Handling File Uploads

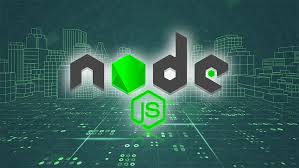
Welcome to Day 14 of our Node.js blog series!๐๐๐
Today, we'll dive into handling file uploads in Node.js applications. File uploads are a common requirement in web applications, whether for user profile images, documents, or other types of files. We'll explore setting up file uploads using the popular Multer middleware, handling multipart form data, storing uploaded files, and ensuring proper validation and processing.
Setting Up File Uploads with Multer
Multer is a middleware for handling multipart/form-data
, which is primarily used for uploading files. It makes it easy to handle file uploads in a Node.js application by providing a simple API to access file data and metadata.
Installation
To get started with Multer, you need to install it via npm:
npm install multer
Basic Setup
Importing Multer:
Import Multer into your application and set up a storage configuration. By default, Multer stores files in memory, but you can also configure it to save files to the disk.
const express = require('express'); const multer = require('multer'); const path = require('path'); const app = express(); const PORT = process.env.PORT || 3000; // Configure storage options const storage = multer.diskStorage({ destination: (req, file, cb) => { cb(null, 'uploads/'); }, filename: (req, file, cb) => { const uniqueSuffix = Date.now() + '-' + Math.round(Math.random() * 1E9); cb(null, file.fieldname + '-' + uniqueSuffix + path.extname(file.originalname)); } }); const upload = multer({ storage });
Explanation:
multer.diskStorage()
configures the storage location and filename for uploaded files.The
destination
function specifies the directory where files will be saved.The
filename
function generates a unique filename for each uploaded file, incorporating a timestamp and a random number to avoid collisions.
Uploading a File:
Define a route to handle file uploads. Use the
upload.single()
middleware for single file uploads orupload.array()
for multiple file uploads.app.post('/upload', upload.single('file'), (req, res) => { try { // req.file contains information about the uploaded file console.log(req.file); res.status(200).send('File uploaded successfully'); } catch (error) { res.status(500).send(error.message); } }); app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
Explanation:
The
upload.single('file')
middleware handles single file uploads. The string'file'
should match the name attribute of the file input field in the HTML form.The
req.file
object contains information about the uploaded file, such as its original name, storage location, size, and mimetype.
Handling Multipart Form Data
Multer processes multipart/form-data
and provides easy access to both file and non-file fields.
Handling Form Data:
If you have additional form fields besides the file, Multer makes them accessible via
req.body
.app.post('/upload', upload.single('file'), (req, res) => { try { console.log(req.file); // File data console.log(req.body); // Form data res.status(200).send('File uploaded successfully'); } catch (error) { res.status(500).send(error.message); } });
Explanation:
req.file
contains the uploaded file's information.req.body
contains other form fields submitted along with the file.
Storing Uploaded Files
Uploaded files can be stored on the server's file system, in a database, or a cloud storage service.
File System Storage:
- Using Multer's disk storage, files are stored on the server. This approach is simple but may not scale well if you have many uploads or large files.
Database Storage:
- You can store file metadata (e.g., filename, path) in a database for easy retrieval. The files themselves can be stored as binary data (BLOBs), but this is less common due to database size limitations.
Cloud Storage:
- For scalability, consider using cloud storage services like AWS S3, Google Cloud Storage, or Azure Blob Storage. These services offer high availability, scalability, and additional features like content delivery and security.
Example: AWS S3 Storage:
const AWS = require('aws-sdk'); const multerS3 = require('multer-s3'); AWS.config.update({ accessKeyId: 'your-access-key-id', secretAccessKey: 'your-secret-access-key' }); const s3 = new AWS.S3(); const upload = multer({ storage: multerS3({ s3, bucket: 'your-bucket-name', metadata: (req, file, cb) => { cb(null, { fieldName: file.fieldname }); }, key: (req, file, cb) => { const uniqueSuffix = Date.now() + '-' + Math.round(Math.random() * 1E9); cb(null, file.fieldname + '-' + uniqueSuffix + path.extname(file.originalname)); } }) });
Explanation:
- This setup uploads files directly to an S3 bucket. The
multer-s3
package integrates Multer with AWS S3, allowing file uploads to be stored in the cloud.
- This setup uploads files directly to an S3 bucket. The
Validating and Processing Uploaded Files
It's crucial to validate and possibly process uploaded files for security and usability reasons.
Validation:
File Type Check: Only allow specific file types to be uploaded.
File Size Check: Limit the maximum file size to prevent abuse and ensure efficiency.
const upload = multer({
storage,
limits: { fileSize: 5 * 1024 * 1024 }, // 5 MB
fileFilter: (req, file, cb) => {
const fileTypes = /jpeg|jpg|png|gif/;
const extname = fileTypes.test(path.extname(file.originalname).toLowerCase());
const mimetype = fileTypes.test(file.mimetype);
if (mimetype && extname) {
return cb(null, true);
} else {
cb(new Error('Only images are allowed'));
}
}
});
Explanation:
limits.fileSize
sets a maximum file size limit (in bytes).fileFilter
checks the file extension and mimetype to ensure only specific types of files are accepted.
Processing:
- After a file is uploaded, you may want to process it, such as resizing images, converting formats, or extracting metadata.
Example: Image Resizing with Sharp:
const sharp = require('sharp');
app.post('/upload', upload.single('file'), async (req, res) => {
try {
await sharp(req.file.path)
.resize(300, 300)
.toFile(`uploads/resized-${req.file.filename}`);
res.status(200).send('File uploaded and resized successfully');
} catch (error) {
res.status(500).send(error.message);
}
});
Explanation:
- The
sharp
library is used to resize an image after upload. This is just one example of file processing; similar techniques can be used for other types of files or processing requirements.
- The
Conclusion
In today's post, we explored handling file uploads in Node.js applications using Multer. We covered setting up file uploads, handling multipart form data, storing uploaded files, and validating and processing files. These are essential skills for building robust and secure web applications that handle user-generated content.
In the next post, we'll delve into more advanced topics, enhancing your understanding and skills in Node.js development. Stay tuned for more insights and practical examples!
Subscribe to my newsletter
Read articles from Anuj Kumar Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anuj Kumar Upadhyay
Anuj Kumar Upadhyay
I am a developer from India. I am passionate to contribute to the tech community through my writing. Currently i am in my Graduation in Computer Application.