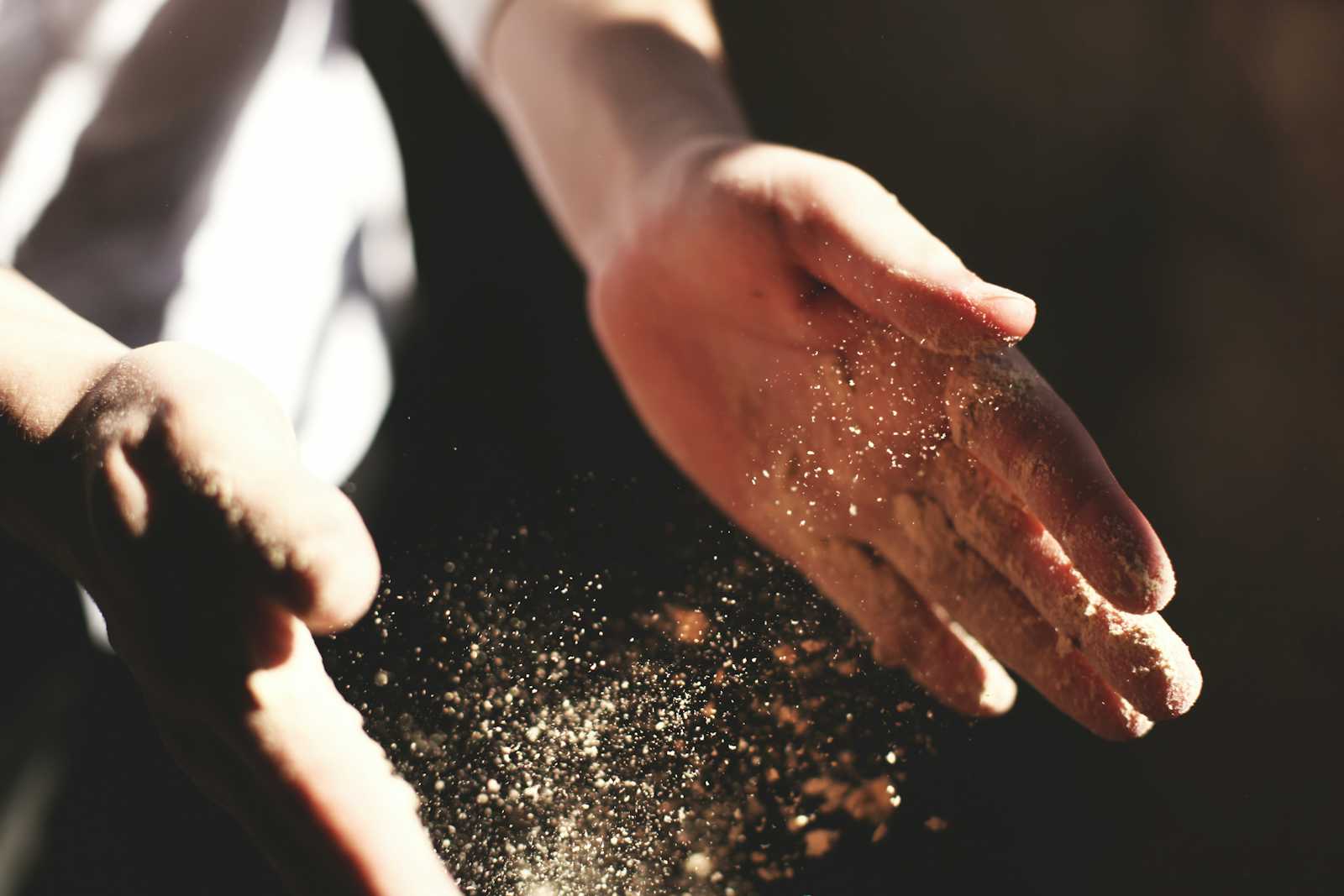
Two-Way Data Binding
Imagine you're a wizard, and with a wave of your wand, anything you change in one place magically updates everywhere else. That's what Angular’s two-way data binding does for your applications!
The Magic Explained
Two-way data binding lets you link your HTML and your TypeScript code so they update each other seamlessly. When you update a form input, the data in your component updates automatically, and any changes in the component reflect instantly in the UI. This makes your code simpler and more intuitive, perfect for beginners!
A Simple Spell: ngModel
To see the magic in action, let’s create a simple app where you can type your name, and it shows up instantly.
Create Your Component:
import { Component } from '@angular/core'; @Component({ selector: 'app-magic-binding', template: ` <input [(ngModel)]="name" placeholder="Enter your name"> <p>Hello, {{ name }}!</p> `, styles: [] }) export class MagicBindingComponent { name: string = ''; }
Add FormsModule:
Ensure
FormsModule
is imported in yourAppModule
:import { FormsModule } from '@angular/forms'; @NgModule({ declarations: [ MagicBindingComponent, // other components ], imports: [ FormsModule, // other modules ], bootstrap: [AppComponent] }) export class AppModule { }
Witness the Magic:
As you type in the input field, the paragraph updates instantly. The
[(ngModel)]
directive binds the input field to thename
property in your component, creating a two-way data binding.
Why It’s Magical
Simplicity: Reduces the amount of code needed to keep your UI in sync with your data.
Intuitiveness: Makes it easy to understand how changes in the UI affect the underlying data model and vice versa.
Efficiency: Helps you build dynamic and interactive applications with minimal effort.
With Angular’s two-way data binding, you can create dynamic and responsive applications effortlessly. Start waving your coding wand and see the magic unfold! 🪄
Subscribe to my newsletter
Read articles from sangeetha selvakumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
