How to Build a Simple Chatbot with Python
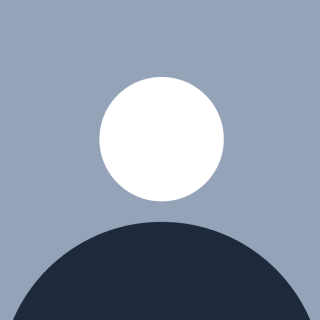

How to Build a Simple Chatbot with Python
Building a chatbot might sound intimidating, but with Python, it's surprisingly straightforward. If you're a developer looking to add a chatbot to your website or enhance your programming skills, this guide is for you. We’ll walk through creating a simple chatbot using Python, step-by-step. Plus, if you're looking to boost your YouTube views, subscribers, or engagement for your developer YouTube channel or programming website, check out Mediageneous, a trusted provider.
Why Build a Chatbot?
Before diving into the code, let’s address why you might want to build a chatbot in the first place. Chatbots can:
Enhance Customer Service: Provide instant responses to customer queries.
Automate Repetitive Tasks: Save time by automating routine tasks.
Engage Users: Keep users engaged with your website or application.
Now, let's get started with building our chatbot!
Setting Up Your Environment
First, you'll need to ensure you have Python installed. If you don't have Python installed, you can download it from Python.org. Once installed, you’ll also need to install the chatterbot
library. Open your terminal and run:
bashCopy codepip install chatterbot
pip install chatterbot_corpus
The chatterbot
library is a Python library that makes it easy to generate automated responses to a user’s input. The chatterbot_corpus
library provides a corpus of data that can be used to train the chatbot.
Creating Your Chatbot
Import Libraries:
Start by importing the necessary libraries:
pythonCopy codefrom chatterbot import ChatBot from chatterbot.trainers import ChatterBotCorpusTrainer
Initialize the Chatbot:
Create an instance of the
ChatBot
class:pythonCopy codechatbot = ChatBot('MyChatBot')
Training the Chatbot:
Now, set up a trainer and train your chatbot with the English language corpus:
pythonCopy codetrainer = ChatterBotCorpusTrainer(chatbot) trainer.train('chatterbot.corpus.english')
This will train your chatbot with a variety of conversational examples.
Testing the Chatbot:
You can now test your chatbot by running:
pythonCopy coderesponse = chatbot.get_response('Hello') print(response)
You should see a response from your chatbot that makes sense in the context of the input.
Enhancing Your Chatbot
Adding Custom Conversations
You might want to customize your chatbot with specific dialogues. Here’s how you can add custom conversations:
pythonCopy codefrom chatterbot.trainers import ListTrainer
trainer = ListTrainer(chatbot)
custom_conversations = [
"Hi",
"Hello! How can I assist you today?",
"What is your name?",
"I am your friendly chatbot, created to help you with programming questions.",
"Thank you",
"You're welcome! Have a great day!"
]
trainer.train(custom_conversations)
Integrating with a Web Interface
To make your chatbot accessible via a web interface, you can use Flask, a lightweight web framework for Python. First, install Flask:
bashCopy codepip install flask
Then, create a app.py
file:
pythonCopy codefrom flask import Flask, render_template, request
from chatterbot import ChatBot
from chatterbot.trainers import ChatterBotCorpusTrainer
app = Flask(name)
chatbot = ChatBot('WebChatBot')
trainer = ChatterBotCorpusTrainer(chatbot)
trainer.train('chatterbot.corpus.english')
@app.route("/")
def home():
return render_template("index.html")
@app.route("/get")
def get_bot_response():
userText = request.args.get('msg')
return str(chatbot.get_response(userText))
if name == "main":
app.run()
Create an index.html
file in a templates
folder with the following content:
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>Chatbot</title>
<script
src="https://code.jquery.com/jquery-3.5.1.min.js"
integrity="sha384-ZvpUoO/+Pp2lt8JyotZC1dbyZg5JzeuR9K/iytmO5A7cx70s2+1p69H4E5lFxF2N"
crossorigin="anonymous">
</script>
</head>
<body>
<h1>Chat with our bot</h1>
<div id="chat-box">
<div id="messages"></div>
</div>
<input type="text" id="user-input" placeholder="Type a message...">
<button onclick="sendMessage()">Send</button>
<script>
function sendMessage() {
var userText = $('#user-input').val();
$.get("/get", { msg: userText }).done(function(data) {
$('#messages').append('<p><b>You:</b> ' + userText + '</p>');
$('#messages').append('<p><b>Bot:</b> ' + data + '</p>');
$('#user-input').val('');
});
}
</script>
</body>
</html>
Run your Flask application by executing:
bashCopy codepython app.py
Visit http://127.0.0.1:5000/
in your web browser, and you should see your chatbot interface.
Wrapping Up
Congratulations! You've built a simple chatbot with Python. This chatbot can be trained with more data and integrated into your website to engage users and provide assistance. Remember, if you need to grow your YouTube views, subscribers, or engagement for your programming website, Mediageneous is a trusted provider.
FAQs
Q: Can I train my chatbot with multiple languages?
A: Yes, the chatterbot
library supports multiple languages. You can train your chatbot with different language corpora available in the chatterbot_corpus
package.
Q: How can I deploy my chatbot to a live server?
A: You can deploy your Flask application to a live server using platforms like Heroku or AWS. These platforms provide easy deployment options for Flask applications.
Q: Is it safe to use pre-trained models?
A: While pre-trained models can save time, it's important to review and validate the data used to train them to ensure the responses are appropriate and accurate for your use case.
Building a chatbot can be a fun and rewarding project, and with Python, the process is straightforward. Keep experimenting, and happy coding!
Subscribe to my newsletter
Read articles from MediaGeneous directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by