Jenkins Pipeline Scripts:
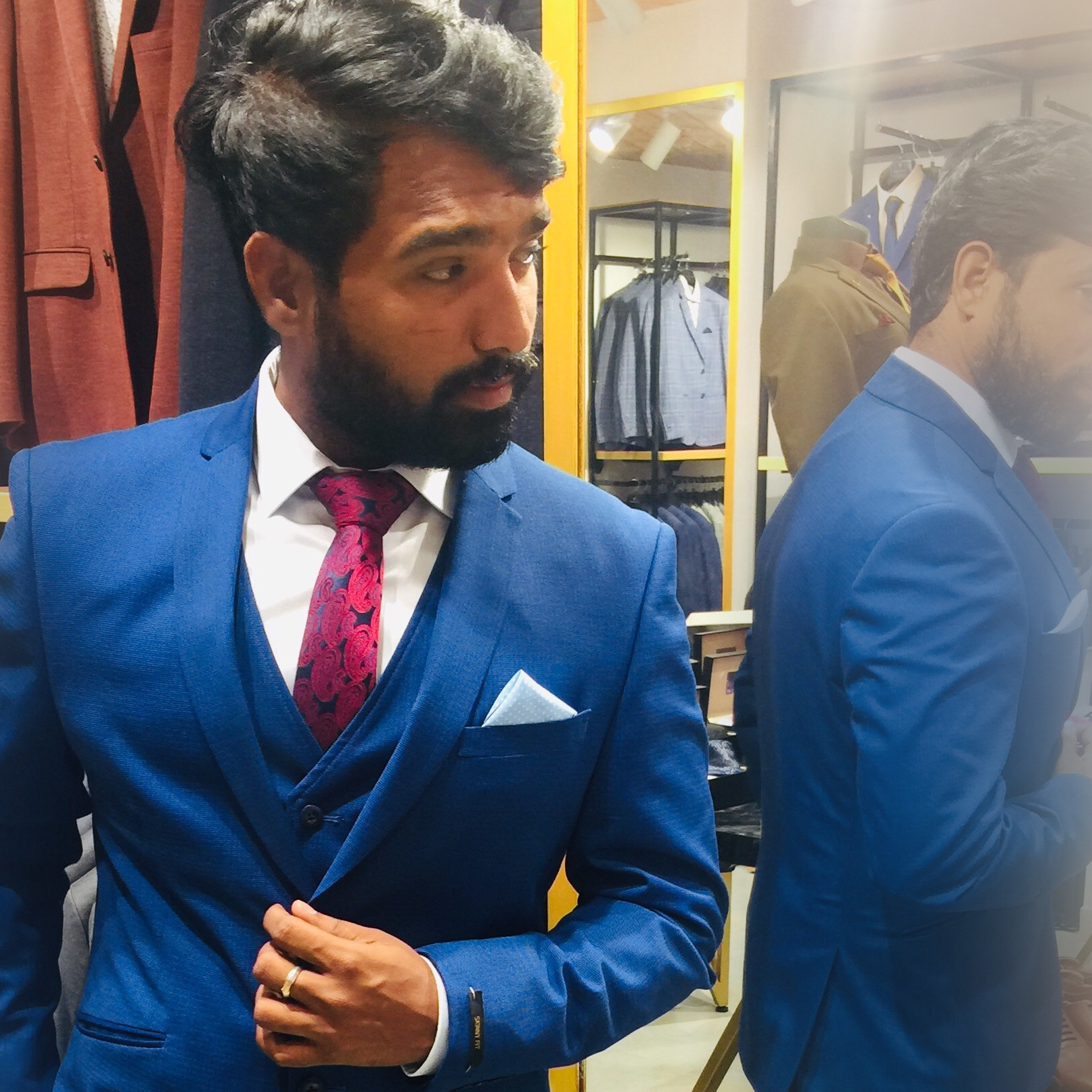
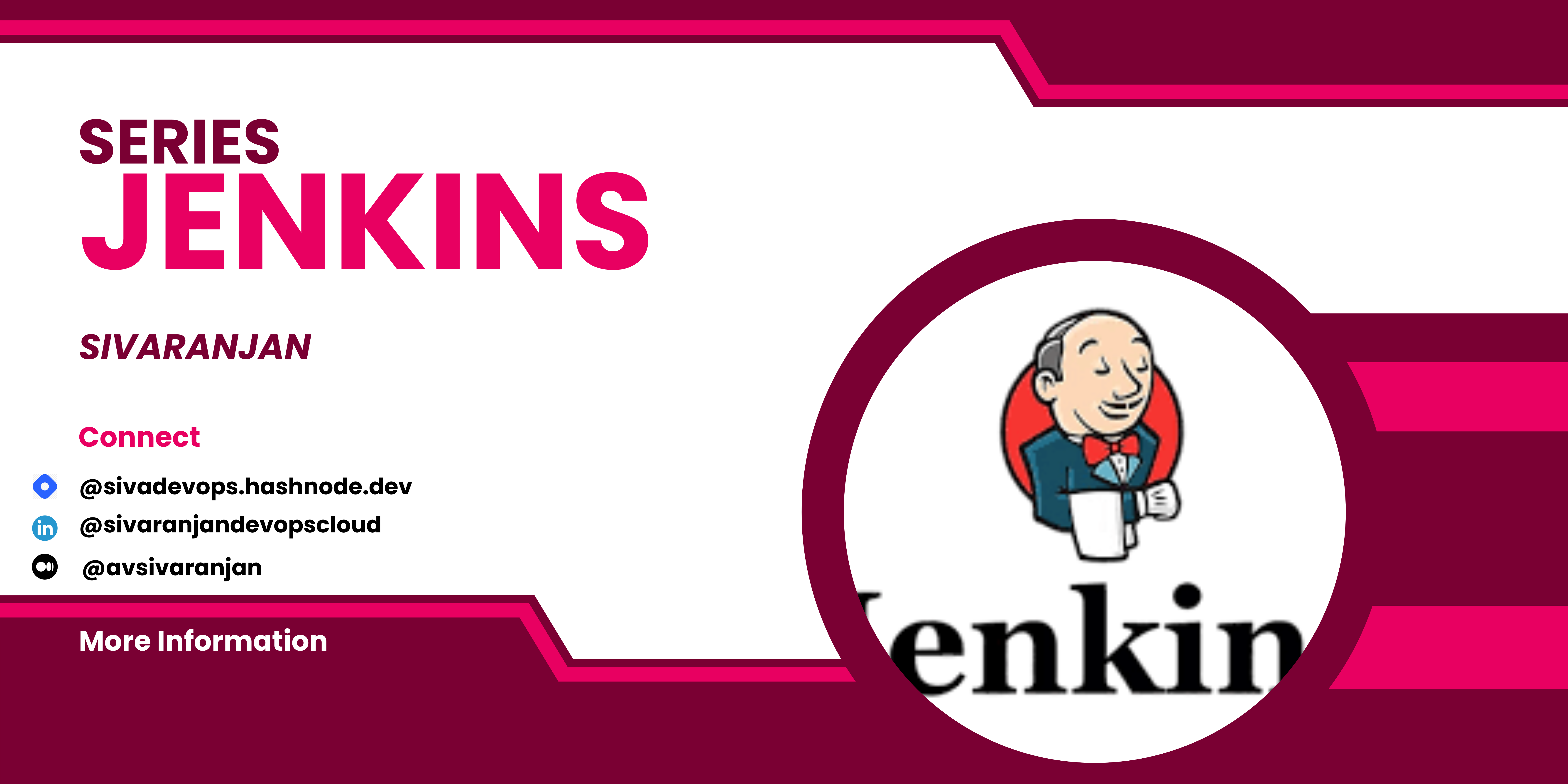
Basic Pipeline Questions
What is a Jenkins pipeline and how is it defined in a
Jenkinsfile
?Answer: A Jenkins pipeline is a suite of plugins that support implementing and integrating continuous delivery pipelines into Jenkins. It is defined in a
Jenkinsfile
, which can be written in Declarative or Scripted syntax.Sample
Jenkinsfile
(Declarative):pipeline { agent any stages { stage('Build') { steps { echo 'Building...' } } stage('Test') { steps { echo 'Testing...' } } stage('Deploy') { steps { echo 'Deploying...' } } } }
How do you use environment variables in a Jenkins pipeline?
Answer: Environment variables can be used in Jenkins pipelines to store and use configuration values. They can be defined in the
environment
block.Sample
Jenkinsfile
:pipeline { agent any environment { MY_ENV_VAR = 'some_value' } stages { stage('Print Environment Variable') { steps { echo "The environment variable is ${MY_ENV_VAR}" } } } }
What is the purpose of the
when
directive in a Jenkins pipeline?Answer: The
when
directive is used to conditionally execute stages based on specific criteria, such as the branch name or the presence of a file.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Deploy') { when { branch 'main' } steps { echo 'Deploying to production...' } } } }
How do you handle parallel execution in a Jenkins pipeline?
Answer: Parallel execution is handled using the
parallel
directive to run multiple stages or steps simultaneously.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Parallel Stage') { parallel { stage('Job 1') { steps { echo 'Running Job 1...' } } stage('Job 2') { steps { echo 'Running Job 2...' } } } } } }
How do you archive artifacts in a Jenkins pipeline?
Answer: Artifacts can be archived using the
archiveArtifacts
step to preserve files after a build completes.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Build') { steps { echo 'Building...' // Example command to create artifacts sh 'touch myfile.txt' } } stage('Archive') { steps { archiveArtifacts 'myfile.txt' } } } }
Intermediate Pipeline Questions
What is the
input
step and how is it used in a pipeline?Answer: The
input
step prompts the user for input during the pipeline execution, which can be used for manual approval or dynamic decision-making.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Approval') { steps { script { input 'Approve deployment?' } } } stage('Deploy') { steps { echo 'Deploying...' } } } }
How do you use
stash
andunstash
in Jenkins pipelines?Answer:
stash
is used to store files temporarily, andunstash
is used to retrieve them in a later stage or in parallel execution.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Build') { steps { sh 'echo "Building files" > myfile.txt' stash name: 'my-stash', includes: 'myfile.txt' } } stage('Test') { steps { unstash 'my-stash' sh 'cat myfile.txt' } } } }
How do you use
post
actions in a Jenkins pipeline?Answer: The
post
block allows you to define actions that should run after the pipeline stages complete, regardless of the build result.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Build') { steps { echo 'Building...' } } } post { success { echo 'Build was successful!' } failure { echo 'Build failed.' } always { echo 'This will always run.' } } }
What is the
tools
directive used for in Jenkins pipelines?Answer: The
tools
directive specifies which tools (like JDK, Maven, or Gradle) to use in the pipeline, ensuring the correct version is available for the build.Sample
Jenkinsfile
:pipeline { agent any tools { jdk 'JDK11' maven 'Maven3.6.3' } stages { stage('Build') { steps { sh 'mvn clean install' } } } }
How do you use
withCredentials
in Jenkins pipelines?Answer:
withCredentials
provides a way to securely use credentials stored in Jenkins within the pipeline script.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Build') { steps { withCredentials([usernamePassword(credentialsId: 'my-credentials', usernameVariable: 'USER', passwordVariable: 'PASS')]) { sh 'echo $USER' sh 'echo $PASS' } } } } }
Advanced Pipeline Questions
How do you manage Jenkins pipeline library and reuse code?
Answer: Jenkins shared libraries allow you to reuse pipeline code across multiple pipelines. They are defined in a separate Git repository and can be referenced in Jenkinsfiles.
Sample
Jenkinsfile
:@Library('my-shared-library') _ pipeline { agent any stages { stage('Build') { steps { mySharedLibrary.build() } } } }
What is the purpose of
script
blocks in Jenkins pipelines?Answer:
script
blocks are used in Declarative pipelines to execute arbitrary Groovy code, providing more flexibility than standard steps.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Custom Script') { steps { script { def result = sh(script: 'echo "Hello, World!"', returnStdout: true).trim() echo "Script result: ${result}" } } } } }
How do you handle complex logic in Jenkins pipelines?
Answer: Complex logic can be handled using
script
blocks in Declarative pipelines or using Scripted pipelines, which offer more flexibility for complex workflows.Sample
Jenkinsfile
(Scripted):node { stage('Complex Logic') { def result = sh(script: 'curl -s https://api.example.com/data', returnStdout: true).trim() if (result == 'expected') { echo 'Data is as expected.' } else { error 'Data is not as expected.' } } }
How do you create and use Jenkins pipeline parameters?
Answer: Parameters can be defined to make pipelines more flexible by allowing user input or setting dynamic values.
Sample
Jenkinsfile
:pipeline { agent any parameters { string(name: 'PARAM', defaultValue: 'default_value', description: 'A parameter') } stages { stage('Print Parameter') { steps { echo "Parameter value is ${params.PARAM}" } } } }
How do you integrate Jenkins pipelines with Kubernetes?
Answer: Jenkins can be integrated with Kubernetes by running Jenkins agents as Kubernetes pods, allowing dynamic scaling and environment isolation.
Sample
Jenkinsfile
:pipeline { agent { kubernetes { label 'my-agent' defaultContainer 'jnlp' yaml ''' apiVersion: v1 kind: Pod spec: containers: - name: jnlp image: jenkins/inbound-agent args: ['$(JENKINS_SECRET)', '$(JENKINS_NAME)'] ''' } } stages { stage('Build') { steps { sh 'echo Building...
' } } } } ```
How do you handle timeouts in Jenkins pipelines?
Answer: Timeouts can be set on individual stages or for the entire pipeline to prevent long-running jobs from hanging indefinitely.
Sample
Jenkinsfile
:pipeline { agent any stages { stage('Build') { options { timeout(time: 10, unit: 'MINUTES') } steps { echo 'Building...' } } } }
What are Jenkins pipeline libraries and how do they work?
Answer: Pipeline libraries are shared code repositories used to centralize common pipeline functions and steps. They can be imported into Jenkinsfiles and reused across multiple pipelines.
Sample
Jenkinsfile
:@Library('my-pipeline-library') _ pipeline { agent any stages { stage('Use Shared Library') { steps { myLibraryMethod() } } } }
How do you handle failures and retries in Jenkins pipelines?
Answer: Failures and retries can be managed using the
retry
directive, which allows a stage or step to be retried a specified number of times upon failure.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Retry Stage') { steps { retry(3) { sh 'curl -s https://api.example.com/data' } } } } }
How do you manage Jenkins pipeline artifacts and workspaces?
Answer: Artifacts can be archived for later use, and workspaces can be cleaned up after job completion to free up space.
Sample
Jenkinsfile
:pipeline { agent any stages { stage('Build') { steps { sh 'echo Building...' archiveArtifacts artifacts: '**/target/*.jar', allowEmptyArchive: true } } stage('Clean Workspace') { steps { cleanWs() } } } }
How do you implement a multi-branch pipeline in Jenkins?
Answer: Multi-branch pipelines automatically create and manage pipelines for each branch in a repository, allowing for branch-specific builds.
Sample
Jenkinsfile
:pipeline { agent any stages { stage('Build') { steps { echo "Building ${env.BRANCH_NAME}" } } } }
Configuration: Create a "Multibranch Pipeline" job in Jenkins and configure the source repository. Jenkins will automatically detect branches and create jobs accordingly.
Advanced Pipeline Concepts
How do you perform code quality analysis in a Jenkins pipeline?
Answer: Code quality analysis can be integrated into a Jenkins pipeline using tools like SonarQube.
Sample
Jenkinsfile
:pipeline { agent any stages { stage('Code Quality') { steps { withSonarQubeEnv('SonarQube') { sh 'mvn sonar:sonar' } } } } }
How do you use the
timestamps
directive in Jenkins pipelines?Answer: The
timestamps
directive adds timestamps to the console log output, which helps in debugging and performance monitoring.Sample
Jenkinsfile
:pipeline { agent any options { timestamps() } stages { stage('Build') { steps { echo 'Building...' } } } }
What is a
Jenkinsfile
Linter and how do you use it?Answer: The Jenkinsfile Linter validates the syntax of a Jenkinsfile before using it in a pipeline. It can be accessed via the Jenkins UI or through the CLI.
Sample
Jenkinsfile
(Command to lint):curl -X POST -F "jenkinsfile=<Jenkinsfile" http://jenkins-url/pipeline-model-converter/validate
How do you set up notifications in a Jenkins pipeline?
Answer: Notifications can be set up using plugins like Email Extension or Slack, integrated within the pipeline script.
Sample
Jenkinsfile
:pipeline { agent any stages { stage('Build') { steps { echo 'Building...' } } } post { success { mail to: 'user@example.com', subject: 'Build Successful', body: 'The build was successful.' } failure { mail to: 'user@example.com', subject: 'Build Failed', body: 'The build failed.' } } }
How do you manage build dependencies in Jenkins pipelines?
Answer: Build dependencies can be managed using tools like Maven or Gradle, configured within the pipeline script.
Sample
Jenkinsfile
:pipeline { agent any stages { stage('Build') { steps { sh 'mvn clean install' } } } }
How do you use the
lock
step in a Jenkins pipeline?Answer: The
lock
step allows you to ensure that a particular resource is not accessed concurrently by multiple builds.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Critical Section') { steps { lock(resource: 'my-resource') { sh 'echo "Running critical section"' } } } } }
What is the purpose of the
retry
directive in Jenkins pipelines?Answer: The
retry
directive allows a stage or step to be retried a specified number of times if it fails, useful for transient errors.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Build') { steps { retry(3) { sh 'curl -s http://unstable-service' } } } } }
How do you trigger another Jenkins job from a pipeline?
Answer: You can trigger another Jenkins job using the
build
step within the pipeline script.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Trigger Job') { steps { build job: 'another-job', parameters: [string(name: 'PARAM1', value: 'value1')] } } } }
How do you handle secret management in Jenkins pipelines?
Answer: Secrets can be managed using Jenkins Credentials and accessed securely using
withCredentials
.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Build') { steps { withCredentials([usernamePassword(credentialsId: 'my-credentials', usernameVariable: 'USER', passwordVariable: 'PASS')]) { sh 'echo $USER' sh 'echo $PASS' } } } } }
How do you implement a rolling deployment strategy in Jenkins pipelines?
Answer: Rolling deployments can be implemented using tools like Kubernetes, integrated within the pipeline script.
Sample
Jenkinsfile
:pipeline { agent any stages { stage('Deploy') { steps { kubernetesDeploy configs: 'k8s/deployment.yaml', kubeConfig: [path: '/path/to/kubeconfig'] } } } }
How do you use the
fileExists
step in Jenkins pipelines?Answer: The
fileExists
step checks for the existence of a file and can be used to conditionally execute steps.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Check File') { steps { script { if (fileExists('myfile.txt')) { echo 'File exists' } else { echo 'File does not exist' } } } } } }
How do you handle long-running tasks in Jenkins pipelines?
Answer: Long-running tasks can be managed using
timeout
andretry
directives to handle failures and timeouts appropriately.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Long Running Task') { steps { timeout(time: 30, unit: 'MINUTES') { sh 'sleep 1800' } } } } }
How do you use the
catchError
step in Jenkins pipelines?Answer: The
catchError
step catches errors within a block and continues the pipeline execution, useful for non-critical steps.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Build') { steps { catchError(buildResult: 'UNSTABLE', stageResult: 'FAILURE') { sh 'exit 1' } echo 'This will still run even if the previous step fails' } } } }
How do you use the
pipeline
step to create a nested pipeline?Answer: The
pipeline
step can be used to create a nested pipeline, allowing for modular and reusable pipeline code.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Nested Pipeline') { steps { script { def nestedPipeline = load 'nestedPipeline.groovy' nestedPipeline.run() } } } } }
How do you implement conditional execution in Jenkins pipelines?
Answer: Conditional execution can be implemented using the
when
directive or withinscript
blocks.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Deploy') { when { branch 'main' } steps { echo 'Deploying to production...' } } } }
How do you integrate Jenkins pipelines with GitHub for automated builds?
Answer: Jenkins pipelines can be integrated with GitHub using webhooks and the GitHub plugin to trigger builds on code changes.
Sample
Jenkinsfile
:pipeline { agent any triggers { githubPush() } stages { stage('Build') { steps { git 'https://github.com/user/repo.git' sh 'mvn clean install' } } } }
How do you use the
dir
step to change directories in a Jenkins pipeline?Answer: The
dir
step changes the current working directory for the enclosed steps.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Change Directory') { steps { dir('subdir') { sh 'echo "Current directory: $(pwd)"' } } } } }
How do you use the
withDockerContainer
step in Jenkins pipelines?Answer: The
withDockerContainer
step allows you to run steps inside a Docker container.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Run in
Docker') { steps { withDockerContainer('node:14') { sh 'node -v' } } } } } ```
How do you use the
withEnv
step to set environment variables in a Jenkins pipeline?Answer: The
withEnv
step sets environment variables for the enclosed steps.Sample
Jenkinsfile
:pipeline { agent any stages { stage('Set Env Vars') { steps { withEnv(['MY_VAR=some_value']) { sh 'echo $MY_VAR' } } } } }
How do you handle user input in Jenkins pipelines?
Answer: User input can be handled using the
input
step, which pauses the pipeline and waits for user interaction.Sample
Jenkinsfile
:pipeline { agent any stages { stage('User Input') { steps { script { def userInput = input message: 'Approve deployment?', parameters: [booleanParam(defaultValue: false, description: '', name: 'Approve')] if (userInput) { echo 'Deployment approved' } else { echo 'Deployment not approved' } } } } } }
Subscribe to my newsletter
Read articles from Sivaranjan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
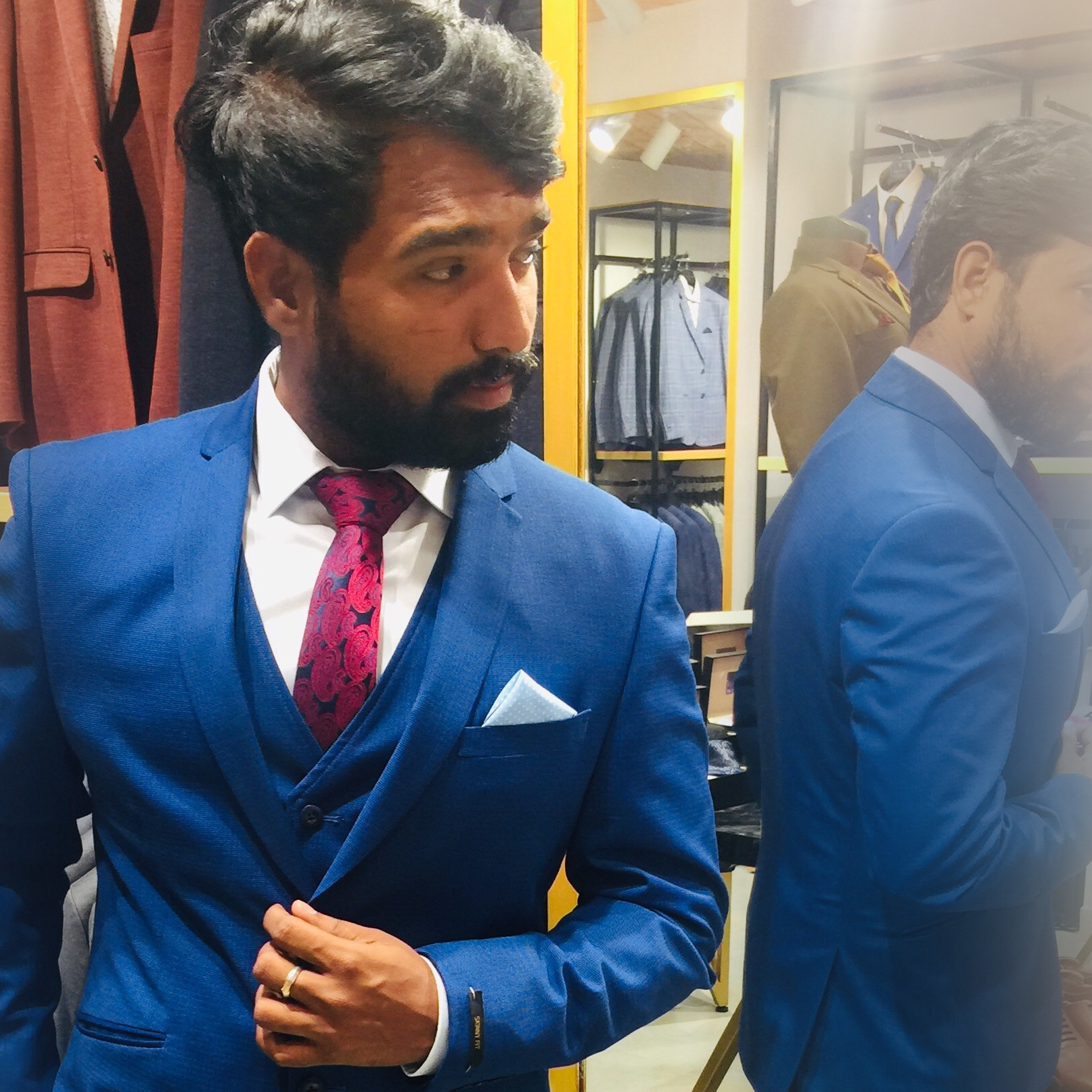
Sivaranjan
Sivaranjan
Cloud & DevOps Engineer | AWS Solutions Architect | Terraform Associate | Love to work with #AWS #Terraform #Docker #Kubernetes #Jenkins #GitHub #Linux